Frontend Integration with Solidity: When working with blockchain technology, integrating a Solidity smart contract with a frontend interface is one of the most critical steps. It transforms a static blockchain solution into an interactive application, enabling users to seamlessly interact with decentralized applications (dApps). In this tutorial, you’ll learn how to master the art of frontend integration with Solidity.
This guide will cover everything from understanding frontend integration to implementing it with essential tools and frameworks like Web3.js, React.js, and Ethers.js. By the end, you’ll have a solid foundation to build your own fully-functional dApp.
Table of Contents
What is Frontend Integration in Solidity?
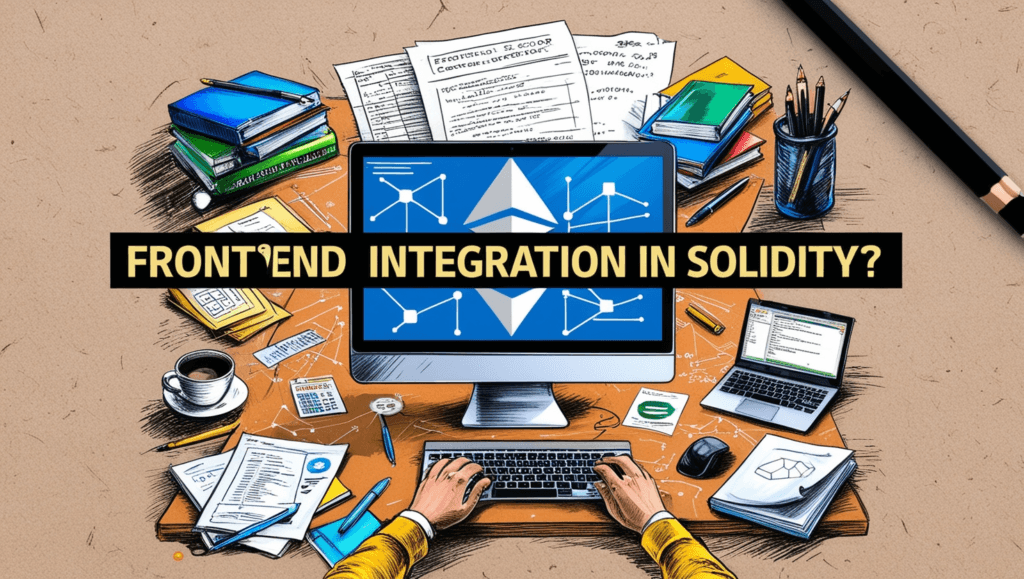
Frontend integration is the process of connecting your Solidity smart contract with a web-based user interface. This connection allows users to interact with the blockchain through intuitive buttons, forms, and visual elements, instead of using complex command-line tools or interacting directly with raw blockchain data.
The key objectives of frontend integration include:
- Enhanced Accessibility: Making blockchain services accessible to non-technical users.
- Streamlined User Experience: Improving how users interact with blockchain applications.
- Real-World Use Cases: Bridging decentralized logic with real-world interactions.
Core Concepts in Frontend Integration
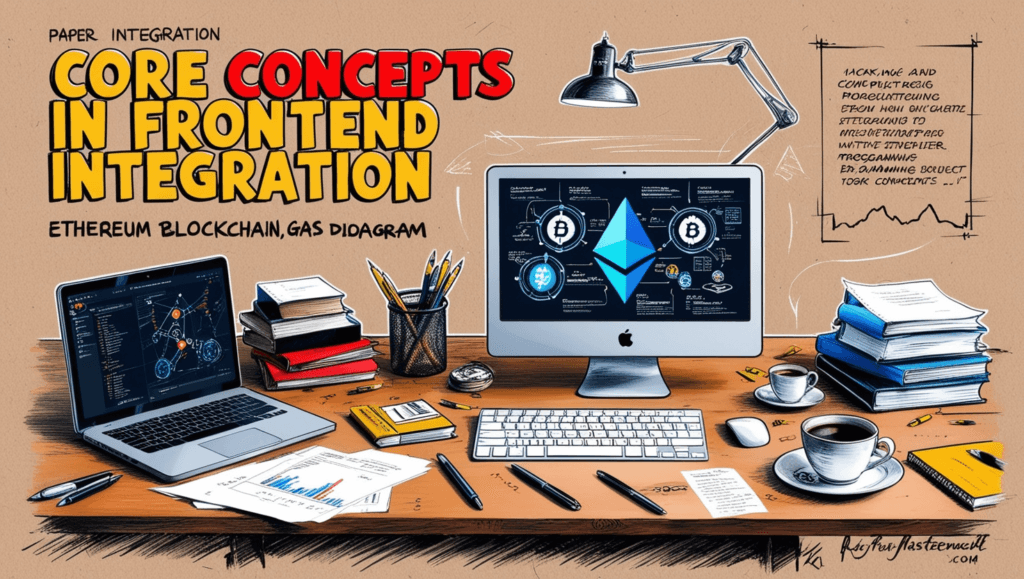
To understand how Solidity integrates with a frontend, you need to grasp several foundational concepts:
1. Smart Contracts
A smart contract is self-executing code stored on the blockchain. It contains logic that defines actions like transferring funds, validating conditions, or storing data.
2. ABI (Application Binary Interface)
The ABI is a JSON representation of your contract’s structure. It serves as a bridge between the blockchain and your application, allowing the frontend to communicate with the smart contract.
3. Web3 Providers
A Web3 provider is a tool that connects the frontend to a blockchain network, enabling interaction with deployed contracts. Popular examples include MetaMask and Infura.
Setting Up Your Development Environment
Before starting frontend integration, ensure your tools and environment are ready.
1. Install Node.js and npm
Node.js and npm are essential for managing packages and building frontend applications. Install them from the official Node.js website.
2. Initialize Your Project
Create a new project directory and initialize npm:
mkdir solidity-frontend
cd solidity-frontend
npm init -y
3. Install Dependencies
Install the required libraries like Web3.js, Ethers.js, and React.js:
npm install web3 ethers react react-dom
Creating a Solidity Smart Contract
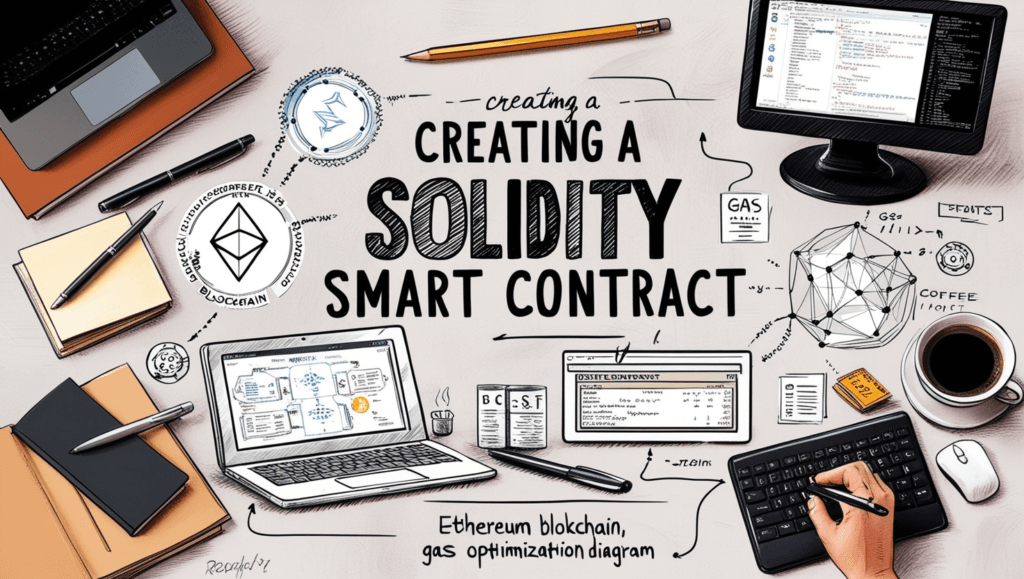
Let’s start with a basic Solidity contract:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract Counter {
uint256 public count;
function increment() public {
count++;
}
function decrement() public {
require(count > 0, "Count cannot be negative");
count--;
}
}
Deploy this contract to a test network like Rinkeby using tools like Remix, Hardhat, or Truffle.
Connecting Your Frontend to Solidity
Step 1: Import Web3.js
Start by importing Web3.js to enable interaction with your smart contract:
import Web3 from 'web3';
const web3 = new Web3(Web3.givenProvider || 'http://localhost:8545');
Step 2: Access the Smart Contract
Using the contract’s ABI and address, set up a connection:
const abi = [/* Contract ABI here */];
const contractAddress = '0xYourContractAddress';
const contract = new web3.eth.Contract(abi, contractAddress);
Step 3: Reading Data
Fetch data from the blockchain:
contract.methods.count().call().then((result) => {
console.log('Count:', result);
});
Step 4: Sending Transactions
Send a transaction to modify the blockchain:
contract.methods.increment().send({ from: userAddress });
Building the Frontend with React.js
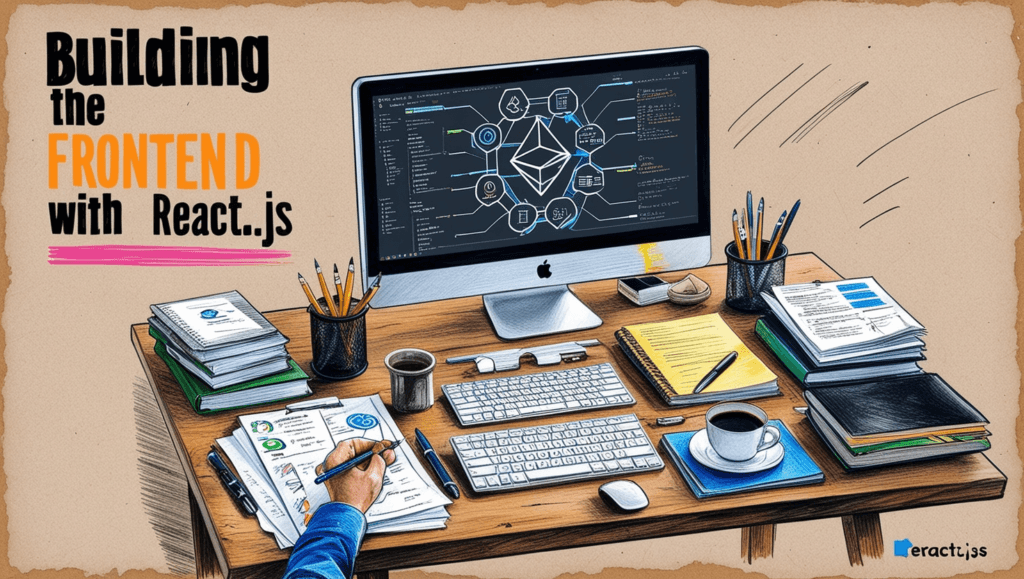
1. Create a React App
Initialize a React app:
npx create-react-app solidity-frontend
cd solidity-frontend
2. Set Up Web3.js in React
Modify the App.js
file to include Web3 setup and contract interaction:
import React, { useState, useEffect } from 'react';
import Web3 from 'web3';
function App() {
const [account, setAccount] = useState('');
const [count, setCount] = useState(0);
useEffect(() => {
const loadBlockchainData = async () => {
const web3 = new Web3(Web3.givenProvider);
const accounts = await web3.eth.requestAccounts();
setAccount(accounts[0]);
const contract = new web3.eth.Contract(abi, contractAddress);
const countValue = await contract.methods.count().call();
setCount(countValue);
};
loadBlockchainData();
}, []);
return (
<div>
<h1>Account: {account}</h1>
<h2>Count: {count}</h2>
</div>
);
}
export default App;
Enhancing Your dApp
1. Adding MetaMask Integration
Encourage users to connect their MetaMask wallet for seamless blockchain access:
const accounts = await window.ethereum.request({ method: 'eth_requestAccounts' });
2. Dynamic Data Updates
Use React hooks like useState
and useEffect
to refresh data dynamically.
3. Error Handling
Implement error-handling mechanisms to improve user experience:
try {
await contract.methods.increment().send({ from: account });
} catch (error) {
console.error('Transaction failed:', error.message);
}
Best Practices for Frontend Integration
- Optimize Smart Contract Calls: Avoid unnecessary calls to minimize gas costs.
- Validate User Inputs: Ensure all user inputs are sanitized to prevent vulnerabilities.
- Responsive Design: Design your UI to be mobile-friendly and accessible.
- Security First: Protect sensitive user data and prevent unauthorized transactions.
Conclusion
Integrating Solidity smart contracts with frontend applications unlocks the true potential of blockchain technology. By following this guide, you can bridge the gap between backend blockchain logic and user-friendly interfaces, empowering users to interact with your dApp effortlessly.
Mastering tools like Web3.js, React.js, and Ethers.js will position you as a skilled blockchain developer capable of delivering powerful decentralized applications. Start small, experiment, and keep building to achieve mastery in Solidity frontend integration.