Solidity Tutorial Chapter 16: When working with Solidity, understanding the difference between storage and memory can make a world of difference, both in terms of cost-effectiveness and the overall efficiency of your smart contract. These two terms may sound like they’re just technical jargon, but they play a huge role in the way your smart contracts function and impact how much gas you’re using.
In this chapter, let’s break down the essentials of Solidity’s storage and memory. We’ll explore when and why you should use each one, what common pitfalls to avoid, and some tips to keep your gas costs as low as possible. By mastering storage and memory in Solidity, you’ll make your smart contracts faster, cheaper, and more scalable.
Table of Contents
What are Storage and Memory in Solidity?
In Solidity, data can be stored in different locations within the Ethereum Virtual Machine (EVM), and each comes with its own costs and behaviors. Solidity gives us three main options to work with: storage, memory, and stack. The latter, stack, is highly limited in terms of size and is usually only relevant for very small variables and quick computations.
Let’s take a look at the two main types we’ll focus on: storage and memory.
Storage in Solidity
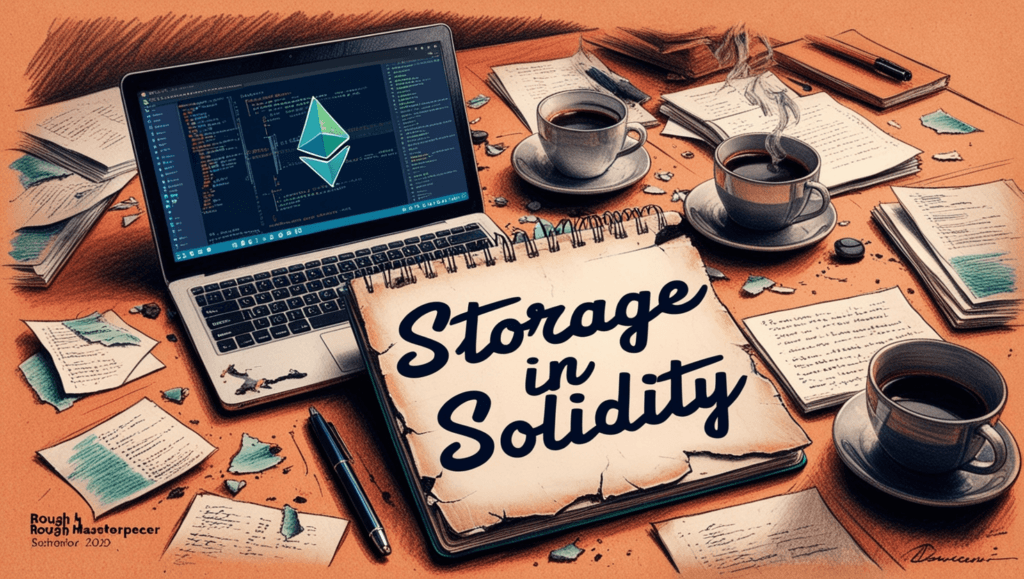
When we talk about storage in Solidity, we’re referring to the persistent data that gets saved directly on the blockchain. In simpler terms, this is the permanent part of your contract’s data that stays even after the function or contract call ends. Every time you store something here, you’re essentially saving it on the blockchain, making it visible and accessible across other functions and transactions.
Storage is where your contract’s state variables live. But beware: since this is on-chain data, it’s the most expensive in terms of gas costs. Every read and write to storage will directly impact how much gas is used, so you want to be strategic about what you store here.
Example of storage in Solidity:
uint public numberOfTokens; // Stored in contract storage
This numberOfTokens
variable is stored in the contract’s state and will remain on-chain for the contract’s lifetime. Updating it will incur gas costs since it changes the blockchain’s state.
Memory in Solidity
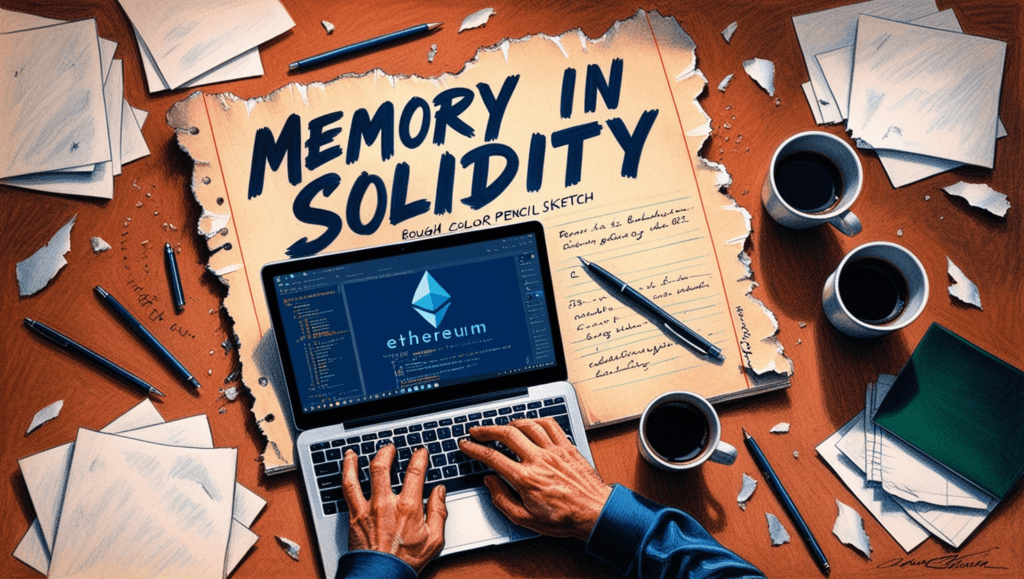
On the other hand, memory in Solidity is for temporary data. It exists only while a function is executing and is wiped out once the function call is complete. Think of memory as a local workspace—quick, efficient, and non-persistent. It’s more affordable than storage, but anything stored here will disappear after the function completes.
Memory is ideal for handling temporary calculations and variables within functions. Since it doesn’t impact the contract’s state on the blockchain, it’s a fantastic option for working with data that doesn’t need to be permanent.
Example of memory in Solidity:
function calculateSum(uint x, uint y) public pure returns (uint) {
uint result = x + y; // Stored temporarily in memory
return result;
}
In this example, result
is stored in memory, not on-chain, which keeps it cost-efficient.
Storage vs. Memory: Key Differences in Solidity
To make the right choice between storage and memory in Solidity, it helps to know the key differences:
- Persistence: Storage is persistent and remains on the blockchain, while memory is temporary and disappears once a function finishes executing.
- Cost: Storage is more expensive than memory, as it requires writing to the blockchain. Memory is cheaper and ideal for temporary values.
- Read/Write Speed: Reading from and writing to storage is slower than memory. This difference may seem small, but it adds up, especially in complex contracts.
When to Use Storage vs. Memory in Solidity
Choosing between storage and memory in Solidity can be tricky, but here are some guidelines:
- Use Storage for Persistent Data: Any data you need to access or update across multiple function calls should go in storage. For instance, user balances, contract states, and configuration settings belong in storage.
- Use Memory for Temporary Calculations: If you’re performing temporary calculations or need a quick local variable, use memory. This keeps your gas costs low and your code efficient.
Let’s go through a practical example to clarify this.
Practical Example: Storage vs. Memory
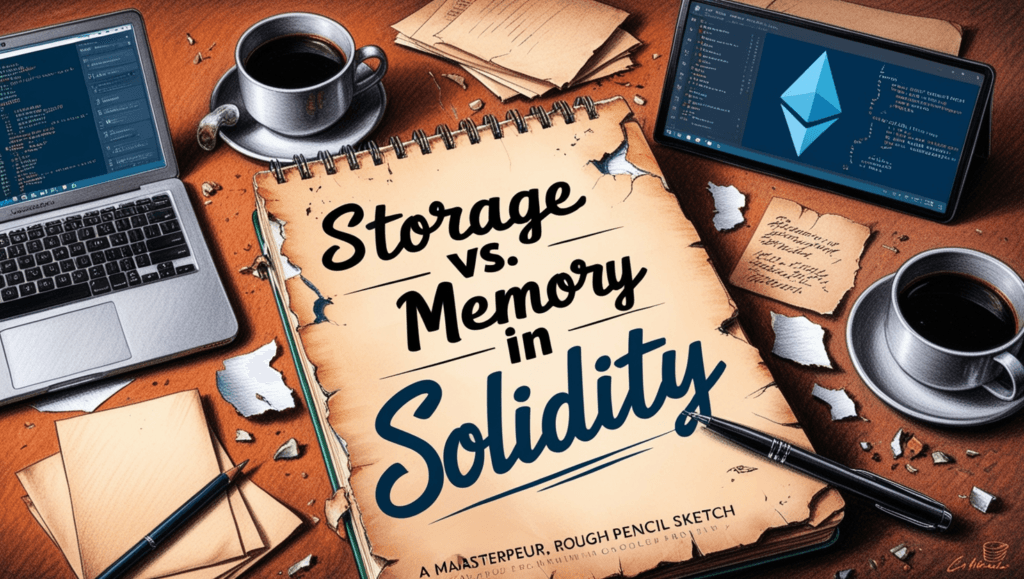
Imagine we’re building a contract that keeps track of users’ balances and provides a way to temporarily calculate rewards based on some conditions.
Here’s a simplified example:
pragma solidity ^0.8.0;
contract Rewards {
mapping(address => uint) public balances;
function addBalance(address user, uint amount) public {
balances[user] += amount; // Updating storage
}
function calculateTemporaryReward(uint rate) public view returns (uint) {
uint temporaryReward = balances[msg.sender] * rate; // Using memory for temp calculation
return temporaryReward;
}
}
In this example:
- The
balances
mapping is stored on-chain as it represents the persistent state of each user’s balance. temporaryReward
is calculated in memory, as it’s a temporary value that isn’t needed after the function finishes executing.
By storing only essential data in storage and using memory for temporary variables, we’re keeping this contract cost-effective.
Common Mistakes with Storage and Memory
Here are some typical mistakes developers make with storage and memory in Solidity:
- Overusing Storage: Many new Solidity developers end up using storage for variables that could easily be kept in memory, leading to unnecessarily high gas fees.
- Mixing Up Storage and Memory with Arrays: When dealing with arrays or structs, forgetting to specify
storage
ormemory
can lead to unexpected behavior. For example,uint[] memory tempArray
anduint[]
storage tempArray
mean very different things in Solidity. - Updating Storage in Loops: Writing to storage within loops is costly. If possible, store data temporarily in memory and then update storage once outside the loop.
Storage and Memory in Arrays and Structs
Arrays and structs add an extra layer of complexity to storage and memory in Solidity. Let’s break down how to work with them efficiently:
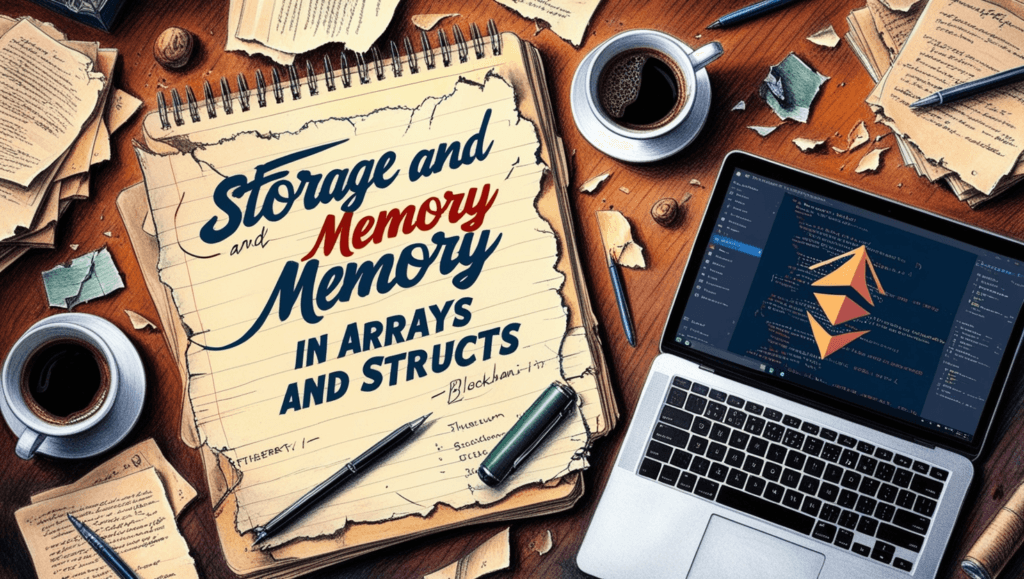
Arrays in Memory
Arrays declared in memory are usually more efficient when dealing with temporary data. Here’s how you can use them:
function processTemporaryArray(uint[] memory data) public pure returns (uint) {
uint total = 0;
for (uint i = 0; i < data.length; i++) {
total += data[i];
}
return total;
}
Here, data
is a temporary array that only exists while processTemporaryArray
is running. It’s cheaper than a storage array since it’s not saved on-chain.
Structs in Storage
Structs are typically used to represent complex data structures that need to persist. For instance, if you’re building a voting contract, you might use a struct for each voter’s details:
struct Voter {
bool voted;
uint weight;
address delegate;
}
mapping(address => Voter) public voters; // Stored in storage
This mapping stores each voter’s information in storage, which persists for the contract’s duration.
Storage vs. Memory: Tips for Optimizing Gas Costs
If you’re looking to optimize your gas costs, here are some practical tips when it comes to using storage and memory in Solidity:
- Minimize Storage Writes: Every time you write to storage, it costs gas. Combine writes when possible, or use memory for calculations.
- Use Memory for Temporary Calculations: Avoid using storage for variables that don’t need to be permanent.
- Specify Data Location Explicitly: Always specify
storage
ormemory
for array and struct variables. Solidity’s default behaviors can sometimes lead to unexpected gas costs. - Batch Updates Outside Loops: If you’re looping through data, perform temporary calculations in memory, then update storage afterward.
Conclusion: Mastering Storage and Memory in Solidity
By now, you should have a solid understanding of storage and memory in Solidity—two critical elements for efficient smart contract design. Storage is your go-to for data that needs to persist on-chain, while memory is ideal for temporary variables within functions. By using each correctly, you can reduce gas costs, boost performance, and create smarter, more scalable contracts.
In the world of Solidity, small optimizations can have a big impact. With this knowledge, you’re now equipped to make those smart, gas-efficient choices that will set your contracts apart on the blockchain.