Inline assembly in Solidity is a powerful tool that allows developers to write low-level code for Ethereum smart contracts, giving them greater control over how their code interacts with the Ethereum Virtual Machine (EVM). By integrating Yul, a low-level language designed to work seamlessly with Solidity, developers can unlock efficiencies and implement custom logic not easily achievable with Solidity alone.
Table of Contents
A study of inline assembly in Solidity smart contracts dives into the power of using low-level code to optimize and enhance smart contract performance. By integrating inline assembly, developers can directly manipulate the Ethereum Virtual Machine (EVM), allowing for more efficient execution and cost savings. This technique is perfect for situations where Solidity’s high-level functions may not be efficient enough. While it offers more control, it also requires a deeper understanding of the EVM and can be trickier to debug. Overall, inline assembly in Solidity gives developers an advanced tool to create faster and more efficient contracts.
In this article, we’ll dive into the key aspects of inline assembly in Solidity, including:
- Integrating Yul with Solidity using assembly
- Inline assembly syntax and conventions
- Writing and testing custom assembly blocks
- Examples of practical applications
- Writing functions in Yul
Let’s get started!
What is Inline Assembly in Solidity?
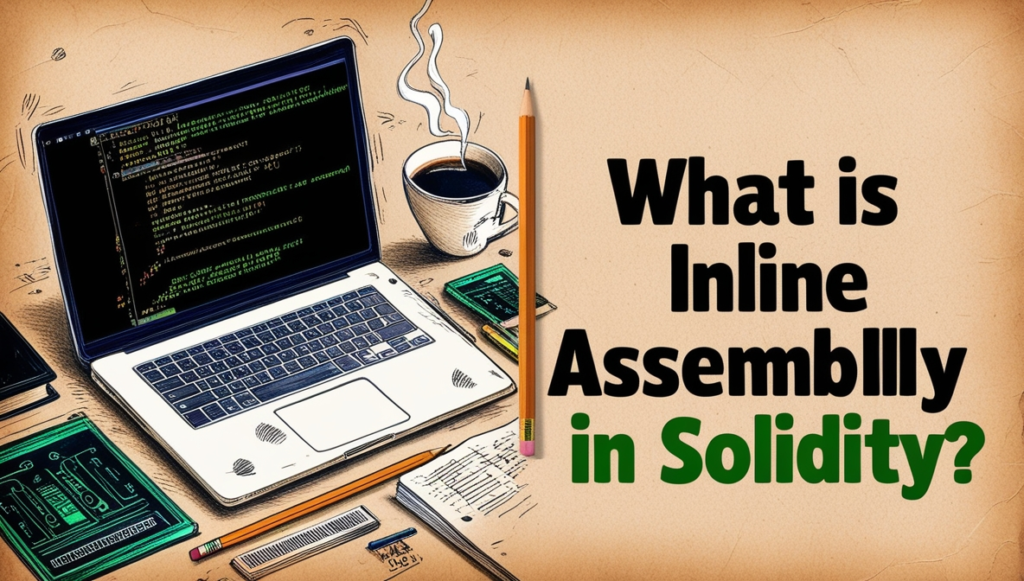
Inline assembly allows developers to embed EVM bytecode directly within Solidity using the assembly
keyword. This approach can be useful when you need to:
- Optimize gas usage.
- Perform operations that are not supported by Solidity natively.
- Gain deeper control over EVM instructions.
Using inline assembly, developers can integrate Yul, a low-level language that provides a more readable syntax compared to raw bytecode. Yul is particularly useful for writing assembly-like code in a structured and developer-friendly way. For those interested in diving deeper, resources like Yul documentation and Solidity assembly tutorials can be invaluable.
Integrating Yul with Solidity Using Assembly
Yul is the intermediate language used by Solidity’s compiler to generate EVM bytecode. It can be used directly within Solidity’s assembly blocks to create highly optimized smart contract code.
Yul and Solidity are complementary; developers often use Yul for specific optimizations that are hard to achieve in higher-level Solidity code. By embedding Yul code in assembly
blocks, you can fine-tune performance-critical parts of your contract.
Here’s a simple example of integrating Yul with Solidity:
pragma solidity ^0.8.26;
contract YulExample {
function add(uint256 a, uint256 b) public pure returns (uint256) {
assembly {
let result := add(a, b)
mstore(0x0, result)
return(0x0, 32)
}
}
}
In this example:
let
: Used to declare variables within Yul.add
: A Yul opcode for addition.mstore
: Stores data in memory.return
: Returns data from memory to the caller.
Inline Assembly Syntax and Conventions
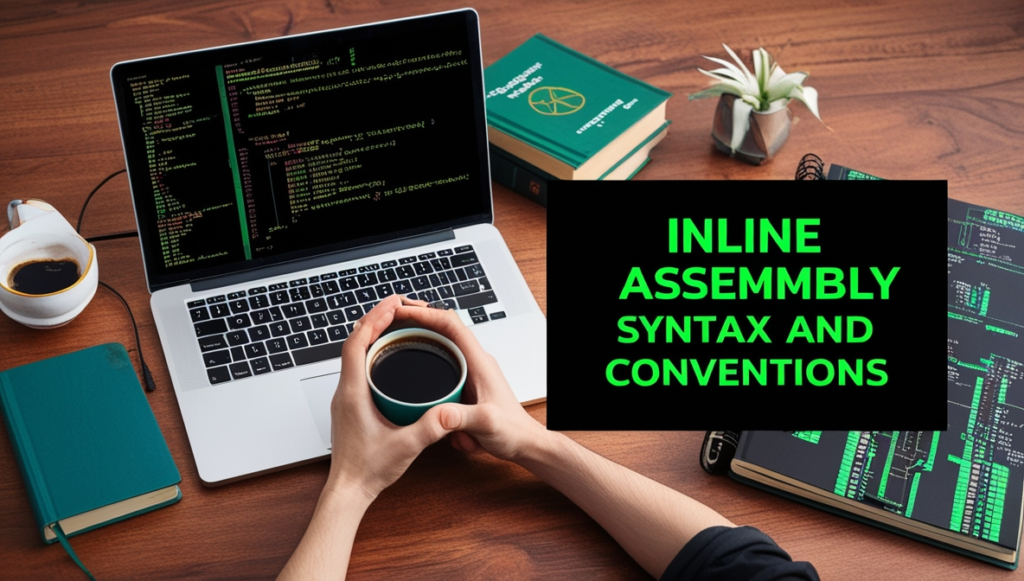
The syntax of inline assembly in Solidity closely mirrors the EVM’s instruction set, but it’s designed to be easier to read and use.
Basic Syntax
Inline assembly code is enclosed within the assembly
block. Here’s a basic example:
assembly {
// Your assembly code here
}
Common Opcodes
mstore
andmload
: Used to store and load data in memory.mstore(position, value)
: Writesvalue
to memory atposition
.mload(position)
: Reads the value from memory atposition
.
sstore
andsload
: Used to store and load data in storage.sstore(slot, value)
: Writesvalue
to storage atslot
.sload(slot)
: Reads the value from storage atslot
.
- Arithmetic Opcodes: Operations like
add
,sub
,mul
,div
. - Control Flow:
jump(label)
andjumpi(label, condition)
: Unconditional and conditional jumps.stop
: Halts execution.
Conventions
- Always initialize variables before using them.
- Use descriptive labels for jumps to improve readability.
- Optimize gas usage by minimizing memory and storage operations.
Writing and Testing Custom Assembly Blocks
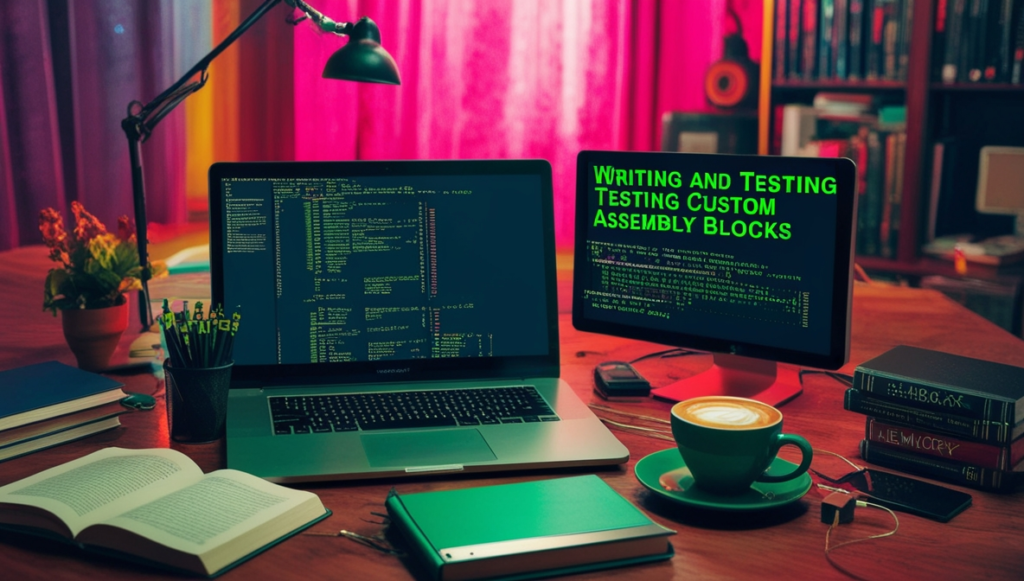
Writing custom assembly blocks involves:
- Identifying Use Cases:
- Optimize computationally intensive tasks.
- Access low-level EVM features.
- Using Inline Assembly:
Here’s an example of calculating the power of a number:
pragma solidity ^0.8.26;
contract PowerExample {
function power(uint256 base, uint256 exponent) public pure returns (uint256) {
assembly {
let result := 1
for { let i := 0 } lt(i, exponent) { i := add(i, 1) } {
result := mul(result, base)
}
mstore(0x0, result)
return(0x0, 32)
}
}
}
- Testing:
- Use unit testing frameworks like Hardhat or Foundry.
- Write edge case tests to ensure correctness.
- Benchmark performance improvements compared to pure Solidity implementations.
Examples of Practical Applications
1. Gas Optimization
Inline assembly can help reduce gas costs for frequently called functions. For instance:
pragma solidity ^0.8.26;
contract GasOptimization {
function multiply(uint256 a, uint256 b) public pure returns (uint256) {
assembly {
let product := mul(a, b)
mstore(0x0, product)
return(0x0, 32)
}
}
}
By avoiding higher-level abstractions, this implementation minimizes gas usage.
2. Custom Cryptographic Functions
Many cryptographic operations are not natively supported by Solidity. Inline assembly enables developers to implement such operations efficiently, providing greater control over the computation process.
3. Advanced Memory Management
By using mstore
and mload
, developers can efficiently manage memory and reduce overhead. This is especially useful in applications requiring extensive data manipulation.
4. Interacting with EVM Internals
Assembly blocks can be used to access calldata directly, bypassing Solidity’s abstraction layer. This allows for fine-tuned control over how inputs are handled. For advanced developers, exploring topics like Solidity assembly opcodes or Solidity assembly calldataload can provide significant insights.
Writing Functions in Yul Assembly in Solidity
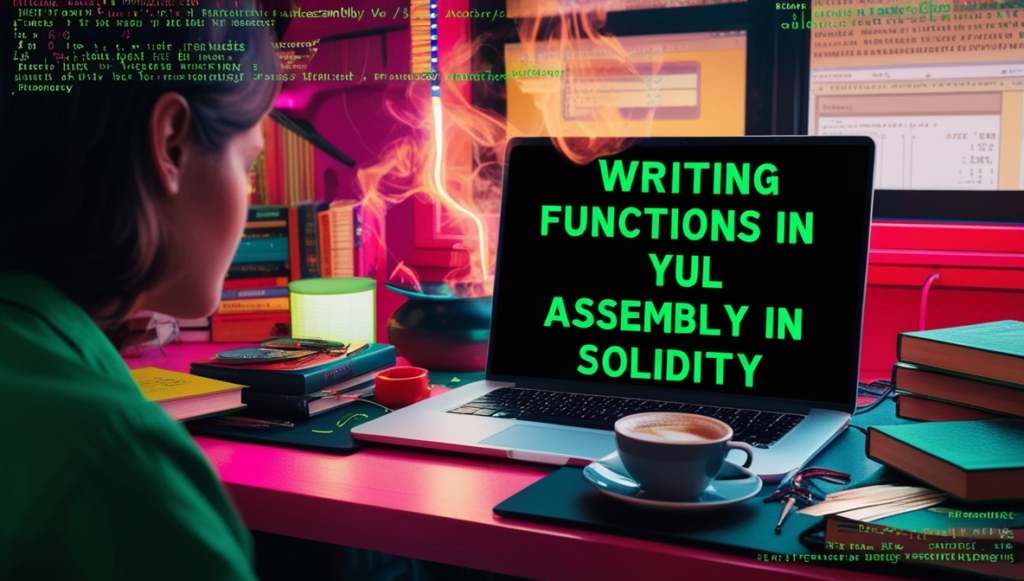
Yul allows developers to write reusable functions within assembly blocks. These functions are helpful for breaking down complex operations into manageable pieces.
Function Syntax in Yul
assembly {
function myFunction(a, b) -> result {
result := add(a, b)
}
}
Example: Factorial Calculation
Here’s an example of a reusable factorial function written in Yul:
pragma solidity ^0.8.26;
contract Factorial {
function calculateFactorial(uint256 n) public pure returns (uint256) {
assembly {
function factorial(num) -> result {
result := 1
for { let i := 1 } le(i, num) { i := add(i, 1) } {
result := mul(result, i)
}
}
let output := factorial(n)
mstore(0x0, output)
return(0x0, 32)
}
}
}
This example demonstrates the modularity and reusability of Yul functions within Solidity contracts. For additional resources, consult Yul Solidity examples and Solidity inline assembly tutorials.
a study of inline assembly in solidity smart contracts
Benefits of Inline Assembly in Solidity
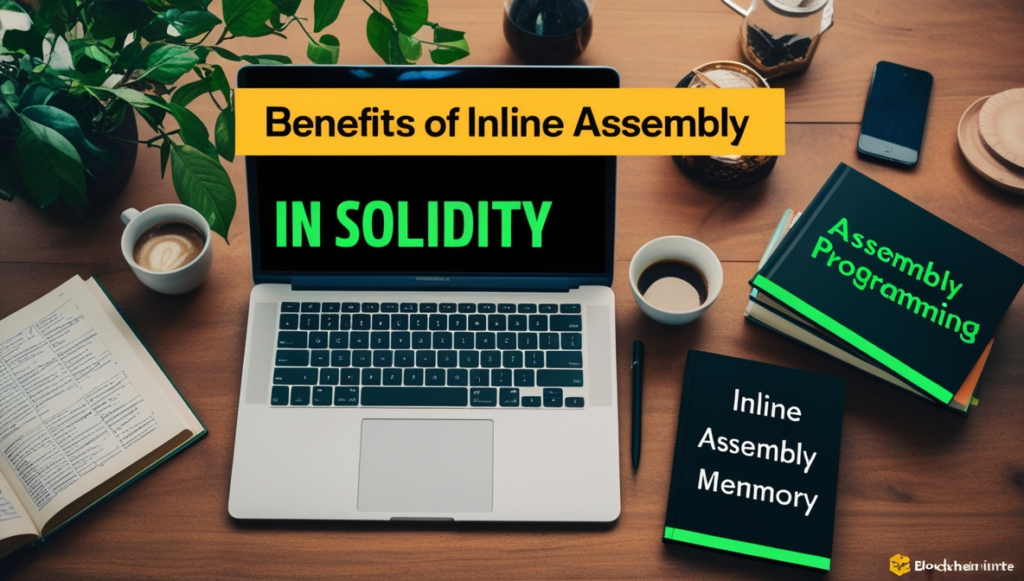
- Gas Efficiency: Write highly optimized code for critical operations.
- Low-Level Access: Interact directly with the EVM, enabling custom behaviors and optimizations.
- Custom Logic: Implement functionality not natively supported by Solidity, enhancing the contract’s capabilities.
Best Practices for Inline Assembly
- Use Assembly Only When Necessary: Avoid using assembly for simple tasks that Solidity can handle efficiently.
- Thorough Testing: Inline assembly is error-prone, so rigorous testing is essential.
- Readable Code: Use comments and descriptive labels to make your assembly code understandable.
- Security First: Be cautious of vulnerabilities like reentrancy and ensure your assembly code is secure.
- Follow Documentation: Refer to the official Yul documentation and Solidity assembly docs to avoid common pitfalls.
Conclusion
Inline assembly in Solidity, combined with Yul, provides developers with powerful tools to optimize and enhance their smart contracts. By understanding the syntax, conventions, and practical applications of assembly, you can unlock new possibilities in Ethereum development. Remember to write clear, well-documented code and test thoroughly to ensure reliability.
Whether you’re looking to optimize gas costs, access low-level EVM features, or write custom logic, inline assembly is a valuable skill for any Ethereum developer. Start experimenting with small assembly blocks and gradually build up your expertise to tackle more complex challenges!