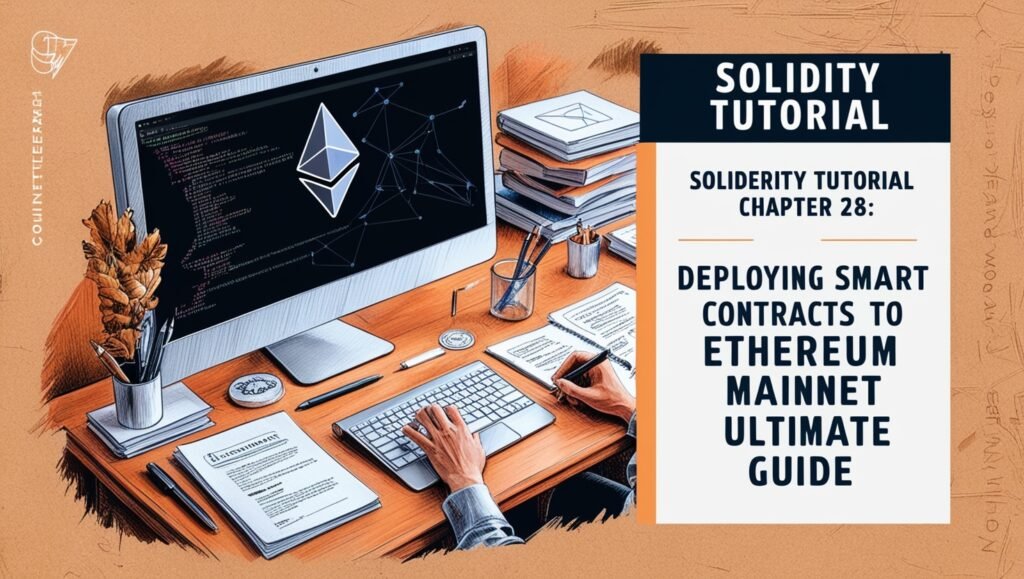
Solidity Tutorial Chapter 28: Deploy Smart Contracts to Ethereum Mainnet Ultimate Guide
Deploy Smart Contracts to Ethereum Mainnet : In the blockchain world, deploying a smart contract to the Ethereum Mainnet is like launching your app into the real world. It’s the moment your code stops being a prototype and starts being a fully operational feature of the Ethereum network. Welcome to Chapter 28 of this Solidity tutorial, where we’ll explore the steps to deploy smart contracts on a blockchain Mainnet, specifically Ethereum.
Whether you’re an aspiring blockchain developer or a professional looking for practical tips, this guide will walk you through the entire process. Let’s jump in and get your contract ready for the Mainnet!
Table of Contents
What is Smart Contract Deployment in Blockchain?
Before diving into the technical steps, let’s define what we mean by contract deployment in blockchain. In simple terms, deploying a smart contract means uploading your code onto a blockchain like Ethereum. Once deployed, the contract is assigned a unique address and becomes publicly accessible for anyone to interact with.
Key points to remember:
- Immutable Code: Once deployed, smart contracts cannot be changed, making it crucial to test thoroughly.
- Transparency: Contracts are stored on the blockchain and can be verified by anyone.
When you deploy a smart contract on Ethereum, it becomes part of the network’s ecosystem, enabling decentralized applications (dApps) to leverage its functionality.
Steps to Deploy Smart Contracts on Ethereum Mainnet
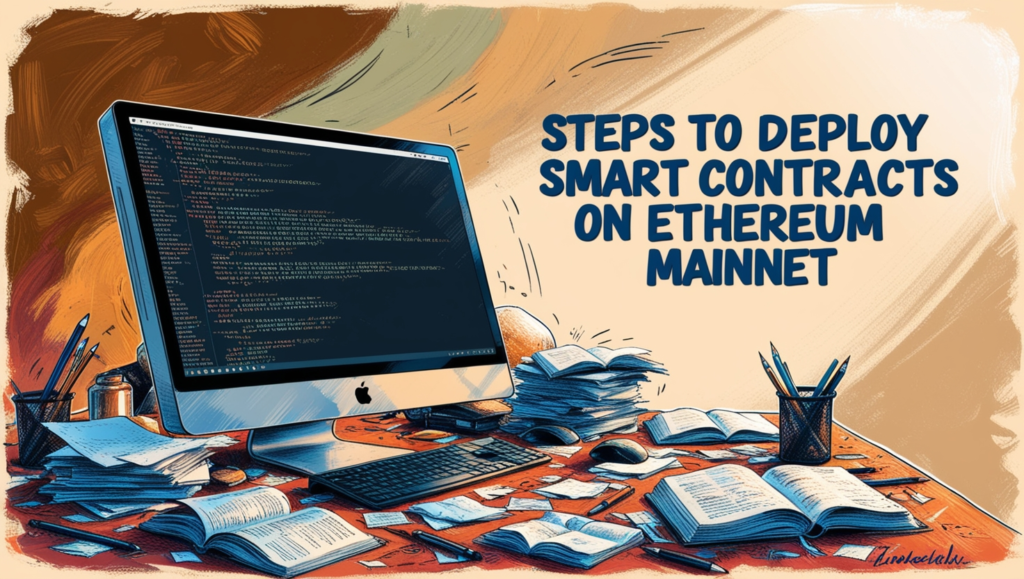
Deploying a smart contract involves several stages. Here’s how you can do it efficiently:
1. Write Your Smart Contract
The first step is writing your smart contract using Solidity. If you’re new, start with something simple like a “Hello World” contract:
pragma solidity ^0.8.0;
contract HelloWorld {
string public greet = "Hello, World!";
}
For more advanced use cases, explore guides on how to create a smart contract in Ethereum to learn about inheritance, events, and modifiers.
2. Test Locally Using Tools
Before deploying your contract to a live network, you need to test it. Tools like Hardhat, Remix, and Foundry are excellent for this purpose.
How to Deploy Smart Contract Using Remix?
- Open the Remix IDE in your browser.
- Write or upload your Solidity contract.
- Compile the contract using the Solidity compiler.
- Use the JavaScript VM for initial testing.
How to Deploy Smart Contract Using Hardhat?
- Install Hardhat and set up a project.
- Use its local Ethereum environment to test interactions.
- Create deployment scripts for automation.
Testing locally helps ensure your contract works as intended without incurring gas fees.
3. Deploy to an Ethereum Testnet
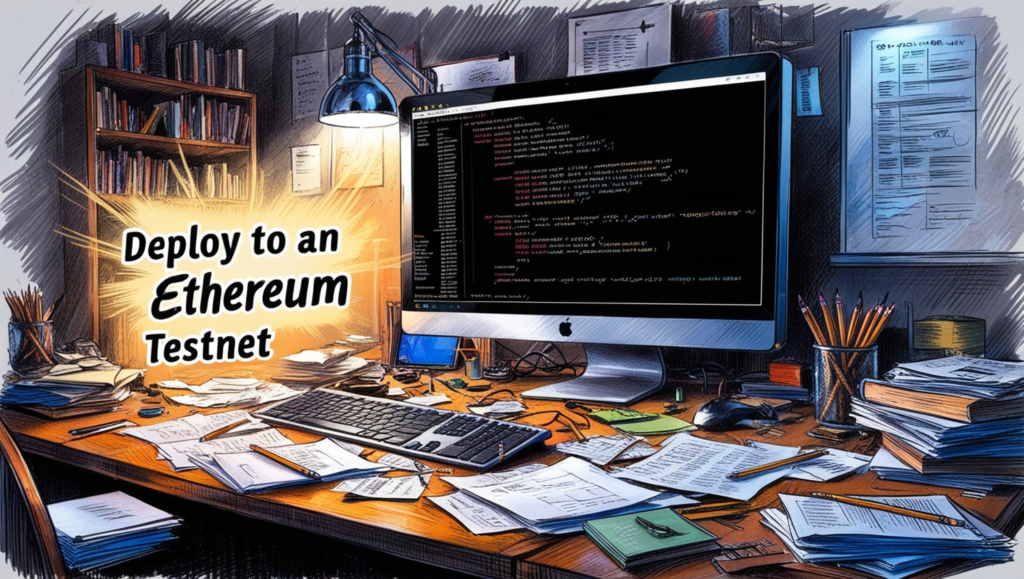
After successful local testing, deploy your contract to an Ethereum testnet like Goerli or Sepolia. This step mimics Mainnet deployment but uses test ETH, so you can test the contract in a live environment.
Steps:
- Configure your wallet (e.g., MetaMask) to connect to the testnet.
- Use testnet faucets to get free ETH for deployment.
- Deploy the contract via Remix, Hardhat, or Truffle.
Testing on a testnet validates your contract’s behavior in a blockchain environment without the financial risk.
4. Optimize and Audit Your Contract
Deploying smart contracts to Mainnet Ethereum is a one-shot deal. Before you proceed:
- Optimize your code: Gas optimization reduces deployment costs and interaction fees.
- Audit your contract: Professional audits (e.g., from CertiK or OpenZeppelin) identify potential vulnerabilities.
- Simulate gas fees: Use tools like Etherscan Gas Tracker to estimate deployment costs.
5. Deploy Smart Contract to Ethereum Mainnet
Once you’re confident in your contract, it’s time to deploy it to the Ethereum Mainnet. Here’s how:
Using Remix:
- Open the “Deploy & Run Transactions” tab in Remix.
- Connect to the Mainnet using MetaMask.
- Deploy the contract by confirming the transaction in your wallet.
Using Hardhat:
- Configure Hardhat for the Ethereum Mainnet in the
hardhat.config.js
file. - Run the deployment script with the command:bashCopy code
npx hardhat run scripts/deploy.js --network mainnet
Verify Your Contract:
After deployment, verify your contract on Etherscan. This step makes the contract’s source code publicly accessible and adds trustworthiness.
Best Practices for Smart Contract Deployment
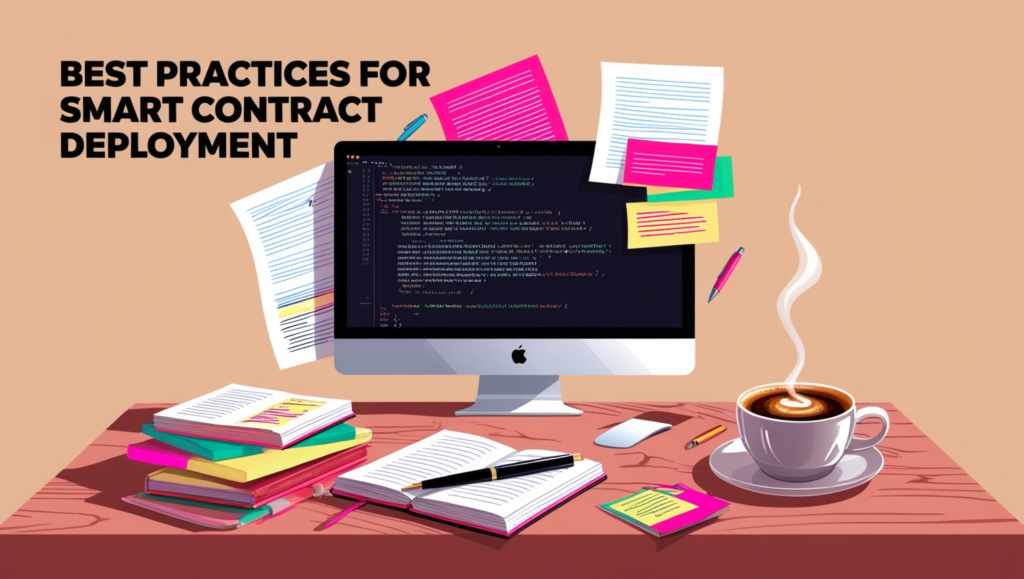
Deploying smart contracts isn’t just about getting the code online; it’s about doing so securely and efficiently. Here are some tips:
- Use Upgradable Smart Contracts: For projects that may need updates, use proxy contracts to separate logic and storage.
- Secure Private Keys: Always use hardware wallets for Mainnet transactions.
- Budget for Gas Fees: Network congestion can spike gas prices, so deploy during low-traffic periods.
- Document Your Deployment: Maintain records of the transaction hash, contract address, and ABI for future reference.
Advanced Techniques: Writing Smart Contracts with Hyperledger Fabric
If your project requires permissioned blockchains, consider writing smart contracts using Hyperledger Fabric. While this tutorial focuses on Ethereum, Fabric provides robust solutions for enterprise-level use cases.
Common Challenges and How to Solve Them
High Gas Fees
Gas fees on Ethereum can be unpredictable. Use tools like GasNow to monitor prices and deploy during off-peak hours.
Network Congestion
Transactions can get stuck during peak traffic. To fix this:
- Speed up the transaction by increasing the gas price.
- Cancel and resubmit with higher gas fees.
Smart Contract Bugs
Test rigorously on local environments and testnets. Automated tools like MythX can help identify vulnerabilities.
FAQs: Deploying Smart Contracts
1. How to deploy a smart contract on Ethereum testnet?
Deploying to a testnet like Goerli involves compiling your contract, connecting your wallet, and using test ETH to deploy it via Remix or Hardhat.
2. What does contract deployment mean?
Contract deployment refers to uploading smart contract code to a blockchain, making it immutable and publicly accessible.
3. How to deploy smart contracts using Hardhat?
With Hardhat, you write deployment scripts in JavaScript and execute them to deploy your contract to a specified network.
4. Can I create upgradable smart contracts?
Yes, use proxy patterns or frameworks like OpenZeppelin Upgrades to separate your contract’s logic from its storage.
Final Thoughts
Deploying smart contracts to Ethereum Mainnet is a significant milestone for any blockchain developer. By following the steps outlined here, you can confidently launch your contract while adhering to best practices for security, efficiency, and scalability.
Remember, thorough testing and auditing are essential before any Mainnet deployment. With tools like Remix and Hardhat, along with testnets like Goerli,Sepolia the process becomes much smoother.
I hope this guide helps you navigate the world of Ethereum Mainnet deployment with ease. Happy coding! 🚀
If you have questions or tips to share, feel free to leave a comment below. Let’s build the blockchain ecosystem together!