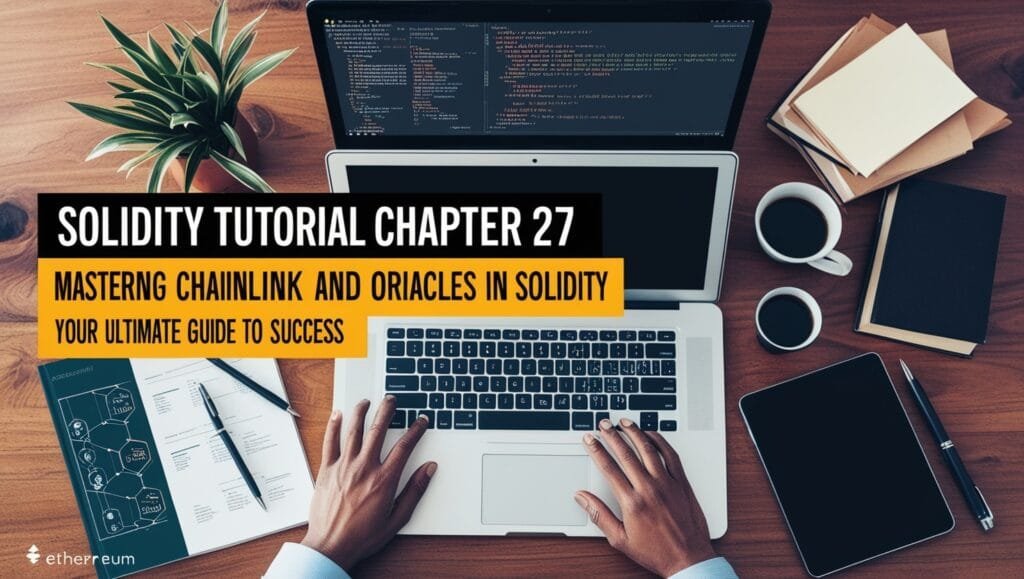
Solidity Tutorial Chapter 27: Mastering Chainlink and Oracles in Solidity: Your Ultimate Guide to Success
Chainlink and Oracles in Solidity: Smart contracts are revolutionizing industries by automating trustless transactions without the need for intermediaries. However, one of the biggest challenges in the world of blockchain is that smart contracts, by default, cannot access real-world data on their own. This is where oracles come into play, acting as a bridge between the blockchain and the external world.
In this tutorial, we will dive into the essential role oracles play in Solidity development, particularly with Chainlink—the leading decentralized oracle network. By the end of this tutorial, you will understand how to implement oracles in Solidity, the Chainlink Price Oracle, and advanced use cases that integrate real-time data into your smart contracts.
We will cover:
- What oracles are and why they are needed in smart contracts.
- The functionality of Chainlink oracles in Solidity.
- Smart contract security considerations when working with oracles.
- Real-world examples of working with blockchain oracles using Chainlink.
- How to implement Chainlink and Oracle in Solidity step-by-step.
Let’s dive in!
Table of Contents
What Are Oracles in the Context of Blockchain and Smart Contracts?
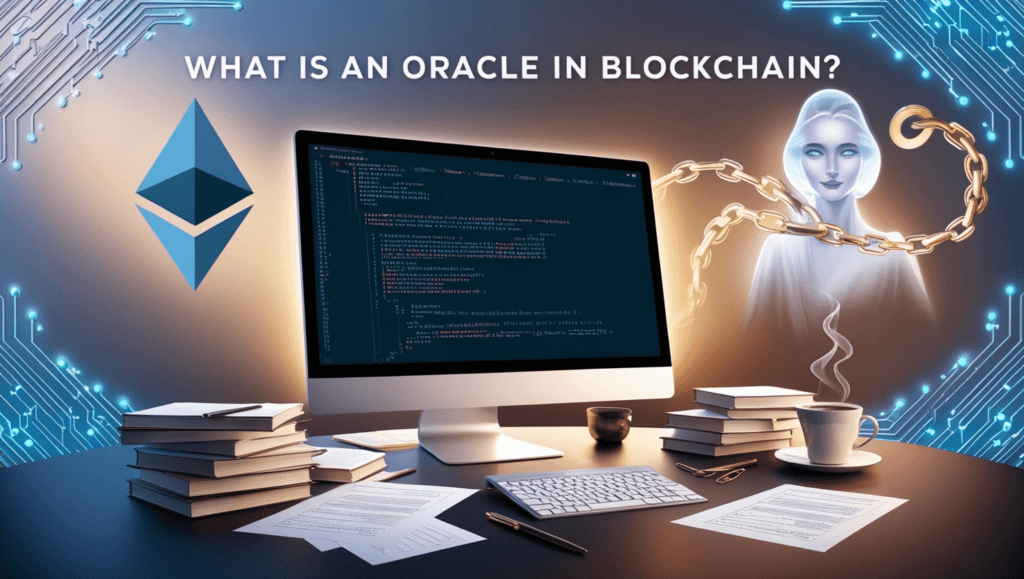
Before we dive into the technical aspects of using Chainlink and oracles in Solidity, let’s first define what oracles are and why they are crucial to smart contract functionality.
Definition of Oracles
In the world of blockchain technology, an oracle is a service that provides external data to smart contracts. Smart contracts, by design, can only interact with data that exists on the blockchain itself. However, many smart contracts need information from the outside world to function properly, such as weather data, stock prices, or even the result of a soccer match. This is where oracles come in: they fetch the required data and deliver it to the blockchain, allowing the smart contract to execute its functions based on real-world conditions.
Why Are Oracles Needed in Smart Contracts?
Smart contracts, by definition, are programs that automatically execute terms of an agreement when certain conditions are met. Without access to real-world data, smart contracts would be confined to a small set of functions. Oracles provide the bridge between the on-chain and off-chain worlds, enabling a new range of decentralized applications (dApps), especially in sectors like DeFi (Decentralized Finance), gaming, insurance, and supply chain management.
Types of Oracles
Oracles can be classified into several types:
- Inbound Oracles: These provide data from external sources to the blockchain. For example, stock prices, weather data, and sports scores.
- Outbound Oracles: These send data from the blockchain to external systems or APIs. For instance, sending a command to an IoT device to perform a certain action.
- Software Oracles: These interact with online services and APIs to gather data like financial data or sports scores.
- Hardware Oracles: These connect physical sensors or devices to the blockchain.
- Consensus-Based Oracles: These aggregate data from multiple sources to ensure accuracy and prevent tampering.
In this tutorial, we will focus on software oracles, particularly Chainlink—which is the most widely used decentralized oracle network for Solidity developers.
Chainlink: The Gold Standard in Blockchain Oracles
Chainlink is the industry leader when it comes to decentralized oracles. It allows smart contracts to securely connect with real-world data, APIs, and payment systems, enabling them to respond to real-world events. Chainlink provides tamper-proof data feeds and is highly regarded for its oracle Ethereum integration.
Key Features of Chainlink Oracles
- Decentralization: Chainlink aggregates data from multiple nodes, making it more resilient to failure and tampering compared to centralized oracles.
- Data Integrity: Chainlink uses cryptographic proofs to validate data, ensuring it is accurate and trustworthy.
- Cross-Chain Compatibility: Chainlink is compatible with multiple blockchain platforms, including Ethereum, Polkadot, Binance Smart Chain, and many others.
- Verifiable Random Functions (VRF): Chainlink provides secure random number generation for decentralized applications like gaming and lotteries.
- Reliable Price Feeds: Chainlink’s price oracle is widely used in DeFi applications to fetch real-time price data for cryptocurrencies, stocks, commodities, and more.
How to Work with Chainlink and Oracles in Solidity?
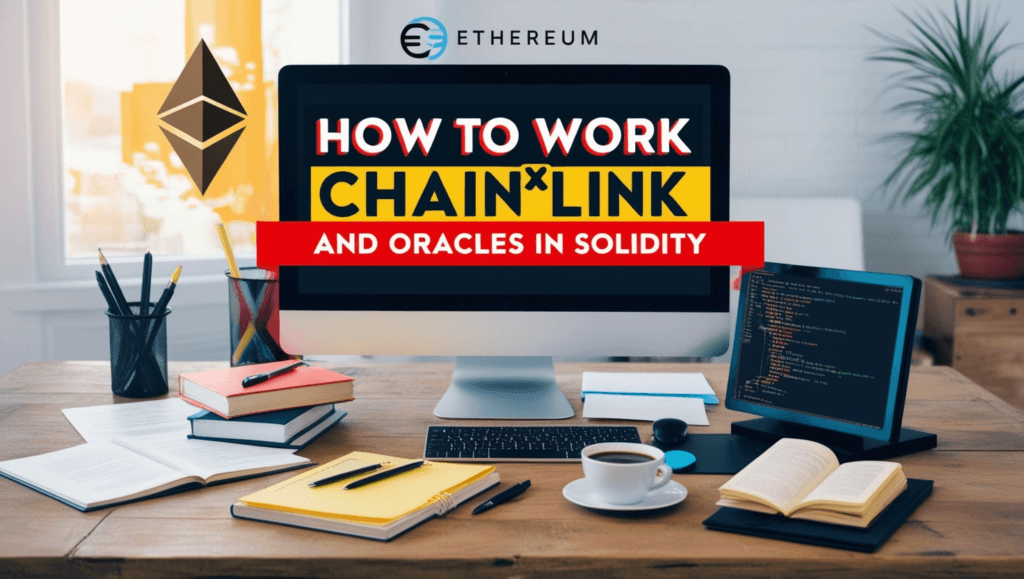
Now that we understand what oracles are and why Chainlink is the go-to solution for Ethereum smart contracts, let’s move into the practical part of this tutorial: Implementing Oracles in Solidity.
Example 1: Fetching the Price of ETH Using Chainlink’s Price Oracle
One of the most common use cases for Chainlink oracles in Solidity is to fetch real-time asset prices. Let’s create a Solidity contract that will fetch the current price of ETH in USD using Chainlink’s price oracle.
Step 1: Import Chainlink’s Aggregator Interface
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@chainlink/contracts/src/v0.8/interfaces/AggregatorV3Interface.sol";
Step 2: Create the Contract
contract PriceFeedExample {
AggregatorV3Interface internal priceFeed;
// Address for the Kovan ETH/USD price feed
constructor() {
priceFeed = AggregatorV3Interface(0x9326BFA02ADD2366b30bacB125260Af641031331); // Kovan ETH/USD price feed
}
// Returns the latest ETH price
function getLatestPrice() public view returns (int) {
(
,
int price,
,
,
) = priceFeed.latestRoundData();
return price; // Returns price with 8 decimal places
}
}
Step 3: Deploy and Test
- Deploy the contract on a testnet such as Kovan.
- Call the
getLatestPrice
function to fetch the current ETH/USD price.
Example 2: Using Chainlink’s Verifiable Random Function (VRF)
Another popular use case for Chainlink oracles is generating random numbers securely. Let’s look at how you can use Chainlink’s VRF to generate verifiable random numbers in a Solidity smart contract.
Step 1: Import Chainlink VRF
import "@chainlink/contracts/src/v0.8/VRFConsumerBase.sol";
Step 2: Create the Contract
contract RandomNumberGenerator is VRFConsumerBase {
bytes32 internal keyHash;
uint256 internal fee;
uint256 public randomResult;
constructor() VRFConsumerBase(
0x6168499e3d1deeb309b2ed3d394fe6d13edbd348, // VRF Coordinator
0xa36085F69e2889c224210F603D836748e7dC0088 // LINK Token
) {
keyHash = 0x6c3699283bda56ad74f6a5d20d727bdeaf8e90e1b88813a9e9c1695b1e5e7a51;
fee = 0.1 * 10 ** 18; // 0.1 LINK
}
function getRandomNumber() public returns (bytes32 requestId) {
require(LINK.balanceOf(address(this)) >= fee, "Not enough LINK");
return requestRandomness(keyHash, fee);
}
function fulfillRandomness(bytes32 requestId, uint256 randomness) internal override {
randomResult = randomness;
}
}
Step 3: Test the Random Number Generator
- Deploy the contract to a test network.
- Use the
getRandomNumber
function to request a random number. - The contract will generate and store a random number that is verifiable and secure.
Example 3: Fetching Data from a Custom API with Chainlink
Chainlink also allows developers to fetch data from custom APIs. Here’s how you can make a request to a third-party API using Chainlink’s External Adapter feature.
Step 1: Import Chainlink Client
import "@chainlink/contracts/src/v0.8/ChainlinkClient.sol";
Step 2: Create the Contract
contract APIConsumer is ChainlinkClient {
uint256 public data;
address private oracle;
bytes32 private jobId;
uint256 private fee;
constructor() {
setPublicChainlinkToken();
oracle = 0x...; // Oracle address
jobId = "7d80a6386ef543a3abb52817f6707e3b"; // Job ID for the external API request
fee = 0.1 * 10 ** 18; // 0.1 LINK
}
function requestData() public returns (bytes32 requestId) {
Chainlink.Request memory request = buildChainlinkRequest(jobId, address(this), this.fulfill.selector);
request.add("get", "https://api.example.com/data");
request.add("path", "data.value");
return sendChainlinkRequestTo(oracle, request, fee);
}
function fulfill(bytes32 _requestId, uint256 _data) public recordChainlinkFulfillment(_requestId) {
data = _data;
}
}
Smart Contract Security Considerations
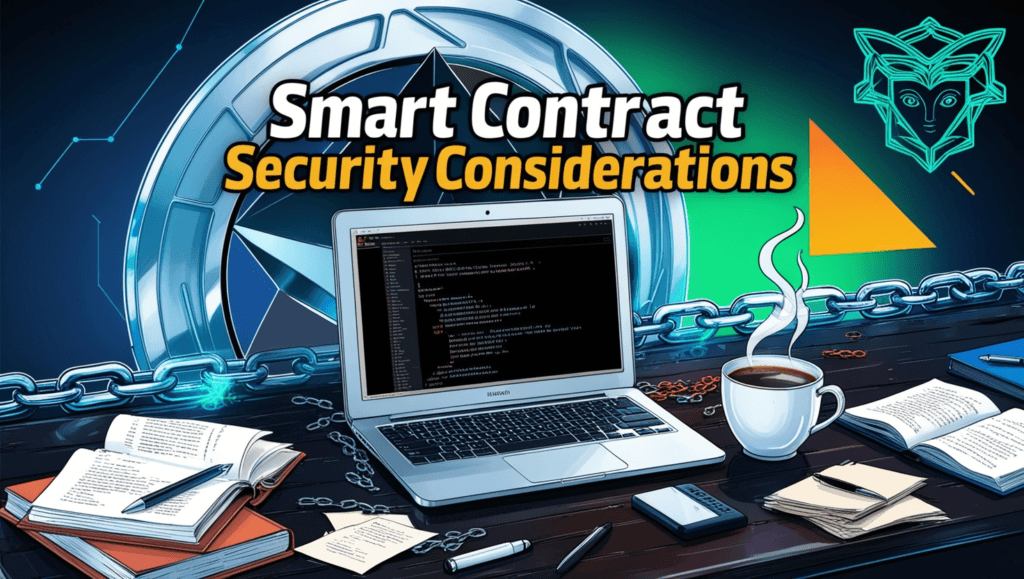
When working with oracles in Solidity, particularly Chainlink oracles, security is paramount. Smart contract security is a crucial aspect of any blockchain application, and oracles introduce additional risks that developers must be mindful of.
Key Security Best Practices:
- Data Integrity: Always ensure the data being fed into your contract is coming from a reliable and secure oracle like Chainlink.
- Fallback Mechanisms: Implement failover mechanisms to handle scenarios where the oracle might be down or malfunctioning.
- Gas Fees: Oracles, especially decentralized ones like Chainlink, require gas to make a request. Ensure your contract has sufficient funds to avoid failures.
- Audit and Test: Always audit and test your contract thoroughly, especially when interacting with third-party oracles.
Conclusion
In this Solidity tutorial, we’ve explored how to work with Chainlink and oracles in Solidity. By the end of this article, you should have a solid understanding of how Chainlink and oracles empower smart contracts to interact with real-world data. Whether you’re implementing price oracles, VRF, or custom API requests, Chainlink offers a powerful, decentralized solution to bridge the gap between blockchain and external systems.
As blockchain technology continues to evolve, oracles will play an increasingly critical role in building more advanced and reliable smart contracts. Whether you’re creating a DeFi application, a gaming contract, or any dApp that requires real-world data, understanding how to implement and use Chainlink oracles is a crucial skill for any Solidity developer.