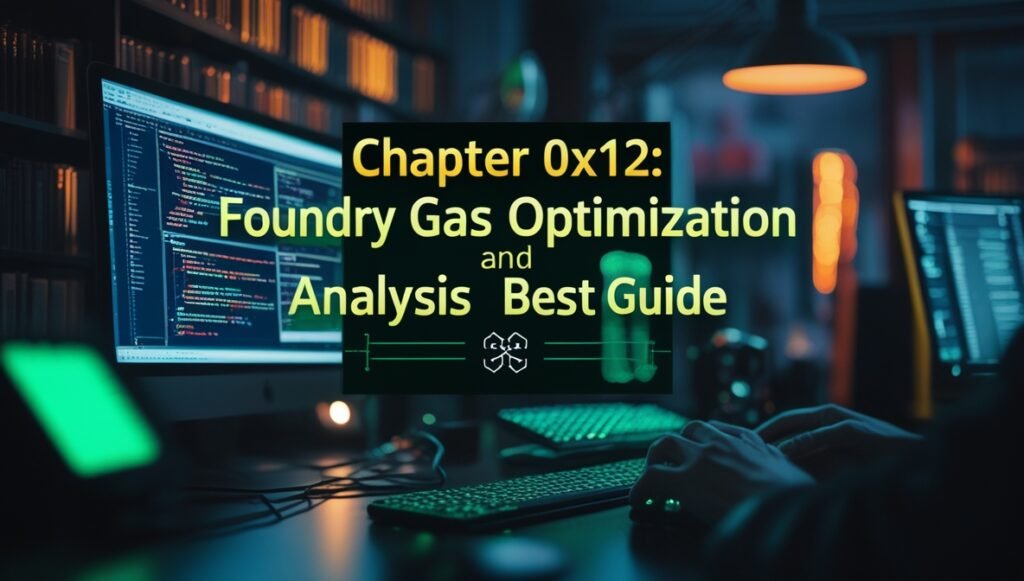
Chapter 0x12: Foundry Gas Optimization and Analysis Best Guide
Introduction
Foundry Gas Optimization and Analysis : Welcome to Chapter 0x12 of our Foundry tutorial series! If you’re working with Solidity smart contracts, you’ve probably noticed that gas fees can be ridiculously high if your code isn’t optimized. That’s where Foundry Gas Optimization and Analysis comes into play!
Table of Contents
In this guide, we’ll explore everything you need to know about optimizing gas costs in Solidity smart contracts using Foundry. Whether you’re a beginner or an experienced blockchain developer, this tutorial will help you write more efficient, cost-effective smart contracts.
Let’s dive in! 🚀
Why Is Gas Optimization Important?
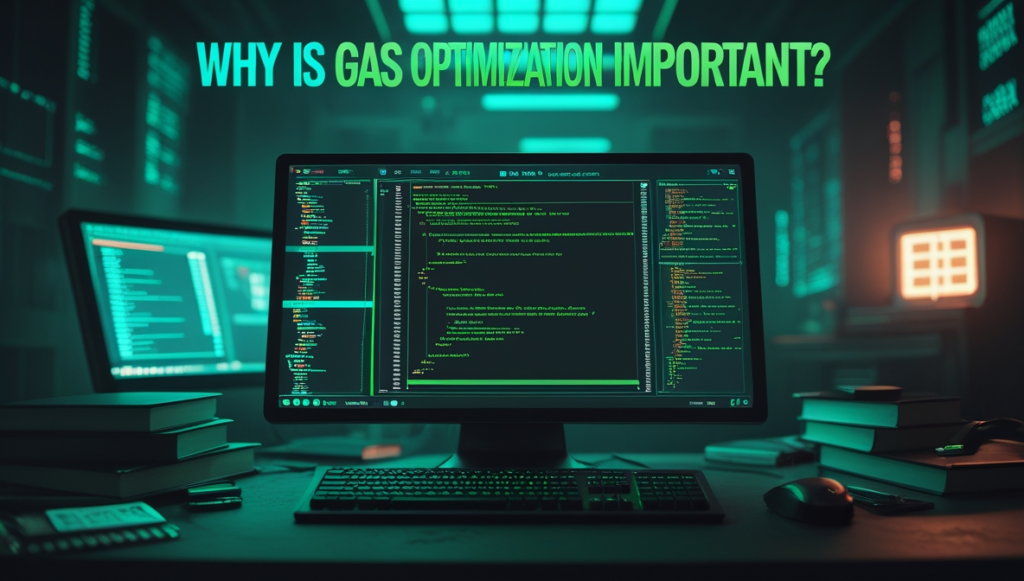
Gas is the unit of computational effort required to execute operations in Ethereum. Every function call, storage operation, and loop iteration consumes gas. High gas consumption = Higher transaction fees for users. Optimizing your smart contract reduces deployment costs, improves efficiency, and enhances user experience.
Benefits of Gas Optimization
- Lower Transaction Costs: Saves ETH for both developers and users.
- Efficient Execution: Faster contract execution on Ethereum.
- Scalability: Optimized contracts perform better in high-demand scenarios.
- Reduced Network Congestion: Helps prevent unnecessary blockchain bloat.
Now, let’s look at how Foundry helps in gas optimization and analysis. 🔍
What is Foundry?
Foundry is an Ethereum development toolchain that provides fast testing, fuzzing, debugging, and gas analysis capabilities. Unlike Hardhat or Truffle, Foundry is Rust-based, making it extremely fast and efficient.
Why Use Foundry for Gas Optimization?
- Faster Testing & Debugging
- Built-in Gas Snapshots to compare gas costs across contract versions.
- Optimized Compilation for better Solidity execution.
- Native Support for Fuzzing to find unexpected gas-heavy scenarios.
Now, let’s get into how to optimize gas usage in Solidity smart contracts using Foundry! 💡
Key Gas Optimization Techniques in Solidity
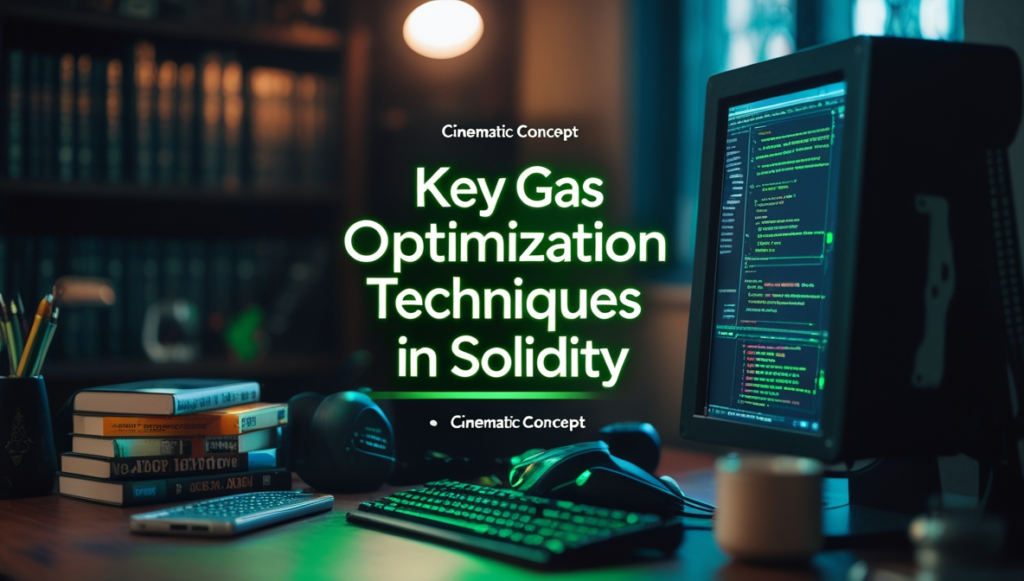
Here are some of the most effective gas optimization techniques when writing Solidity smart contracts in Foundry.
1. Use calldata
Instead of memory
for Function Arguments
Why? calldata
is cheaper than memory
because it does not copy data.
Example:
// Less Efficient
function processArray(uint256[] memory data) external {
// Process data
}
// Optimized
function processArray(uint256[] calldata data) external {
// Process data
}
2. Use unchecked
for Arithmetic Operations
Why? Solidity 0.8+ includes automatic overflow/underflow checks, but they cost gas.
Example:
function add(uint256 a, uint256 b) external pure returns (uint256) {
unchecked {
return a + b;
}
}
3. Use storage
and memory
Wisely
- Storage is expensive (~20,000 gas per operation).
- Memory is cheaper but still adds cost.
- Stack variables are cheapest (use
uint256
instead of struct where possible).
Example:
contract GasOptimized {
uint256 public num; // storage variable (expensive)
function updateNum(uint256 _num) external {
num = _num; // Costly write operation
}
}
Optimization: Use local variables instead of writing to storage frequently.
4. Packing Storage Variables
Why? Solidity stores variables in 32-byte slots. Packing multiple small variables into a single slot saves gas.
Example:
// Not optimized
contract Example {
uint128 a;
uint128 b; // Uses two storage slots
}
// Optimized
contract OptimizedExample {
uint128 a;
uint128 b; // Now packed into a single slot
}
5. Minimize Use of Loops
Loops are gas-expensive! If possible, use mappings or events instead of looping over large data sets.
Foundry Gas Optimization and Analysis in Action
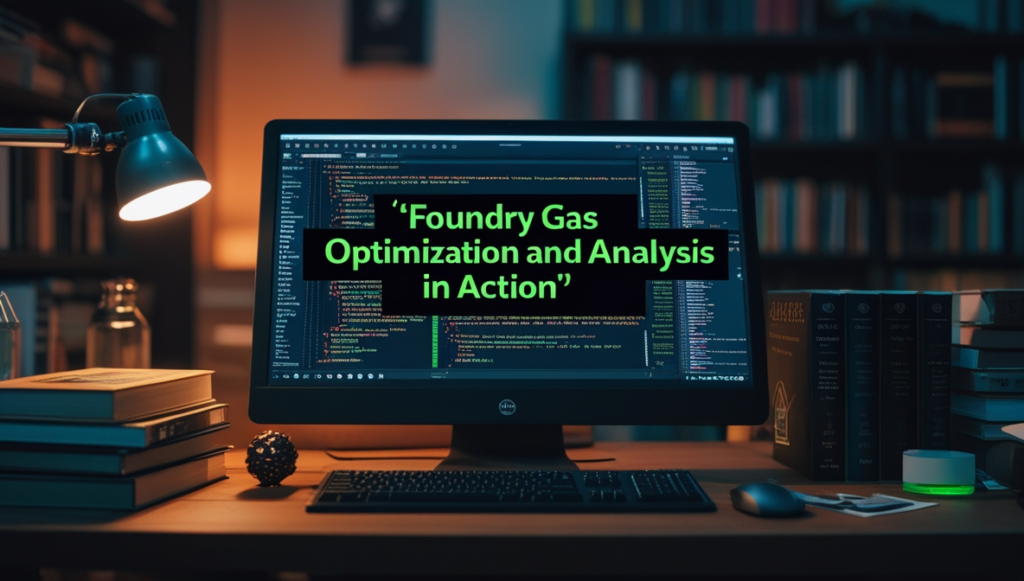
1. Using Foundry’s Gas Snapshots
Foundry allows you to track gas usage over time using built-in gas snapshots.
Running Gas Snapshots in Foundry
forge snapshot
This will generate a report showing how much gas each function consumes.
2. Fuzz Testing for Gas Optimization
Foundry’s built-in fuzzing tool tests your contract with random inputs, helping you find scenarios where gas costs spike.
Running Fuzz Tests in Foundry
forge test --fuzz-runs 500
3. Using forge analyze
for Detailed Reports
Foundry provides an in-depth gas analysis report:
forge analyze
This helps identify high-gas functions and optimize them.
Additional Resources
Want to explore more? Check out these resources:
- Foundry Gas Optimization and Analysis PDF 📄
- Foundry Gas Optimization and Analysis GitHub 🛠️
- Foundry Gas Optimization and Analysis Example 🔥
Conclusion
Optimizing gas usage in Solidity smart contracts using Foundry saves money, improves efficiency, and enhances user experience. By using gas snapshots, fuzz testing, and storage optimizations, you can significantly reduce transaction costs.
Recap of Key Takeaways:
✅ Use calldata
instead of memory
for function arguments.
✅ Apply unchecked
math operations when safe.
✅ Minimize storage writes and use storage packing.
✅ Avoid unnecessary loops and optimize data structures.
✅ Use Foundry’s gas snapshots, fuzz testing, and analysis tools.
By following these techniques, your smart contracts will be cost-efficient, scalable, and well-optimized! 🚀
What’s Next?
If you enjoyed this guide, stay tuned for the next chapter in our Foundry tutorial series! Let me know in the comments if you have any questions! Happy coding! 🎉