Foundry Writing Deployment and Utility Scripts and Security Testing : If you’re stepping into the world of smart contract development, mastering Foundry is a game-changer. Foundry is an advanced Ethereum development framework that helps developers write, test, deploy, and secure smart contracts efficiently. In this chapter, we’ll dive deep into writing deployment and utility scripts, automating tasks, advanced scripting with forge script, and security testing.
Table of Contents
By the end of this tutorial, you’ll know how to:
- Deploy smart contracts using Foundry
- Automate repetitive tasks using custom scripts
- Leverage advanced scripting capabilities in Foundry
- Perform security testing on smart contracts using Foundry
This guide is tailored for beginners, so we’ll break down each concept in a clear and human-friendly manner. Let’s get started!
Foundry Writing Deployment and Utility Scripts
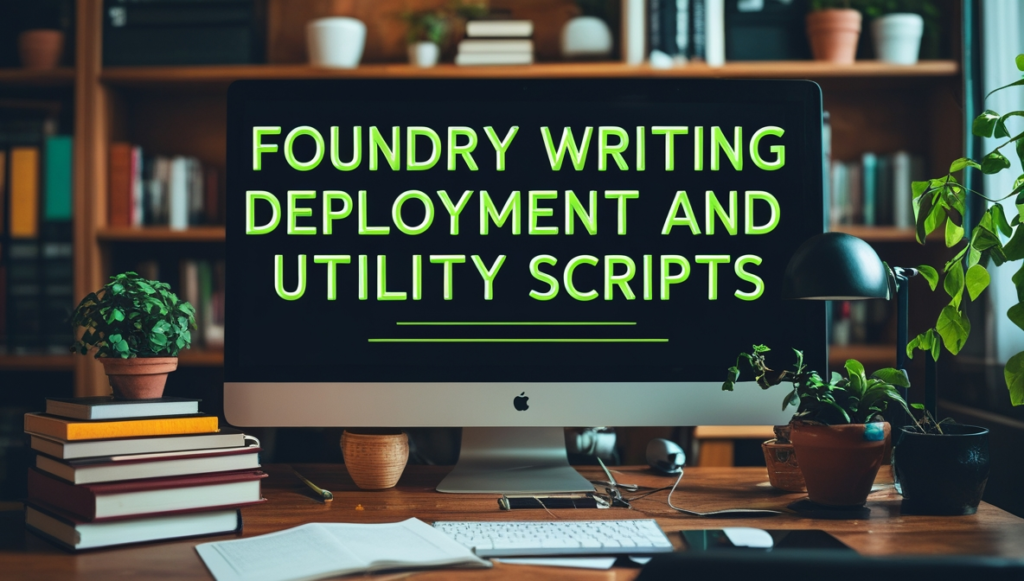
Why Use Foundry for Smart Contract Deployment?
Deploying a smart contract using Foundry is a seamless process. Unlike other frameworks, Foundry offers blazing-fast compilation, a built-in scripting engine, and native support for Solidity and Rust-like syntax.
Key benefits of deploying contracts with Foundry:
- Faster deployment with
forge script
- Better debugging and testing tools
- Lightweight and efficient
- Supports multiple networks
Writing Your First Deployment Script
To deploy a smart contract using Foundry, follow these steps:
1. Install Foundry
curl -L https://foundry.paradigm.xyz | bash
foundryup
2. Initialize a Foundry Project
forge init my-foundry-project
cd my-foundry-project
3. Write a Smart Contract
Create a simple contract inside the src/
folder:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.26;
contract MyContract {
string public message;
constructor(string memory _message) {
message = _message;
}
}
4. Write the Deployment Script
Inside the script/
folder, create Deploy.s.sol
:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.26;
import "forge-std/Script.sol";
import "../src/MyContract.sol";
contract Deploy is Script {
function run() external {
vm.startBroadcast();
new MyContract("Hello, Foundry!");
vm.stopBroadcast();
}
}
5. Deploy the Contract
forge script script/Deploy.s.sol --rpc-url YOUR_RPC_URL --broadcast
Now, your contract is deployed! You can interact with it using cast
or Foundry’s testing tools.
Foundry Automating Repetitive Tasks with Custom Scripts
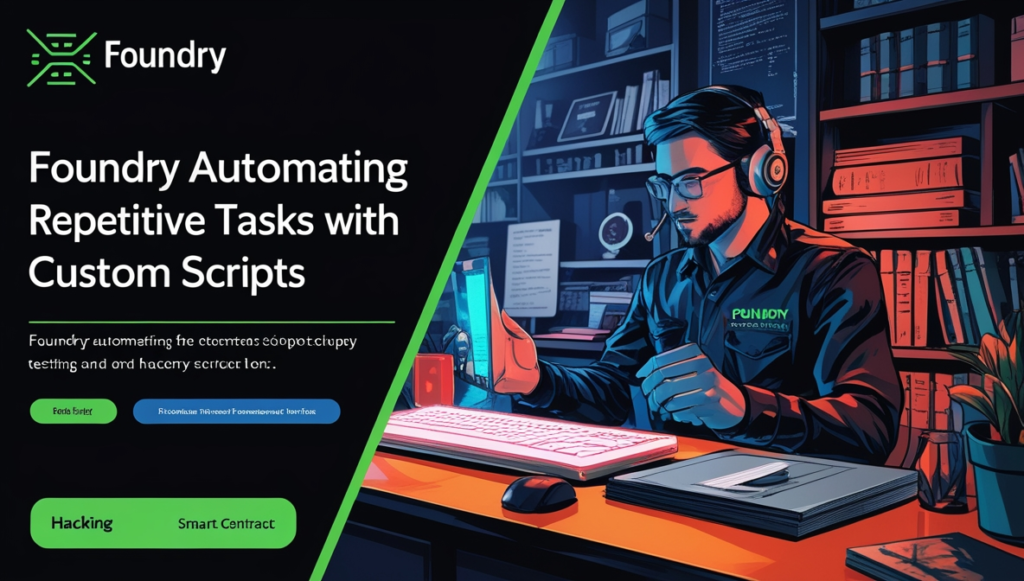
How To Automate Repetitive Tasks Using Custom Scripts in Foundry?
Developers often need to repeat tasks like contract verification, interacting with deployed contracts, or executing batch transactions. Foundry allows automation with Forge scripts.
Example: Automating a Token Transfer
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.26;
import "forge-std/Script.sol";
import "../src/MyContract.sol";
contract TransferTokens is Script {
function run(address _to, uint256 _amount) external {
vm.startBroadcast();
MyContract myContract = MyContract(0xYourContractAddress);
myContract.transfer(_to, _amount);
vm.stopBroadcast();
}
}
Run the script with:
forge script script/TransferTokens.s.sol --rpc-url YOUR_RPC_URL --broadcast
Now you can execute bulk transfers, approvals, and other on-chain actions automatically!
Foundry Advanced Scripting with Forge Script
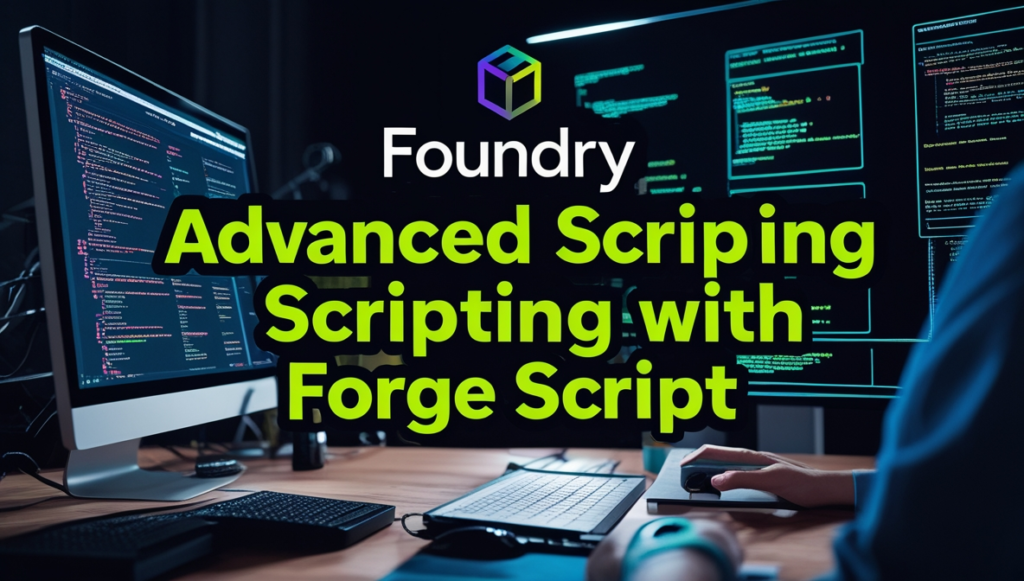
What is Forge Script?
forge script
is a powerful tool in Foundry that allows you to write complex automation tasks, such as contract interactions, testing, and debugging.
Advanced Foundry – Creating a Deployment Script
Example of an advanced deploy script:
contract DeployAdvanced is Script {
function run() external {
vm.startBroadcast();
address deployedAddress = address(new MyContract("Advanced Deployment"));
console.log("Contract deployed at:", deployedAddress);
vm.stopBroadcast();
}
}
Run with:
forge script script/DeployAdvanced.s.sol --rpc-url YOUR_RPC_URL --broadcast
Foundry Smart Contract Security Testing
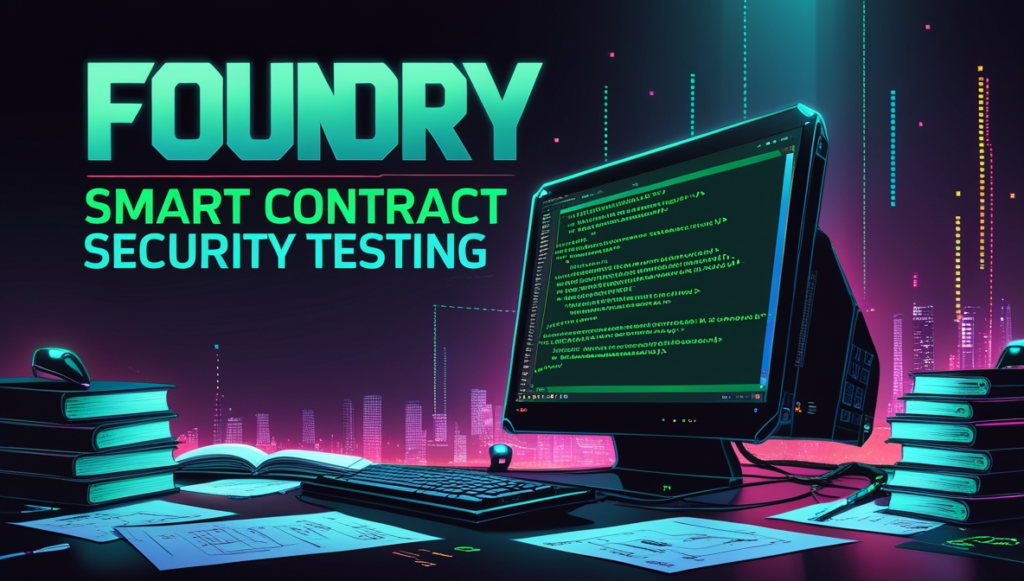
Why Security Testing is Important
Smart contracts are immutable, meaning bugs and vulnerabilities can’t be fixed after deployment. Security testing helps detect:
- Reentrancy attacks
- Integer overflows and underflows
- Access control vulnerabilities
Foundry Smart Contract Security Testing
Foundry has built-in tools for fuzz testing and invariant testing, making it a great choice for secure development.
Example: Fuzz Testing a Contract
contract MyTest is Test {
MyContract myContract;
function setUp() public {
myContract = new MyContract("Hello, Security!");
}
function testFuzzing(string memory _message) public {
myContract.setMessage(_message);
assertEq(myContract.message(), _message);
}
}
Run with:
forge test --fuzz-runs 1000
Foundry Invariant Testing
Invariant testing ensures certain conditions always hold true, no matter the inputs.
Example:
function testInvariant() public {
assert(myContract.getBalance() >= 0);
}
Run:
forge test --runs 10000
Conclusion
Mastering Foundry deployment and utility scripts, automating tasks, leveraging advanced scripting, and implementing security testing will make you a pro Ethereum developer. Here’s a recap of what we covered:
✅ Deploying smart contracts using Foundry
✅ Automating repetitive tasks with custom scripts
✅ Advanced scripting techniques
✅ Security testing with fuzzing and invariants
By integrating these skills, you can build secure, efficient, and well-tested smart contracts!
🚀 Next Steps
- Experiment with Foundry scripting on different networks
- Explore more advanced deployment strategies
- Improve security practices using fuzz and invariant testing
Stay tuned for more in-depth tutorials!
Happy Coding! 🎯