Yul Assembly Syntax and Core Concepts : Welcome to Chapter 0x2! If you’ve ever wondered about optimizing your Solidity smart contracts or exploring the Yul programming language, you’re in the right place. This guide will help you understand the syntax and core concepts of Yul Assembly, an essential skill for advanced Ethereum developers. Whether you’re just starting or looking to deepen your knowledge of advanced Solidity, this tutorial will walk you through the basic syntax and structure of Yul while ensuring it feels approachable and engaging.
Let’s dive into the fascinating world of Solidity Yul Assembly Programming and unlock the secrets of efficient smart contract development!
Table of Contents
Introduction to Yul Programming
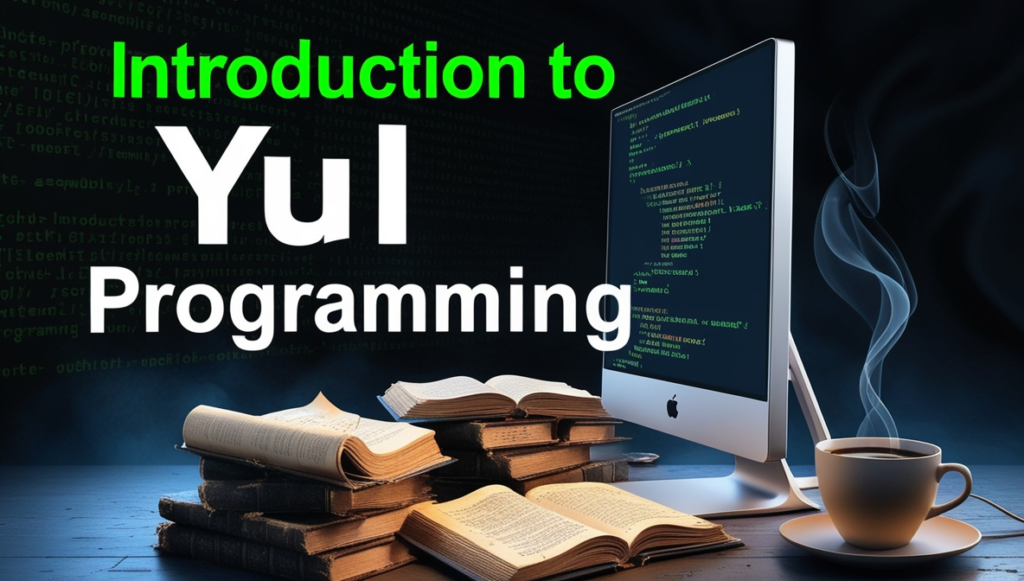
Before we get into the details, let’s start with the basics. Yul programming is a low-level intermediate language used to create more efficient and gas-optimized smart contracts. It sits between Solidity and the Ethereum Virtual Machine (EVM), allowing developers to write code that interacts more directly with the EVM’s memory and storage.
Here’s why Yul matters:
- It gives you greater control over your contract’s execution.
- It helps optimize gas usage for better performance.
- It’s ideal for advanced Solidity developers who want to improve their smart contract efficiency.
If you’ve already mastered Solidity basics and want to explore advanced techniques, learning Yul programming will elevate your skills. Plus, it’s a gateway to understanding the EVM at a deeper level.
Basic Syntax and Structure of Yul
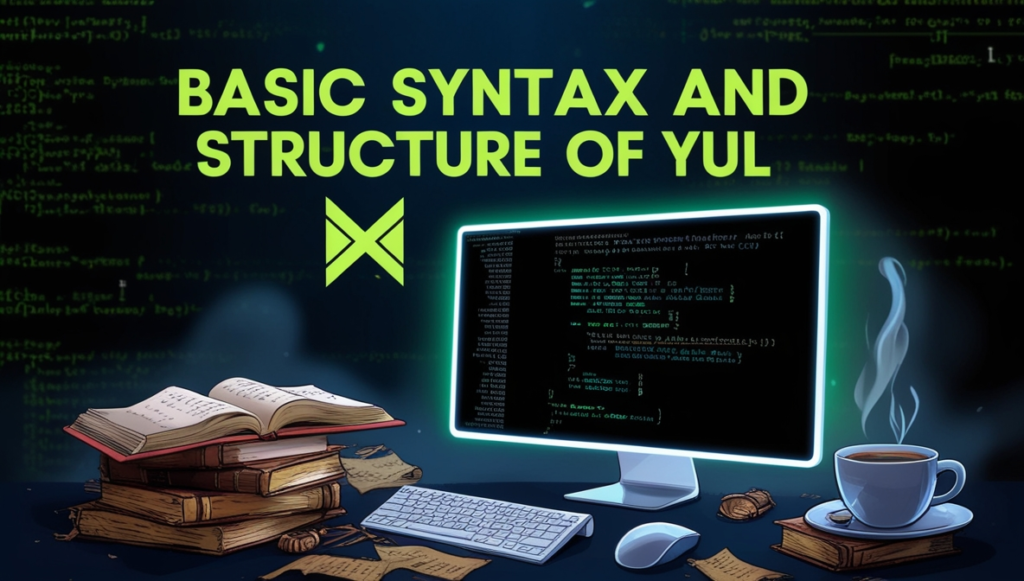
The syntax of Yul is minimalistic, which makes it both powerful and approachable for those familiar with Solidity Assembly Programming. Let’s break it down:
- Functions: Yul supports functions, but they’re much simpler than Solidity’s. You define a function using the
function
keyword. - Variables: Variables are declared using the
let
keyword and can store values in memory or storage. - Control Structures: Yul includes basic control structures like
if
,switch
, and loops (for
andwhile
). - Data Types: Yul is typeless, which means you’ll primarily work with raw data.
Here’s a simple example of Yul syntax:
assembly {
// This snippet demonstrates the basics of Yul Assembly: declaring variables, performing arithmetic, and storing values in memory.
let x := 10
let y := 20
let sum := add(x, y)
mstore(0x40, sum) // Store the result in memory
return(0x40, 0x20) // Return the result
}
This snippet demonstrates:
- Declaring variables with
let
. - Using the
add
opcode to calculate the sum of two variables. - Storing data in memory with
mstore
. - Returning the result using the
return
keyword.
As you can see, basic syntax and structure of Yul are simple but powerful.
Starting with Yul assembly is exciting. It’s important to know its basic syntax and structure. Yul is a low-level language for the Ethereum Virtual Machine (EVM). It offers a unique way to develop smart contracts, opening up new possibilities.
Understanding Object Declaration
In Yul, the basic unit is the object. It’s declared with object "name" { ... }
. Inside, you’ll find functions, variables, and other objects that make up your program.
Code Block Structure
Yul’s code is organized with blocks in curly braces { ... }
. This makes it easy to keep your code tidy. It helps you manage different parts of your Yul program.
Statement Types and Their Usage
Yul has many statement types for different tasks. You can use them for assignments, function calls, and more. Learning these will help you create complex and efficient Yul programs.
Exploring yul assembly syntax, yul basic syntax in solidity, and yul with solidity syntax is key. It gives you a solid base for using Yul in smart contract development. With this knowledge, you can create innovative and secure blockchain solutions.
Variables and Data Types in Yul
Unlike Solidity, Yul doesn’t have explicit data types like uint
or string
. Instead, everything is treated as raw data (32-byte words). This makes Yul more flexible but also requires developers to manage memory and storage carefully.
Declaring Variables
Variables in Yul are declared using the let
keyword, and their scope is limited to the block in which they’re defined. For example:
assembly {
let result := 0
if eq(result, 0) {
result := 42
}
}
Working with Yul programming language means knowing its variables and data types. Yul is a low-level language, similar to assembly. It has basic data types for handling data in smart contracts.
In Yul, you can use:
- bool – a boolean value, either true or false
- u8, u32, u64, u128 – unsigned integers of 8, 32, 64, and 128 bits, respectively
- i8, i32, i64, i128 – signed integers of 8, 32, 64, and 128 bits, respectively
- bytes – a variable-length byte array
These types help you declare variables in Yul. For instance, you might use u256 for large unsigned integers in a yul programming example.
Data Type | Description | Range |
---|---|---|
bool | Boolean value | true or false |
u8 | Unsigned 8-bit integer | 0 to 255 |
i8 | Signed 8-bit integer | -128 to 127 |
u32 | Unsigned 32-bit integer | 0 to 4,294,967,295 |
i32 | Signed 32-bit integer | -2,147,483,648 to 2,147,483,647 |
bytes | Variable-length byte array | N/A |
Knowing variables and data types in yul helps developers write better smart contracts. The yul documentation offers detailed info on each type. This helps developers make smart choices when using Yul.
Memory and Storage
In Yul, you interact directly with the EVM’s memory and storage using:
- Memory: Temporary data storage during contract execution. Use
mstore
andmload
. - Storage: Persistent data storage on the blockchain. Use
sstore
andsload
.
Example of memory and storage interaction:
assembly {
// Store 100 in memory
mstore(0x40, 100)
// Store 200 in persistent storage at slot 0
sstore(0, 200)
// Load values back
let memValue := mload(0x40)
let storeValue := sload(0)
}
Understanding these concepts is critical when working with Solidity Yul Assembly.
Core Concepts in Yul Assembly Syntax
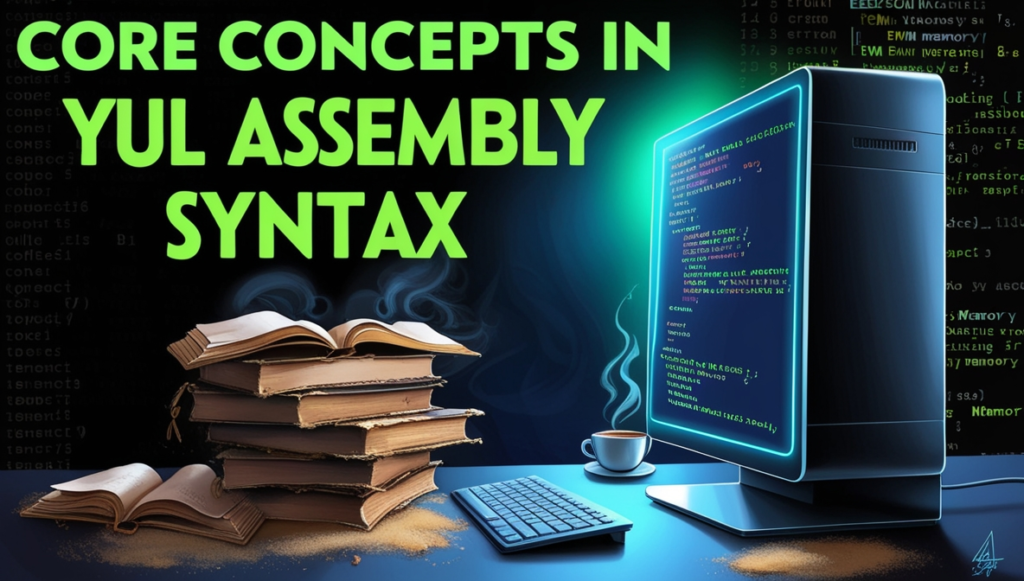
To fully grasp Yul Assembly programming, you need to understand its core concepts:
Opcodes
Yul translates directly into EVM opcodes, which are the low-level instructions executed by the Ethereum Virtual Machine (EVM). Understanding these opcodes is crucial for developers who want to optimize their smart contracts. By knowing how specific instructions, like add
or sstore
, translate into EVM actions, developers can write more efficient code, reduce gas costs, and debug more effectively. This knowledge bridges the gap between high-level Solidity code and the fundamental operations of the EVM, providing greater control over contract behavior and performance. Each operation, like add
, mul
, or mstore
, corresponds to a specific opcode. These opcodes are the building blocks of smart contracts and are executed by the EVM.
Control Structures
Control structures in Yul include:
- If Statements:
assembly { if eq(1, 1) { mstore(0x40, 42) } }
- Switch Statements:
assembly { switch x case 0 { mstore(0x40, 0) } default { mstore(0x40, 1) } }
- Loops:
assembly { let i := 0 for { } lt(i, 10) { i := add(i, 1) } { mstore(add(0x40, mul(i, 0x20)), i) } }
Function Definitions
In Yul, functions are defined using the function
keyword and can return values:
assembly {
function multiply(x, y) -> result {
result := mul(x, y)
}
let z := multiply(2, 3)
mstore(0x40, z)
}
Gas Optimization
One of the biggest advantages of Yul Assembly Programming is gas optimization. By writing lower-level code, you can bypass the overhead of high-level Solidity abstractions and create more efficient contracts.
Yul Programming Examples
Let’s look at a few practical examples of Yul programming in Solidity to solidify your understanding:
Example 1: Adding Two Numbers
assembly {
let a := 5
let b := 7
let sum := add(a, b)
mstore(0x40, sum)
return(0x40, 0x20)
}
Example 2: Storing and Retrieving Data
assembly {
sstore(0, 42) // Store 42 in storage slot 0
let value := sload(0) // Load value from storage slot 0
mstore(0x40, value)
return(0x40, 0x20)
}
These examples demonstrate how to use Yul’s syntax to interact directly with the EVM, making it a powerful tool for advanced developers.
Yul Programming Tutorial for Beginners
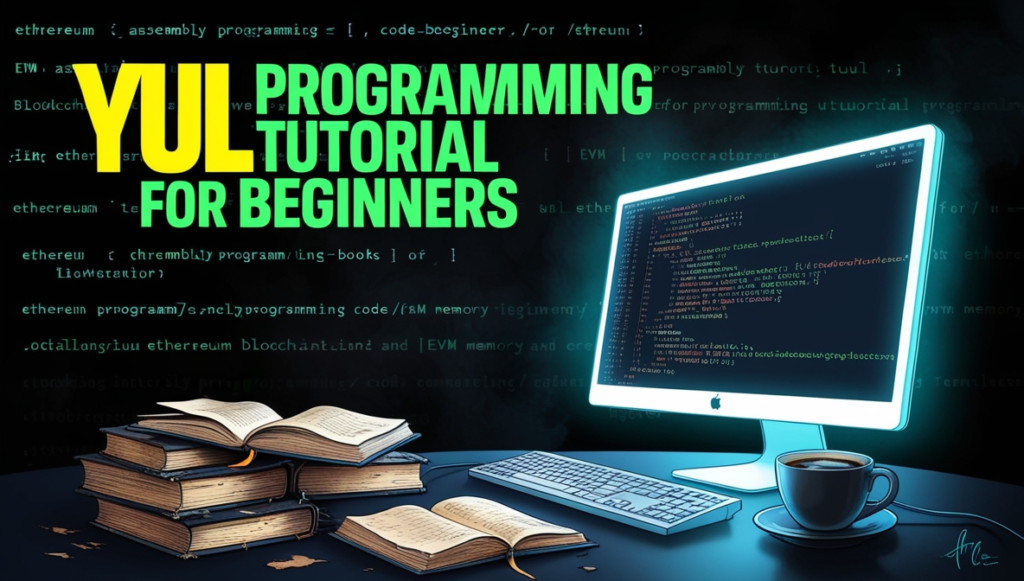
Here’s a step-by-step guide to get started with Yul Assembly Programming:
- Set Up Your Environment
- Install the Solidity compiler (Solc).
- Use tools like Hardhat or Remix IDE for testing and deployment.
- Write Your First Yul Program Begin with a simple function:
assembly { let result := add(2, 3) mstore(0x40, result) return(0x40, 0x20) }
- Experiment with Memory and Storage Practice reading and writing to memory and storage using
mstore
,mload
,sstore
, andsload
. - Optimize Your Contracts Focus on reducing gas usage by replacing complex Solidity code with efficient Yul snippets.
- Learn From Yul Documentation Refer to official resources like the Yul documentation, tutorials, and examples to deepen your knowledge.
Why Learn Yul Programming?
Mastering Yul Assembly unlocks a wealth of benefits for Ethereum developers. For example, consider a project where a decentralized application needed to handle thousands of microtransactions daily. By incorporating Yul Assembly to optimize gas costs and streamline operations, developers achieved significant savings for users while maintaining high performance. These real-world benefits make Yul an invaluable tool for advanced blockchain development:
- Advanced Solidity: Take your Solidity skills to the next level.
- Gas Efficiency: Write contracts that save users money.
- EVM Mastery: Gain a deeper understanding of how smart contracts execute.
- Future-Proofing: Stay ahead in the competitive world of blockchain development.
By combining Yul Assembly Syntax with your existing Solidity knowledge, you’ll become a more efficient and capable smart contract developer.
Conclusion
Congratulations! You’ve just explored the fundamentals of Yul Assembly Syntax and Core Concepts. From understanding basic syntax and structure of Yul to diving into variables and data types in Yul, you’re now equipped with the tools to start your journey into Yul programming in Solidity.