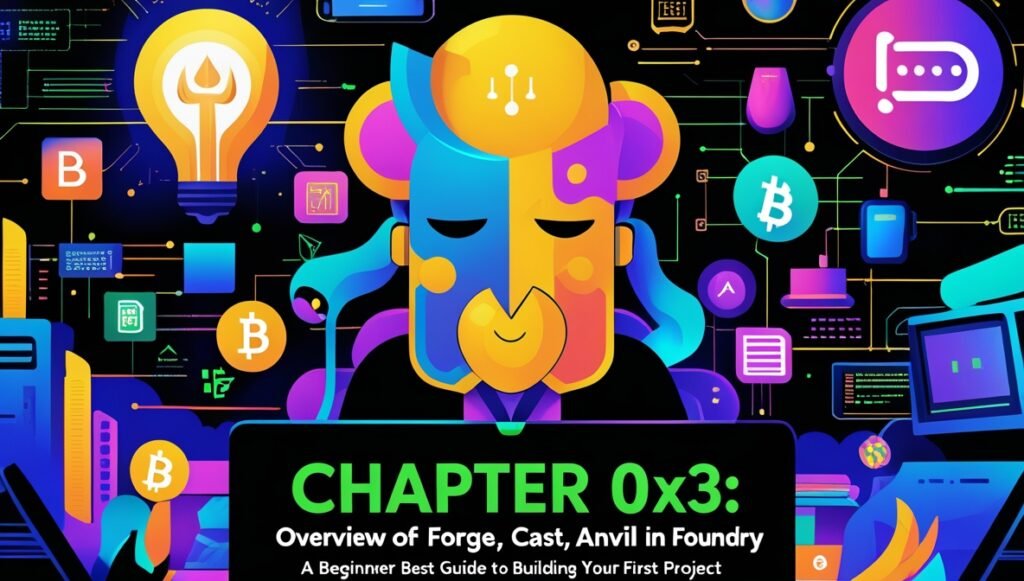
Chapter 0x3: Overview of Forge, Cast, and Anvil in Foundry – A Beginner’s Best Guide to Building Your First Project
Overview of Forge, Cast, and Anvil in Foundry : If you’re diving into the world of blockchain development, you’ve probably heard of Foundry, a powerful toolkit for Ethereum development. Foundry is a developer’s best friend when it comes to writing, testing, and deploying smart contracts. But what exactly are Forge, Cast, and Anvil? How do they fit into the Foundry ecosystem? And how can you use them to create your first project?
In this tutorial, we’ll break down everything you need to know about Foundry, its core components, and how to get started with your first project. Whether you’re a beginner or just looking to brush up on your skills, this guide will walk you through the basics in a casual, human-friendly tone. Let’s get started!
Table of Contents
What is Foundry?
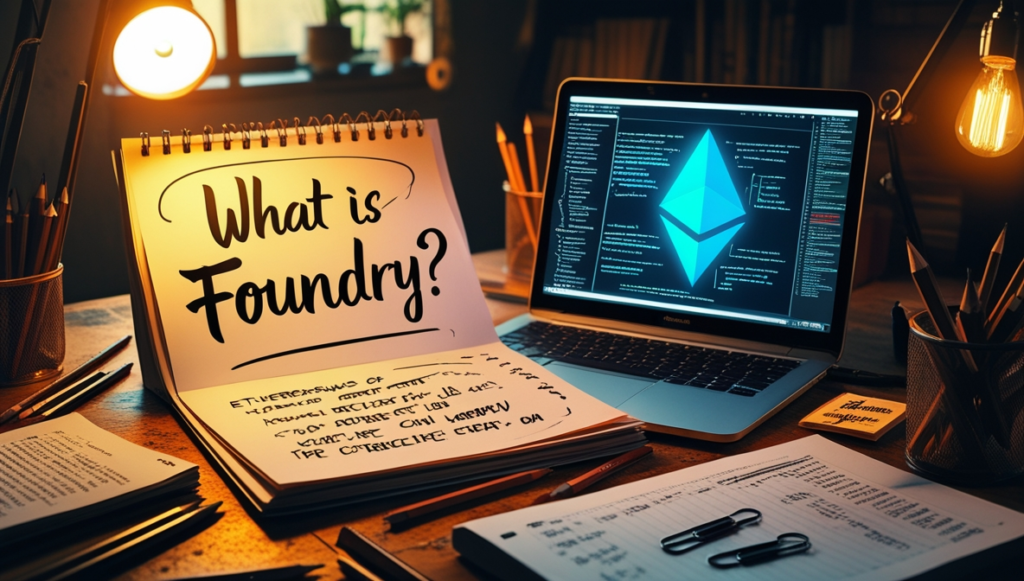
Foundry is a fast, portable, and modular toolkit for Ethereum application development. It’s designed to make your life as a developer easier by providing tools for writing, testing, and deploying smart contracts. Foundry is written in Rust, which makes it incredibly fast and efficient compared to other Ethereum development frameworks.
The three main components of Foundry are:
- Forge: A smart contract testing framework.
- Cast: A command-line tool for interacting with Ethereum networks.
- Anvil: A local Ethereum node for testing and development.
Together, these tools form the backbone of Foundry, enabling developers to build and deploy smart contracts with ease.
What is Forge, Cast, and Anvil in Foundry?
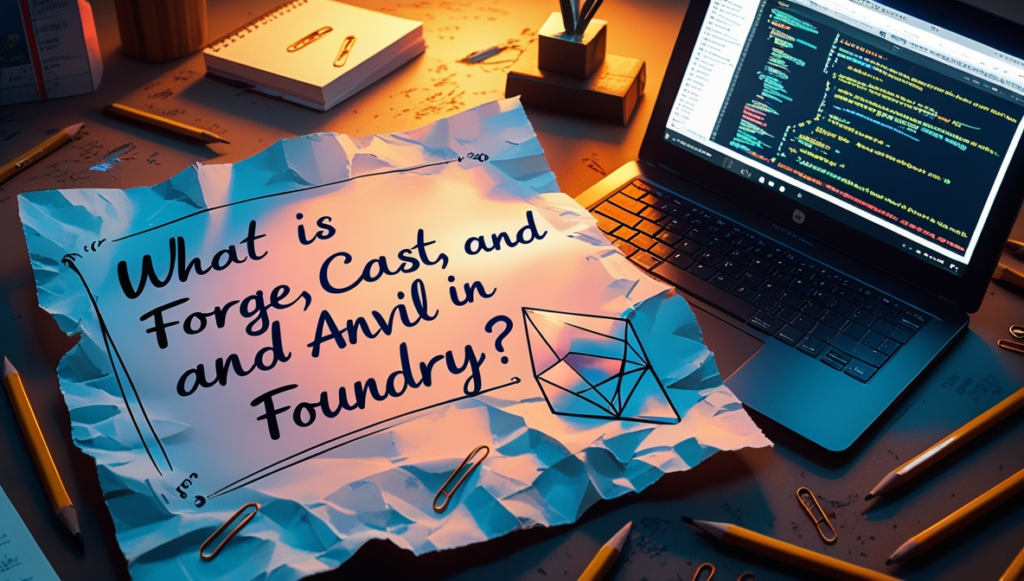
Overview of Forge : What is Forge in Foundry?
Forge is Foundry’s smart contract testing framework. It allows you to write and run tests for your Solidity smart contracts. Forge is incredibly fast and supports features like fuzz testing (randomized testing) and invariant testing (testing for specific conditions).
With Forge, you can:
- Compile your smart contracts.
- Run tests to ensure your contracts work as expected.
- Debug issues with detailed error messages.
Forge is the go-to tool for developers who want to ensure their smart contracts are robust and secure before deploying them to the blockchain.
What is Cast In Foundry?
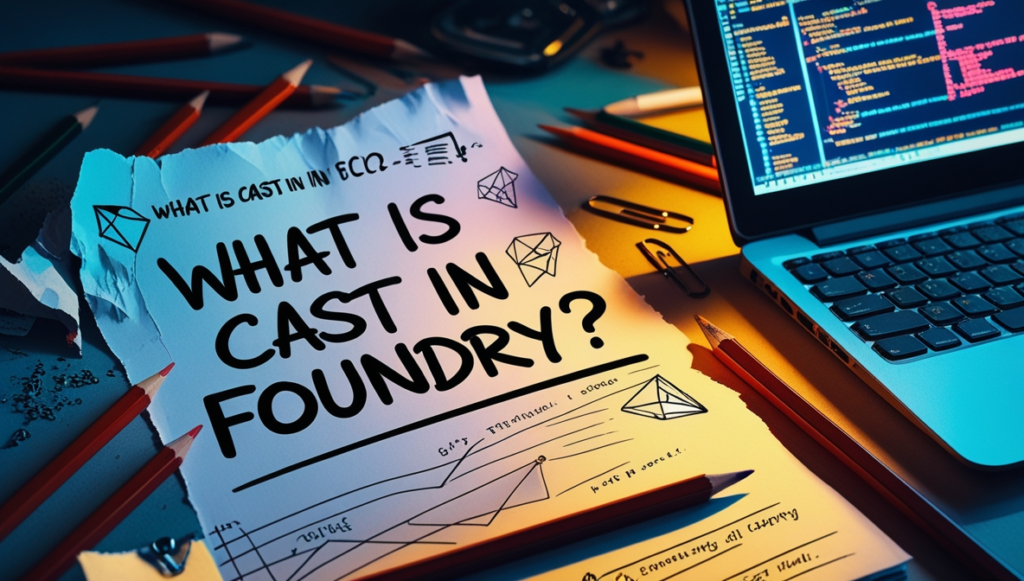
Cast is Foundry’s command-line tool for interacting with Ethereum networks. Think of it as your Swiss Army knife for blockchain interactions. With Cast, you can:
- Send transactions.
- Query blockchain data.
- Call smart contract functions.
- Decode transaction data.
Cast is particularly useful for developers who want to interact with smart contracts directly from the command line without needing a full-fledged frontend.
What is Anvil in Foundry?
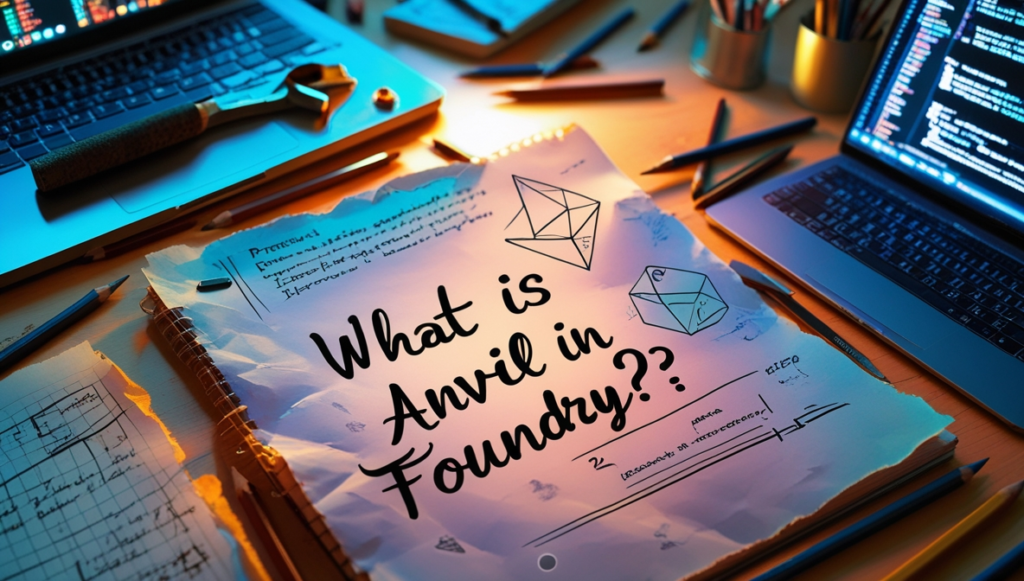
Anvil is Foundry’s local Ethereum node. It’s a lightweight, fast, and customizable node that you can use for testing and development. Anvil allows you to:
- Deploy smart contracts locally.
- Simulate Ethereum transactions.
- Test your contracts in a controlled environment.
Anvil is perfect for developers who want to test their smart contracts without spending real ETH on gas fees.
Foundry Forge, Cast, and Anvil: How Do They Work Together?
Imagine you’re building a smart contract. Here’s how Foundry’s tools come into play:
- Forge: You write and test your smart contract using Forge. It compiles your Solidity code and runs tests to ensure everything works as expected.
- Anvil: You deploy your contract to a local Ethereum node using Anvil. This allows you to test your contract in a simulated environment.
- Cast: Once your contract is deployed, you use Cast to interact with it. You can call functions, send transactions, and query data directly from the command line.
Together, these tools provide a seamless workflow for Ethereum development.
Commonly Used Commands in Foundry
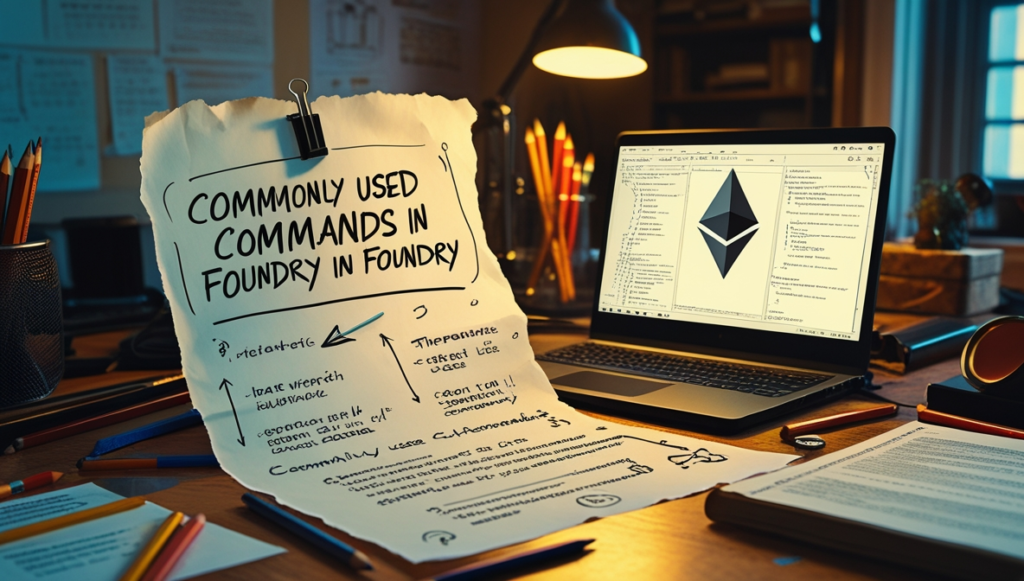
Now that you understand the basics, let’s dive into some of the most commonly used commands in Foundry.
1. forge init
The forge init
command is used to create a new Foundry project. It sets up the basic directory structure and configuration files for your project.
forge init my_project
This command will create a new directory called my_project
with the following structure:
src/
: Contains your Solidity smart contracts.test/
: Contains your test files.lib/
: Contains external dependencies.foundry.toml
: Configuration file for Foundry.
2. forge build
The forge build
command compiles your Solidity smart contracts. It reads the contracts from the src/
directory and compiles them into bytecode.
forge build
After running this command, you’ll see a new out/
directory containing the compiled contracts.
3. forge test
The forge test
command runs the tests for your smart contracts. It reads the test files from the test/
directory and executes them.
forge test
Forge provides detailed output, including information about passed and failed tests, gas usage, and more.
4. forge clean
The forge clean
command removes the out/
and cache/
directories, which contain compiled contracts and cached data.
forge clean
This command is useful if you want to start fresh or if you’re encountering issues with your build.
Creating Your First Project in Foundry
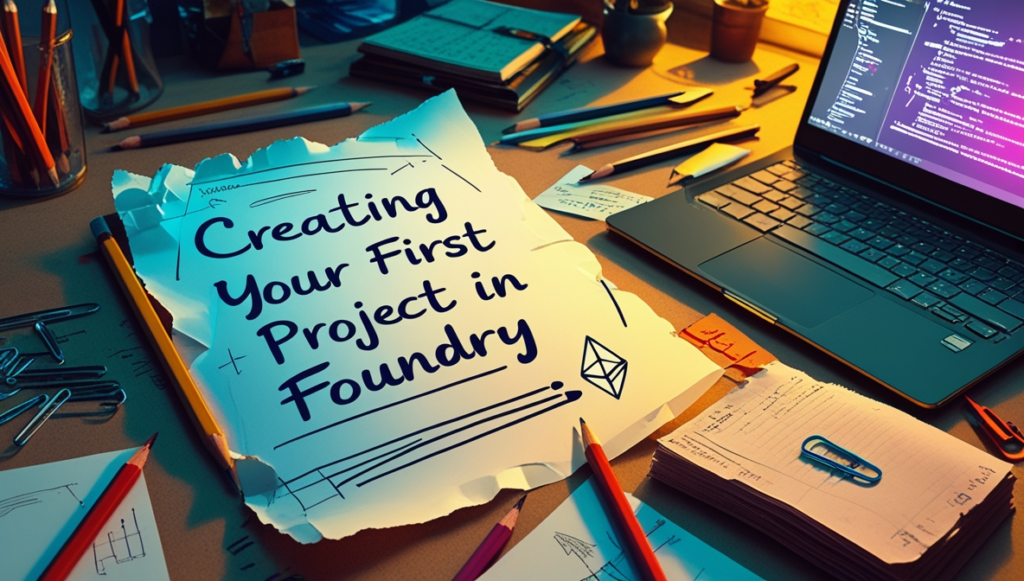
Let’s walk through the process of creating your first Foundry project step by step.
Step 1: Install Foundry
Before you can use Foundry, you’ll need to install it. You can do this by running the following command:
curl -L https://foundry.paradigm.xyz | bash
After the installation is complete, run foundryup
to install the latest versions of Forge, Cast, and Anvil.
Step 2: Initialize a New Project
Once Foundry is installed, you can create a new project using the forge init
command:
forge init my_first_project
This will create a new directory called my_first_project
with the basic structure for a Foundry project.
Step 3: Write Your First Smart Contract
Navigate to the src/
directory and create a new Solidity file called MyContract.sol
. Here’s an example of a simple smart contract:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract MyContract {
uint256 public value;
function setValue(uint256 _value) public {
value = _value;
}
function getValue() public view returns (uint256) {
return value;
}
}
This contract allows you to store and retrieve a value.
Step 4: Compile Your Contract
Use the forge build
command to compile your contract:
forge build
If everything is set up correctly, you should see a success message.
Step 5: Write Tests
Navigate to the test/
directory and create a new test file called MyContract.t.sol
. Here’s an example of a test:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "forge-std/Test.sol";
import "../src/MyContract.sol";
contract MyContractTest is Test {
MyContract myContract;
function setUp() public {
myContract = new MyContract();
}
function testSetValue() public {
myContract.setValue(42);
assertEq(myContract.getValue(), 42);
}
}
This test checks if the setValue
and getValue
functions work as expected.
Step 6: Run Tests
Use the forge test
command to run your tests:
forge test
If everything is working correctly, you should see a success message.
Step 7: Deploy and Interact with Your Contract
You can deploy your contract to a local Ethereum node using Anvil:
anvil
Then, use Forge to deploy your contract:
forge create --rpc-url http://localhost:8545 --private-key <your_private_key> src/MyContract.sol:MyContract
Finally, use Cast to interact with your contract:
cast send <contract_address> "setValue(uint256)" 42 --rpc-url http://localhost:8545 --private-key <your_private_key>
Conclusion
Foundry is a powerful toolkit that simplifies Ethereum development. With Forge, Cast, and Anvil, you have everything you need to write, test, and deploy smart contracts. Whether you’re a beginner or an experienced developer, Foundry’s speed and flexibility make it an excellent choice for blockchain development.
By following this tutorial, you’ve learned the basics of Foundry and created your first project. Now it’s time to explore further and build something amazing!
Happy Hacking!!