Foundry Smart Contract Security Testing : Welcome to Chapter 0x18 of our Foundry tutorial series! In this chapter, we’re diving deep into simulating attacks like reentrancy, overflows, and underflows using Foundry — the fastest, most developer-friendly tool for smart contract development in Solidity.
Table of Contents
Foundry Smart Contract Security Testing
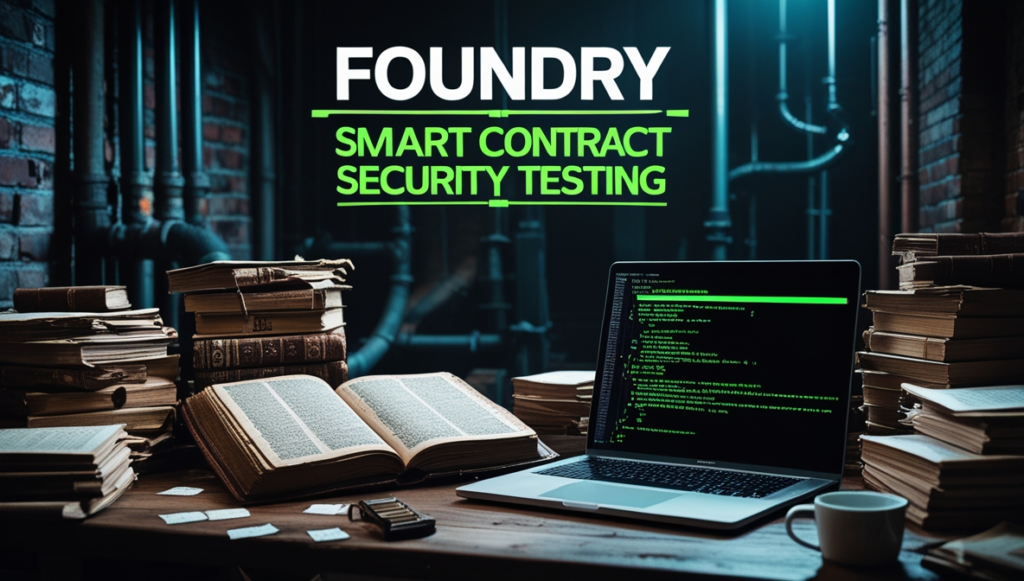
If you’re a beginner in the world of smart contract security, don’t worry — we’ll keep things simple, descriptive, and hands-on. You’ll walk away knowing how to use Foundry to test for vulnerabilities, how to set up Continuous Integration (CI) and Deployment Pipelines, and how to integrate popular audit tools like Slither and MythX.
By the end, you’ll know:
- How to simulate reentrancy, overflow, and underflow attacks in Foundry
- How to test smart contracts against known vulnerabilities
- How to use Slither and MythX for smart contract audits
- How to set up CI/CD pipelines for automatic testing and deployment
Let’s start hacking (ethically, of course!) 💻
🚀 Why Smart Contract Security Matters
Smart contracts are immutable. That means if you mess something up in production, it’s like writing a permanent bug into stone. That’s why Foundry smart contract auditing techniques are crucial. Testing isn’t just good practice — it’s a necessity.
Some infamous bugs like The DAO hack (2016) and Parity Wallet bug (2017) were avoidable with better testing. We’ll show you how to avoid those same mistakes using Foundry.
🛠️ Setting Up Foundry (If You Haven’t Already)
If you’re just joining in, install Foundry with:
curl -L https://foundry.paradigm.xyz | bash
foundryup
And to create your project:
forge init my-security-project
cd my-security-project
Let’s start breaking stuff (intentionally) 🧪
🧵 Part 1: Simulating Reentrancy Attacks in Foundry
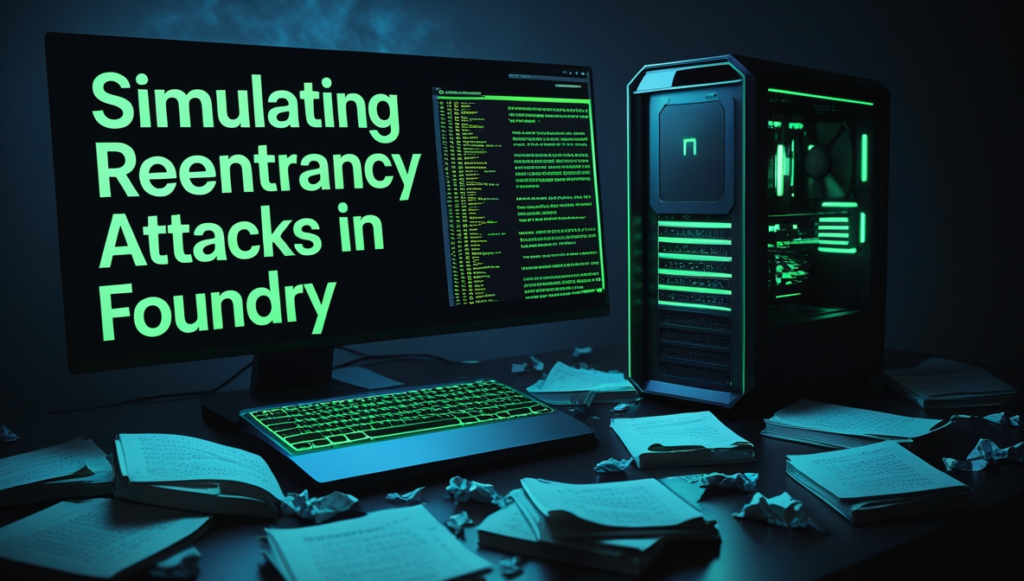
🧠 What is a Reentrancy Attack?
A reentrancy attack happens when a smart contract calls another contract before updating its internal state, allowing malicious re-entry into the function.
🔬 Example Code: Vulnerable Contract
// contracts/VulnerableBank.sol
contract VulnerableBank {
mapping(address => uint256) public balances;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw() public {
uint256 amount = balances[msg.sender];
require(amount > 0);
(bool success, ) = msg.sender.call{value: amount}("");
require(success);
balances[msg.sender] = 0;
}
}
🧪 How to Test Reentrancy Attack Using Foundry
// test/ReentrancyTest.t.sol
contract ReentrancyTest is Test {
VulnerableBank bank;
function setUp() public {
bank = new VulnerableBank();
}
function testReentrancy() public {
// Simulate the attack and assert failure
}
}
When writing Foundry smart contract reentrancy finding methods, simulate a contract that reenters withdraw()
and drains funds.
✅ Why It Matters
Testing for Foundry Reentrancy Attacks in Solidity Smart Contracts helps you understand Foundry Smart Contract Security Risks early.
📉 Part 2: Simulating Overflows and Underflows Attacks in Foundry
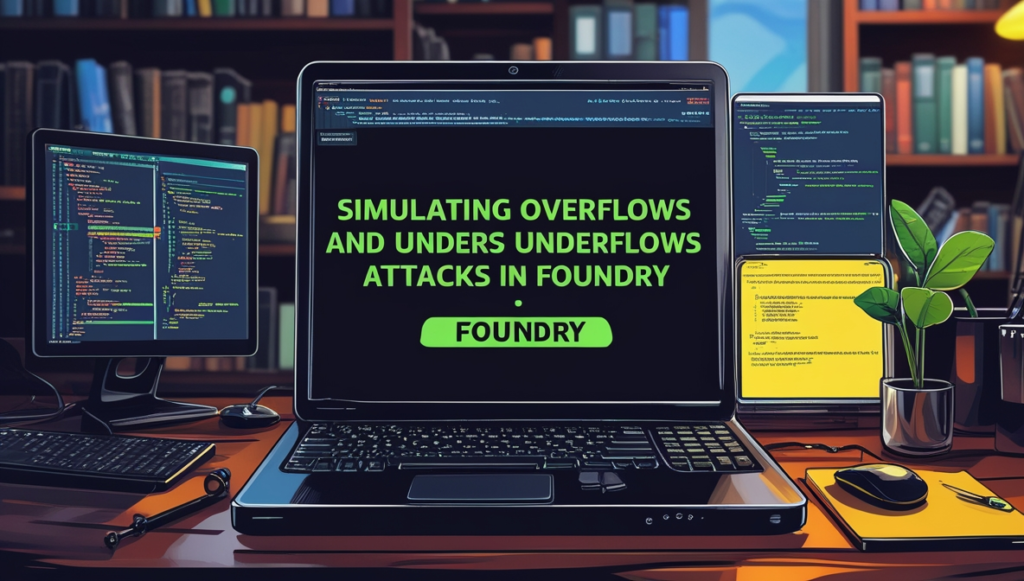
🔍 What’s an Overflow/Underflow?
These bugs occur when a number exceeds or goes below its data range (e.g., uint8
goes from 255 to 0).
🔬 Vulnerable Example
contract OverflowContract {
uint8 public count = 255;
function addOne() public {
count += 1; // Overflow!
}
}
🔬 Foundry Test for Overflow
contract OverflowTest is Test {
OverflowContract contractWithBug;
function setUp() public {
contractWithBug = new OverflowContract();
}
function testOverflow() public {
vm.expectRevert();
contractWithBug.addOne();
}
}
🛡️ How to Test Integer Overflow Underflow Attack Using Foundry
Foundry includes --via-ir
and --optimize
flags to catch these bugs early. You can even simulate them manually, as shown.
These are your basic foundry smart contract overflow and underflow finding methods.
🛠️ Foundry Testing Contracts Against Known Vulnerabilities
When it comes to Foundry smart contract testing, one big win is using community-curated attack patterns. Here are a few:
- Unchecked
call()
results - tx.origin misuse
- Fallback function gas griefing
You can write custom tests for these patterns and even integrate them into your CI pipeline.
function testFallbackGasGrief() public {
// simulate fallback gas griefing scenarios
}
Using these methods, you’re testing contracts against known vulnerabilities with ease.
🔒 Foundry Smart Contract Hacking (Ethically!)
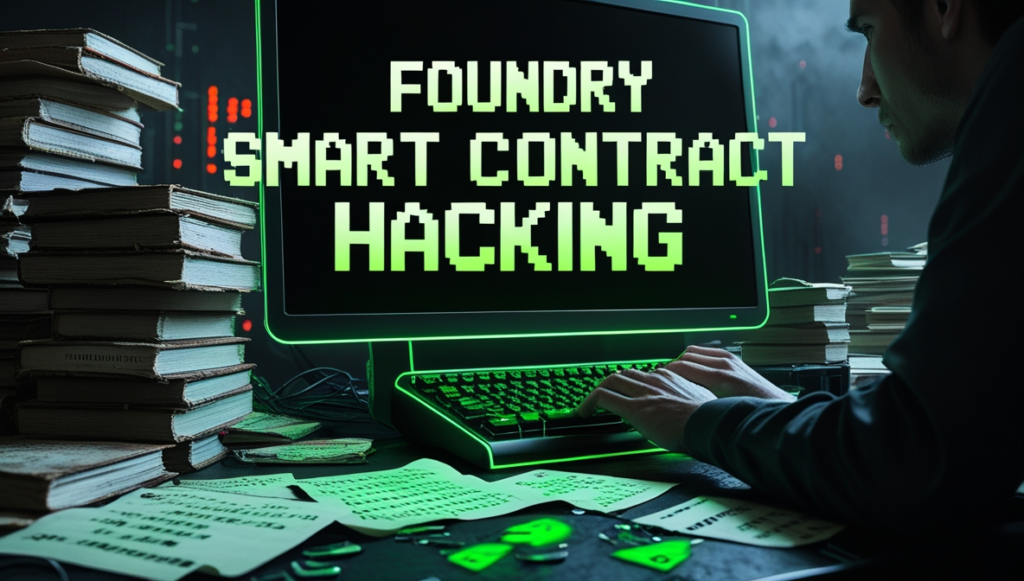
Foundry gives you raw power to simulate real-world hacks safely in your dev environment.
Other cool stuff you can simulate:
- Front-running attacks
- Flash loan exploits
- Gas griefing
- Block timestamp manipulation
These simulations help in foundry vulnerabilities finding before anyone else can exploit them.
🔍 Integrating Tools Like Slither and MythX for Security Audits
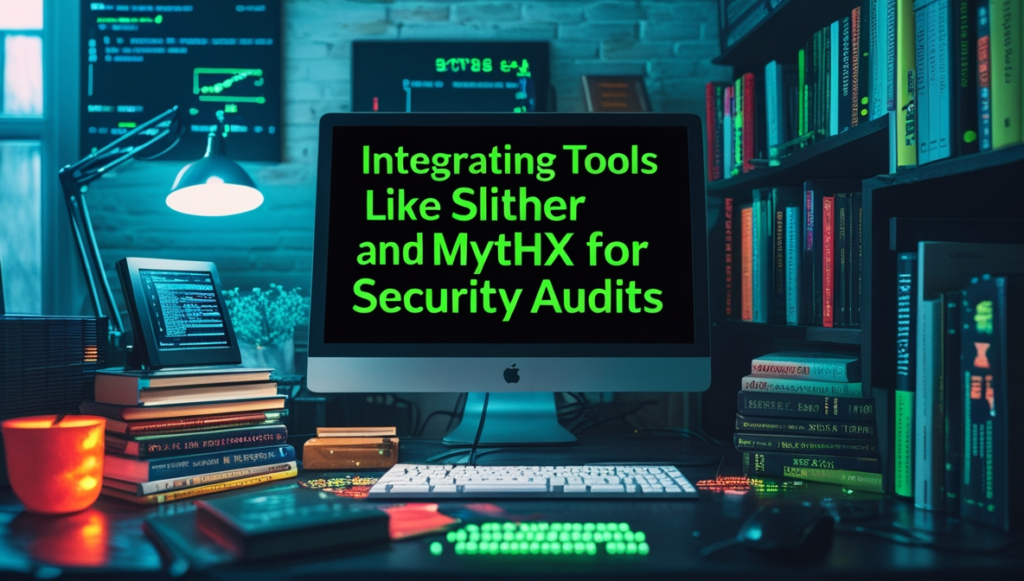
Let’s bring in the big guns 🔍
🐍 Slither
Slither is a static analysis tool by Trail of Bits.
Install:
pip install slither-analyzer
Usage:
slither ./src
It’ll help you catch:
- Reentrancy
- Shadowing
- Access control bugs
☁️ MythX
MythX is a cloud-based scanner for smart contracts.
Steps:
- Register on MythX
- Use tools like MythX CLI or Remix plugin
Why this matters? It automates the Foundry Smart Contract Auditing Techniques with pro-level insights.
These tools greatly assist with Integrating tools like Slither and MythX for security audits.
🔁 Foundry Continuous Integration (CI) and Deployment Pipelines
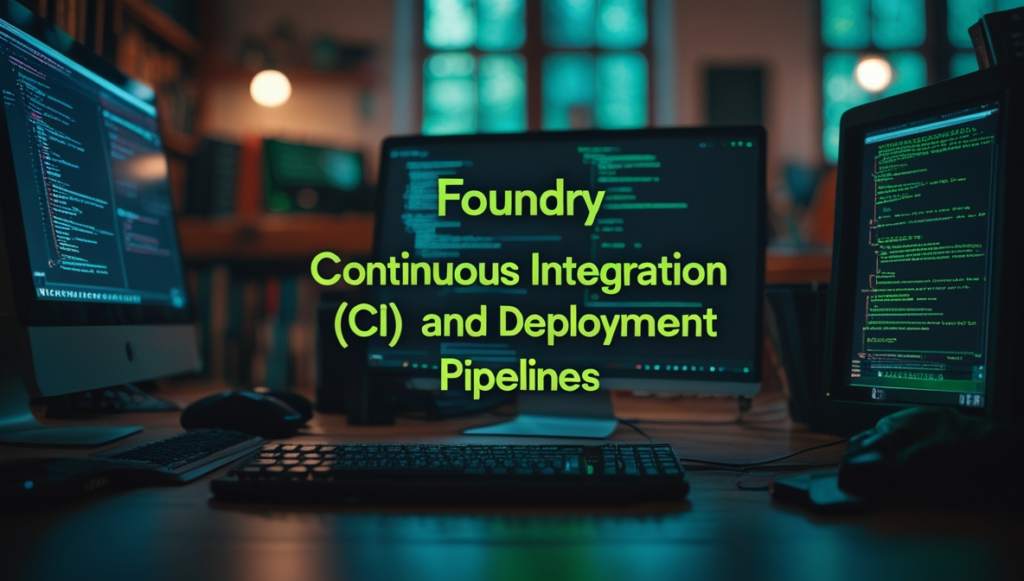
Automate everything! That’s the goal of DevOps. You don’t want to run tests manually forever.
✅ Foundry + GitHub Actions
Create .github/workflows/foundry.yml
name: Foundry CI
on: [push]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Install Foundry
run: |
curl -L https://foundry.paradigm.xyz | bash
foundryup
- name: Run Tests
run: forge test
With this setup, you’ve got Continuous Integration (CI) and Deployment Pipelines up and running!
Add auto-deployment scripts using Hardhat or Foundry and deploy on push or PR merge.
🔚 Wrapping Up: What You’ve Learned
You now know how to:
- Start simulating attacks like reentrancy in Foundry
- Simulate attacks like overflows and underflows in Foundry
- Use Foundry for smart contract testing and vulnerability finding
- Integrate Slither and MythX for audit automation
- Build a robust CI/CD pipeline with Foundry and GitHub
By practicing these Foundry smart contract security risks, you protect your users and your funds. And by integrating automated testing tools and pipelines, you save yourself from disasters and bugs in production.
Always remember — it’s better to break your contract in testnet than have it broken in mainnet.
Stay secure, and happy hacking! 🔐💻
📚 FAQs
Q: How to test reentrancy attack using Foundry?
A: Write a mock attack contract, use Foundry’s vm
cheats to simulate the interaction, and verify if your contract’s funds are drained.
Q: How to test integer overflow underflow attack using Foundry?
A: Simulate boundary values like uint8(255) + 1
, use vm.expectRevert()
to ensure the operation fails.
Q: What are some Foundry smart contract vulnerabilities finding methods?
A: Use custom test cases, static analysis tools like Slither, and fuzzing tools built into Foundry.
Q: Is Foundry good for beginners?
A: Yes! It’s fast, easy to learn, and integrates seamlessly with audit tools.
✍️ Final Note
Keep practicing, stay curious, and let Foundry be your hacking lab for secure, rock-solid dApps.
Till the next chapter, happy building! 🧱🚀