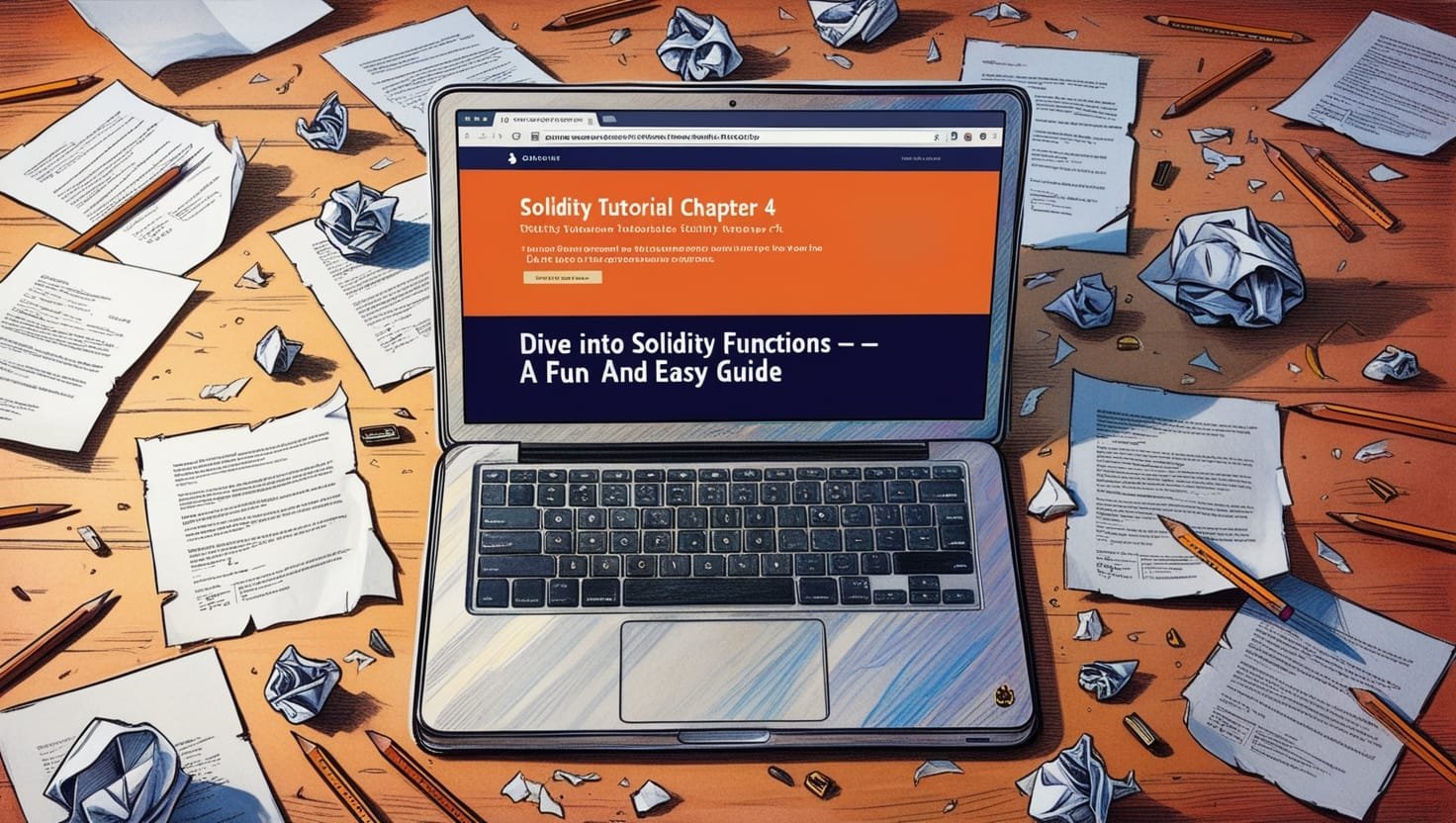
Solidity Tutorial Chapter 4 : Dive Into Solidity Functions – A Fun and Easy Guide
Solidity Tutorial Chapter 4 : Welcome back to our Solidity tutorial series! Now that we’ve covered the basics of data types and variables, it’s time to dive into something even more exciting—functions in Solidity. Whether you’re new to coding or just starting with smart contracts, understanding functions is crucial to writing more advanced and secure code.
In this chapter, we’re going to break down Solidity functions in a super casual, easy-to-understand way. No heavy technical jargon, just real talk about how things work. By the end, you’ll have a solid understanding of what functions are, how they work, and how to use them effectively in your smart contracts. Let’s get started!
What are Functions in Solidity?
At its core, a function is a block of code designed to perform a particular task. Think of it as a recipe in the kitchen. You follow a set of steps to create something, and those steps make up the function.
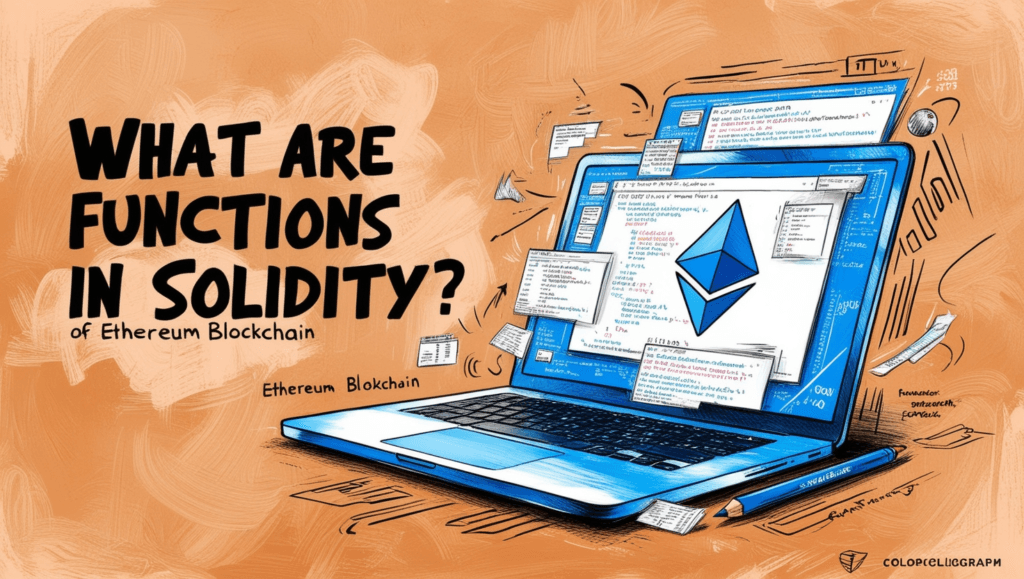
In Solidity, functions allow you to execute specific tasks within your smart contract, such as transferring funds, calculating values, or managing user data. Functions are key to building interactive and dynamic smart contracts.
Types of Functions in Solidity
Now, let’s break down the different types of functions in Solidity so you can get comfortable with how they’re used.
1. Public Functions
Public functions can be called from anywhere—both within the contract and externally (like from another contract or user). If you want certain functionalities to be accessible by users, you’d typically use public functions.
function myPublicFunction() public {
// code here
}
Example: A simple contract that allows users to store and retrieve data using public functions.
contract DataStorage {
uint data;
function storeData(uint _data) public {
data = _data;
}
function retrieveData() public view returns (uint) {
return data;
}
}
2. Private Functions
As the name suggests, private functions can only be accessed and used within the contract itself. This means no other contract or external user can call it. Private functions are great when you have internal logic that you don’t want exposed to the outside world.
function myPrivateFunction() private {
// code here
}
3. Internal Functions
Internal functions are a mix of public and private. They can be called within the contract and by derived contracts (contracts that inherit from your contract), but they’re not accessible externally. It’s like sharing secrets with your family, but not with the world.
function myInternalFunction() internal {
// code here
}
4. External Functions
External functions can only be called from outside the contract. This is useful when you want certain actions to only be triggered externally (by users or other contracts).
function myExternalFunction() external {
// code here
}
Function Modifiers – Adding Extra Controls
Now that we’ve talked about the types of functions, let’s look at function modifiers. These allow you to add extra conditions or controls on how and when a function can be called.
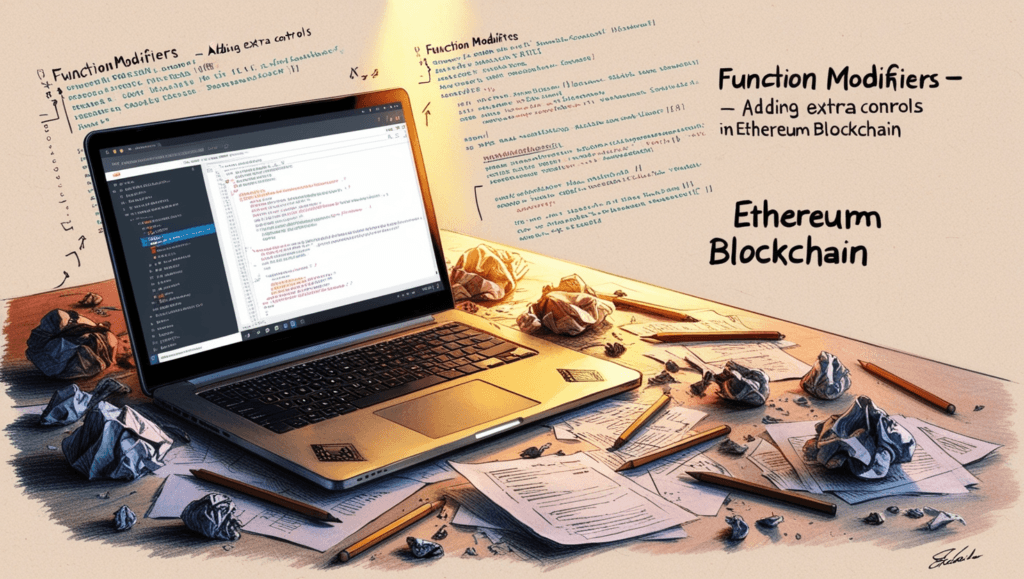
Modifiers are super handy for adding security layers to your functions. You can control access, restrict certain actions, or ensure certain conditions are met before a function is executed.
Example: Only Owner Modifier
modifier onlyOwner {
require(msg.sender == owner, "Not authorized");
_;
}
function restrictedFunction() public onlyOwner {
// code that only the owner can execute
}
In this example, only the owner of the contract can call the restrictedFunction
. The onlyOwner
modifier adds a layer of security by checking that the person calling the function is the owner of the contract.
View and Pure Functions
Now, let’s talk about view and pure functions, which are special types of functions that don’t alter the state of the contract.
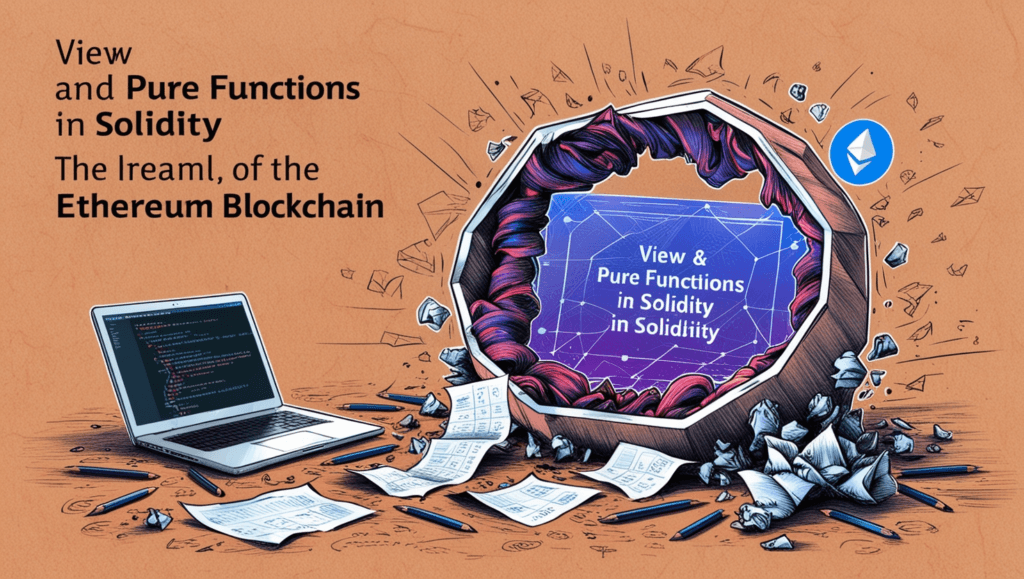
View Functions
A view function is used when you just want to read data from the blockchain, but not modify it. Think of it as reading a book—you’re gathering information but not changing anything.
function getBalance() public view returns (uint) {
return balance;
}
In this example, the getBalance
function is used to check the balance, but it doesn’t change any data. It’s purely for viewing.
Pure Functions
A pure function, on the other hand, doesn’t read or modify the contract’s data at all. It’s used for internal calculations or logic that doesn’t rely on any external data.
function add(uint a, uint b) public pure returns (uint) {
return a + b;
}
Here, the add
function simply adds two numbers together and returns the result. It doesn’t interact with any contract data, so it’s marked as pure.
Payable Functions – Sending and Receiving Ether
If you’ve worked with Ethereum, you know that Ether is the native cryptocurrency of the platform. In Solidity, you can write functions that are capable of sending and receiving Ether by using the payable keyword.
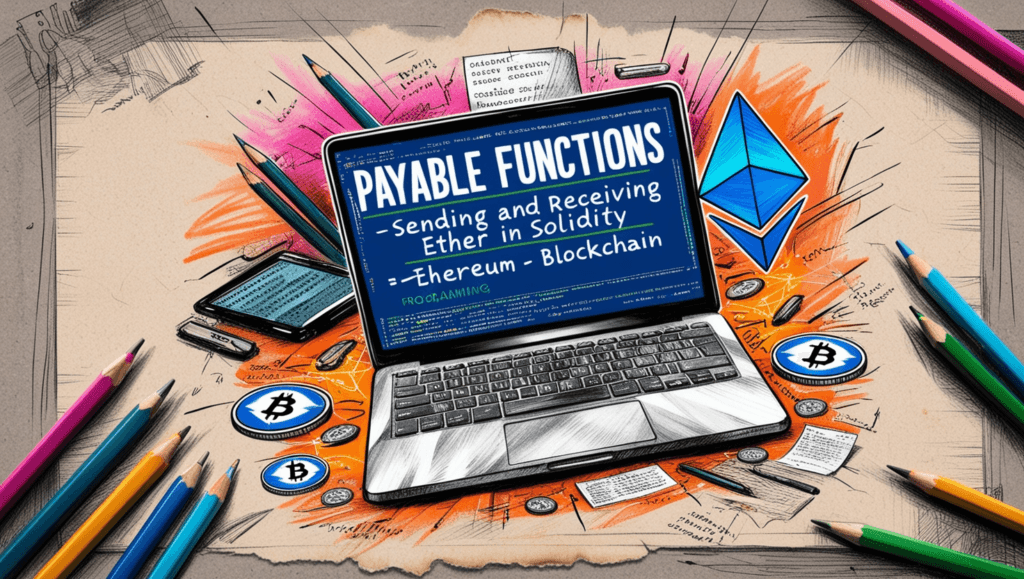
Example: Simple Payment Function
function payMe() public payable {
// contract can now receive Ether
}
By marking a function as payable, you allow the contract to receive Ether. It’s as simple as that! Without the payable modifier, a function cannot handle Ether transactions.
Returning Values from Functions
Functions can also return values. Let’s say you want to calculate something and return the result to the user.
Example: Return Value from a Function
function multiply(uint a, uint b) public pure returns (uint) {
return a * b;
}
In this example, the function multiply
takes two numbers, multiplies them, and returns the result. It’s a pure function because it doesn’t rely on any contract data, and it returns a calculated value.
Gas Optimization with Functions
Every time you execute a function on the Ethereum blockchain, you’re consuming gas, which costs Ether. As a developer, it’s essential to optimize your functions to reduce the gas fees for users.
Here are a few tips for gas optimization:
- Minimize State Changes: Writing to the blockchain (like changing variables) consumes a lot of gas. Keep state changes to a minimum.
- Use View and Pure Functions: Functions that don’t alter the state of the contract (view and pure functions) use less gas.
- Shorter Functions: Simpler, more concise functions use less gas than long, complex ones.
- Batch Operations: Instead of performing multiple individual actions, try to batch them together to reduce gas costs.
Wrapping Up
That’s a wrap on functions in Solidity! Understanding how functions work is a key part of writing smart contracts, and they’ll be central to everything you build. Whether you’re writing public, private, or payable functions, mastering the basics will make you a more confident and capable Solidity developer.
In this chapter, we explored the different types of functions, how to use modifiers for added security, and how to return values and optimize for gas. Now, you’re ready to take your Solidity knowledge to the next level!
Stay tuned for our next chapter, where we’ll dig into events and error handling in Solidity!