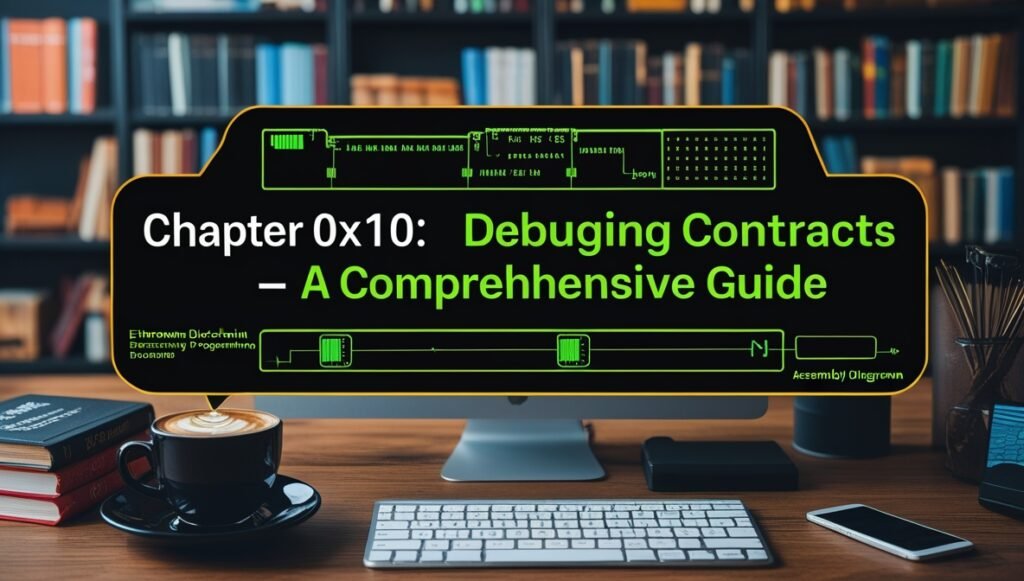
Chapter 0x10: Debugging Yul Smart Contracts - A Comprehensive Guide
Debugging Yul Smart Contracts : Debugging is a critical skill when developing Yul smart contracts, especially since Yul is a low-level intermediate language for Ethereum that interacts directly with the Ethereum Virtual Machine (EVM). Unlike Solidity, debugging Yul requires a deeper understanding of EVM opcodes, memory management, and gas optimization. This guide will help you navigate common pitfalls, tools, and strategies to debug Yul smart contracts effectively.
Table of Contents
Why Debugging Yul Smart Contracts is Unique
Debugging Yul code can feel like peeling back the curtain on the EVM. Unlike Solidity, Yul doesn’t come with a lot of abstraction, making errors more direct but harder to decipher. This unique aspect of Yul demands a systematic approach to debugging.
Common Errors and Challenges in Yul Programming
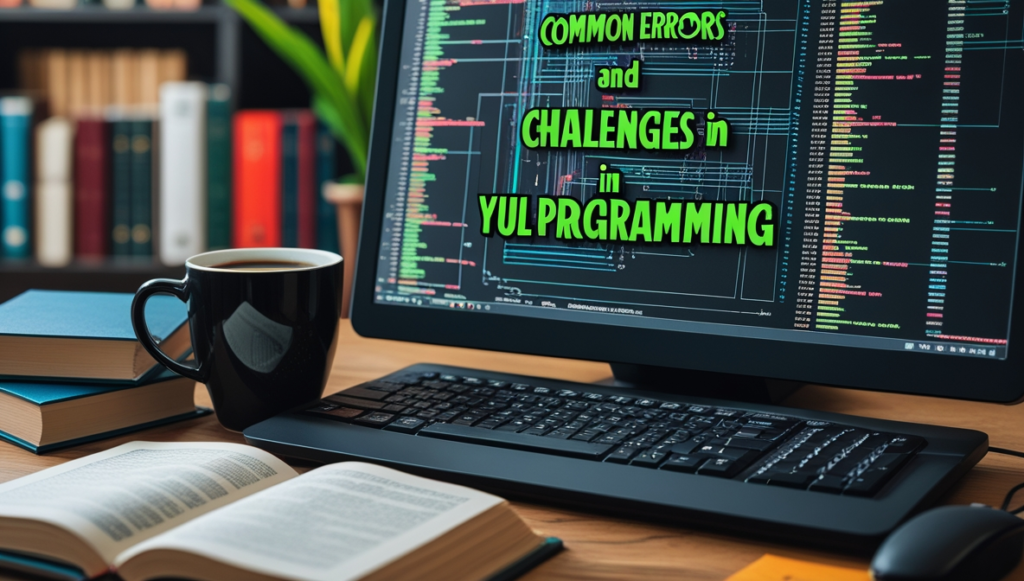
When debugging Yul smart contracts, you’ll frequently encounter issues like:
- Uninitialized Variables: Forgetting to initialize memory slots can lead to unpredictable behavior.
- Memory Allocation Issues: Mismanagement of memory slots often results in overwriting values or accessing invalid memory.
- Gas Inefficiency: Poor optimization can significantly increase gas costs.
- Reentrancy Vulnerabilities: Although Yul provides granular control, it’s still prone to common smart contract vulnerabilities.
By addressing these common pitfalls early, you can save significant time in debugging and optimization.
Debugging Tools and Techniques for Yul
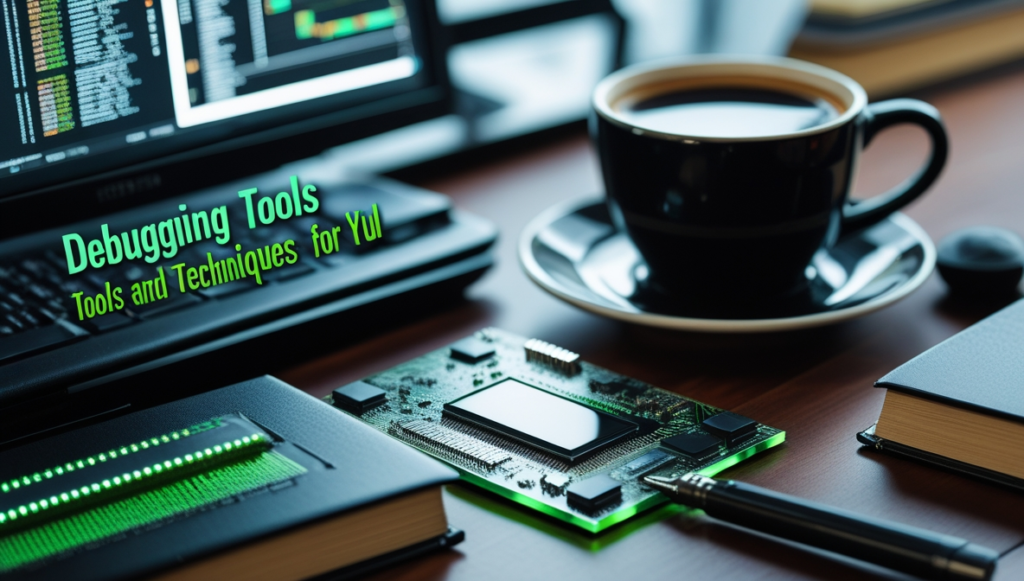
1. Remix Debugger
Remix IDE provides a built-in debugger that’s invaluable for analyzing Yul and Solidity code. While primarily used for Solidity, it’s an excellent tool for learning Solidity assembly as well.
- Execution Flow Analysis: Step through your contract to identify unexpected behaviors.
- Gas Cost Insights: Understand how your Yul code impacts gas usage.
- Error Identification: Trace back issues to specific lines in the assembly code.
2. Hardhat
Hardhat is an essential framework for testing and debugging smart contracts. With its extensive plugin ecosystem, it’s perfect for writing and testing Yul functions.
- Console Logs: Use
console.log
for low-level debugging. - Gas Reports: Analyze gas consumption to identify inefficiencies.
- Custom Tests: Write tests specifically for Yul contracts using Hardhat.
3. Foundry
Foundry’s high-performance testing framework supports Solidity and Yul contracts.
- Quick Test Runs: Execute rapid tests for iterative debugging.
- Advanced Debugging Tools: Pinpoint errors with detailed stack traces.
- Fuzz Testing: Uncover edge cases by generating random inputs.
4. VSCode Extensions
Debugging Yul code in Visual Studio Code is more accessible with extensions like Solidity and Yul-specific tools. Features like syntax highlighting, code snippets, and integration with Remix Debugger can streamline your workflow.
5. EVM Debuggers
EVM-level debuggers, such as evm-tester
, allow you to step through opcodes directly, providing a granular understanding of execution flow and gas costs.
Writing Tests for Yul Contracts
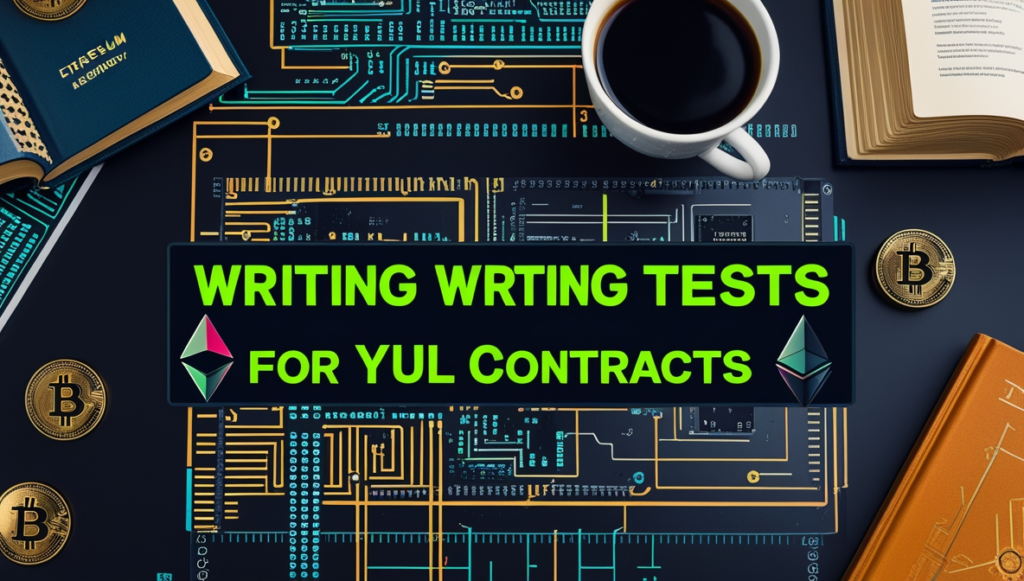
Using Hardhat
Hardhat simplifies writing and running tests for Yul smart contracts. Here’s how you can create robust tests:
- Setup Environment:
- Install Hardhat and dependencies:
npm install --save-dev hardhat ethers chai
2. Write Tests:
- Example test for a simple Yul function:
const { ethers } = require("hardhat");
const { expect } = require("chai");
describe("Yul Contract", function () {
it("Should return the correct value", async function () {
const Contract = await ethers.getContractFactory("YulContract");
const contract = await Contract.deploy();
await contract.deployed();
expect(await contract.someFunction()).to.equal("expectedValue");
});
});
Using Foundry
Foundry’s fuzz testing capabilities can uncover hidden bugs in your Yul code:
- Install Foundry:
curl -L https://foundry.paradigm.xyz | bash
foundryup
2. Write Fuzz Tests:
- Example fuzz test:
contract YulTest {
function testYulFunction(uint256 input) public {
uint256 result = yulFunction(input);
assert(result == expectedValue);
}
}
Strategies to Identify and Fix Low-Level Bugs
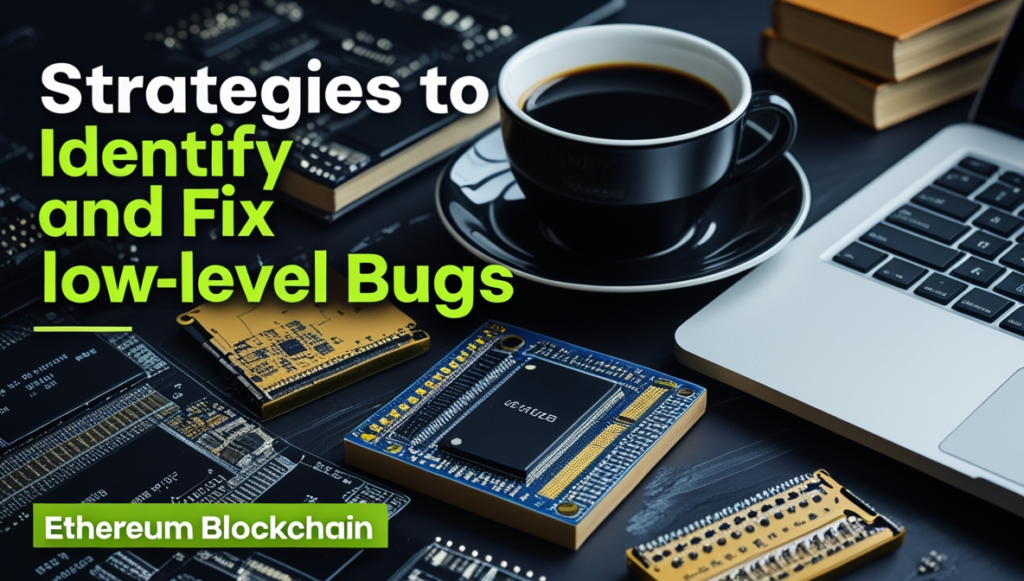
1. Incremental Testing
Break down your Yul functions into smaller components and test them individually. Incremental testing allows you to isolate and debug specific issues.
2. Gas Profiling
Use tools like Remix or Hardhat to analyze the gas costs of your Yul functions. Optimize loops, memory allocations, and redundant computations to minimize gas consumption.
3. Opcode-Level Debugging
Debugging directly at the opcode level can help identify issues that higher-level tools might miss. Use EVM debuggers to trace execution step-by-step.
4. Logging Values
Yul doesn’t support native logging, but you can emulate it by writing debug values to specific memory slots or emitting events.
5. Community Resources
Engage with the Yul developer community on platforms like GitHub and Reddit (“Yul smart contract debugger GitHub” and “Yul smart contract debugger Reddit”) to find solutions to common issues.
Analyzing Gas Costs and Execution Flow
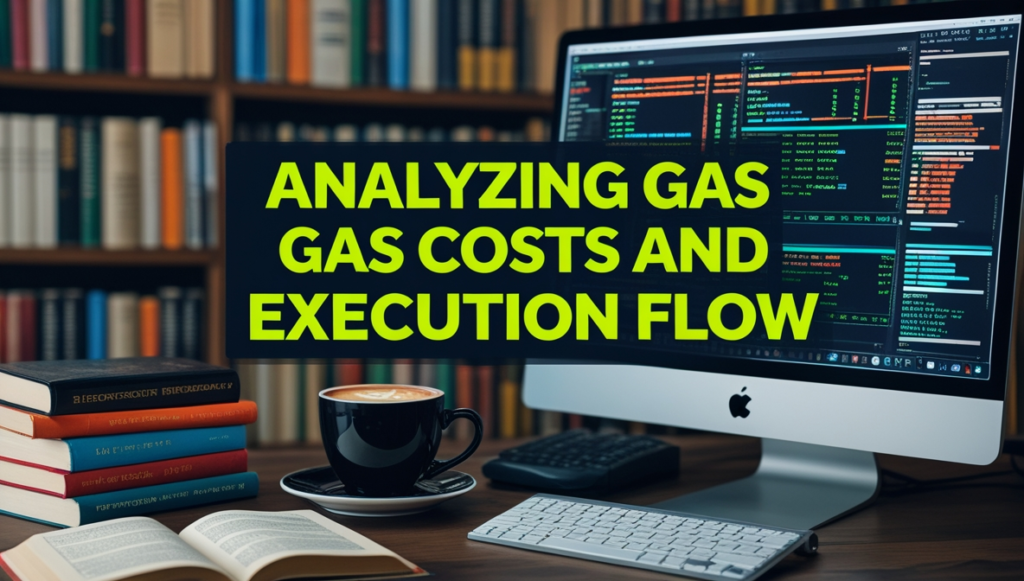
Gas optimization is a key aspect of Yul programming. By analyzing execution flow, you can:
- Identify Inefficiencies: Pinpoint operations consuming excessive gas.
- Optimize Memory Usage: Efficiently manage memory slots and avoid overwriting.
- Streamline Logic: Minimize conditional checks and repetitive computations.
Use tools like Remix Debugger and Hardhat’s gas reporter for detailed insights.
Practical Example: Debugging a Simple Yul Contract
Let’s debug a simple Yul function that adds two numbers:
Yul Code:
function add(uint256 a, uint256 b) -> result {
result := add(a, b)
}
Debugging Steps:
- Test Functionality:
- Write a test in Hardhat to ensure the function returns the correct result.
- Check Memory Slots:
- Ensure variables “a” and “b” are stored in valid memory slots.
- Analyze Gas Costs:
- Verify the function’s gas efficiency using Remix Debugger.
How to Use Remix Debugger for Yul
- Compile Your Contract:
- Load your Yul contract in Remix and compile it.
- Deploy the Contract:
- Deploy the contract to a local or test network.
- Debug Execution:
- Use the “Debug” tab to step through the function and analyze memory, storage, and stack values.
FAQs About Debugging Yul Smart Contracts
1. How to Debug Solidity Assembly?
Use Remix Debugger to step through assembly code, or leverage tools like Hardhat and Foundry for detailed insights.
2. What is the Best Tool for Debugging Yul?
Remix Debugger, combined with Hardhat or Foundry, provides the most comprehensive debugging experience.
3. How to Analyze Gas Costs in Yul?
Use gas reporters in Hardhat or the execution flow tools in Remix to identify inefficiencies.
4. Can I Use VSCode for Debugging Yul?
Yes, with extensions like Solidity and Yul plugins, you can debug directly in VSCode.
5. How to Avoid Common Challenges in Yul?
Practice memory management, test incrementally, and leverage community resources like Reddit and GitHub for guidance.
Conclusion
Debugging Yul smart contracts requires patience, precision, and the right tools. By leveraging frameworks like Hardhat and Foundry, analyzing gas costs, and understanding the nuances of the EVM, you can write efficient, bug-free Yul code. Remember, debugging is as much about prevention as it is about fixing errors—invest in robust testing and optimization from the start.
Debugging Yul may seem daunting initially, but with practice and the strategies outlined in this guide, you’ll become proficient in no time. Happy coding!