Upgradable Smart Contracts in Solidity: With blockchain technology constantly evolving, it’s essential to have smart contracts that are flexible and updatable. Traditionally, smart contracts on Ethereum are immutable — once deployed, they can’t be changed. While this immutability is great for security, it makes it hard to fix bugs, add new features, or improve performance over time.
Enter upgradable smart contracts. This approach provides flexibility and future-proofing for smart contracts, allowing developers to upgrade functionality while retaining the original contract’s address and data. In this guide, we’ll break down how upgradable smart contracts in Solidity work, why they’re important, and the best practices for keeping them secure.
Table of Contents
Why Upgradable Smart Contracts Matter
When you’re building complex Decentralized Applications (DApps) on Ethereum, you don’t want to be stuck with bugs or limited functionality. Upgradable smart contracts solve this by separating a contract’s code from its data, allowing for changes to be made later. This is especially valuable for DeFi (decentralized finance) projects, NFTs, and other blockchain-based apps that may need to adapt over time.
Let’s dive into how smart contract upgrades work in Solidity and how they enable developers to manage and deploy flexible code in the blockchain technology space.
Key Components: Proxy and Implementation Contracts
At the core of upgradable contracts are proxy contracts and implementation contracts. Here’s what each does:
- Proxy Contract: This contract is the “face” of your DApp. Users interact with the proxy, which forwards requests to the implementation contract. The proxy’s address remains constant, meaning the DApp’s front end doesn’t need updating whenever the contract is upgraded.
- Implementation Contract: This holds the logic (i.e., the code) that the proxy calls. You can replace this implementation contract with a new one, allowing you to modify your app’s functionality without affecting users.
This approach also enables transparent upgrades — end-users won’t even notice that the backend has been upgraded, as the user experience remains unchanged.
Step-by-Step Guide to Creating Upgradable Smart Contracts in Solidity
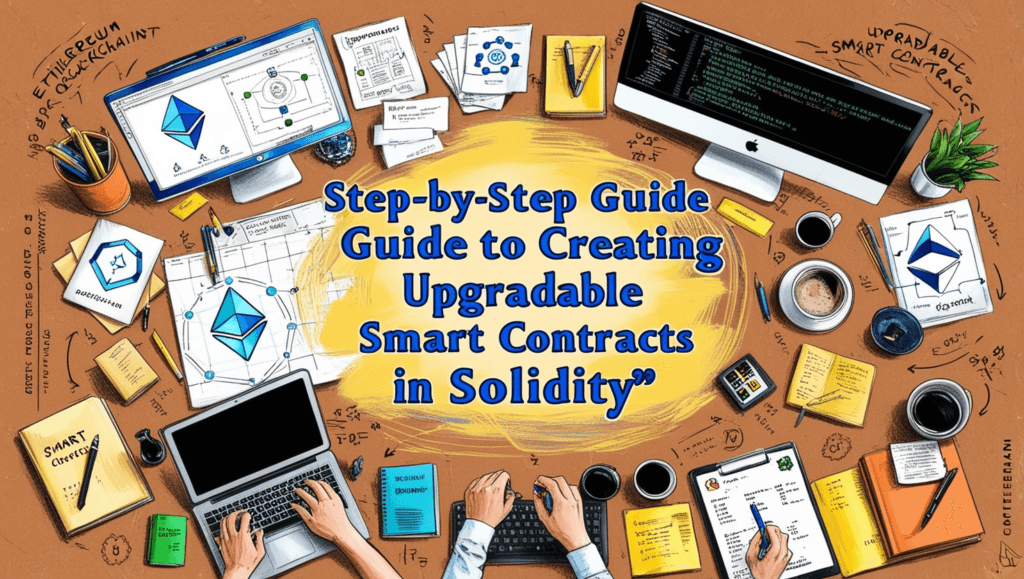
Now, let’s walk through how to set up upgradable smart contracts in Solidity.
1. Set Up the Proxy Contract
The proxy contract acts as a delegate. By using Solidity’s delegatecall
feature, the proxy calls the implementation’s code while keeping the proxy’s own storage. This way, the data is maintained even when we switch the implementation.
Here’s a basic example of a proxy contract:
pragma solidity ^0.8.0;
contract Proxy {
address public implementation;
function upgrade(address newImplementation) public {
implementation = newImplementation;
}
fallback() external payable {
(bool success, ) = implementation.delegatecall(msg.data);
require(success, "delegatecall failed");
}
}
In this proxy setup, we define an upgrade
function that updates the implementation
address. When delegatecall
is used, all calls to the proxy contract will be executed by the implementation contract.
2. Create the Implementation Contract
The implementation contract contains your business logic. Here’s an example:
pragma solidity ^0.8.0;
contract Implementation {
uint public version;
function setVersion(uint _version) public {
version = _version;
}
}
Deploy the implementation contract with your initial logic. When you need to upgrade, you’ll deploy a new version of this contract and point the proxy to it.
3. Deploy and Link the Contracts
To deploy an upgradable smart contract, deploy the implementation contract first, then deploy the proxy contract, setting the initial implementation address in the proxy. This setup is ready to handle future updates without disrupting the end-users.
Upgrade Mechanisms: Transparent and UUPS Upgrades
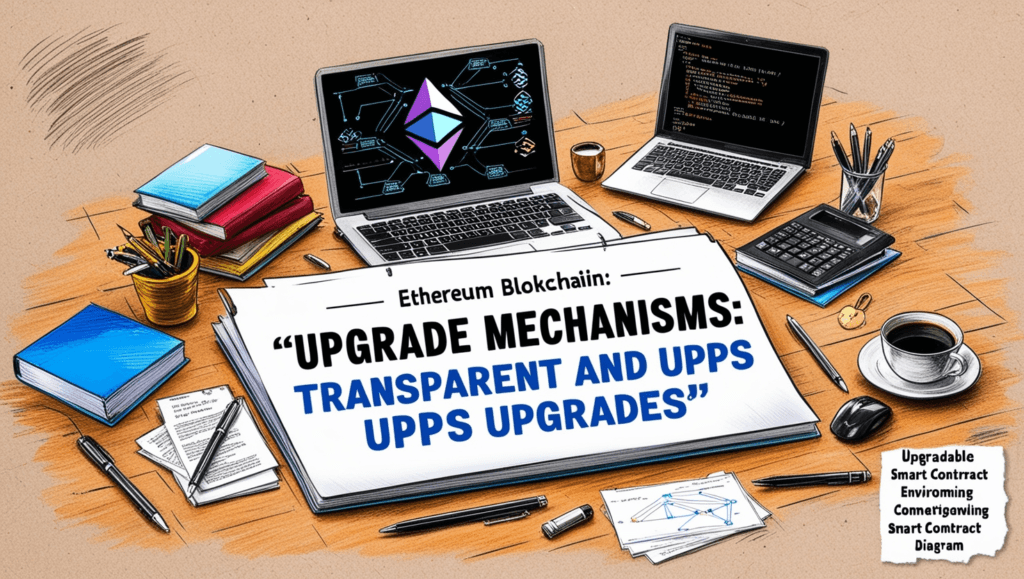
There are two popular ways to handle upgrades in Solidity: Transparent Upgrades and UUPS (Universal Upgradeable Proxy Standard).
- Transparent Upgrades: Only an admin can upgrade the implementation, providing a secure and controlled process. This is widely used in DeFi applications for security.
- UUPS Upgrades: In UUPS, the contract itself can handle upgrades. It’s often more efficient and saves on gas, especially when frequent upgrades are needed. UUPS upgrades are commonly used in NFTs and DeFi due to their performance benefits.
How to Upgrade the Smart Contract?
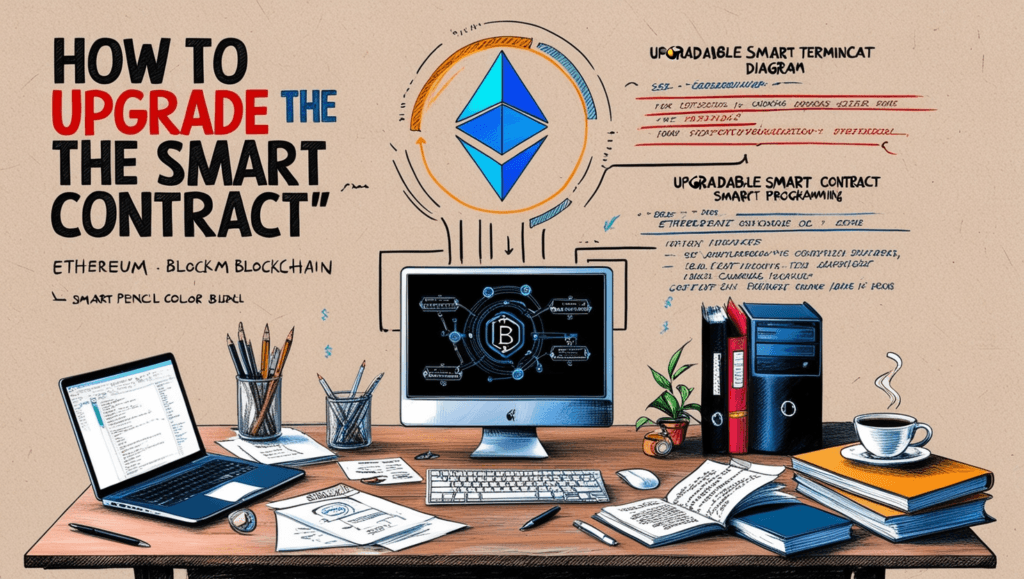
When a new version of the implementation contract is needed, deploy it and link it with the proxy contract by calling the upgrade
function in the proxy. This keeps the contract’s state intact but updates the logic.
Example of upgrading with the proxy:
Proxy proxy = new Proxy();
Implementation newImplementation = new Implementation();
proxy.upgrade(address(newImplementation));
This way, all data and the contract address remain the same, but users can interact with the latest features and fixes.
Security Considerations for Upgradable Smart Contracts
Security is always a top priority in blockchain development. With upgradable contracts, there are added risks, as improper upgrades or vulnerabilities in the implementation can expose the system. Here are some best practices:
- Access Control: Ensure only trusted accounts (like the contract owner or a multi-signature wallet) can initiate upgrades.
- Pausable Contracts: Use a “pause” function to halt activity in emergencies. This gives time to patch vulnerabilities if something goes wrong with an upgrade.
- Testing on Testnet: Thoroughly test upgrades on a test network (like Rinkeby) to identify any issues before deployment.
By following these practices, you reduce the risk of attacks and keep your smart contract security robust.
Gas Optimization Techniques
Gas optimization is crucial for any Ethereum contract, especially upgradable ones that may have added complexity. Here are some optimization tips:
- Efficient Storage Usage: Minimize storage variables in the implementation to save on gas fees, as storage costs are high on Ethereum.
- Minimal Proxy Logic: Keep the proxy contract as simple as possible to avoid extra gas costs in forwarding calls.
- Use UUPS for Cost Efficiency: UUPS contracts tend to be more efficient for upgrades, making them ideal for frequently updated applications.
Best Practices for Upgradable Smart Contracts
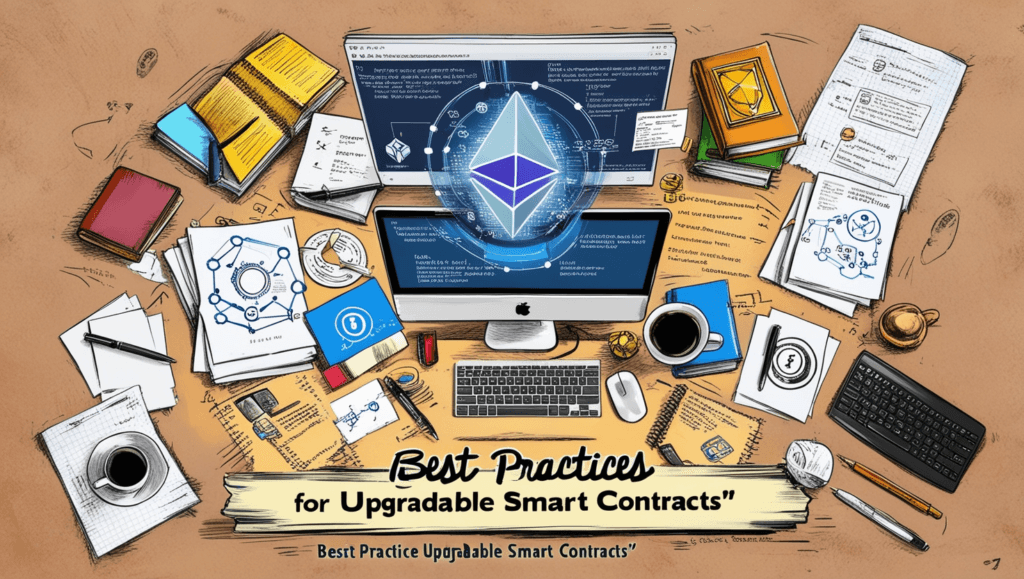
Creating a reliable upgradable contract involves planning for future updates:
- Organize Storage Carefully: Plan storage layout to avoid data conflicts during upgrades. Since
delegatecall
preserves the proxy’s storage, even minor misalignments can lead to issues. - Utilize Libraries: Libraries like OpenZeppelin’s upgradeable contract library provide tested solutions for proxies, making your code more secure.
- Follow Standards: Using standardized practices like OpenZeppelin’s Proxy Patterns helps avoid common pitfalls and saves time.
- Document Everything: Proper documentation ensures that future developers (or even you) can follow the logic of your contract upgrades.
The Future of Upgradable Smart Contracts
As blockchain technology grows, so does the demand for upgradable smart contracts in various sectors. From supply chain management to decentralized finance and NFTs, upgradable contracts allow DApps to stay relevant, adapt to changes, and remain secure.
With Ethereum moving toward scalability and more developers embracing Web3 development, upgradable contracts will likely play a central role. Their flexibility in smart contract security, gas optimization, and ease of maintenance make them essential for projects aiming to scale or adapt over time.
Wrapping Up
Building upgradable smart contracts in Solidity is a game-changer, especially for developers creating advanced DApps on Ethereum. With this guide, you should have a solid understanding of how to structure a proxy-implementation setup, handle secure upgrades, and implement gas-saving techniques.
By following best practices for upgradable smart contracts and taking security considerations seriously, you’ll be well-prepared to build future-proof applications that stand the test of time in the blockchain space.