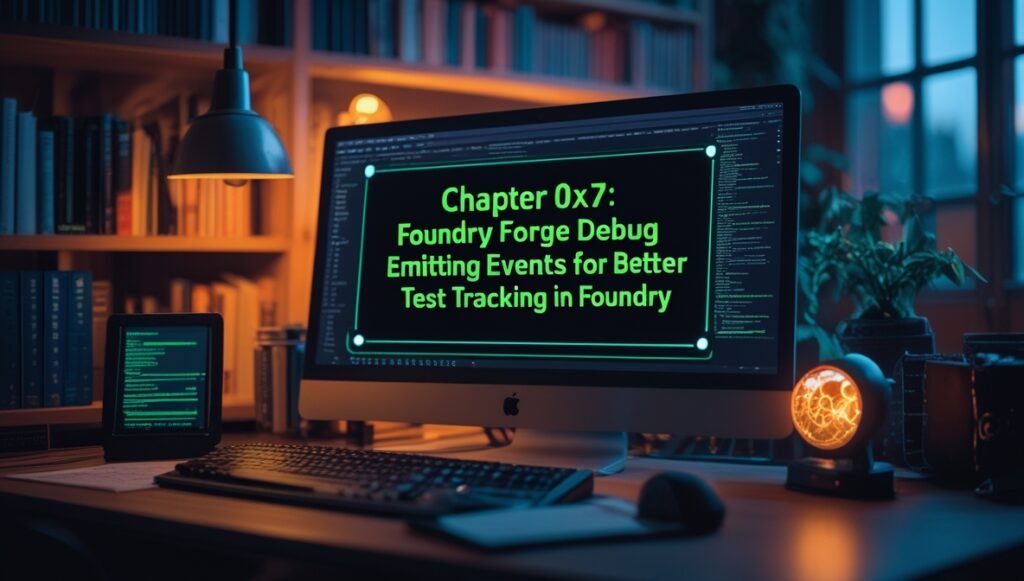
Chapter 0x7: Foundry Forge Debug And Emitting Events for Better Test Tracking in Foundry
Introduction to Foundry Debugging
Foundry Forge Debug And Emitting Events : When working with smart contracts in Solidity, debugging can be a real challenge. That’s where Foundry, a powerful smart contract testing framework, comes in. Foundry simplifies debugging and testing, making it easier to build robust and secure blockchain applications.
In this guide, we’ll break down debugging in Foundry using forge debug, emitting events for better test tracking, and customizing Foundry configurations. Whether you’re a beginner or an experienced Solidity developer, this tutorial will provide valuable insights into optimizing your debugging workflow.
Table of Contents
1. Using forge debug in Foundry
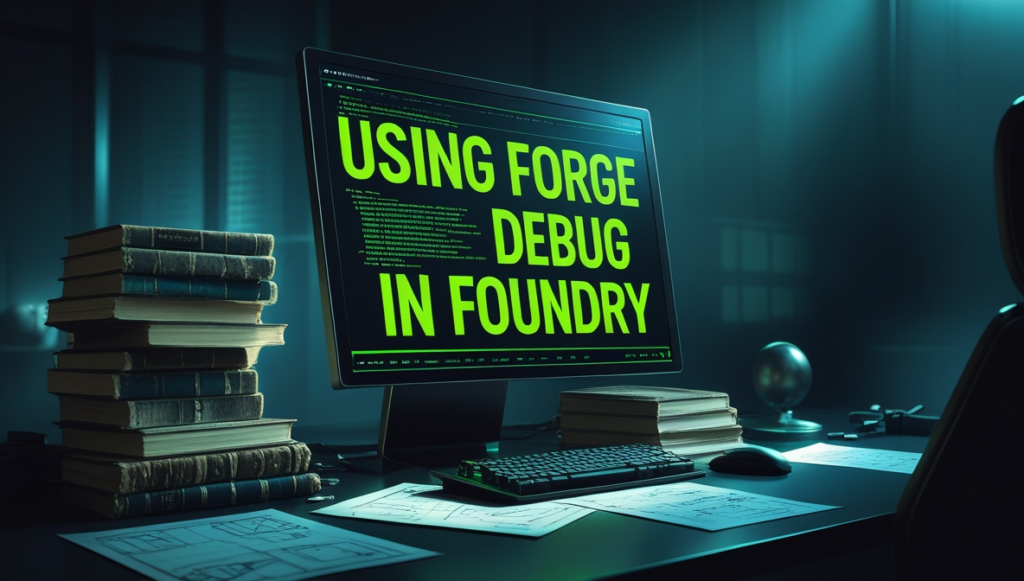
What is forge debug?
forge debug
is a powerful debugging tool in Foundry that helps developers inspect smart contracts and diagnose issues in their tests. It allows step-through execution, transaction tracing, and state inspection, making debugging failed tests much easier.
Setting Up forge debug
Before we jump into using forge debug
, ensure you have Foundry installed. If you haven’t set it up yet, you can install it using:
curl -L https://foundry.paradigm.xyz | bash
foundryup
Now, navigate to your smart contract project and run:
forge test --debug
This will launch the interactive debugger, allowing you to step through the execution.
Key Features of forge debug
- Step-through execution: Pause execution at specific lines.
- Inspect transaction traces: Understand how the contract’s state changes.
- Analyze failed tests: Identify where your smart contract test failed and why.
- Emit logs and events: View key execution steps in real-time.
Using forge debug
, you can set breakpoints, step through execution, and analyze variables. This is especially useful when dealing with complex smart contract interactions.
2. Emitting Events for Better Test Tracking in Foundry
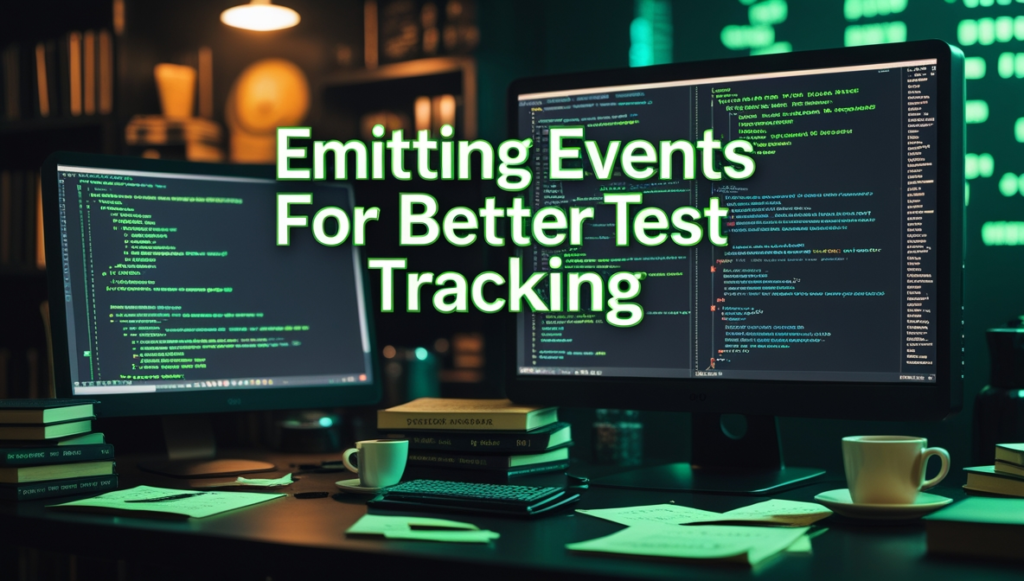
Events are an essential part of Solidity, allowing developers to track execution flow and detect failures. Emitting events during tests helps in debugging by providing a clear execution trail.
Why Use Events for Debugging?
- Better traceability: Helps in tracking contract state changes.
- Easy logging: Provides a history of function calls and variable changes.
- Improved debugging: Pinpoints the exact location of a failure.
How to Emit Events in Foundry?
Consider the following example of a Solidity contract with events:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.19;
contract DebugExample {
event DebugLog(string message, uint256 value);
uint256 public counter;
function increment() public {
counter += 1;
emit DebugLog("Counter incremented", counter);
}
}
In our Foundry test, we can track this event:
import "forge-std/Test.sol";
import "src/DebugExample.sol";
contract DebugExampleTest is Test {
DebugExample debugExample;
function setUp() public {
debugExample = new DebugExample();
}
function testIncrement() public {
debugExample.increment();
// Check if event was emitted
assertTrue(vm.logs[0].topics.length > 0, "Event not emitted");
}
}
Here, vm.logs
is used to inspect event logs, ensuring proper function execution.
3. Debugging Failed Tests In Foundry
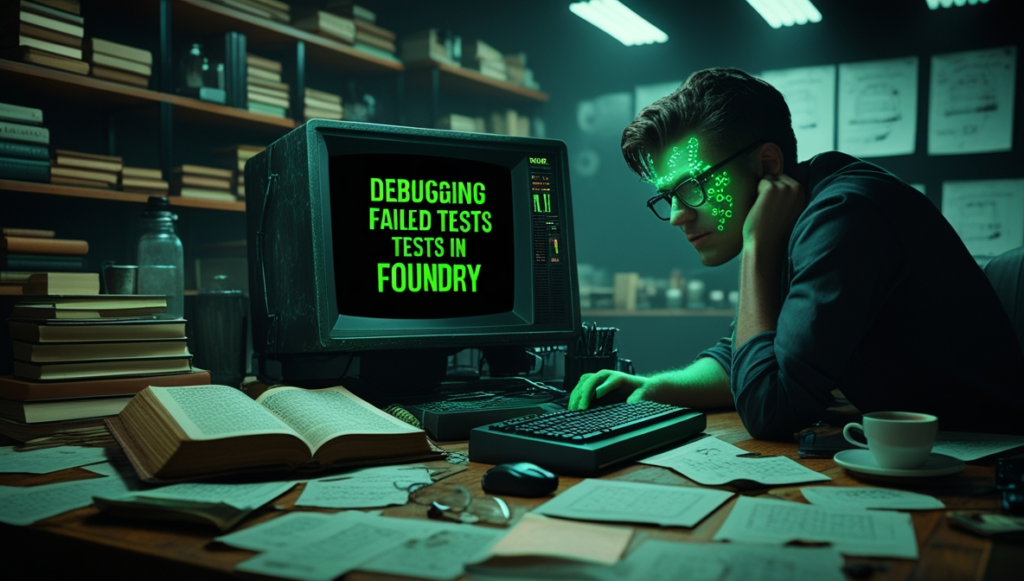
Even with careful development, smart contract tests can fail. Debugging these failures effectively is crucial for ensuring contract reliability.
Common Reasons for Failed Tests in Foundry
- Reverts due to require/assert statements
- Gas limits exceeded
- Incorrect function calls or parameters
- State inconsistencies
Using forge test –debug for Failed Tests
If a test fails, you can run:
forge test --debug
This opens the debugger, allowing you to step through execution. You can inspect the transaction stack, analyze emitted events, and detect state changes.
Using Vm Logs in Foundry
vm.log
helps track internal execution steps, making it easier to pinpoint failures:
vm.log("Step 1: Function called");
vm.log("Step 2: Variable value", myVar);
These logs provide detailed execution information in the Foundry test environment.
4. Customizing Foundry Configurations
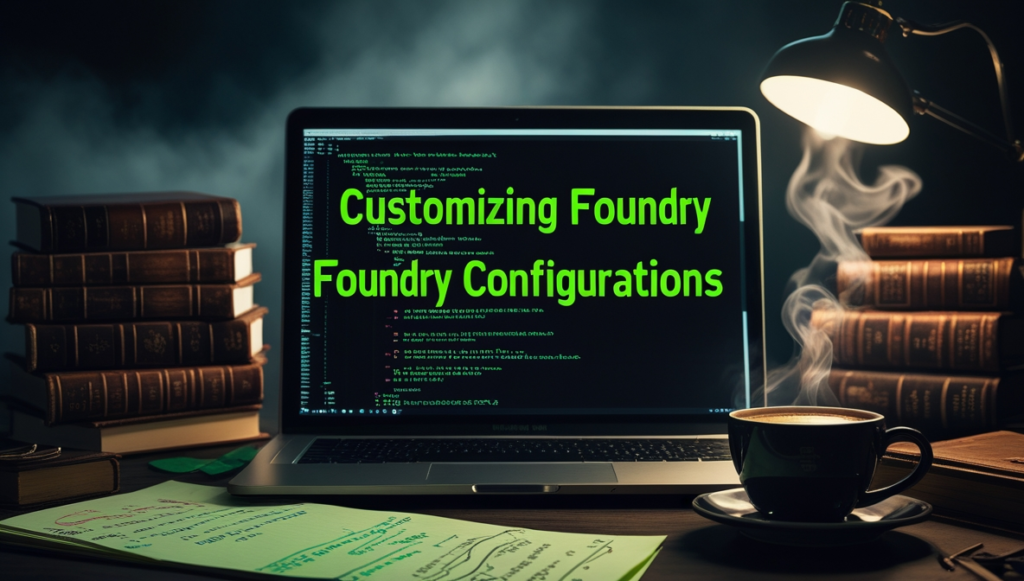
Foundry allows extensive configuration via foundry.toml
. You can modify default settings to optimize testing and debugging.
Common Configurations
You can create a foundry.toml
file in your project root:
[profile.default]
solc_version = "0.8.19"
fuzz_runs = 1000
depth_limit = 100
optimizer = true
optimizer_runs = 200
verbosity = 4
Key Configurations Explained
- solc_version: Specifies Solidity version.
- fuzz_runs: Number of test iterations for fuzzing.
- depth_limit: Limits execution depth to prevent infinite loops.
- optimizer: Enables Solidity optimizer for gas efficiency.
- verbosity: Adjusts logging level for debugging.
To apply these configurations, run:
forge build
This ensures that your contract is compiled with the specified settings.
5. Interacting with Smart Contracts in Foundry Web3
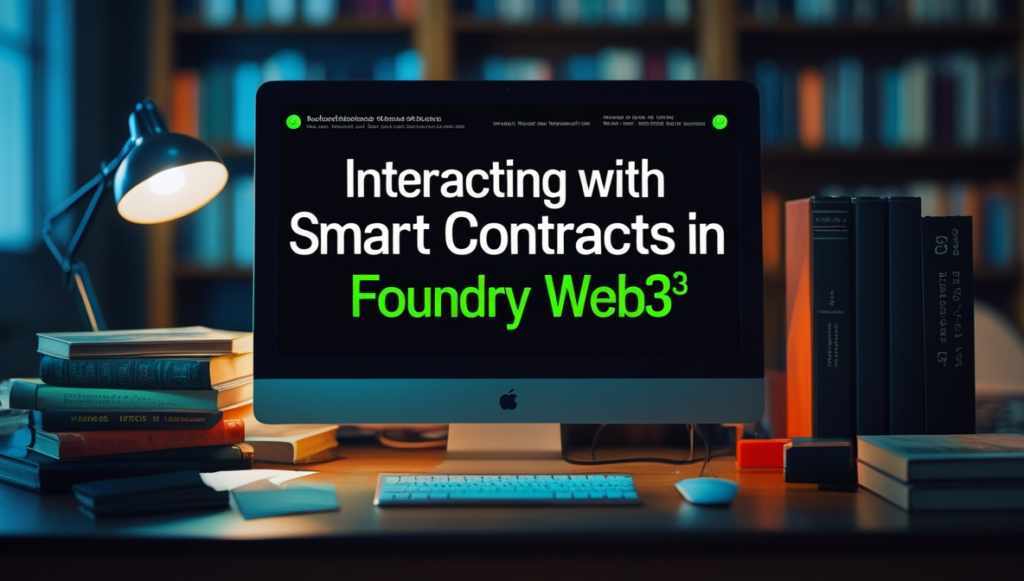
Beyond testing, Foundry provides tools to interact with deployed smart contracts using RPC.
Using Foundry RPC
You can call smart contract functions via Foundry’s built-in RPC support:
cast call <contract_address> "functionName(uint256) returns (uint256)" 123
This allows interaction with deployed contracts without writing additional scripts.
Interacting with Deployed Contracts in Foundry
To deploy a contract and interact with it:
forge create DebugExample --rpc-url <your_rpc_url>
Once deployed, you can call its functions using cast send
.
Conclusion
Debugging smart contracts in Foundry doesn’t have to be complicated. With tools like forge debug
, event emissions, and custom configurations, you can streamline testing and debugging efficiently.
Key Takeaways:
- Use
forge debug
to step through smart contract execution. - Emit events to track function calls and state changes.
- Utilize
vm.log
for granular debugging insights. - Configure
foundry.toml
to optimize testing and debugging. - Leverage Foundry RPC to interact with deployed contracts.
By implementing these best practices, you can develop more reliable, secure, and optimized smart contracts with Foundry.
Happy debugging! 🚀