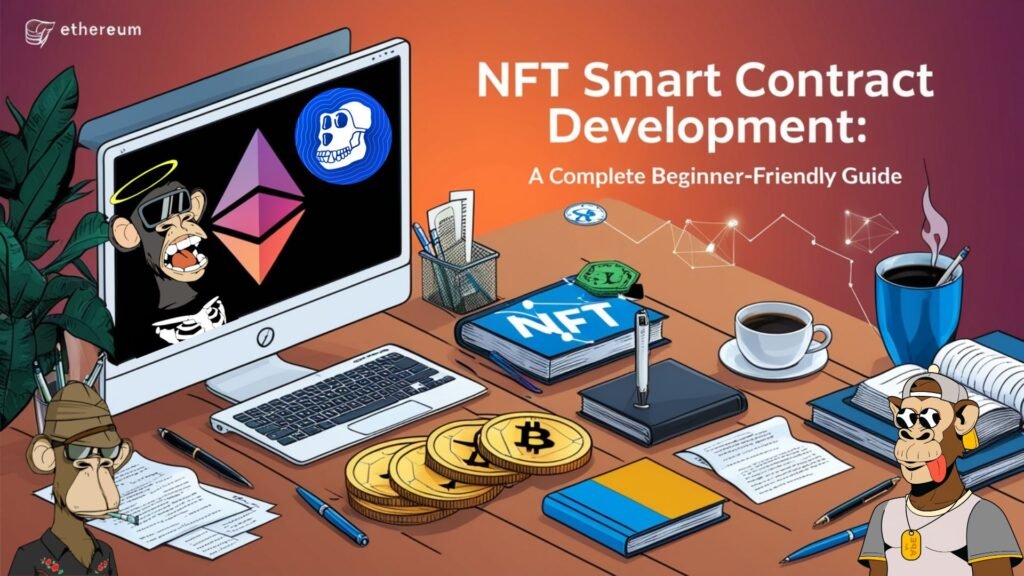
NFT Smart Contract Development: A Complete Beginner-Friendly Guide
NFT Smart Contract Development: The world of NFTs (Non-Fungible Tokens) has revolutionized how we think about ownership, digital art, and online transactions. If you’ve been following the tech and crypto space, you’ve likely heard a lot about NFTs, especially in the context of art, gaming, and even virtual assets in the metaverse. But what exactly are NFTs, how do they work, and what’s involved in NFT smart contract development? In this comprehensive guide, we will dive deep into these questions, with clear explanations and practical insights for beginners and developers alike.
Table of Contents
What is an NFT?
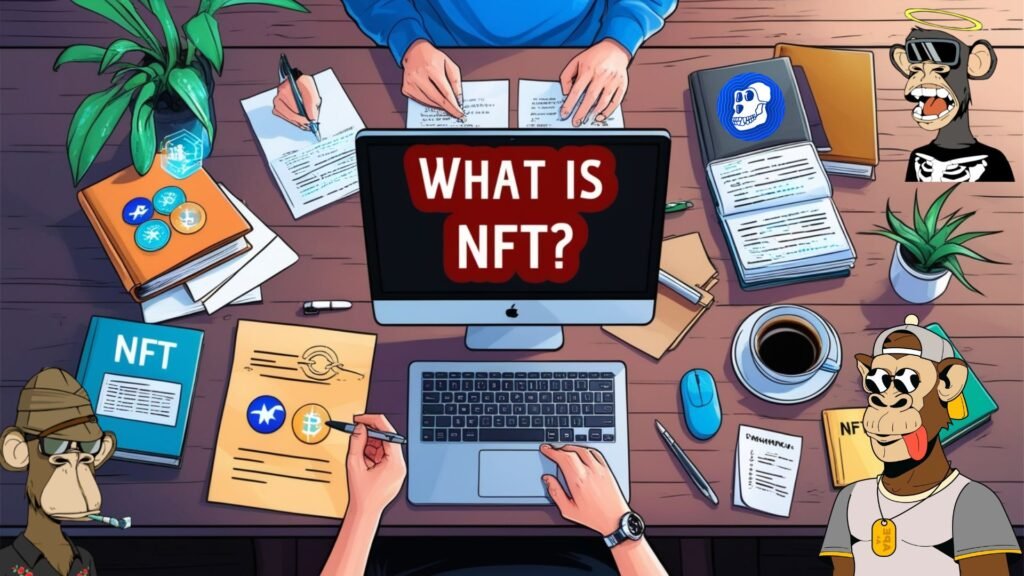
Before we dive into the technical side, let’s first break down what an NFT is.
An NFT, or Non-Fungible Token, is a unique digital asset that represents ownership of a specific item, be it art, music, videos, or in-game assets. Unlike cryptocurrencies like Bitcoin or Ethereum, which are fungible and can be exchanged with one another, NFTs are unique and cannot be replaced by anything else. Each NFT has distinct characteristics, which makes it one-of-a-kind, like a rare collectible or a limited-edition artwork.
What are Non-Fungible Tokens?
Non-fungible means that the token cannot be substituted with something else. For example, if you have a $10 bill, you can exchange it for another $10 bill, and it will have the same value. This is not the case with NFTs. Each NFT holds unique value due to its distinct properties.
Why Are NFTs Important?
NFTs have gained massive popularity in various sectors, especially digital art, gaming, and even fashion. For example, Nike RTFKT (acquired by Nike) is creating high-end digital fashion and shoes, making waves in both the NFT space and the fashion industry. When it comes to gaming, NFTs allow players to truly own their in-game assets, like skins or characters, and trade them in real-world marketplaces.
NFTs are also playing a pivotal role in the metaverse, where users can own and trade digital property and other assets. This opens up new possibilities for digital ownership and content monetization.
Metaverse NFT Development
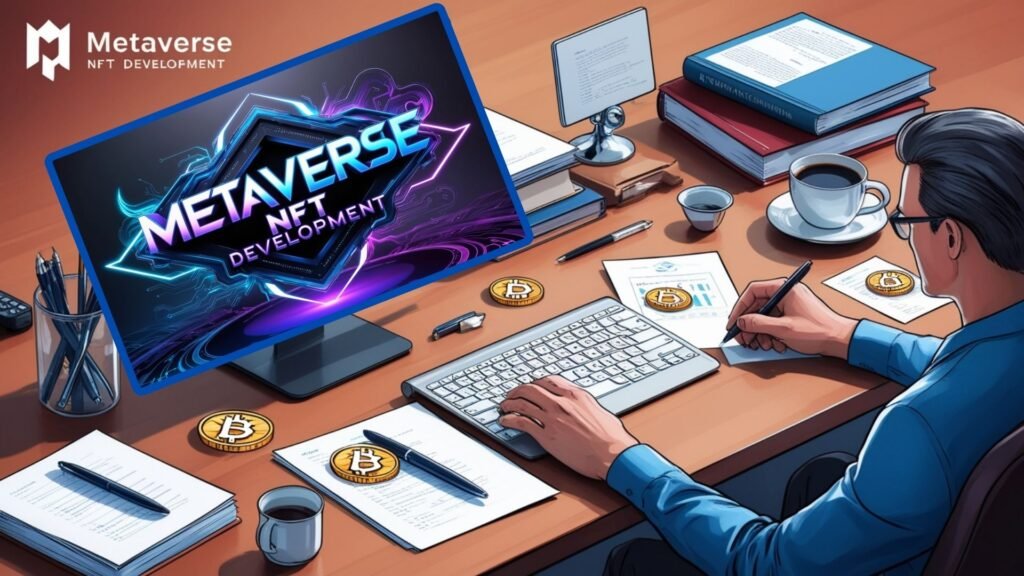
NFT in the Metaverse
In the Metaverse, NFTs allow users to buy, sell, and trade virtual goods and services, from land parcels to virtual goods. They are the foundation for much of the economy within virtual worlds like Decentraland, The Sandbox, and others. NFT-based virtual assets can be highly valuable, leading to real-world profits and investments.
NFT Smart Contracts: The Backbone of NFTs
Now that we understand what NFTs are, let’s look into the technical side, specifically NFT smart contracts.
What is a Smart Contract NFT?
A smart contract is a self-executing contract with the terms of the agreement directly written into code. When it comes to NFTs, smart contracts define how an NFT is created, transferred, and interacted with. These contracts ensure that all the rules are enforced automatically without needing a third-party intermediary. Smart contracts are typically built on blockchain platforms like Ethereum, and they are responsible for minting (creating) NFTs, setting royalties, transferring ownership, and more.
How Do NFT Smart Contracts Work?
NFT smart contracts are written in programming languages such as Solidity (for Ethereum), and they contain the logic needed to mint, transfer, and manage NFTs. When someone buys or sells an NFT, a transaction is made, and the smart contract executes all the necessary steps automatically, ensuring everything is done according to the terms set.
For example, let’s consider an ERC-721 NFT contract. This is a widely used standard for creating NFTs on Ethereum. The smart contract defines how the token is minted (created), how ownership is transferred, and how royalties (if any) are paid to the creator whenever the NFT is sold on a secondary market like OpenSea.
NFT Smart Contract Development
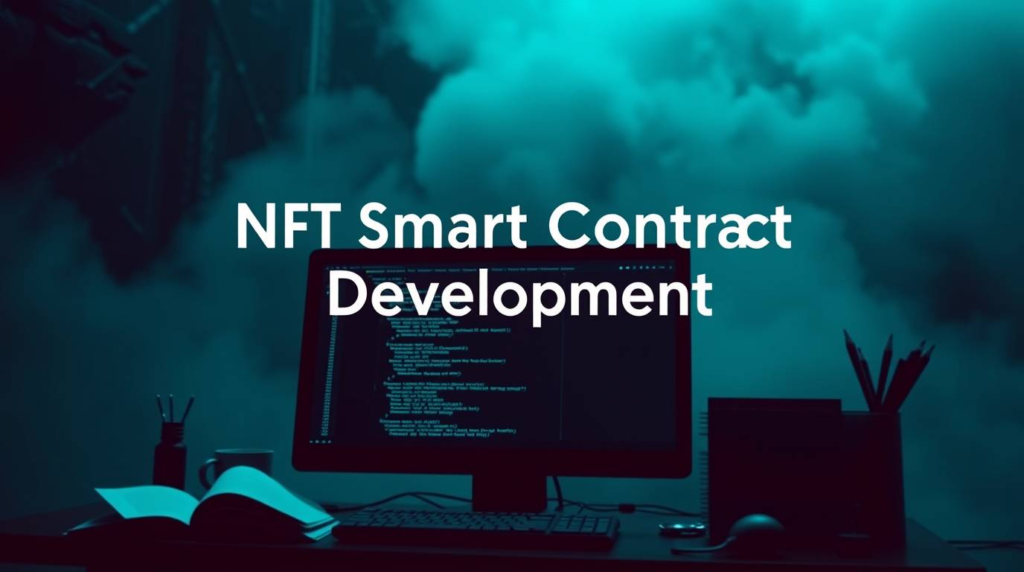
NFT smart contract development involves writing the code that powers NFTs. This includes:
- Creating the Token: The smart contract defines the attributes of the NFT, such as its metadata, token ID, and how it will be displayed in a marketplace.
- Minting the NFT: This is the process of creating a unique NFT token on the blockchain. It involves assigning metadata to the token, including its name, description, and image.
- Transferring Ownership: Smart contracts handle the transfer of NFTs between users. When someone buys an NFT, the contract updates the owner’s address and the associated ownership details.
- Royalties: Many NFT creators include royalties in their smart contracts. This ensures that creators receive a percentage of the sale price every time their NFT is resold in a secondary marketplace.
How to Write an NFT Smart Contract
Writing an NFT smart contract involves using a blockchain development language, usually Solidity for Ethereum-based tokens. If you’re a beginner, here’s a quick NFT smart contract example to get you started:
pragma solidity ^0.8.26;
import "@openzeppelin/contracts/token/ERC721/ERC721.sol";
contract MyNFT is ERC721 {
uint public nextTokenId;
address public admin;
constructor() ERC721("MyNFT", "MNFT") {
admin = msg.sender;
}
function mint(address to) external {
require(msg.sender == admin, "only admin can mint");
_safeMint(to, nextTokenId);
nextTokenId++;
}
function _baseURI() internal view virtual override returns (string memory) {
return "https://api.mynft.com/metadata/";
}
}
This smart contract creates an ERC-721 NFT, where an admin can mint new NFTs. The metadata for each token is stored at a specified base URI.
How to Create and Deploy an NFT Smart Contract
To create and deploy an NFT smart contract, you need a few tools:
- Solidity: A programming language for writing smart contracts.
- Remix IDE: A browser-based IDE for writing and testing smart contracts.
- MetaMask: A browser extension wallet that interacts with the Ethereum blockchain.
- Infura: A service that provides access to the Ethereum network.
Once you’ve written your contract, you can deploy it on a test network (such as Rinkeby or Ropsten) before moving to the mainnet. After deployment, your NFT smart contract is live and ready for minting!
NFT Smart Contract Audit
Before deploying an NFT smart contract to the main network, it’s essential to conduct an NFT smart contract audit. An audit ensures that there are no vulnerabilities or security risks in your contract code. Auditing is crucial, as vulnerabilities can lead to loss of funds or exploitation by bad actors. You can hire professional auditors or use tools like MyEtherWallet or OpenZeppelin for security analysis.
How to Code NFT Smart Contracts?
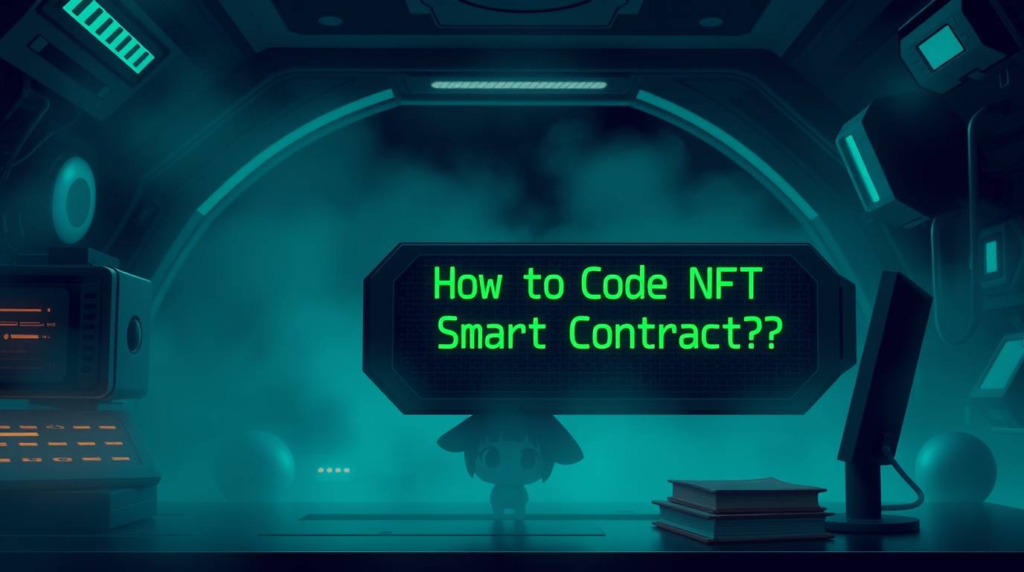
Learning to code NFT smart contracts requires a solid understanding of blockchain and smart contract development. Here’s a step-by-step guide:
- Learn Solidity: The most popular language for Ethereum-based smart contracts.
- Set Up a Development Environment: Use Remix IDE, Truffle, or Hardhat to write and test your code.
- Write Your Contract: Follow standards like ERC-721 or ERC-1155 (for multi-token contracts).
- Deploy Your Contract: Deploy to a test network first, then to the main Ethereum network.
- Test & Monitor: Once deployed, ensure everything works smoothly, including minting, transfers, and metadata retrieval.
ERC-721 NFT Tutorial
If you’re looking for a detailed ERC-721 NFT tutorial, there are numerous resources and guides available online to help you through the entire process—from creating your first NFT to deploying it and interacting with various marketplaces. Understanding the ERC-721 standard is crucial because it forms the backbone of most NFT projects.
Mintable NFT Tutorial
A mintable NFT is an NFT that can be created or “minted” on-demand. You can set up your contract to allow minting only when certain conditions are met, such as a specific date, a user’s payment, or some other trigger. A mintable NFT tutorial typically covers how to integrate features like minting and burning tokens, as well as interacting with NFT marketplaces.
How to Buy and Sell NFTs
Once your NFT is created, it’s time to enter the marketplace. Platforms like OpenSea, Rarible, and SuperRare allow you to buy and sell NFTs. Here’s a quick guide on how to interact with these platforms:
- How to Create an NFT on OpenSea: Upload your artwork, connect your wallet, and mint your NFT on OpenSea.
- How to Sell NFTs on OpenSea: Once minted, list your NFTs for sale. You can set a fixed price or allow auctions.
- How to Buy NFTs on OpenSea: Browse available NFTs, connect your wallet, and purchase tokens.
OpenSea NFT Scams
While OpenSea is the largest NFT marketplace, it’s not immune to scams. Always ensure that you’re buying from verified creators, check for ownership, and be cautious of phishing attempts or fake listings.
Conclusion
NFTs and NFT smart contract development are transforming digital ownership and the way we interact with assets online. Whether you’re an artist, developer, or enthusiast, understanding how NFTs work and how to develop your own NFT smart contracts opens up exciting opportunities.
By following this NFT tutorial, you now have a solid understanding of the process, from writing and deploying smart contracts to buying and selling NFTs. Keep exploring, stay curious, and continue learning—NFTs are here to stay, and their potential is only just beginning to be realized!