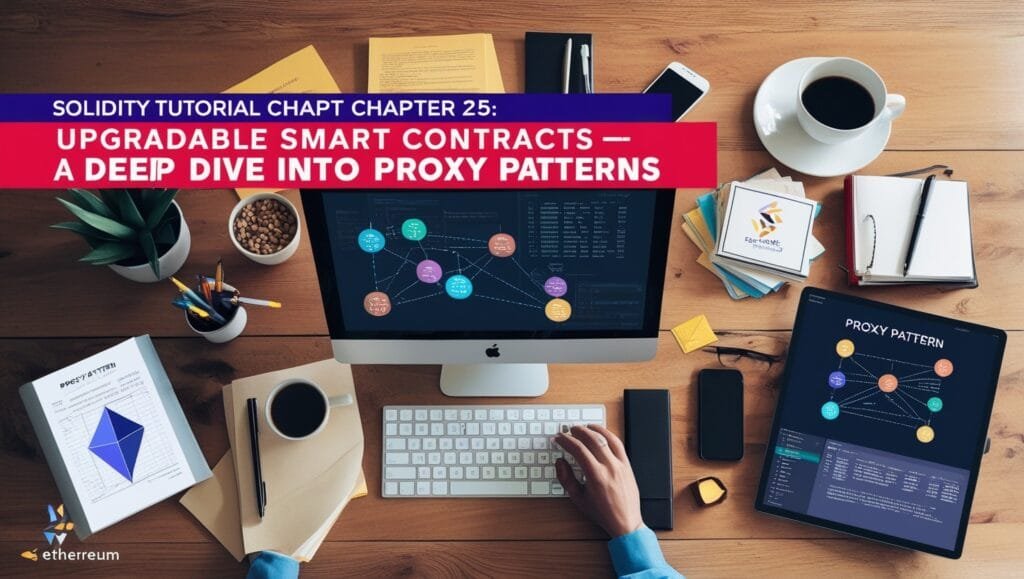
Solidity Tutorial Chapter 25: Upgradable Smart Contracts- A Deep Dive into Proxy Patterns
Upgradable Smart Contracts: In the world of blockchain and smart contracts, immutability is one of the most important features. However, it also presents a challenge: once a smart contract is deployed, it can’t be changed. This becomes an issue when bugs need to be fixed or new features need to be added after a contract has been deployed.
To solve this, upgradable smart contracts are introduced. These smart contracts allow the logic (code) to be updated without changing the stored data (state). The key to achieving this is using proxy patterns, which help decouple the data and the logic. In this article, we will discuss several proxy patterns used for creating upgradable smart contracts, including the UUPS, Simple Proxy, Transparent Proxy, Beacon Proxy, and Diamond Proxy patterns. We will also provide simple examples for each one to make it easy to understand.
Table of Contents
What Are Upgradable Smart Contracts?
Upgradable smart contracts are designed to allow changes to the business logic of a smart contract after it has been deployed. The data (state) of the contract is preserved, but the contract’s logic can be updated without losing any stored information. This is done using proxies, which point to different versions of the implementation contract.
The key idea is that the proxy contract manages the contract’s state, while the implementation contract handles the logic. If we need to upgrade the logic, we can deploy a new version of the implementation contract and update the proxy to point to it.
Why Are Upgradable Smart Contracts Important?
- Bug Fixes: Traditional smart contracts can’t be altered after deployment, leaving no room to fix errors. Upgradability solves this issue.
- Feature Expansion: As projects evolve, adding new functionalities is crucial. Upgradable contracts enable seamless integration.
- Cost Efficiency: Instead of deploying an entirely new contract and migrating data, upgradable contracts save time and gas fees.
- User Trust: Users remain confident as their interactions and balances stay intact during upgrades.
Advantages of Upgradable Smart Contracts
- Flexibility: Modify logic as needed without redeploying from scratch.
- Efficiency: Save gas fees and time with seamless updates.
- Continuity: Ensure user balances and interactions remain intact.
Key Concepts Behind Upgradability
Upgradable smart contracts are typically built using a proxy pattern. Here’s a breakdown of the core concepts:
- Proxy Contract: Acts as a mediator that forwards user requests to the implementation contract.
- Implementation Contract: Contains the logic and functions of the smart contract.
- Storage Separation: Ensures that the proxy contract retains the storage layout while logic can be updated.
How Do They Work?
The mechanism for upgrading contracts is fairly simple:
- The proxy contract holds the state.
- The implementation contract holds the logic (functions).
- When an upgrade is needed, a new implementation contract is deployed and the proxy contract is updated to point to the new implementation.
What Are Proxy Patterns?
A proxy pattern allows one contract (the proxy) to delegate calls to another contract (the implementation), separating the storage and logic layers. This design enables the logic to be updated while retaining the same address and storage, ensuring continuity and upgradability.
Now, let’s explore the different proxy patterns used to implement upgradable contracts.we’ll explore upgradable smart contracts and dive deep into the proxy patterns that make upgradability possible, such as:
- UUPS Pattern
- Simple Proxy Pattern
- Transparent Proxy Pattern
- Beacon Proxy Pattern
- Diamond Proxy Pattern
Each pattern will be explained in detail, with practical examples, so you can understand how to implement them in your projects.
1. UUPS (Universal Upgradeable Proxy Standard) Pattern
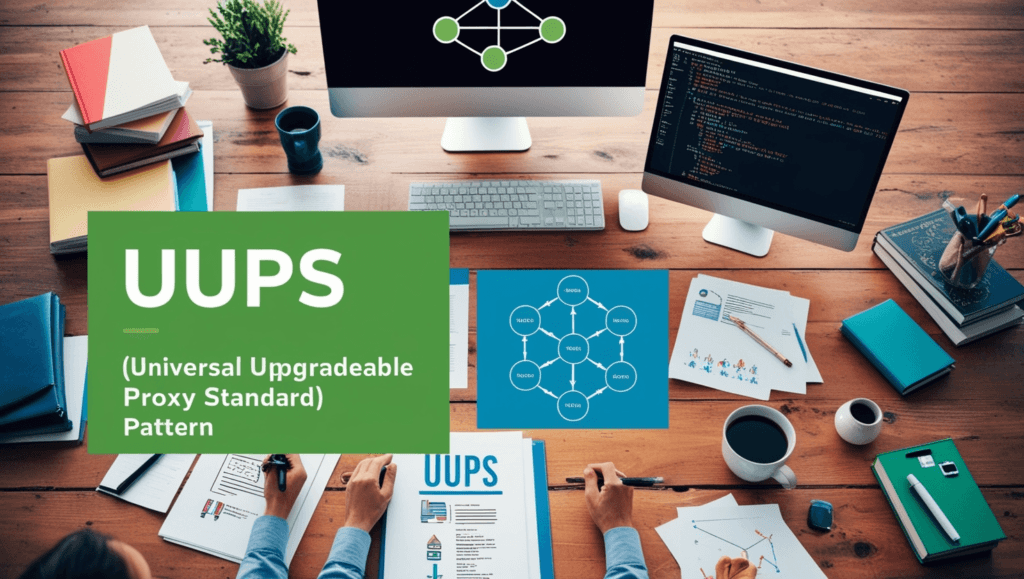
The UUPS pattern is one of the most efficient ways to implement upgradable smart contracts. This pattern integrates the upgrade logic into the implementation contract itself, making it cost-efficient.
How It Works:
- The implementation contract includes an upgrade function.
- The proxy contract points to the implementation contract and forwards function calls.
- The upgrade function allows the proxy to point to a new implementation contract.
Example:
Old Implementation Contract (Before Upgrade):
pragma solidity ^0.8.26;
contract OldImplementation {
uint public value;
// Function to set the value
function setValue(uint _value) public {
value = _value;
}
}
This is a basic implementation contract that has a value
variable and a function to set the value.
New Implementation Contract (After Upgrade):
pragma solidity ^0.8.26;
contract NewImplementation {
uint public value;
// Function to set the value with an added logic
function setValue(uint _value) public {
value = _value + 1; // New logic (increased by 1)
}
}
In the new implementation, we modified the setValue
function. Instead of setting the value directly, we increment it by 1.
Proxy Contract:
pragma solidity ^0.8.26;
contract Proxy {
address public implementation;
constructor(address _implementation) {
implementation = _implementation; // Initial implementation contract
}
// Upgrade to a new implementation
function upgradeTo(address newImplementation) public {
implementation = newImplementation;
}
// Fallback function to forward calls to the implementation contract
fallback() external payable {
(bool success, ) = implementation.delegatecall(msg.data);
require(success);
}
}
Explanation:
- The
Proxy
contract stores the address of the current implementation contract. - The
upgradeTo
function is used to update the implementation contract. - The
fallback
function forwards calls to the current implementation contract using thedelegatecall
function. This keeps the contract’s state intact while executing the logic in the implementation contract.
2. Simple Proxy Pattern
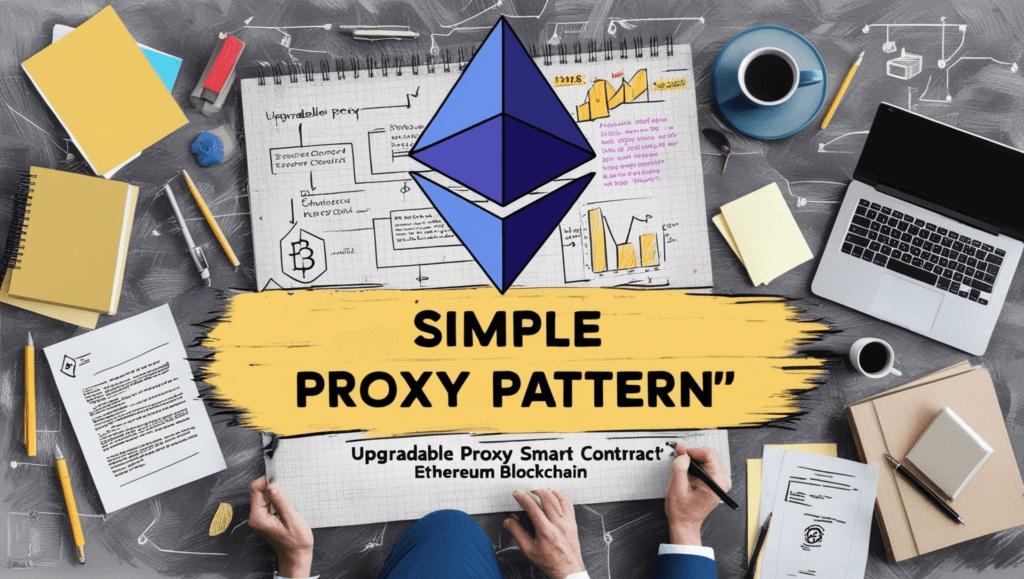
The Simple Proxy Pattern is the most basic and straightforward pattern for making smart contracts upgradable. It delegates all function calls to the implementation contract using delegatecall
.
How It Works:
- The proxy contract forwards function calls to the implementation contract.
- The state remains in the proxy contract.
Example:
Implementation Contract:
pragma solidity ^0.8.26;
contract Implementation {
uint public value;
// Function to set the value
function setValue(uint _value) public {
value = _value;
}
}
The Implementation
contract defines a simple setValue
function that sets a value.
Proxy Contract:
pragma solidity ^0.8.26;
contract Proxy {
address public implementation;
constructor(address _implementation) {
implementation = _implementation; // Set the initial implementation address
}
// Fallback function to forward calls to the implementation contract
fallback() external payable {
(bool success, ) = implementation.delegatecall(msg.data);
require(success);
}
}
Explanation:
- The
Proxy
contract stores the address of theImplementation
contract. - The
fallback
function forwards all calls made to the proxy to theImplementation
contract usingdelegatecall
.
3. Transparent Proxy Pattern
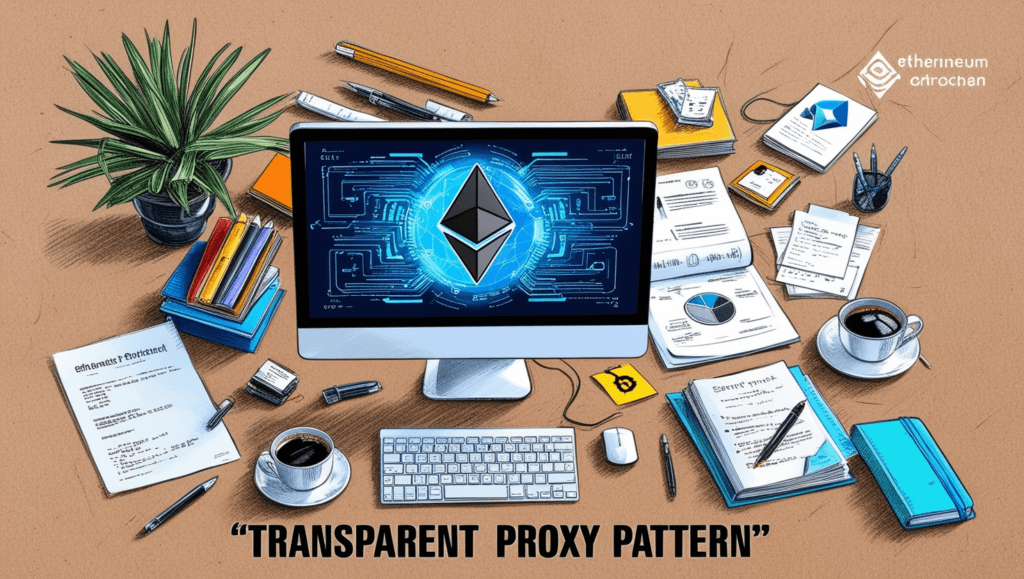
The Transparent Proxy Pattern adds an admin role to control upgrades. Only the admin can upgrade the contract, making the system more secure.
How It Works:
- The proxy contract forwards calls to the implementation contract.
- Only the admin can change the implementation contract.
Example:
Implementation Contract:
pragma solidity ^0.8.26;
contract Implementation {
uint public value;
// Function to set the value
function setValue(uint _value) public {
value = _value;
}
}
Proxy Contract with Admin Control:
pragma solidity ^0.8.26;
contract Proxy {
address public implementation;
address public admin;
modifier onlyAdmin() {
require(msg.sender == admin, "Not the admin");
_;
}
constructor(address _implementation) {
implementation = _implementation;
admin = msg.sender; // Set the contract deployer as the admin
}
// Admin can upgrade the implementation
function upgradeTo(address newImplementation) public onlyAdmin {
implementation = newImplementation;
}
// Fallback function to forward calls to the implementation contract
fallback() external payable {
(bool success, ) = implementation.delegatecall(msg.data);
require(success);
}
}
Explanation:
- The
Proxy
contract stores the address of the implementation contract and includes anonlyAdmin
modifier to restrict who can upgrade the contract. - The
upgradeTo
function is used by the admin to change the implementation contract. - The
fallback
function forwards the call to the current implementation contract.
4. Beacon Proxy Pattern
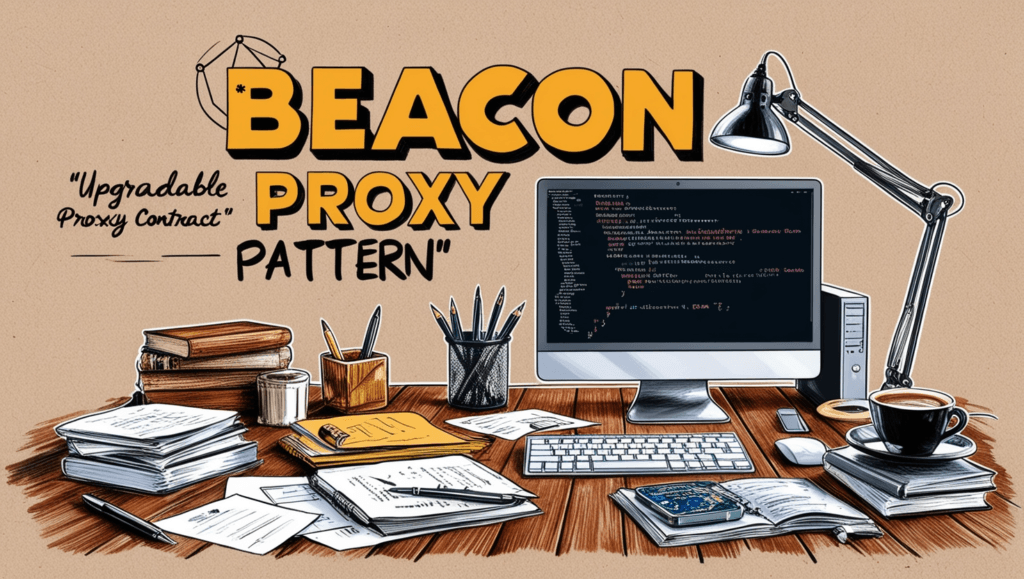
The Beacon Proxy Pattern introduces a beacon contract that holds the address of the implementation contract. Multiple proxy contracts can refer to the same beacon.
How It Works:
- The beacon contract stores the address of the implementation contract.
- Multiple proxies can refer to the same beacon to get the implementation address.
Example:
Beacon Contract:
pragma solidity ^0.8.26;
contract Beacon {
address public implementation;
// Function to set the implementation address
function setImplementation(address newImplementation) public {
implementation = newImplementation;
}
}
Proxy Contract:
pragma solidity ^0.8.26;
contract Proxy {
Beacon public beacon;
constructor(address _beacon) {
beacon = Beacon(_beacon);
}
// Fallback function to forward calls to the implementation contract from the beacon
fallback() external payable {
address implementation = beacon.implementation();
(bool success, ) = implementation.delegatecall(msg.data);
require(success);
}
}
Explanation:
- The
Beacon
contract holds the implementation contract address. - The
Proxy
contract references the beacon to get the latest implementation address. - The
fallback
function delegates calls to the implementation contract obtained from the beacon.
5. Diamond Proxy Pattern
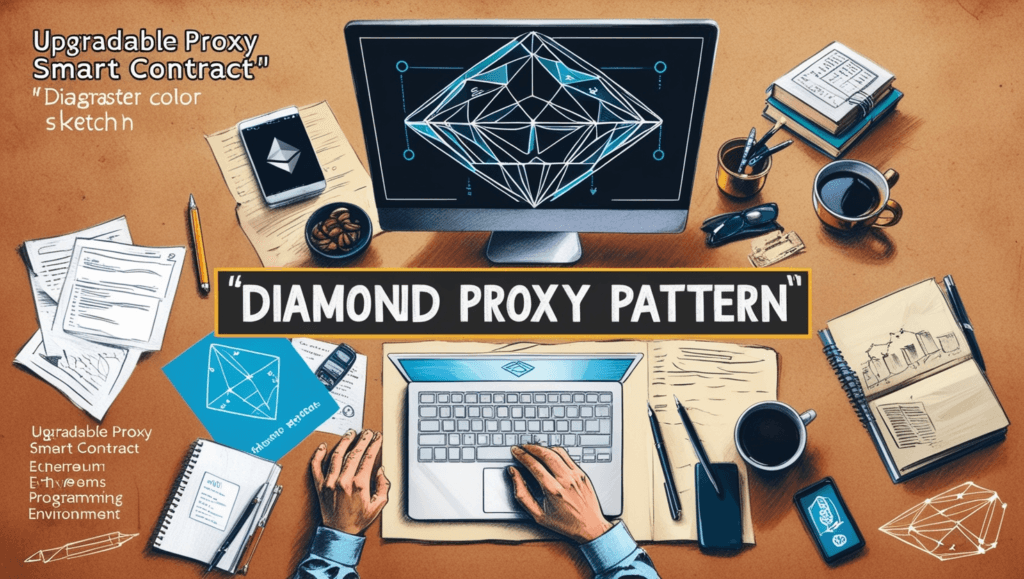
The Diamond Proxy Pattern is a more complex pattern that enables multiple facets (or functionalities) to be managed through a single proxy.
How It Works:
- The proxy delegates calls to various facets (contracts) that handle different parts of the logic.
- The proxy stores references to multiple facets and delegates calls accordingly.
Example:
Facet Contract (Account Management):
pragma solidity ^0.8.26;
contract AccountFacet {
uint public accountBalance;
// Function to deposit into the account
function deposit(uint amount) public {
accountBalance += amount;
}
}
Proxy Contract:
pragma solidity ^0.8.26;
contract DiamondProxy {
mapping(bytes4 => address) public facets;
// Function to set the facet address for a specific function selector
function setFacet(bytes4 selector, address facetAddress) public {
facets[selector] = facetAddress;
}
// Fallback function to delegate calls to the appropriate facet
fallback() external payable {
address facet = facets[msg.sig];
require(facet != address(0), "Facet not found");
(bool success, ) = facet.delegatecall(msg.data);
require(success);
}
}
Explanation:
- The
DiamondProxy
contract maintains a mapping of function selectors (the first 4 bytes of the function signature) to facet addresses. - The
setFacet
function allows adding or updating the facet for specific functions. - The
fallback
function delegates the calls to the correct facet based on the function selector.
How to Choose the Right Pattern?
Here’s a quick cheat sheet:
- UUPS: Lightweight and cost-effective for simple upgrades.
- Simple Proxy: Best for straightforward applications with minimal upgradability needs.
- Transparent Proxy: Adds better control for upgrades.
- Beacon Proxy: Perfect for managing upgrades across multiple instances.
- Diamond Proxy: Ideal for highly modular, scalable systems.
Comparison of Proxy Patterns
Pattern | Best For | Advantages | Challenges |
---|---|---|---|
Simple Proxy | Small contracts | Easy to implement | No access control |
Transparent Proxy | Controlled upgrades | Governance-ready | Slightly higher gas costs |
UUPS | Gas efficiency | Lightweight proxy | Requires careful upgrades |
Beacon Proxy | Multi-contract upgrades | Centralized logic management | Beacon reliability |
Diamond Proxy | Complex applications | Modular upgrades | High complexity |
Best Practices for Upgradable Smart Contracts
- Maintain Storage Compatibility: Ensure storage variables in the new implementation match the previous layout.
- Use OpenZeppelin Libraries: OpenZeppelin provides pre-audited tools to reduce security risks.
- Test Extensively: Simulate upgrades in different environments to identify potential issues.
- Avoid Complex Storage Layouts: Simple storage structures minimize the risk of conflicts during upgrades.
Common Mistakes to Avoid
- Ignoring Storage Layout: Overwriting storage variables can corrupt existing data.
- Skipping Security Audits: Upgradable contracts introduce complexity, requiring rigorous audits.
- Insufficient Testing: Always test both deployment and upgrade processes thoroughly.
Real-World Applications of Upgradable Smart Contracts
- DeFi Protocols: Protocols like Aave and Compound frequently update their smart contracts to introduce new features or improve security.
- NFT Marketplaces: Platforms like OpenSea leverage upgradable contracts for scalability and feature additions.
- DAO Governance: Upgradable contracts enable DAOs to adapt governance models without disrupting operations.
Challenges and Considerations
- Increased Complexity: Proxy patterns introduce additional layers of complexity.
- Potential Vulnerabilities: The upgradeability process can be exploited if not properly secured.
- Developer Expertise: Requires a deep understanding of Solidity and proxy patterns.
Conclusion
Upgradable smart contracts are essential for maintaining flexibility and extensibility in decentralized applications. By using proxy patterns such as UUPS, Simple Proxy, Transparent Proxy, Beacon Proxy, and Diamond Proxy, developers can ensure that their contracts remain upgradeable without losing data.
Each of the patterns discussed provides a unique way to handle upgrades, but all aim to keep the contract’s logic modular and easily updatable. Depending on the use case, a developer might choose one pattern over another to balance simplicity, security, and functionality.