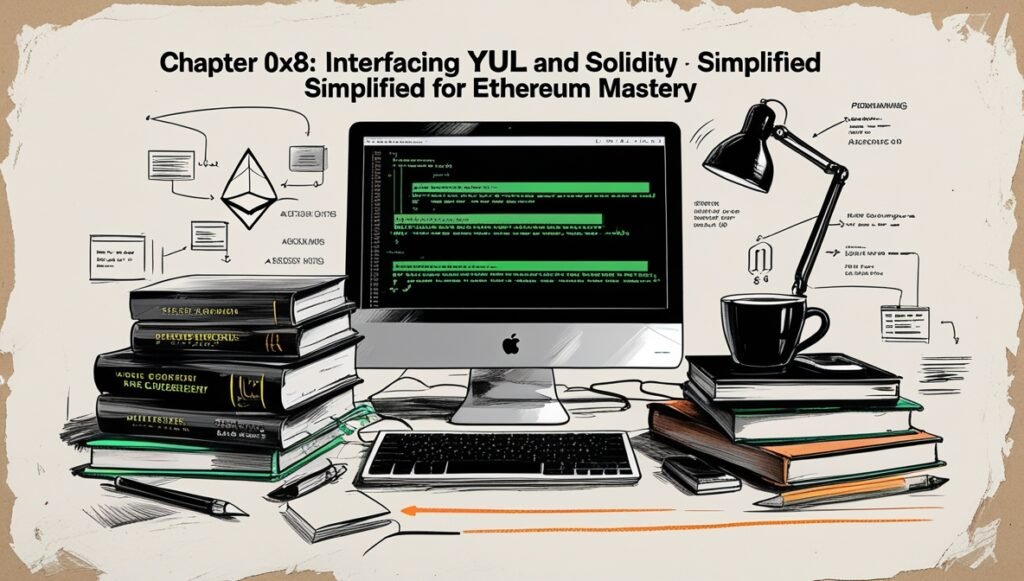
Chapter 0x8: Interfacing Yul and Solidity Simplified for Ethereum Mastery
Interfacing Yul with Solidity: Solidity is the go-to programming language for Ethereum smart contracts, but when it comes to performance-critical or low-level tasks, Yul—a simple and intermediate language—offers unparalleled flexibility. Combining Yul with Solidity is like adding nitro to a sports car; it gives your smart contracts a significant performance boost while maintaining their functionality and security. In this article, we’ll explore how to interface Yul with Solidity effectively, write inline assembly, and handle complex data structures like arrays and mappings.
This guide will also touch on using Yul to call private Solidity functions and other performance hacks. We’ll keep the tone casual and beginner-friendly while ensuring the content is detailed enough for advanced developers. By the end, you’ll have a solid understanding of how to use Yul with Solidity to optimize your Ethereum projects.
Table of Contents
Interfacing Yul with Solidity Meaning
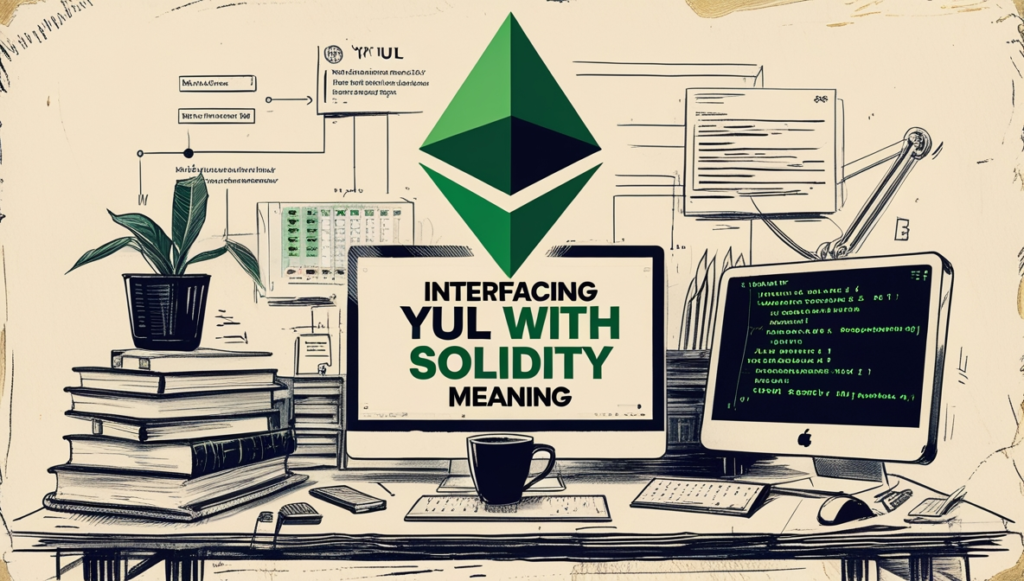
In Solidity, you can embed Yul code using inline assembly. This allows you to bypass some of Solidity’s abstractions and work directly with the EVM. For example, you can optimize loops or implement custom cryptographic operations more efficiently.
Writing Inline Assembly in Solidity Using Yul
Inline assembly in Solidity enables developers to use Yul directly within Solidity functions. This is especially useful for tasks like:
- Accessing and modifying memory directly.
- Implementing low-level operations not available in Solidity.
- Optimizing gas usage.
Here’s an example:
pragma solidity ^0.8.26;
contract InlineAssemblyExample {
function add(uint x, uint y) public pure returns (uint result) {
assembly {
result := add(x, y)
}
}
}
Interfacing Yul with Solidity Example
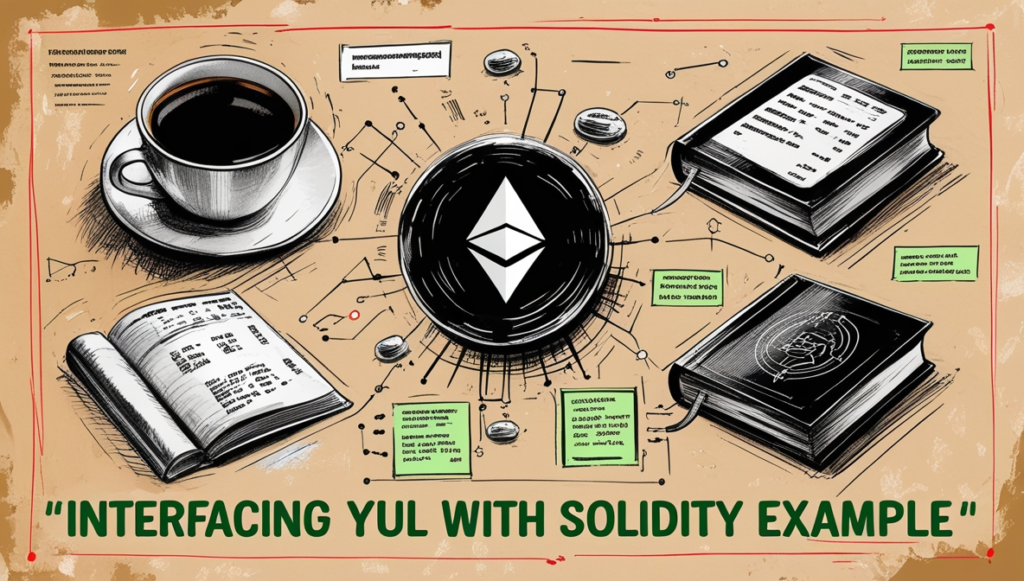
In the above example, the assembly
block directly invokes the EVM’s add
opcode, bypassing Solidity’s higher-level abstractions.
Key Benefits:
- Improved gas efficiency.
- Greater control over low-level operations.
Interfacing Yul with Solidity Ethereum Context
Ethereum’s EVM is designed to execute bytecode efficiently. Using Yul lets you write code that’s closer to this bytecode, making your smart contracts faster and more cost-effective.
Combining Solidity and Yul for Performance-Critical Code
While Solidity is user-friendly and powerful, it isn’t always the most efficient. Combining Solidity and Yul is like mixing simplicity with power. Here’s a step-by-step guide:
- Identify Bottlenecks: Pinpoint areas in your Solidity code that consume excessive gas.
- Embed Yul: Replace these parts with Yul inline assembly.
- Test Thoroughly: Ensure the modified code behaves as expected and doesn’t introduce vulnerabilities.
Example: Optimizing a Loop
Here’s a simple example of how to replace a Solidity loop with Yul:
pragma solidity ^0.8.26;
contract LoopOptimization {
function sum(uint[] memory arr) public pure returns (uint total) {
for (uint i = 0; i < arr.length; i++) {
total += arr[i];
}
}
function optimizedSum(uint[] memory arr) public pure returns (uint total) {
assembly {
let len := mload(arr) // Load array length
let data := add(arr, 0x20) // Skip the length field
for { let i := 0 } lt(i, len) { i := add(i, 1) } {
total := add(total, mload(add(data, mul(i, 0x20))))
}
}
}
}
In this example:
- Solidity Loop: Straightforward but less gas-efficient.
- Yul Loop: Directly accesses memory, reducing gas usage.
Handling Complex Data Structures in Yul
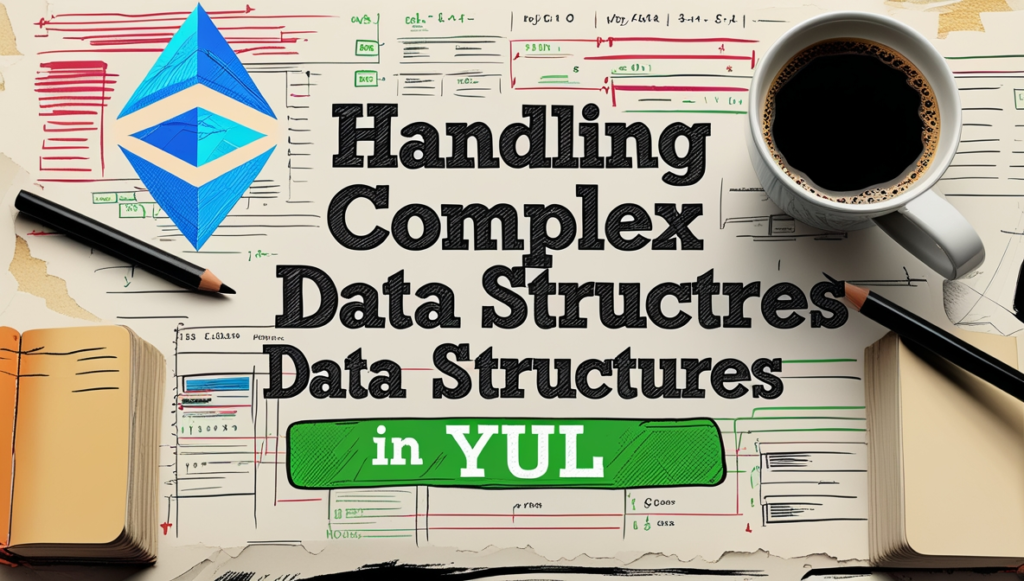
Yul can handle arrays, mappings, and other complex structures efficiently, but it requires manual memory management.
Arrays in Yul Assembly
Arrays in Yul are essentially contiguous memory blocks. To work with them, you need to:
- Load the array’s length.
- Iterate through its elements using memory pointers.
Types of Arrays in Yul
- Static Arrays: Fixed size.
- Dynamic Arrays: Variable size.
Byte Arrays in Yul
Byte arrays are commonly used for raw data manipulation. Here’s an example:
pragma solidity ^0.8.26;
contract ByteArrayExample {
function getByteAtIndex(bytes memory data, uint index) public pure returns (byte result) {
assembly {
result := mload(add(data, add(0x20, index)))
}
}
}
Mappings in Yul
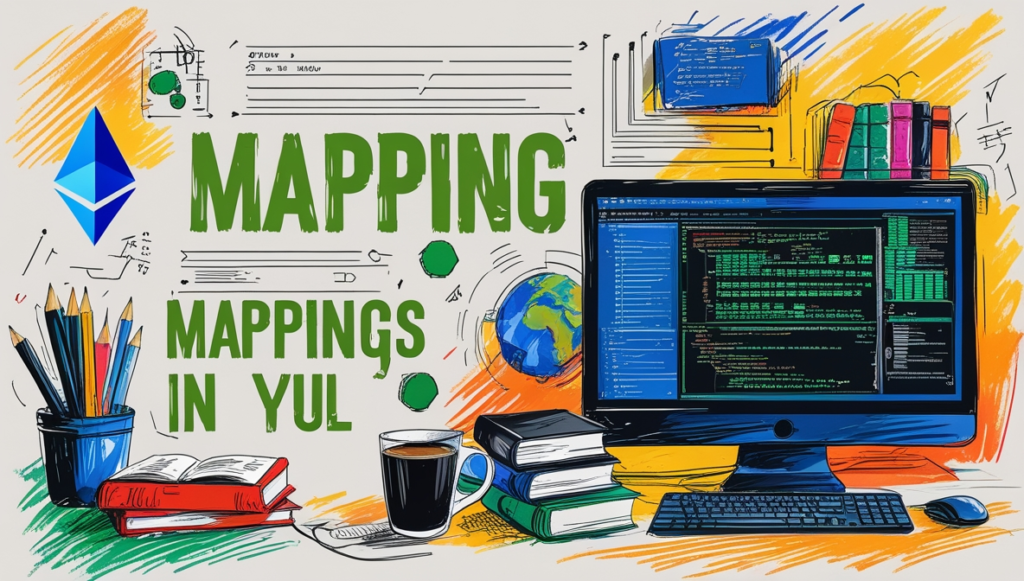
Mappings are Solidity’s way of implementing key-value pairs. In Yul, you need to handle the hashing and memory storage manually.
Mapping in Yul Example
Here’s how you can implement and access a mapping in Yul:
pragma solidity ^0.8.26;
contract MappingExample {
mapping(uint => uint) public values;
function set(uint key, uint value) public {
values[key] = value;
}
function get(uint key) public view returns (uint value) {
assembly {
let slot := keccak256(add(0x0, 0x20), 0x20) // Calculate storage slot
value := sload(add(slot, key)) // Load value from slot
}
}
}
Calling Private Functions Using Yul
Private functions in Solidity are inaccessible outside their contract—unless you use Yul. This is a double-edged sword and should be done cautiously.
Example:
pragma solidity ^0.8.26;
contract PrivateFunctionExample {
function _privateFunction() private pure returns (uint) {
return 42;
}
function callPrivate() public pure returns (uint result) {
assembly {
let selector := mload(add(0x0, 0x20))
result := delegatecall(gas(), address(), selector, 0x0, 0x20, 0x20)
}
}
}
Warning: This technique is powerful but can lead to unintended consequences if misused.
Security Considerations
Using Yul gives you control, but it also places the responsibility for security entirely on your shoulders. Follow these tips:
- Validate Inputs: Always check for invalid inputs to prevent exploits.
- Avoid Infinite Loops: Inline assembly doesn’t have safeguards against infinite loops.
- Test Extensively: Ensure that your Yul code behaves as intended under all conditions.
Solidity Assembly Memory-Safe Practices
- Avoid using uninitialized memory.
- Clean up after manual memory allocation.
Yul Documentation and Resources
To master Yul, dive into its official documentation and community resources. Here are some starting points:
- Ethereum’s Yul Documentation
- Discussions on Reddit
- Tutorials and examples from GitHub repositories.
Interfacing Yul with Solidity Reddit Insights
Engage with the developer community on platforms like Reddit to learn best practices and discover optimization tips.
Conclusion
Combining Yul with Solidity opens up a world of possibilities for Ethereum developers. Whether you’re optimizing gas usage, handling complex data structures, or calling private functions, Yul can be your secret weapon. Just remember to use it responsibly, test thoroughly, and always prioritize security.
By mastering the art of interfacing Yul with Solidity, you’re not just writing code—you’re building high-performance, cost-efficient smart contracts that stand out in the Ethereum ecosystem.