Solidity Tutorial Chapter 17: Libraries in Solidity – Libraries in Solidity are a powerful tool for creating reusable, gas-efficient, and well-structured code in your smart contracts. By understanding how libraries work and when to use them, you can simplify your Solidity code, cut down on redundancy, and make your contracts more cost-effective. In this chapter, we’ll explore how libraries function in Solidity, the advantages they offer, and the best practices to follow to get the most out of them.
Table of Contents
What Are Libraries in Solidity?
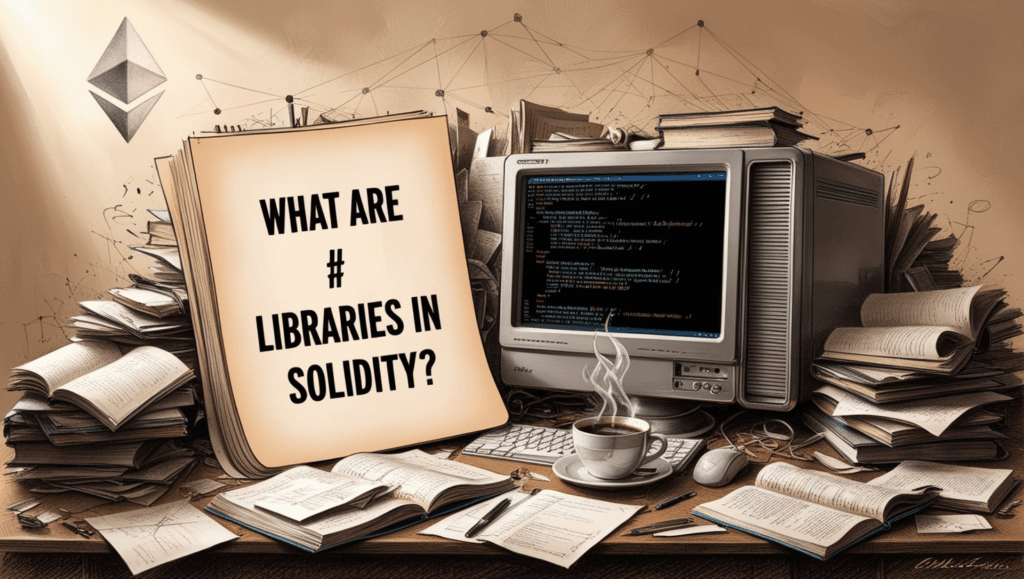
In Solidity, libraries are collections of functions that you can use across different contracts without having to rewrite the same code. They allow developers to bundle commonly-used code into a module, making it easier to reuse functions and optimize gas costs. Think of libraries as helper modules that contain code meant to be shared across multiple contracts.
Libraries are incredibly helpful when dealing with mathematical calculations, utility functions, or custom data structures. Since they don’t store state and can’t hold Ether, they are ideal for functions that perform calculations or data manipulations without altering the contract’s state.
Why Use Libraries in Solidity?
Libraries in Solidity come with several benefits:
- Code Reusability: Libraries promote reusability, allowing you to use the same functions across different contracts without redundancy.
- Gas Efficiency: Because libraries share code, they help reduce deployment costs. When used correctly, they keep your contracts streamlined and gas-efficient.
- Reduced Code Duplication: Instead of duplicating functions across multiple contracts, you can centralize these functions in a library.
- Improved Maintainability: Libraries keep your codebase cleaner, which makes it easier to read and maintain.
Types of Libraries in Solidity
Solidity offers two main types of libraries:
- Embedded Libraries: These libraries don’t need to be deployed separately; their code is embedded directly into the contract that uses them.
- Linked Libraries: Linked libraries are deployed separately, and contracts refer to them. They’re great for larger code bases because they help save space and reduce deployment costs.
How to Create a Library in Solidity
Creating a library in Solidity is quite straightforward. Here’s a basic example of a library that contains functions to calculate basic mathematical operations. Let’s name our library MathLibrary
.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
library MathLibrary {
function add(uint a, uint b) internal pure returns (uint) {
return a + b;
}
function subtract(uint a, uint b) internal pure returns (uint) {
require(b <= a, "Subtraction overflow");
return a - b;
}
function multiply(uint a, uint b) internal pure returns (uint) {
return a * b;
}
}
In this example:
- We define three basic functions—
add
,subtract
, andmultiply
—that can be used for simple math operations. - The keyword
internal
is used because libraries are intended for internal use, within the contract itself or within other contracts that import them.
Using a Library in a Solidity Contract
Once you’ve created a library, you can use it in your contract by attaching the library functions to data types. For instance, let’s say we want to use MathLibrary
in a simple contract to perform arithmetic operations.
pragma solidity ^0.8.0;
import "./MathLibrary.sol";
contract Calculator {
using MathLibrary for uint;
function calculate(uint a, uint b) public pure returns (uint sum, uint difference, uint product) {
sum = a.add(b); // Using add function from MathLibrary
difference = a.subtract(b); // Using subtract function from MathLibrary
product = a.multiply(b); // Using multiply function from MathLibrary
}
}
In this contract, we:
- Import
MathLibrary
. - Use the
using
keyword to allow theuint
data type to access library functions. - Call functions from
MathLibrary
to perform the calculations.
By using libraries in this way, we’ve made the contract code simpler and more readable, while also keeping it efficient.
Best Practices for Using Libraries in Solidity
While libraries are extremely useful, there are some best practices to follow to ensure that you’re using them effectively and securely.
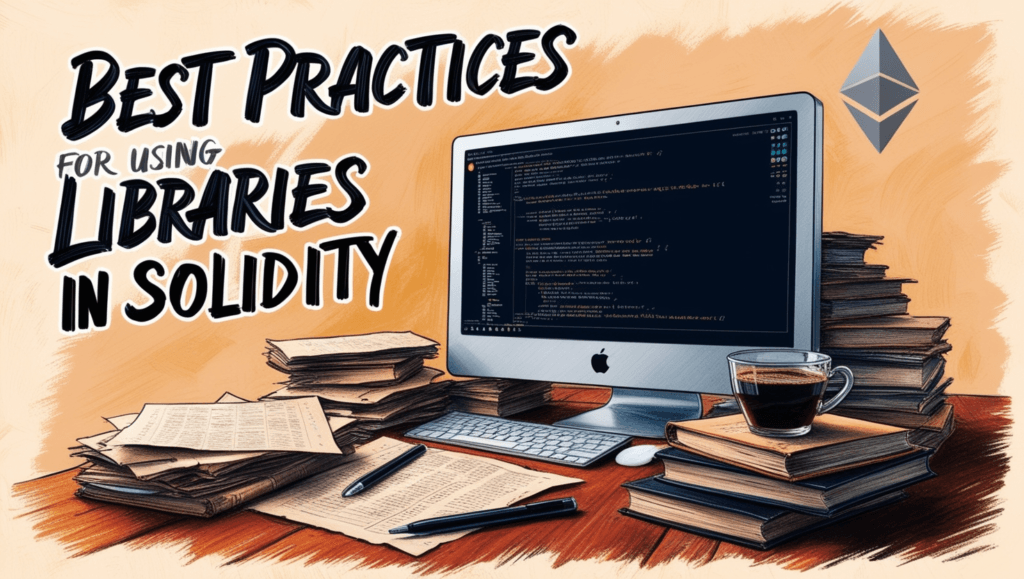
1. Use Libraries for Repeated Calculations or Utility Functions
Functions that are commonly used across multiple contracts, like math operations or string manipulations, are prime candidates for libraries. This approach allows you to avoid code repetition and centralizes common functionality.
2. Make Use of Pure or View Functions
Since libraries can’t hold state, all functions within a library should either be pure
or view
. Pure
functions don’t access the blockchain’s state, while view
functions can only read from the state but not modify it. This keeps your functions lightweight and maximizes gas efficiency.
3. Avoid State Variables in Libraries
Libraries don’t have their own storage, which means they can’t store state variables or manage balances. Libraries should only perform actions that don’t require persistent data. For instance, functions that involve calculations or data validation are ideal for libraries, but anything that requires state changes should be left to contracts.
4. Be Cautious with Linked Libraries
While linked libraries are a great way to save gas, they require careful handling because they are deployed separately and have their own address. If you update the library, you’ll need to redeploy the contracts using it, which can be complex and costly.
5. Use Using For
for Data Type-Specific Libraries
The using
keyword allows you to attach library functions to a specific data type, making it easier to work with certain types of data in your contract. This approach can make your code cleaner and more intuitive.
using MathLibrary for uint;
Real-World Example of Libraries in Solidity
To see libraries in action, let’s consider a practical scenario. Suppose we’re building a contract to handle a simple voting system. We can use a library to validate user inputs and ensure the data conforms to certain standards.
pragma solidity ^0.8.0;
library ValidationLibrary {
function isValidVote(uint vote) internal pure returns (bool) {
return (vote == 1 || vote == 0); // 1 for Yes, 0 for No
}
}
contract Voting {
using ValidationLibrary for uint;
mapping(address => uint) public votes;
function castVote(uint vote) public {
require(vote.isValidVote(), "Invalid vote");
votes[msg.sender] = vote;
}
}
In this contract:
- The library
ValidationLibrary
checks if a vote is valid (either 1 or 0). - The
castVote
function usesisValidVote
to ensure that the vote is within acceptable bounds. - This structure simplifies the contract logic and keeps validation rules centralized in the library.
Benefits of Using Libraries in Solidity
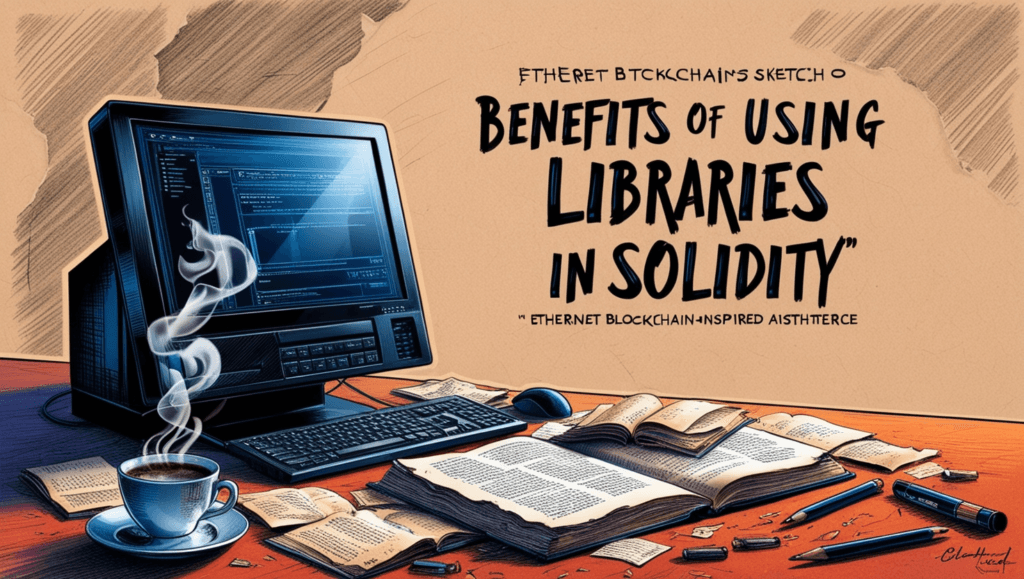
Let’s summarize why libraries can significantly improve Solidity code:
- Gas Efficiency: Libraries reduce code duplication, which saves on gas costs during deployment and execution.
- Code Organization: Libraries help organize code by grouping related functions together, making the codebase cleaner.
- Enhanced Maintainability: Updating code is easier when functionality is organized within libraries rather than scattered across multiple contracts.
- Modularity: Libraries promote modular design, making your contracts more flexible and scalable.
Common Libraries in Solidity
Several common libraries are widely used in Solidity to help with basic tasks. Here are a few examples:
- SafeMath: This library helps prevent overflow and underflow in arithmetic operations.
- Address Library: Used to safely handle addresses in contracts.
- Strings Library: Provides functions for string manipulation, such as converting integers to strings.
For example, the SafeMath library is particularly helpful to avoid arithmetic errors, as it automatically reverts transactions if an operation results in overflow.
Conclusion: Why Mastering Libraries in Solidity Matters
Libraries in Solidity are more than just tools—they’re an essential part of writing clean, efficient, and scalable smart contracts. By keeping your code organized, reusing functions, and minimizing gas costs, libraries help you get the most out of your Solidity development.
As you continue to build more complex applications on the blockchain, mastering libraries will become invaluable for streamlining your code and enhancing your contract’s functionality. Whether it’s performing calculations, validating data, or managing reusable functions, libraries allow you to focus on writing high-quality, efficient smart contracts.