Solidity Design Patterns : Every developer encounters common problems when coding. Solidity design patterns provide reusable solutions to these recurring issues. Instead of reinventing the wheel, you can leverage tried-and-tested patterns that make your code more efficient, readable, and secure.
Table of Contents
Key Benefits of Solidity Design Patterns
- Improved Efficiency: Patterns help reduce gas fees and optimize performance.
- Enhanced Security: Following established practices minimizes vulnerabilities.
- Better Readability: Clean code is easier to maintain and audit.
- Reusability: Patterns save time by eliminating the need to start from scratch.
With these benefits in mind, let’s explore some of the most effective smart contract design patterns for Ethereum development.
Top Solidity Design Patterns You Should Know
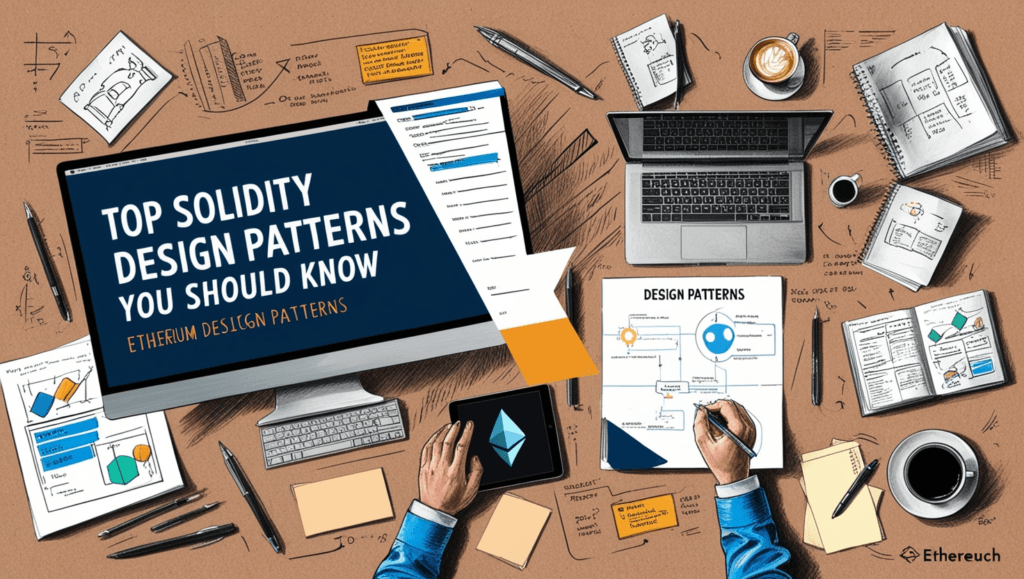
1. Factory Pattern: Creating Multiple Contracts Efficiently
The Factory pattern is a go-to solution for developers who need to deploy multiple instances of a smart contract. This pattern is ideal for applications like decentralized marketplaces, token systems, or NFT collections.
How It Works:
A factory contract is responsible for deploying and managing other contracts dynamically. This approach ensures scalability and allows for better organization of multiple contract instances.
Example:
pragma solidity ^0.8.0;
contract Product {
string public name;
constructor(string memory _name) {
name = _name;
}
}
contract Factory {
address[] public deployedProducts;
function createProduct(string memory _name) public {
Product newProduct = new Product(_name);
deployedProducts.push(address(newProduct));
}
}
Why Use It?
- Centralizes contract creation.
- Simplifies contract management.
2. Proxy Pattern: Building Upgradeable Smart Contracts
One of the biggest challenges in blockchain is immutability. But what if your smart contract needs upgrades? The Proxy pattern solves this by separating storage and logic, enabling seamless contract updates.
Transparent Proxy and UUPS
There are different proxy implementations, like Transparent Proxy and UUPS (Universal Upgradeable Proxy Standard). Both allow you to modify contract logic while preserving data storage.
Example:
pragma solidity ^0.8.0;
contract Proxy {
address public implementation;
function upgrade(address _newImplementation) public {
implementation = _newImplementation;
}
fallback() external payable {
(bool success, ) = implementation.delegatecall(msg.data);
require(success, "Delegatecall failed");
}
}
Advantages:
- Future-proof your contracts.
- Maintain backward compatibility.
3. Pull Over Push Payments: Securing Ether Transfers
Directly sending Ether in a smart contract can expose it to risks like reentrancy attacks. The Pull pattern addresses this by letting users withdraw their funds instead of receiving automatic payments.
Example Implementation:
pragma solidity ^0.8.0;
contract PullPayment {
mapping(address => uint256) public balances;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw() public {
uint256 amount = balances[msg.sender];
require(amount > 0, "No balance available");
balances[msg.sender] = 0;
payable(msg.sender).transfer(amount);
}
}
Why It’s Better:
- Reduces vulnerability to hacking.
- Increases transparency in payments.
4. Access Control: Managing Permissions
Permission management is critical in smart contract development. The Access Control pattern allows you to define roles, such as owner or admin, who can execute specific functions.
Example:
pragma solidity ^0.8.0;
contract Ownable {
address public owner;
modifier onlyOwner() {
require(msg.sender == owner, "Not the owner");
_;
}
constructor() {
owner = msg.sender;
}
function transferOwnership(address newOwner) public onlyOwner {
owner = newOwner;
}
}
Benefits:
- Restricts unauthorized access.
- Streamlines role management.
5. Circuit Breaker: Emergency Safety Mechanism
The Circuit Breaker pattern is like an emergency stop button for your smart contract. It’s used to pause or halt contract functionality during critical events, such as a detected bug or exploit.
Example:
pragma solidity ^0.8.0;
contract CircuitBreaker {
bool public stopped = false;
modifier stopInEmergency() {
require(!stopped, "Contract is stopped");
_;
}
function toggleContractActive() public {
stopped = !stopped;
}
function execute() public stopInEmergency {
// Function logic here
}
}
Advantages:
- Protects users during vulnerabilities.
- Provides time for fixes without losing funds.
How to Choose the Right Solidity Design Pattern
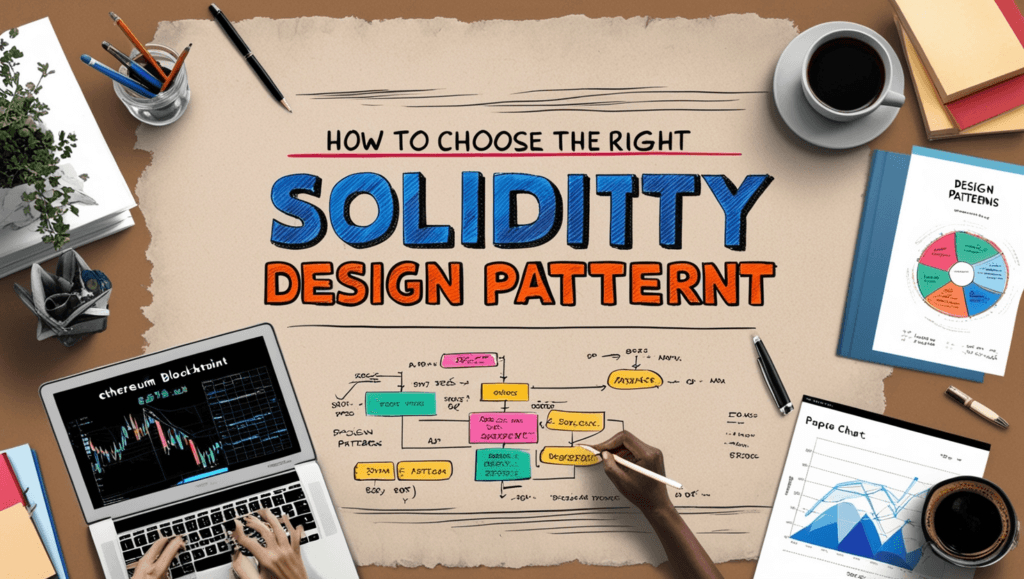
Choosing the right Solidity patterns depends on your project’s needs. Here’s a quick guide:
- Scalability: Use the Factory pattern for dynamic contract creation.
- Upgradability: The Proxy pattern is your best friend.
- Security: Pull payments and Circuit Breakers help mitigate risks.
- Access Management: Implement the Access Control pattern.
Best Practices for Smart Contract Development
To get the most out of Solidity design patterns, follow these best practices:
- Stick to Standards: Use trusted libraries like OpenZeppelin to avoid reinventing the wheel.
- Audit Regularly: Security audits are non-negotiable for public contracts.
- Gas Optimization: Optimize your contract logic to minimize costs.
- Comprehensive Testing: Simulate real-world scenarios to ensure contract stability.
FAQs on Solidity Design Patterns
What are Solidity design patterns ?
Solidity design patterns like Factory, Proxy, and Access Control are great for beginners because they provide clear solutions to common problems.
How do I secure Ethereum smart contracts?
Use patterns like Circuit Breakers, Pull payments, and Access Control, along with thorough auditing and testing.
Can I upgrade smart contracts?
Yes, with the Proxy pattern, you can upgrade contract logic without losing data.
What is a multi signature wallet in Solidity?
A multi signature wallet requires multiple approvals to execute transactions. It is a secure pattern used in DAOs and for managing shared funds.
Are Solidity design patterns beginner-friendly?
Yes! Many patterns, like the Ownership pattern, are easy to understand and implement. Beginners can start with these before moving to advanced patterns like proxies and factories.
How do Solidity design patterns enhance security?
Patterns like Emergency Stop and Pull Over Push Payments reduce vulnerabilities like reentrancy attacks and prevent unauthorized access to sensitive functions.
What is the Proxy pattern in Solidity?
The Proxy pattern enables smart contracts to be upgraded. It works by forwarding calls from a proxy contract to an implementation contract, allowing updates to the implementation without changing the contract’s address.
Why are Solidity design patterns important?
They ensure consistency, reduce the likelihood of bugs, enhance security, and make your smart contracts easier to audit and upgrade.
What is the Factory pattern in Solidity?
The Factory pattern is essential for smart contract development. It lets developers create multiple instances of a contract programmatically while maintaining an organized structure for scalability and tracking.
What tools can I use to implement Solidity design patterns?
Tools like Hardhat, Foundry, and libraries like OpenZeppelin Contracts make it easier to implement and test these patterns effectively.
How does the Proxy pattern enhance Ethereum smart contracts?
The Proxy pattern, widely used in Ethereum smart contracts, allows for upgradable smart contracts by decoupling the storage logic from the implementation logic. It enables developers to update contract functionality without changing its address.
Conclusion
Mastering Solidity design patterns is a critical step toward becoming a proficient blockchain developer. By incorporating these patterns into your smart contract development process, you’ll write code that is not only secure but also scalable and efficient.
Whether you’re learning from a Solidity tutorial for beginners or refining advanced techniques, these patterns will help you build better DApps. Start experimenting with them today, and don’t forget—clean, secure code is the foundation of every successful Ethereum project.
Now that you know the tools, what will you create next?