Solidity Tutorial Chapter 3 : Hey there, fellow blockchain adventurer! 👋 If you’ve been following along in our Solidity tutorials, you already know the basics of writing smart contracts. Now it’s time to dig deeper into something every Solidity developer needs to master: data types and variables.
Think of this chapter as the foundation of all your smart contract creations. Just like every good recipe needs the right ingredients, your smart contract needs the right types of data. In this blog, we’ll keep it super casual, breaking things down step by step so you can start using Solidity variables with confidence.
Why Are Data Types and Variables Important?
Before we jump into the code, let’s quickly talk about what data types and variables are and why they matter in Solidity smart contracts. Simply put, data types are different forms of information you can store, like numbers, text, addresses, and even true/false values.
A variable is like a box where you keep that information. The kind of box (or variable) you choose depends on the type of data you’re storing. For example, if you’re storing a number, you’ll use a number box (an integer). If you’re storing text, you’ll use a text box (a string).
So, let’s take a look at the different types of “boxes” available in Solidity and how to use them!
Common Solidity Data Types You Should Know
Here’s a quick rundown of the most commonly used data types in Solidity:
- uint: Short for “unsigned integer,” this is used for positive whole numbers.
- int: Stores both positive and negative numbers.
- bool: Short for “boolean,” it stores true or false values.
- address: Stores Ethereum addresses (this one is super important for blockchain!).
- string: Used to store text, like messages or names.
- bytes: Stores raw data (we’ll explain this one too, don’t worry).
You’ll see each of these used often when coding smart contracts, so let’s break them down one by one.
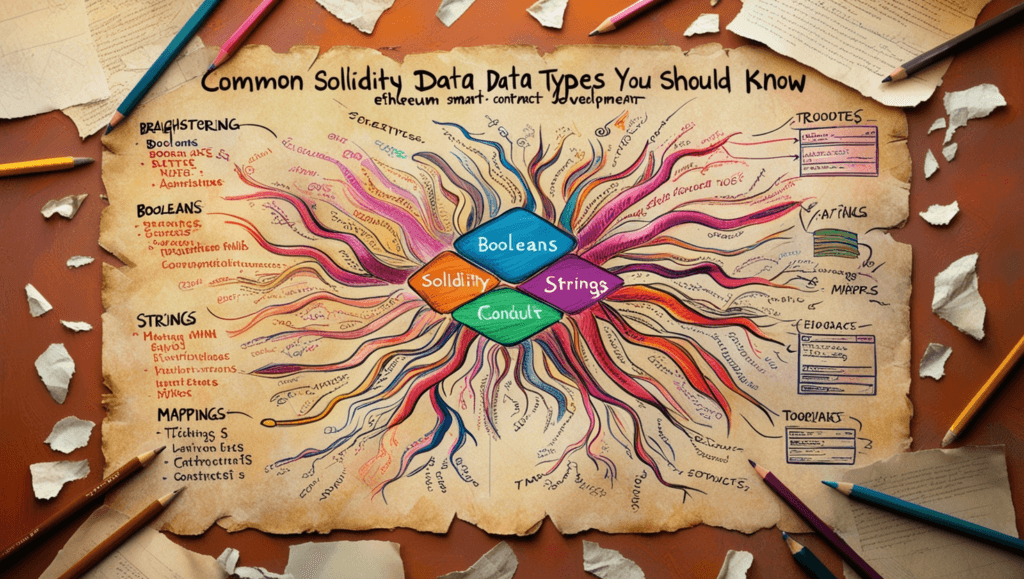
Unsigned Integers (uint
)
Let’s kick things off with unsigned integers—or as we call them in Solidity, uint
. These are used for storing positive whole numbers (no negative values allowed!). Whether you’re storing token balances, ages, or counts, this is the go-to data type.
Example:
pragma solidity ^0.8.0;
contract MyContract {
uint public myNumber = 100;
}
In the example above, myNumber
is a variable of type uint
, which is storing the value 100
. And because it’s public
, anyone can see this number.
But wait—there’s more to uint
! You can specify how big the number should be by using uint8
, uint16
, uint32
, all the way up to uint256
. In most cases, uint
is shorthand for uint256
(which can store really big numbers).
Integers (int
)
Need to store negative numbers? That’s where int
comes into play. An integer can store both positive and negative numbers, which is super handy when you’re working with things like temperatures or balances that can dip below zero.
Example:
pragma solidity ^0.8.0;
contract MyContract {
int public temperature = -20;
}
Here, temperature
is of type int
and stores -20
. Like uint
, you can specify the size of the integer (e.g., int8
, int16
, etc.).
Booleans (bool
)
Next up, we’ve got the boolean data type. A boolean (or bool
) is used to store true/false values. This is perfect for when you need to keep track of things like whether a contract is active or not.
Example:
pragma solidity ^0.8.0;
contract MyContract {
bool public isActive = true;
}
In the above example, we’ve declared a bool
variable called isActive
and set it to true
. This means the contract is currently active. You can toggle this between true
and false
as needed.
Ethereum Addresses (address
)
In the world of blockchain, addresses are key. They represent accounts and smart contracts, and you’ll use them all the time in Solidity. The address
data type is used to store Ethereum addresses.
Example:
pragma solidity ^0.8.0;
contract MyContract {
address public myAddress = 0x1234567890abcdef1234567890abcdef12345678;
}
Here, we’re storing an Ethereum address in the myAddress
variable. Addresses in Solidity are always 20 bytes long and stored in hexadecimal format (as shown above).
Strings (string
)
Want to store some text? That’s where strings come in. In Solidity, string
is used to store text data, whether it’s a name, a message, or any other type of text.
Example:
pragma solidity ^0.8.0;
contract MyContract {
string public greeting = "Hello, Blockchain World!";
}
In this example, we’ve declared a string
variable called greeting
and assigned it the value "Hello, Blockchain World!"
. Strings can be read, updated, and used in various ways throughout your smart contracts.
Bytes (bytes
)
Bytes are a bit more advanced, but don’t worry, we’ll keep it simple. Bytes store raw data and are often used when you need to work with lower-level operations in blockchain, like cryptographic hashes or binary data.
Example:
pragma solidity ^0.8.0;
contract MyContract {
bytes32 public myHash = keccak256(abi.encodePacked("Blockchain"));
}
In this example, we use the bytes32
type to store a cryptographic hash. Don’t worry too much about the technical stuff right now; just know that bytes
are useful for handling raw data and hashes.
Declaring Variables in Solidity
Now that you’ve got a good understanding of the basic data types, let’s look at how to actually declare variables in Solidity.
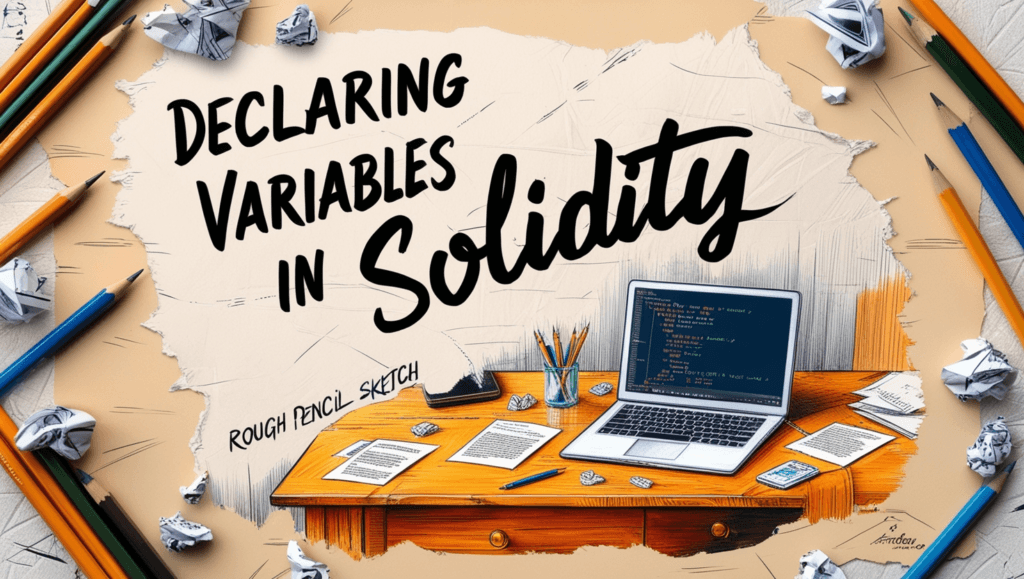
In Solidity, variables come in three main flavors:
- State variables: These are stored permanently on the blockchain.
- Local variables: These exist only within functions and aren’t stored on the blockchain.
- Global variables: These provide information about the blockchain itself, like the current block number.
pragma solidity ^0.8.0;
contract MyContract {
uint public stateVariable = 50; // State variable
function getLocalVariable() public pure returns (uint) {
uint localVariable = 10; // Local variable
return localVariable;
}
function getBlockNumber() public view returns (uint) {
return block.number; // Global variable
}
}
- State variable: Stored permanently on the blockchain (
stateVariable
). - Local variable: Only exists within the function (
localVariable
). - Global variable: Provides blockchain-specific information like
block.number
.
Wrapping It Up: Key Takeaways
You’ve just completed another important chapter in your Solidity journey! 🎉 In this tutorial, we covered:
- Unsigned integers (
uint
): Great for storing positive numbers. - Integers (
int
): Handle both positive and negative numbers. - Booleans (
bool
): Store true or false values. - Addresses (
address
): Used for Ethereum addresses. - Strings (
string
): Perfect for storing text. - Bytes (
bytes
): Handle raw data and cryptographic hashes.
These are the basic building blocks of your smart contracts, and knowing when and how to use them is key to writing efficient and secure code. In the next chapter, we’ll dive into something super exciting—conditional logic in Solidity. We’ll show you how to add smarts to your contracts using if
statements, loops, and more.
Until then, keep experimenting with these data types and variables, and you’ll soon become a Solidity pro! Keep up the awesome work, and I’ll see you in the next tutorial! 🌟