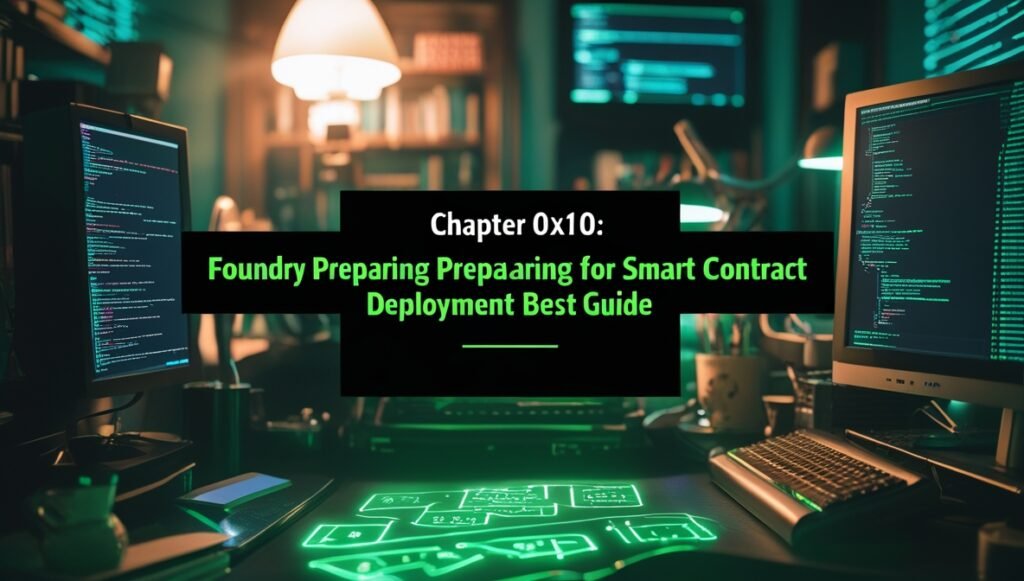
Chapter 0x10: Foundry Preparing for Smart Contract Deployment Best Guide
Foundry Preparing for Smart Contract Deployment : Deploying smart contracts is a crucial step in blockchain development. Whether you’re building decentralized applications (dApps), DeFi platforms, or NFT projects, understanding how to deploy and verify contracts efficiently is essential. In this guide, we’ll walk through deploying contracts using Foundry, verifying them on Etherscan, and integrating Foundry with other tools to enhance your workflow.
Table of Contents
This tutorial is beginner-friendly, with step-by-step instructions, explanations, and best practices to ensure a smooth deployment process. By the end of this guide, you’ll have a solid grasp of:
- Preparing for deployment in Foundry
- Using
forge create
to deploy smart contracts - Verifying contracts on Etherscan
- Advanced deployment topics
- Integrating Foundry with other tools
Let’s dive in!
1. Foundry Preparing for Smart Contract Deployment
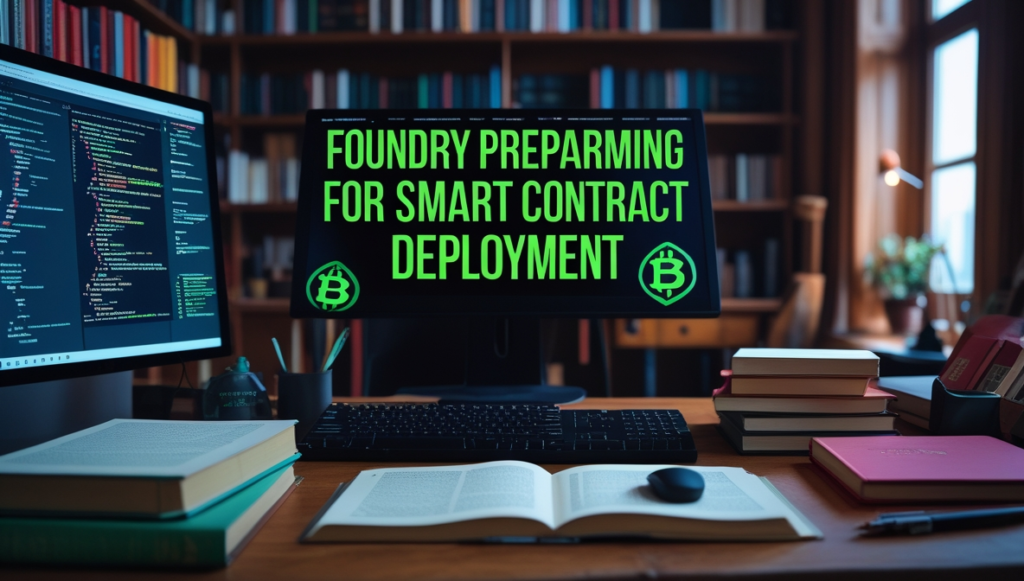
Before deploying contracts, we need to set up the environment and ensure everything is configured correctly. Foundry is a powerful Solidity development framework that simplifies testing, deployment, and debugging.
Step 1: Install Foundry
If you haven’t installed Foundry yet, run the following command:
curl -L https://foundry.paradigm.xyz | bash
foundryup
This installs Foundry and updates it to the latest version.
Step 2: Initialize a Foundry Project
To create a new project, run:
forge init my-foundry-project
cd my-foundry-project
This initializes a new project with a proper directory structure.
Step 3: Write a Simple Smart Contract
Create a new Solidity file inside the src
directory, for example, SimpleContract.sol
. This contract will be deployed using Foundry.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.26;
contract SimpleContract {
uint public value;
function setValue(uint _value) public {
value = _value;
}
}
Once the contract is ready, it’s time to deploy it.
2. Using forge create
to Deploy Contracts
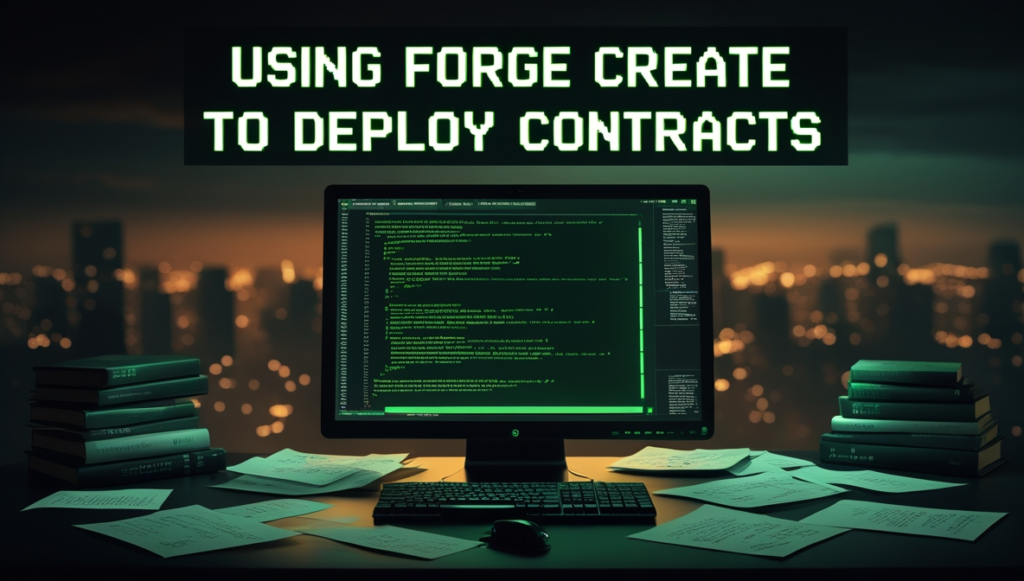
forge create
to Deploy ContractsThe forge create
command allows us to deploy contracts effortlessly. Here’s how to use it.
Step 1: Compile the Contract
Before deployment, compile the contract to check for errors:
forge build
If everything is fine, the contract is ready for deployment.
Step 2: Set Up a Wallet for Deployment
Deploying a contract requires a funded Ethereum wallet. You can use MetaMask, Alchemy, or Infura. Export your private key and set it in your environment variables:
export PRIVATE_KEY=your_private_key_here
export ETH_RPC_URL=https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID
Step 3: Deploy the Contract
Use forge create
to deploy:
forge create --rpc-url $ETH_RPC_URL --private-key $PRIVATE_KEY src/SimpleContract.sol:SimpleContract
You’ll receive a contract address after successful deployment.
Step 4: Verify Deployment
Check the transaction status on Etherscan using the provided contract address.
3. Verifying Contracts on Etherscan
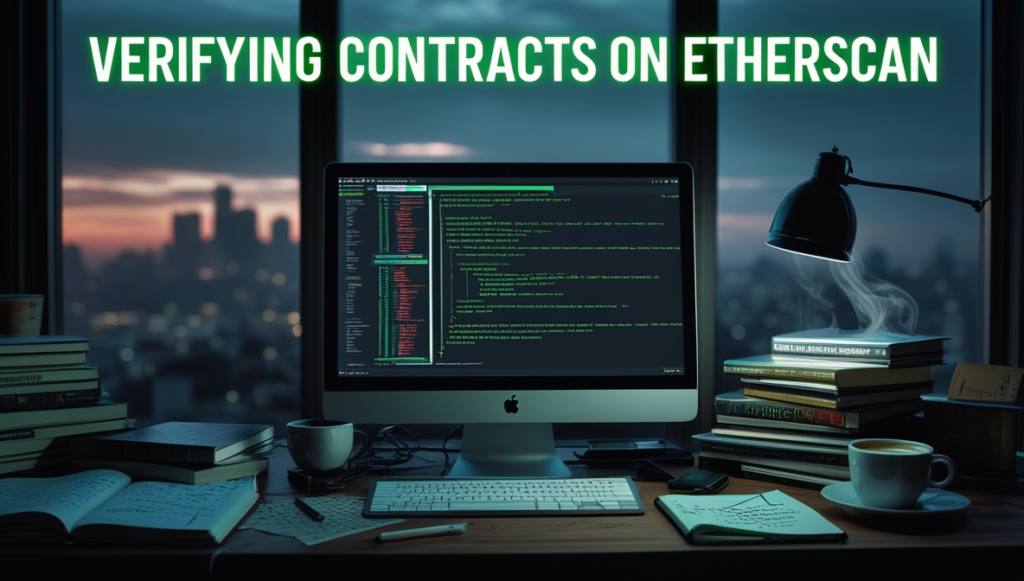
Verifying your contract on Etherscan makes it publicly viewable and callable by other dApps and users.
Step 1: Install Etherscan API Key
Register on Etherscan and get an API key. Then, set it as an environment variable:
export ETHERSCAN_API_KEY=your_api_key_here
Step 2: Verify the Contract
Use the following command to verify:
forge verify-contract --chain-id 1 --etherscan-api-key $ETHERSCAN_API_KEY CONTRACT_ADDRESS src/SimpleContract.sol:SimpleContract
Once verified, your contract’s source code will be visible on Etherscan.
Verifying on Testnets
For testnet verification, specify the correct chain ID:
- Sepolia:
--chain-id 11155111
- Goerli:
--chain-id 5
Example:
forge verify-contract --chain-id 11155111 --etherscan-api-key $ETHERSCAN_API_KEY CONTRACT_ADDRESS src/SimpleContract.sol:SimpleContract
This works for other explorers like Blockscout and BscScan as well.
4. Advanced Topics: Automating Deployments & Scripts
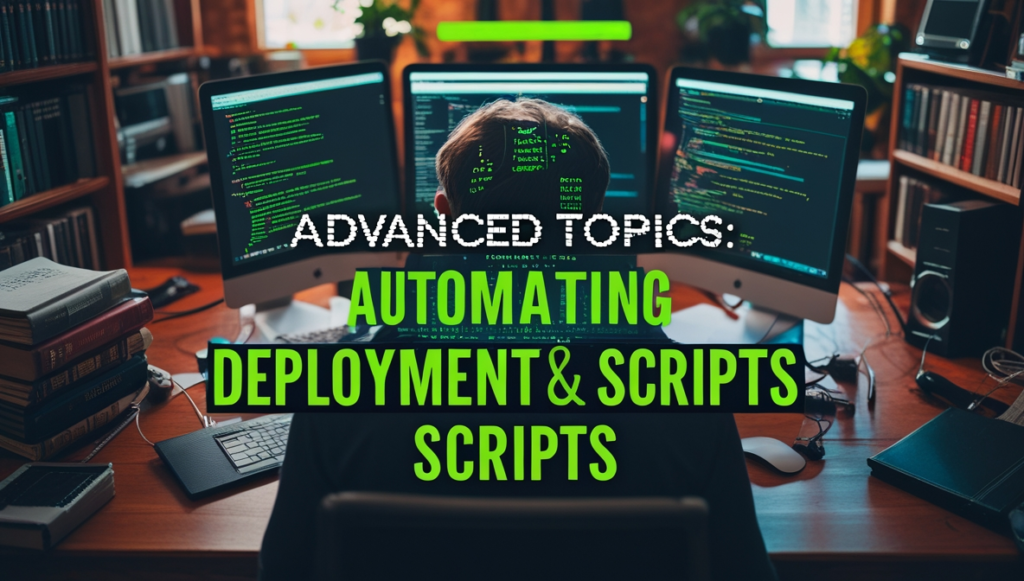
Writing a Deployment Script
Instead of manually running forge create
, use deployment scripts for automation. Create a script/Deploy.s.sol
file:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.26;
import "forge-std/Script.sol";
import "../src/SimpleContract.sol";
contract DeployScript is Script {
function run() external {
vm.startBroadcast();
new SimpleContract();
vm.stopBroadcast();
}
}
Run the script:
forge script script/Deploy.s.sol --rpc-url $ETH_RPC_URL --private-key $PRIVATE_KEY --broadcast
This automates contract deployment in a structured way.
5. Integrating Foundry with Other Tools
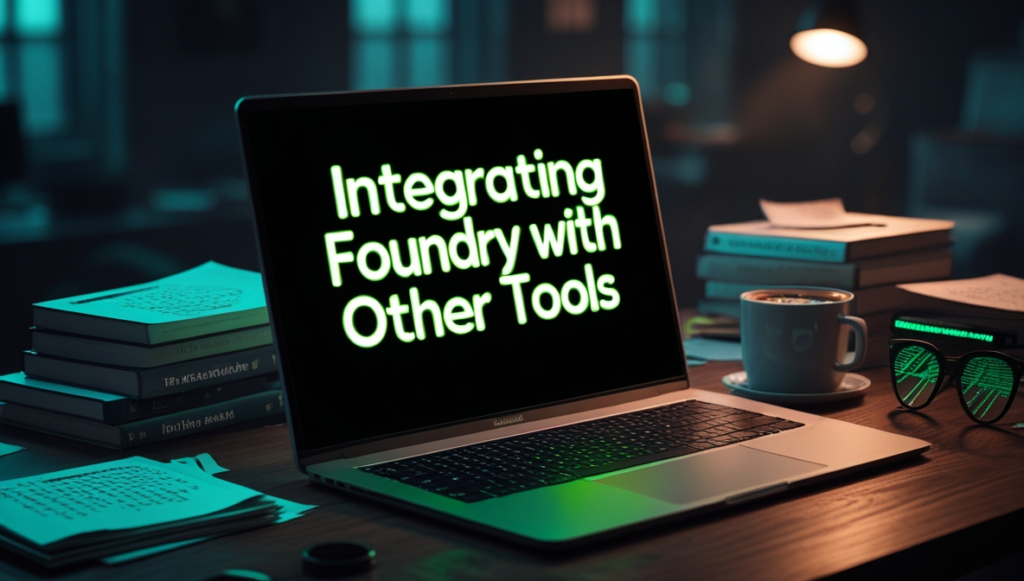
Foundry can be integrated with various Web3 tools to enhance development.
Using Foundry with Hardhat
Foundry works seamlessly alongside Hardhat. You can use Hardhat for testing while deploying with Foundry.
npx hardhat test
forge test
This enables flexibility in smart contract development.
Integrating Foundry with Python (Web3.py)
For backend interactions, use Web3.py to call deployed contracts:
from web3 import Web3
w3 = Web3(Web3.HTTPProvider("https://mainnet.infura.io/v3/YOUR_PROJECT_ID"))
contract = w3.eth.contract(address='YOUR_CONTRACT_ADDRESS', abi=YOUR_ABI)
print(contract.functions.value().call())
Foundry and The Graph
Use The Graph to index smart contract data and make querying easier for dApps.
Conclusion
By following this tutorial, you’ve learned how to:
- Prepare for deployment using Foundry
- Deploy smart contracts using
forge create
- Verify contracts on Etherscan
- Automate deployment with scripts
- Integrate Foundry with other Web3 tools
Foundry simplifies smart contract development, offering powerful features like scripting, verification, and flexible integrations. Now that you understand the deployment process, you can start building and deploying your own decentralized applications!
For more in-depth learning, check out Foundry Docs and experiment with advanced scripting.
Happy coding!