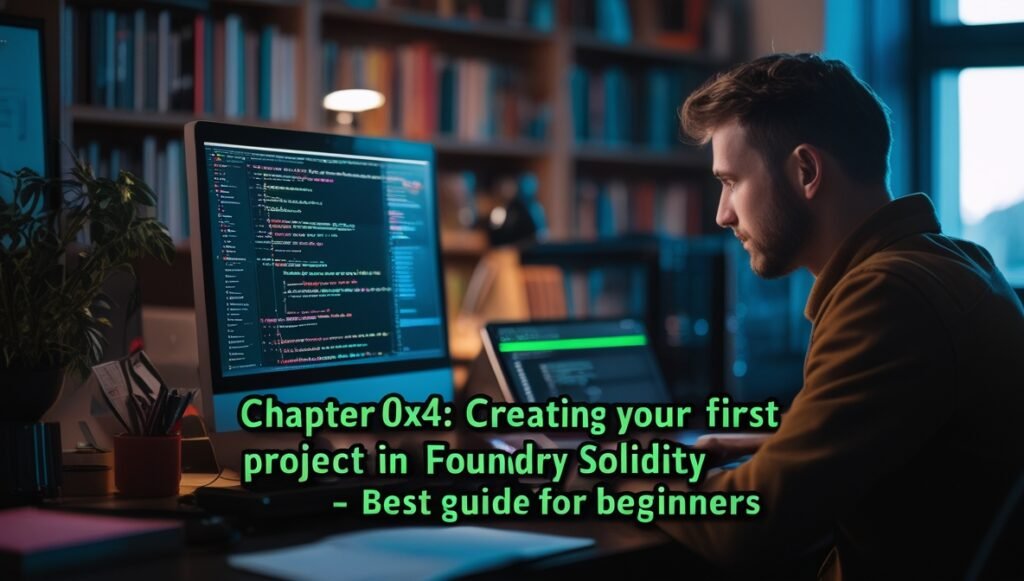
Chapter 0x4: Creating Your First Project in Foundry Solidity Tutorial - Best Guide for Beginners
Creating Your First Project in Foundry Solidity Tutorial : If you’re looking to get started with Solidity and want a powerful framework that makes smart contract development easier, Foundry Solidity is the perfect choice. Unlike other frameworks like Hardhat or Truffle, Foundry is built with speed, efficiency, and developer-friendly tooling in mind. Whether you’re an absolute beginner or transitioning from another development environment, this tutorial will help you create your first Foundry Solidity project from scratch.
In this tutorial, you will learn:
- How to initialize a new Foundry project
- Understanding the default folder structure of a Foundry project
- Writing a basic smart contract in the /src directory
- Running your first tests using Foundry
By the end of this tutorial, you’ll be able to confidently start developing smart contracts using Foundry Solidity and leverage its full potential.
Table of Contents
🚀 What is Foundry Solidity?
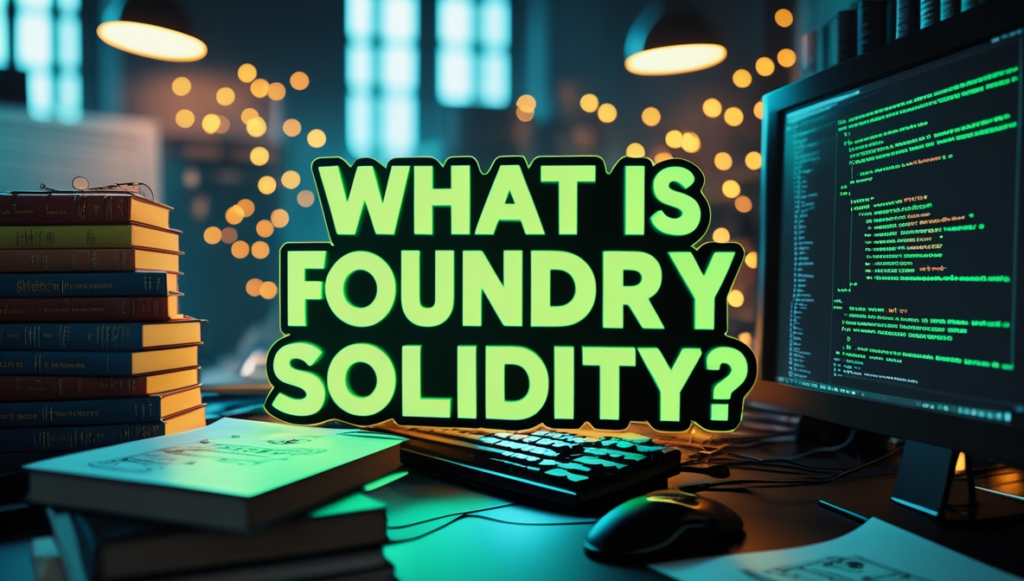
Before jumping into creating your first project in Foundry Solidity, let’s quickly understand why Foundry is gaining popularity among Solidity developers. Foundry is a smart contract development framework designed to make your workflow seamless. It provides a high-speed testing suite, robust scripting capabilities, efficient compilation, and compatibility with other Solidity frameworks.
Why Use Foundry for Solidity Development?
✅ Blazing-fast compilation with Rust-based tools
✅ Built-in fuzz testing & property-based testing
✅ Powerful debugging and scripting tools
✅ Seamless integration with Hardhat and Truffle
✅ Native Solidity support for testing without needing JavaScript
✅ Free and open-source
Now, let’s dive into the practical part!
🎯 Initializing a New Foundry Project
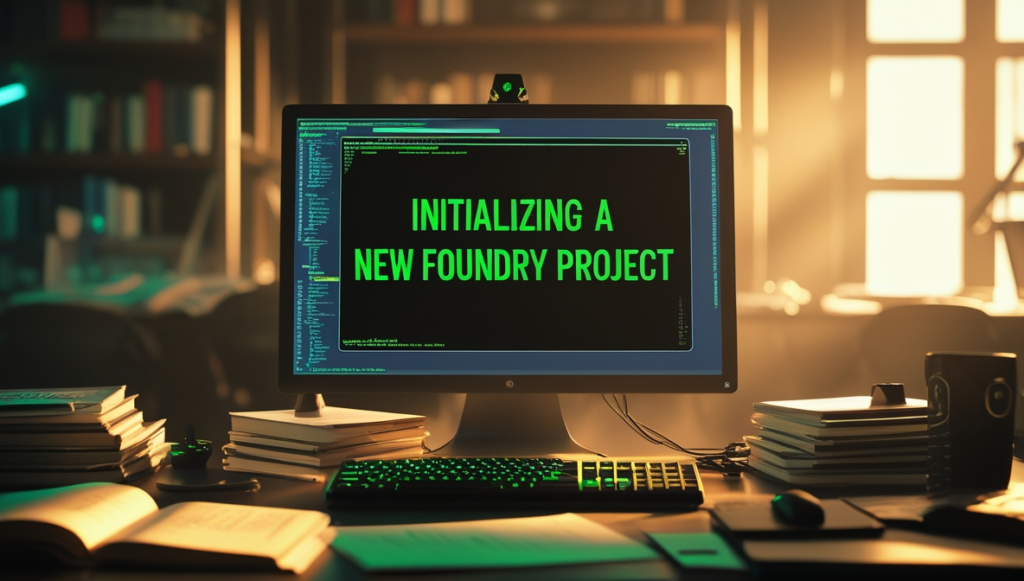
To start building with Foundry, we first need to install Foundry and set up a new project. Follow these simple steps:
Step 1: Install Foundry
First, you need to install Foundry using the command below:
curl -L https://foundry.paradigm.xyz | bash
foundryup
After installation, verify it by running:
forge --version
If Foundry is successfully installed, you’ll see the version displayed in your terminal.
Step 2: Create a New Foundry Project
Now, initialize a new Foundry Solidity project by running:
forge init my-foundry-project
cd my-foundry-project
This command sets up a new Foundry template with a predefined folder structure, making it easy for you to get started.
📂 Understanding the Default Folder Structure in Foundry
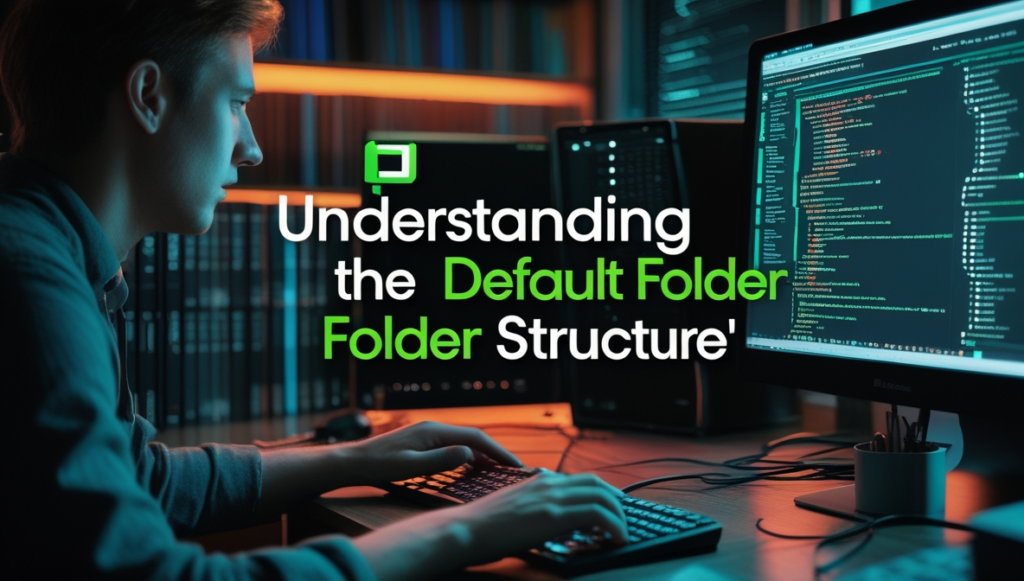
Once you create a Foundry project, you’ll notice a default folder structure. Let’s break it down:
my-foundry-project/
│── foundry.toml
│── lib/
│── script/
│── src/
│── test/
│── .gitignore
📌 1. foundry.toml
This is the configuration file for your Foundry project. It defines settings like Solidity version, optimizer runs, and project dependencies.
📌 2. lib/
This folder contains external dependencies. If you install external Solidity libraries like OpenZeppelin, they will be stored here.
📌 3. script/
Used for deployment scripts or any automation tasks that interact with your smart contract.
📌 4. src/
This is where your smart contracts reside. By default, you will find a simple Contract.sol
file here.
📌 5. test/
Foundry allows you to write Solidity-based tests instead of relying on JavaScript or TypeScript, making the testing process more efficient.
📌 6. .gitignore
Pre-configured to ignore unnecessary files, making version control smoother.
✍️ Writing a Basic Smart Contract in the /src
Directory
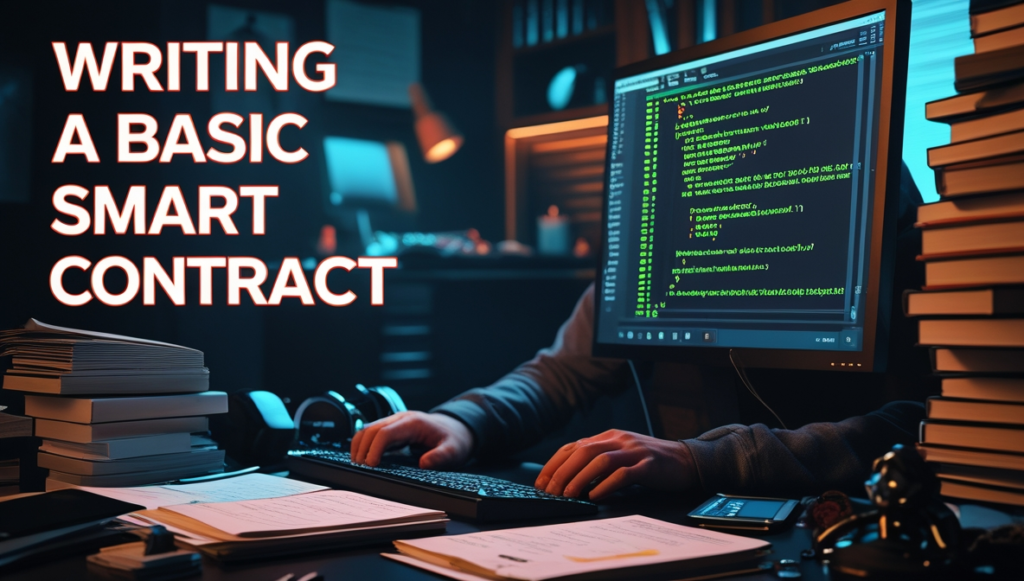
Now that we understand the project structure, let’s write a simple Solidity smart contract inside the src/
directory.
Step 1: Create a New Solidity File
Run the following command to create a new contract file:
touch src/HelloWorld.sol
Step 2: Add Solidity Code
Open src/HelloWorld.sol
and paste the following code:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.26;
contract HelloWorld {
string public message;
constructor(string memory _message) {
message = _message;
}
function setMessage(string memory _newMessage) public {
message = _newMessage;
}
}
This contract allows users to store and update a message on the blockchain.
🛠️ Compiling the Smart Contract Using Foundry
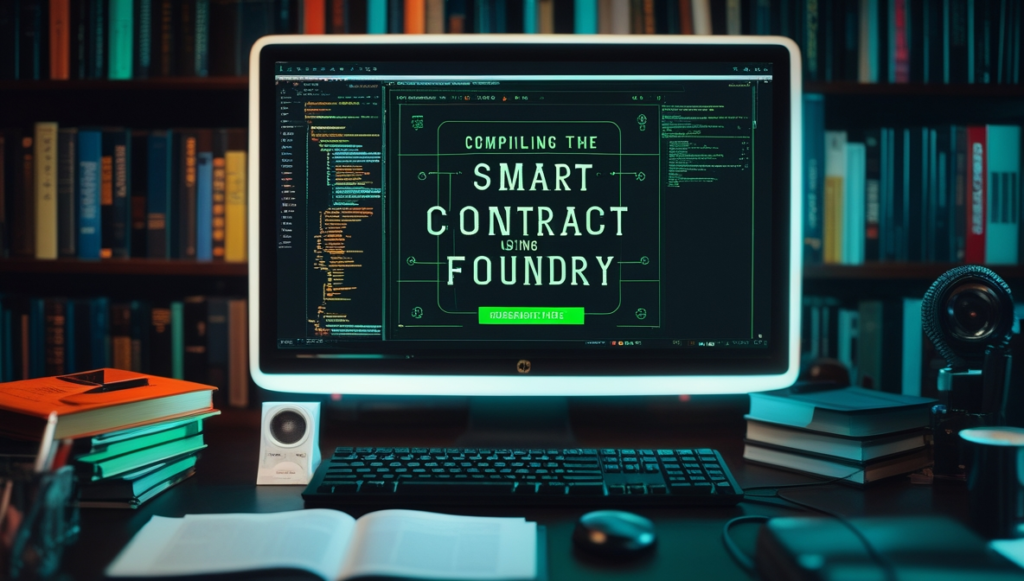
Before deploying, let’s compile the smart contract using Foundry’s forge build
command:
forge build
If everything is set up correctly, you should see output indicating a successful compilation.
🧪 Running Your First Tests
One of the best features of Foundry Solidity is its built-in testing framework, which allows you to write unit tests directly in Solidity.
Step 1: Create a Test File
Run this command to create a test file:
touch test/HelloWorld.t.sol
Step 2: Write the Test Cases
Open test/HelloWorld.t.sol
and add the following test cases:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.26;
import "forge-std/Test.sol";
import "../src/HelloWorld.sol";
contract HelloWorldTest is Test {
HelloWorld hello;
function setUp() public {
hello = new HelloWorld("Hello, Foundry!");
}
function testInitialMessage() public {
assertEq(hello.message(), "Hello, Foundry!");
}
function testSetMessage() public {
hello.setMessage("New Message");
assertEq(hello.message(), "New Message");
}
}
Step 3: Run the Tests
Execute the test using:
forge test
If everything works correctly, you’ll see a successful test execution output!
🎯 Next Steps
You’ve successfully:
✅ Initialized a new Foundry project
✅ Understood the default folder structure
✅ Written a basic Solidity smart contract
✅ Compiled and tested your smart contract
🔥 What to Learn Next?
- Learn Foundry scripting (
forge script
) - Deploy contracts on testnets like Sepolia
- Explore Foundry’s fuzz testing & debugging tools
- Integrate Foundry Solidity with frontend frameworks
📢 Conclusion
Foundry is an efficient, fast, and beginner-friendly Solidity development framework that makes writing, testing, and deploying smart contracts easier. Whether you’re just starting or transitioning from another framework, Foundry Solidity Tutorial is worth learning. Start experimenting, build innovative projects, and contribute to the Ethereum ecosystem!
Happy Coding! 🚀