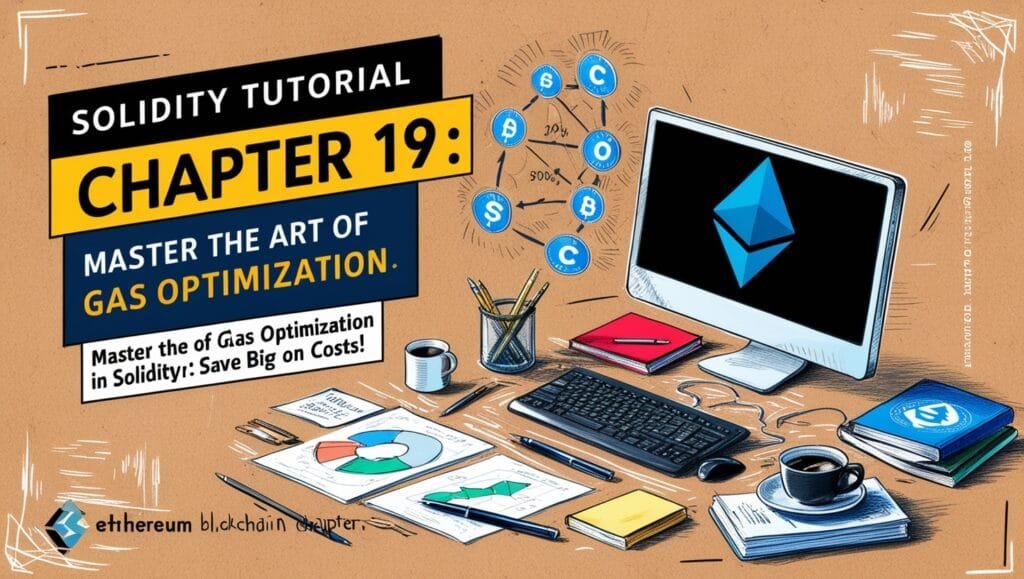
Solidity Tutorial Chapter 19: Master the Art of Gas Optimization in Solidity: Save Big on Costs!
Solidity Tutorial Chapter 19:Gas Optimization in Solidity – When it comes to Solidity, managing gas costs is essential. Gas fees can add up, especially for complex smart contracts or high-frequency operations. In this guide, we’ll explore some practical gas optimization techniques in Solidity, so you can make your contracts as efficient (and wallet-friendly) as possible.
Table of Contents
Why Gas Optimization Matters in Solidity
To understand why gas optimization is so critical in Solidity, let’s look at what gas really is. When you interact with the Ethereum blockchain, every operation in your smart contract costs a small amount of “gas.” This gas has a real cost in Ether (ETH) and can quickly become expensive, especially if the contract is poorly optimized. Optimizing gas is not just about saving ETH—it also makes your contracts faster and more attractive to users. A contract with lower gas fees is one that users are more likely to engage with, which is good news for any Solidity developer.
Practical Gas Optimization Techniques in Solidity
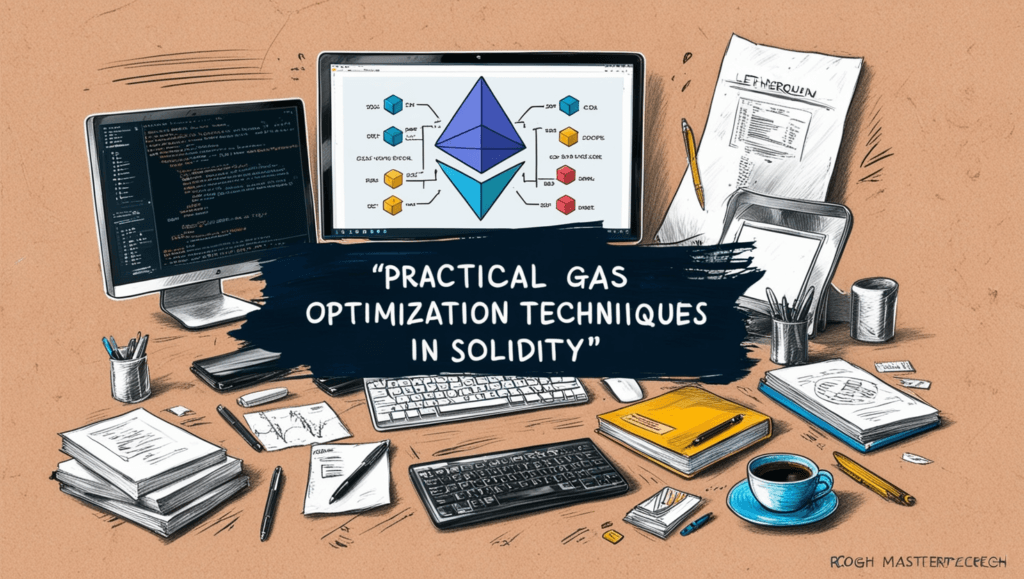
1. Use calldata
over memory
for External Function Calls
When dealing with function parameters in Solidity, especially with functions marked as external
, consider using calldata
instead of memory
.
- Why?
calldata
is non-modifiable and points to data directly, which saves memory space and gas. - Example:
function processNames(string calldata name) external returns(bool) {
// Processing...
}
Using calldata
can lower gas costs significantly compared to memory
, especially for large data structures.
2. Avoid Using Loops Whenever Possible
Loops (like for
and while
) can be tempting, but they’re gas-hungry in Solidity. Since each iteration costs gas, loops can quickly inflate transaction costs, particularly with large datasets.
- Tip: If you must use loops, keep them short and avoid nested loops.
- Alternative: For tasks that require repetitive actions, consider off-chain solutions or limit loop usage to essential operations only.
3. Use Fixed-Size Arrays and Structs
Solidity gives you options to use both fixed-size and dynamic-size arrays. Fixed-size arrays and structs are generally more gas-efficient than dynamic ones.
- Example
uint[5] fixedArray;
- Reason: Fixed sizes require less storage because the length is predetermined. For complex structures, keeping arrays fixed-sized ensures more predictable and lower gas fees.
4. Leverage Bitwise Operations
For contracts that involve mathematical calculations or checks, bitwise operations are often faster and cheaper than arithmetic.
- Example: Instead of using
x / 2
, considerx >> 1
, which is cheaper. - Why: Bitwise operations work directly with binary data and consume less gas than traditional operations.
5. Optimize Storage Accesses
Gas fees are significantly higher when accessing storage variables compared to memory variables. Since storage is a persistent data layer, each read or write operation incurs higher gas costs.
- Tip: Minimize the use of
storage
in functions, especially within loops. - Example
// Expensive
for (uint i = 0; i < data.length; i++) {
data[i] = i;
}
// Cheaper
uint length = data.length;
for (uint i = 0; i < length; i++) {
data[i] = i;
}
By moving data.length
to memory, we reduce storage access costs in each iteration.
6. Use Events to Store Data Instead of Storage Variables
For data that doesn’t need to be retrieved frequently, using events can save gas. Events are stored in logs, which are cheaper to store and do not consume storage fees in the same way as state variables.
- Example
event NewData(uint256 indexed id, string value);
function addData(uint256 id, string calldata value) external {
emit NewData(id, value);
}
Events are perfect for one-time information storage that isn’t directly queried in smart contracts but is useful for external analysis or logging.
Advanced Gas Optimization Techniques
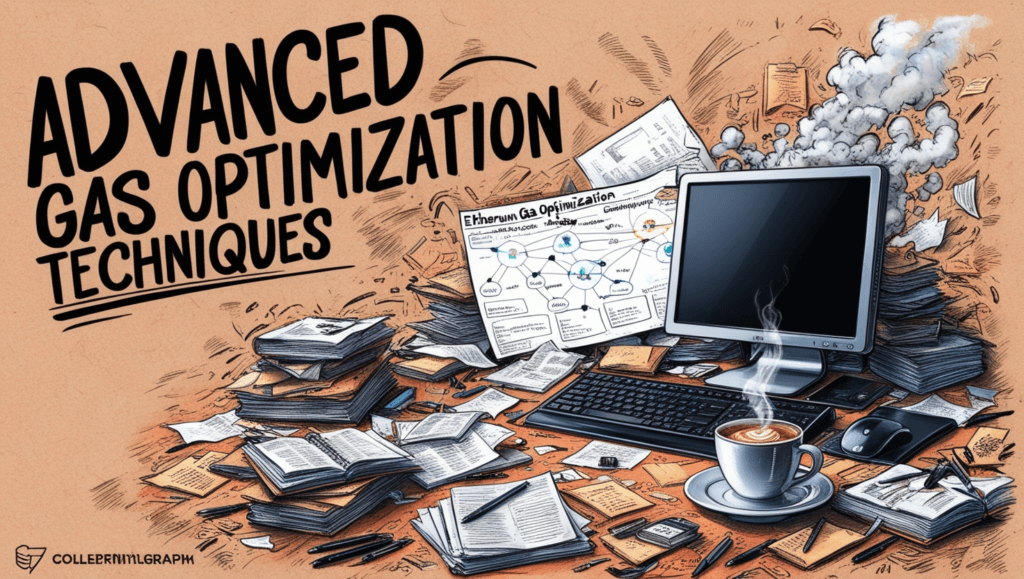
7. Packing Structs and Variables
The Ethereum Virtual Machine (EVM) stores data in 32-byte slots, so packing smaller data types together can reduce storage usage.
- Tip: Group similar data types and sizes together to avoid empty slots.
- Example
struct Optimized {
uint16 a;
uint16 b;
uint32 c;
bool d;
}
By packing variables into the smallest possible slots, you reduce the amount of storage used and thus lower gas costs.
8. Use Inline Assembly for Gas-Intensive Logic
For particularly gas-heavy sections, you can use inline assembly. Assembly lets you directly access the EVM’s low-level opcodes, providing full control and minimizing gas usage.
- Example:
function add(uint x, uint y) public pure returns (uint result) {
assembly {
result := add(x, y)
}
}
- Caution: Inline assembly can be tricky to read and maintain, so only use it when the benefits outweigh the complexity.
9. Implement a Gas Limit Check
Sometimes, especially for functions that users might trigger repeatedly, setting a gas limit check helps avoid excessive gas usage.
- How: Set a
gasLimit
parameter that prevents the function from running if it exceeds a certain threshold. - Example
modifier limitedGas(uint gasLimit) {
require(gasleft() <= gasLimit, "Gas limit exceeded!");
_;
}
This helps in controlling function execution and saving costs on repeated invocations.
Common Misconceptions About Gas Optimization
Myth #1: Optimization is Always Necessary
While gas optimization can reduce costs, over-optimizing every function may make code harder to read and maintain. It’s best to prioritize optimization for functions that are called frequently or involve complex operations.
Myth #2: More Lines Mean More Gas
The number of lines doesn’t necessarily affect gas costs—it’s the specific operations you use. Simple, well-optimized code often performs better than complex, overly compressed code.
Myth #3: Only Developers Need to Worry About Gas
Anyone interacting with Solidity contracts benefits from gas optimization. For example, dApps with high gas costs can deter users, so optimizing gas usage makes your contracts more appealing.
Wrapping Up: Why Gas Optimization Makes a Big Difference
Gas optimization is more than a technical detail—it’s key to building efficient, user-friendly contracts. Optimized gas usage keeps your contracts lightweight, reduces user costs, and makes your dApp more competitive. Each of the techniques we covered today can be applied independently or combined for even better results.
For Solidity developers, being mindful of gas is part of what makes the difference between a good contract and a great one. By integrating these techniques into your coding practices, you can build contracts that are both powerful and efficient, setting your project up for long-term success.
These gas optimization techniques should serve as a handy toolkit to help you manage gas costs in Solidity. Keep exploring and tweaking to see how each method impacts your unique project needs.