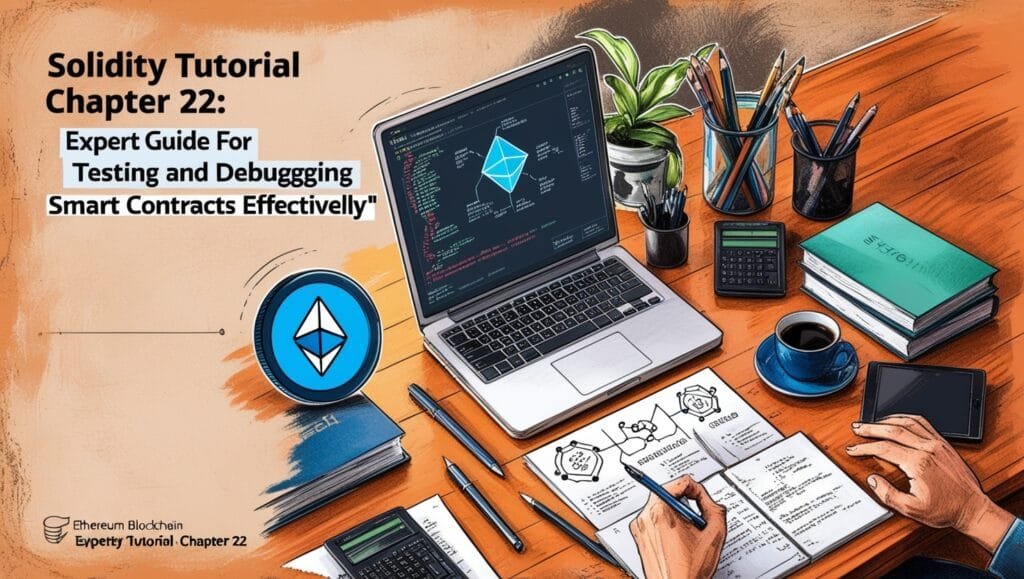
Solidity Tutorial Chapter 22: Expert Guide for Testing and Debugging Smart Contracts Effectively
Testing and Debugging Smart Contracts in Solidity: In the world of blockchain development, testing and debugging smart contracts is a must-have skill. Smart contracts are immutable once deployed, meaning there’s no room for mistakes. Thorough testing and effective debugging are crucial to ensure security, functionality, and performance. This chapter provides a step-by-step guide to testing and debugging Solidity contracts, with practical examples, tools, and tips to simplify the process.
Table of Contents
Why Testing and Debugging Smart Contracts Matter
Smart contracts run on blockchain technology, handling real-world assets and sensitive data. Failing to test them rigorously or leaving bugs unaddressed can lead to financial losses or compromised systems. Here’s why testing and debugging are essential:
- Smart Contract Security: Bugs can expose vulnerabilities, leading to exploits or hacks.
- Gas Optimization: Debugging inefficient code reduces transaction costs.
- Reliable Decentralized Applications (DApps): Testing ensures your app works as expected under all conditions.
- Seamless Upgrades: Debugging simplifies transitioning to upgradable smart contracts without compromising functionality.
Setting Up Your Testing Environment
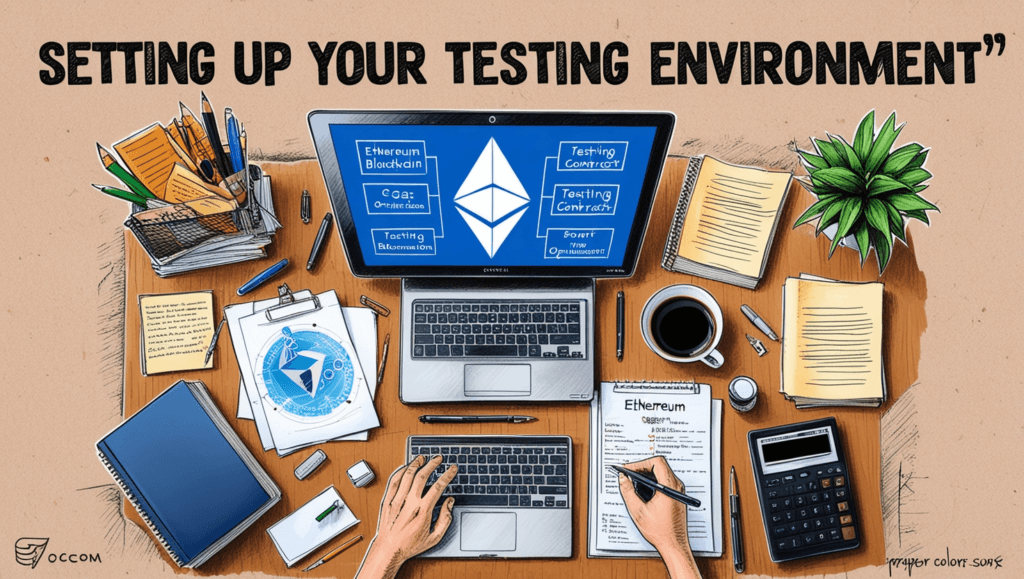
Before diving into testing, you need the right tools. The most popular frameworks for testing and debugging Solidity contracts are Hardhat and Foundry.
Step 1: Install Hardhat
Hardhat offers a developer-friendly environment for testing and debugging.
1. Create a project directory:
mkdir SolidityTesting
cd SolidityTesting
2 .Install Hardhat:
npm init -y
npm install --save-dev hardhat
3. Set up your project:
npx hardhat
Select “Create a basic sample project” for a ready-to-go structure.
Step 2: Install Foundry
Foundry is a lightweight and powerful framework for writing and testing Solidity directly.
1. Install Foundry:
curl -L https://foundry.paradigm.xyz | bash
foundryup
2. Initialize your project:
forge init FoundryTesting
Both tools provide robust features for testing and debugging, so choose based on your workflow preference.
Writing a Smart Contract for Testing
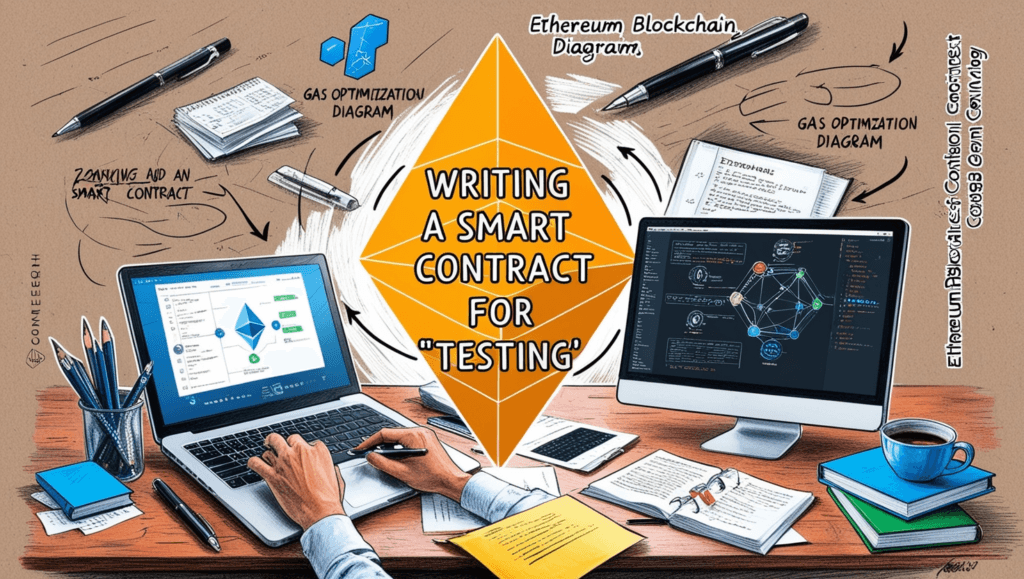
Let’s use a simple voting smart contract to illustrate testing and debugging.
Example Contract: Voting.sol
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract Voting {
mapping(string => uint256) private votes;
string[] public candidates;
function addCandidate(string memory name) public {
candidates.push(name);
}
function vote(string memory name) public {
votes[name] += 1;
}
function getVotes(string memory name) public view returns (uint256) {
return votes[name];
}
}
This contract allows users to add candidates, vote, and view the number of votes for each candidate.
Testing Smart Contract with Hardhat
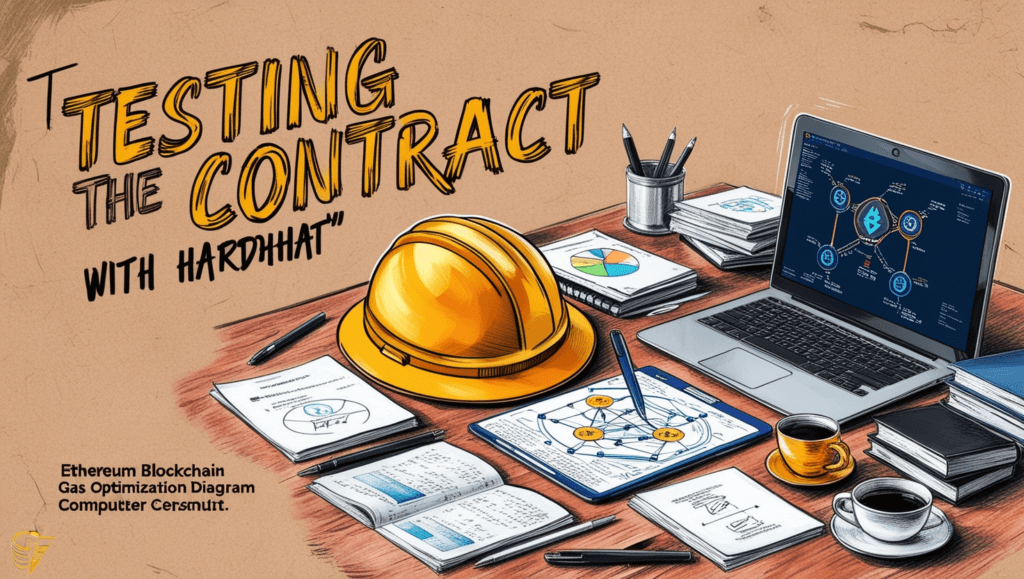
Hardhat makes it easy to write JavaScript-based tests.
Step 1: Create a Test File
In the test
folder, create a file named VotingTest.js
:
const { expect } = require("chai");
const { ethers } = require("hardhat");
describe("Voting Contract", function () {
let voting;
beforeEach(async function () {
const Voting = await ethers.getContractFactory("Voting");
voting = await Voting.deploy();
await voting.deployed();
});
it("Should add a candidate", async function () {
await voting.addCandidate("Alice");
const candidates = await voting.candidates(0);
expect(candidates).to.equal("Alice");
});
it("Should vote for a candidate", async function () {
await voting.addCandidate("Alice");
await voting.vote("Alice");
const votes = await voting.getVotes("Alice");
expect(votes).to.equal(1);
});
});
Step 2: Run the Tests
Execute the tests:
npx hardhat test
Hardhat provides detailed outputs, highlighting both successful and failing tests.
Testing Smart Contract with Foundry
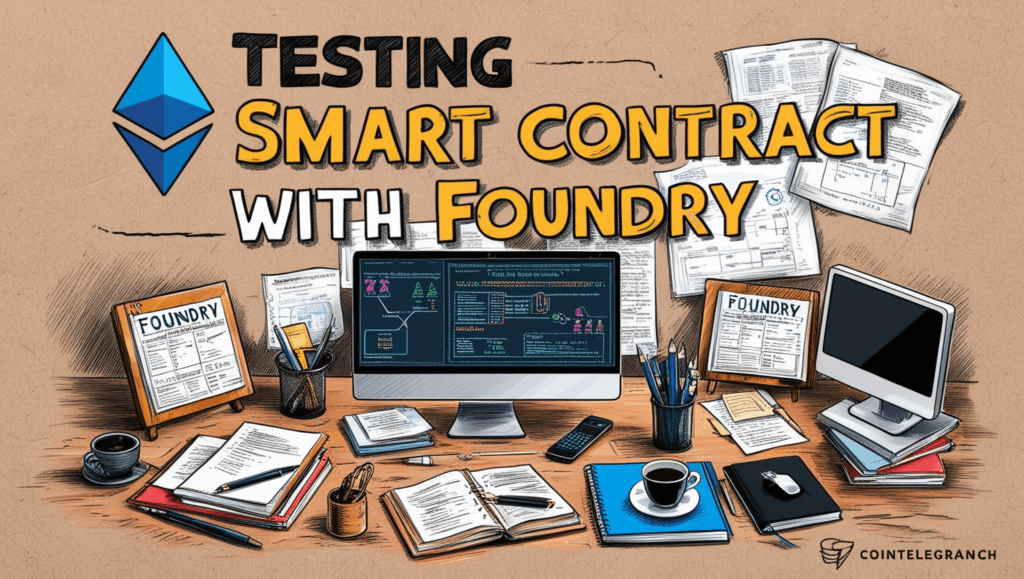
Foundry allows you to write tests directly in Solidity, which can be more intuitive for developers.
Step 1: Create a Test File
In the test
folder, create a file named VotingTest.t.sol
:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "forge-std/Test.sol";
import "../src/Voting.sol";
contract VotingTest is Test {
Voting voting;
function setUp() public {
voting = new Voting();
}
function testAddCandidate() public {
voting.addCandidate("Alice");
string memory candidate = voting.candidates(0);
assertEq(candidate, "Alice");
}
function testVote() public {
voting.addCandidate("Alice");
voting.vote("Alice");
uint256 votes = voting.getVotes("Alice");
assertEq(votes, 1);
}
}
Step 2: Run the Tests
Execute Foundry tests with:
forge test
Foundry’s output is detailed, showing gas usage and error traces.
Debugging Smart Contracts
Testing identifies bugs, but debugging pinpoints their causes. Here’s how to debug effectively:
Debugging with Hardhat
Hardhat’s console.log
is a lifesaver for debugging Solidity contracts.
Add Logs in Your Contract
import "hardhat/console.sol";
function vote(string memory name) public {
console.log("Voting for:", name);
votes[name] += 1;
}
When running tests, logs appear in the terminal, helping you trace execution paths.
Debugging with Foundry
Foundry’s built-in debugging tools provide detailed traces of transaction failures.
For example, if a test fails, Foundry highlights:
- The line of code causing the issue
- The values of relevant variables
- A detailed error message
Best Practices for Testing and Debugging
- Test Every Function: Ensure all public and external functions are tested.
- Simulate Edge Cases: Test for unexpected inputs, overflows, and reentrancy attacks.
- Use Static Analysis Tools: Tools like Slither and MythX catch vulnerabilities early.
- Monitor Gas Usage: Optimize your contract by analyzing gas-heavy functions.
- Write Failing Tests First: Use failing tests to define expected behavior, then fix the code.
Debugging Real-World Scenarios
Debugging isn’t limited to simple contracts. Use these techniques for:
- DeFi Protocols: Simulate liquidations and extreme market conditions.
- NFT Contracts: Debug metadata retrieval and transfer mechanics.
- Supply Chain Apps: Track multi-step workflows for inconsistencies.
Conclusion
Testing and debugging Solidity smart contracts require patience and precision. By using tools like Hardhat and Foundry, and following best practices, you can ensure your contracts are secure, efficient, and reliable. Whether you’re building decentralized finance (DeFi) platforms, non-fungible tokens (NFTs), or any other Ethereum-based application, robust testing and debugging are non-negotiable.
With this guide, you’re equipped to tackle any challenges in smart contract testing and debugging. Start small, iterate, and refine your skills to become a Solidity pro!
Got a tricky bug? Share your experience in the comments below!