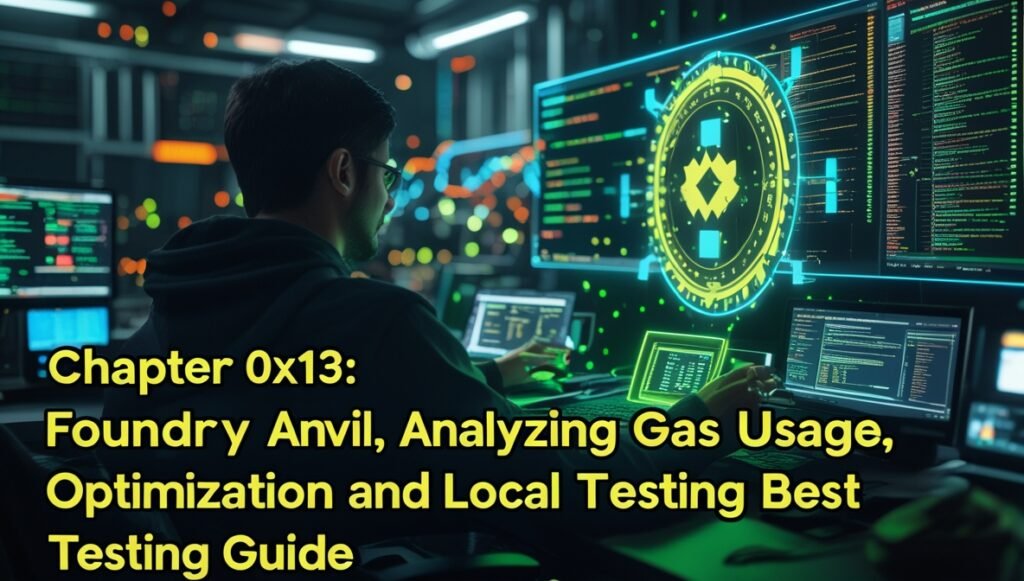
Chapter 0x13: Foundry Anvil, Analyzing Gas Usage, Optimization And Local Testing Best Guide
Foundry Anvil, Analyzing Gas Usage, Optimization & Local Testing : If you’re developing Ethereum smart contracts, understanding gas usage is crucial. The cost of running your contract directly impacts usability and efficiency. Foundry, a powerful toolchain for Ethereum development, provides excellent tools for analyzing and optimizing gas costs while also offering a robust local testing environment with Anvil.
Table of Contents
In this tutorial, we’ll cover:
- Analyzing gas usage with Forge snapshot
- Optimizing gas in smart contracts
- Benchmarks and comparisons with other tools
- Foundry Anvil: Local Ethereum Testing Node
This guide is written for beginners and aims to break down complex concepts into easy-to-understand steps. By the end, you’ll be able to analyze gas usage, optimize smart contracts, and test them locally using Anvil.
🔍 Foundry Analyzing Gas Usage with Forge Snapshot
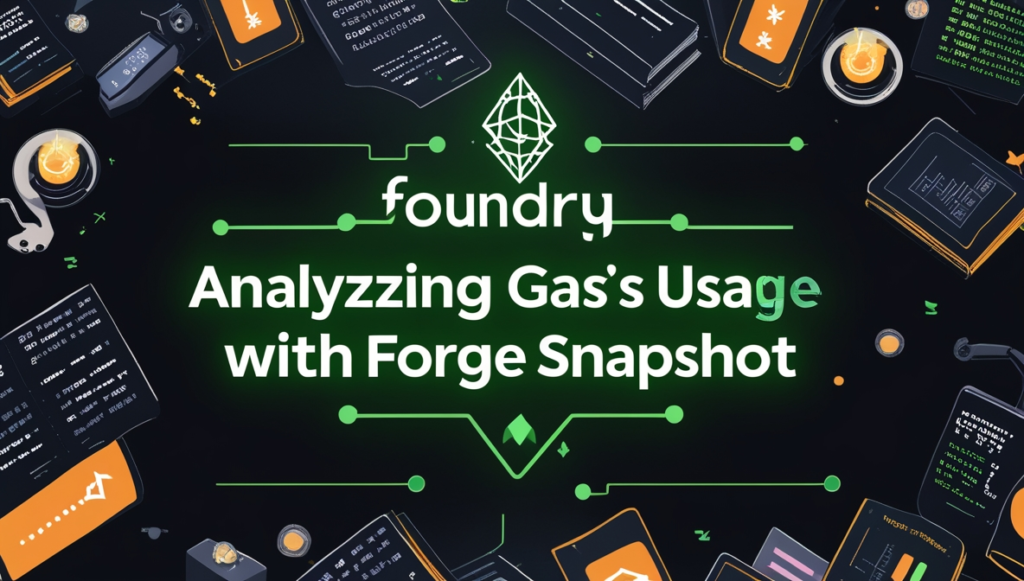
What is a Gas Snapshot?
A gas snapshot helps you track gas consumption in your smart contract functions. Foundry provides the forge snapshot
command to analyze and compare gas costs across different versions of your contract.
Why Use Forge Gas Snapshot?
- Identify high gas-consuming functions
- Compare gas usage before and after optimizations
- Benchmark against other smart contract implementations
How to Generate a Gas Snapshot in Foundry?
To generate a gas snapshot, run:
forge snapshot
This will create a gas-snapshot
file containing detailed reports of function-level gas usage. These insights help you tweak contract logic for better efficiency.
Understanding Foundry Forge Gas Report
The foundry forge gas report provides insights into how much gas each function consumes. A sample report may look like this:
| Function | Min | Max | Avg |
|------------------------|------|------|------|
| deposit() | 2500 | 2700 | 2600 |
| withdraw() | 3000 | 3200 | 3100 |
This helps developers identify expensive operations and areas for optimization.
⚡ Optimizing Gas in Smart Contracts
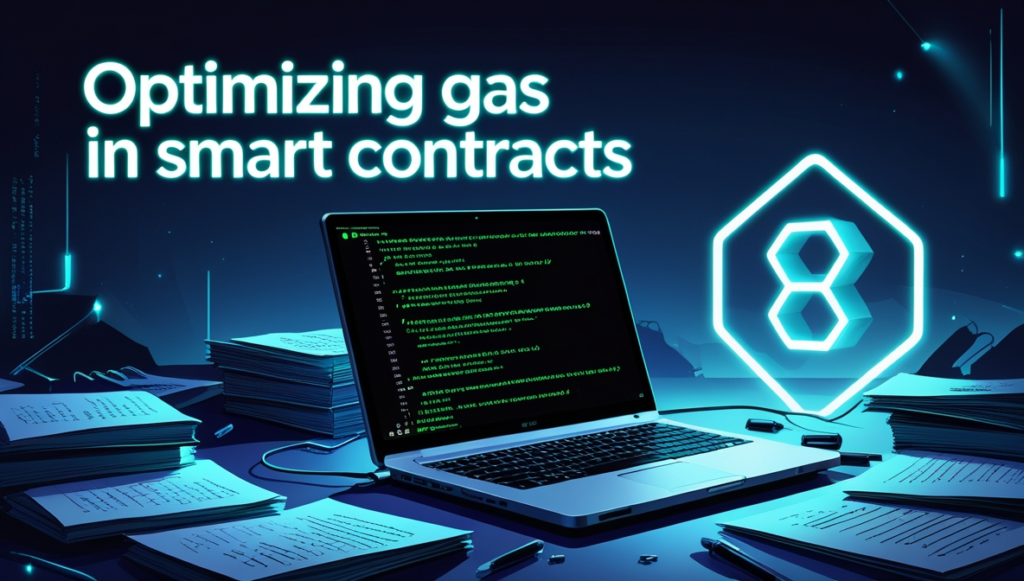
Why Optimize Gas?
Gas fees can be expensive, and high costs can deter users from interacting with your smart contract. Gas-efficient contracts run faster and are cheaper to deploy and use.
Best Practices for Gas Optimization
1️⃣ Use Memory Over Storage
Storage operations are costly. Instead of writing frequently to storage
, use memory
when possible:
function getName() public view returns (string memory) {
string memory name = "Ethereum";
return name;
}
2️⃣ Use Unchecked Math Where Safe
Solidity 0.8+ has automatic overflow checks, but in certain cases, you can skip them using unchecked
:
function add(uint a, uint b) public pure returns (uint) {
unchecked {
return a + b;
}
}
This reduces unnecessary checks, saving gas.
3️⃣ Optimize Loop Usage
Loops can be gas-heavy. Avoid unnecessary iterations and use mapping
instead of arrays where possible.
4️⃣ Reduce External Calls
Each external contract call costs extra gas. Minimize unnecessary calls and use view
or pure
functions whenever possible.
5️⃣ Use Events Instead of Storage
If you need to store logs, consider using events instead of contract storage.
event UserRegistered(address indexed user);
function register() public {
emit UserRegistered(msg.sender);
}
These optimizations help in reducing contract execution costs significantly.
📊 Benchmarks and Comparisons with Other Tools
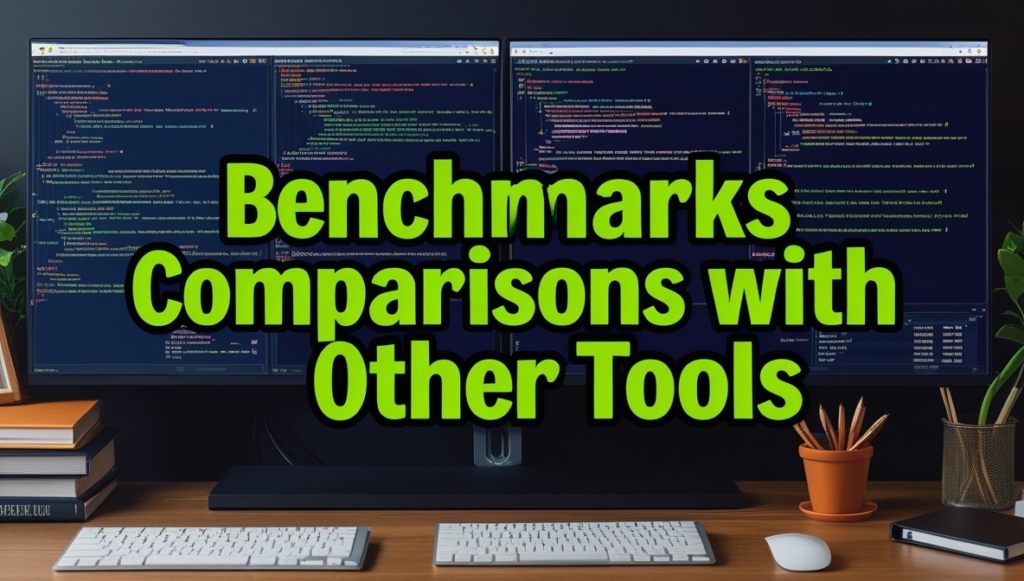
While Foundry is a great tool, it’s important to compare its efficiency with other frameworks like Hardhat and Truffle.
Feature | Foundry | Hardhat | Truffle |
---|---|---|---|
Gas Analysis | ✅ forge snapshot | ❌ No built-in | ❌ No built-in |
Testing Speed | ⚡ Very Fast | 🐢 Slower | 🐢 Slowest |
Built-in Fuzzing | ✅ Yes | ❌ No | ❌ No |
Anvil Local Node | ✅ Yes | ❌ No | ❌ No |
Foundry offers the fastest testing, built-in gas analysis, and better debugging compared to Hardhat and Truffle.
🛠️ Foundry Anvil: Local Ethereum Testing Node
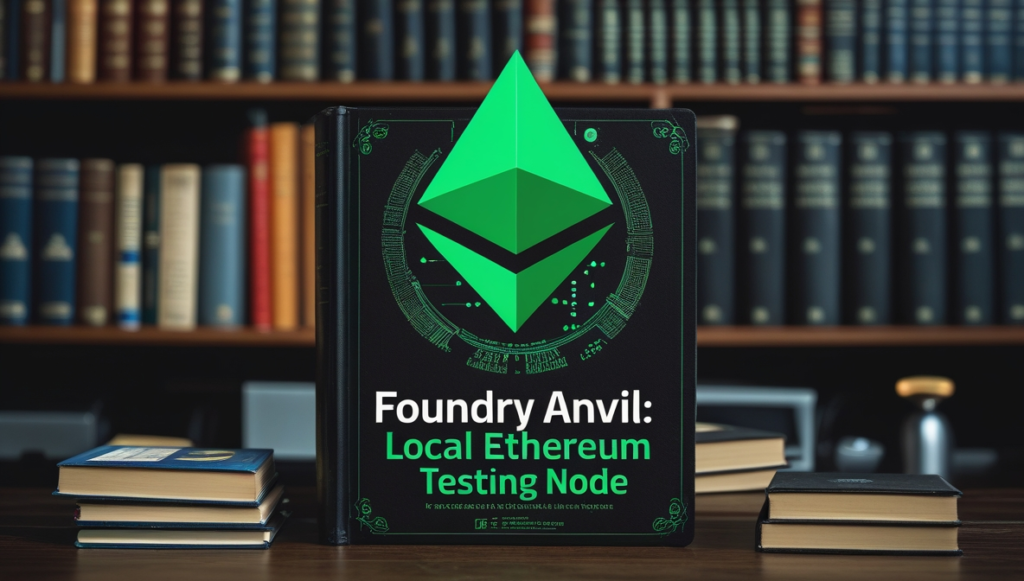
What is Anvil?
Anvil is a local Ethereum testing node included in Foundry. It’s like Hardhat’s node but faster and more efficient.
Why Use Foundry’s Anvil?
- Instant blockchain setup for local testing
- Faster than Hardhat’s local node
- Easy integration with Foundry’s testing framework
How to Use Foundry Anvil?
To start Anvil, run:
anvil
It will spin up a local Ethereum test network. You can deploy and test contracts just like on a real blockchain.
Deploying Smart Contracts to Anvil
Once Anvil is running, deploy a contract using Foundry:
forge create --rpc-url http://127.0.0.1:8545 --private-key YOUR_PRIVATE_KEY ContractName
Foundry Anvil vs Hardhat Node
Feature | Foundry Anvil | Hardhat Node |
---|---|---|
Speed | ⚡ Fastest | 🐢 Slower |
Pre-funded Accounts | ✅ Yes | ✅ Yes |
Gas Reporting | ✅ Yes | ❌ No |
Debugging Tools | ✅ Yes | ❌ No |
Foundry Anvil is ideal for smart contract testing, deployment simulations, and performance benchmarking.
🎯 Conclusion
Foundry is a powerful Ethereum development tool that offers gas analysis, optimization, and fast local testing. Whether you’re an experienced developer or just starting, understanding forge gas snapshots, gas optimization, and Anvil local nodes will significantly improve your smart contract efficiency.
Key Takeaways:
✅ Use forge snapshot
to analyze gas usage
✅ Optimize smart contracts by reducing storage, loops, and external calls
✅ Foundry outperforms Hardhat and Truffle in speed and gas reporting
✅ Anvil is the fastest local Ethereum test node
By following these best practices, you can build efficient, cost-effective, and well-tested smart contracts.
🔗 Additional Resources
Now, go ahead and optimize your smart contracts like a pro! 🚀