Solidity Tutorial Chapter 7 : Hey there, fellow Solidity enthusiast! Welcome to Chapter 7 of our Solidity journey. If you’ve made it this far, congratulations—you’re already ahead of the curve! Today, we’re diving into two of the most powerful data structures in Solidity: arrays and mappings. These bad boys are essential when it comes to handling and organizing data in smart contracts.
We’ll break things down step by step, keeping it super casual and beginner-friendly. You’ll learn what arrays and mappings are, how to use them effectively, and how they can be your best friends when building robust, efficient Solidity Smart Contracts.
So, grab a snack, get comfy, and let’s unlock the magic of arrays and mappings!
What Are Arrays in Solidity?
Think of arrays as containers that hold multiple values of the same type. Imagine you’re at a party, and the host gives you a tray with slots for cupcakes. Each cupcake represents a value, and the tray (array) holds them all. You can easily pick a cupcake (value) based on its position (index) in the tray.
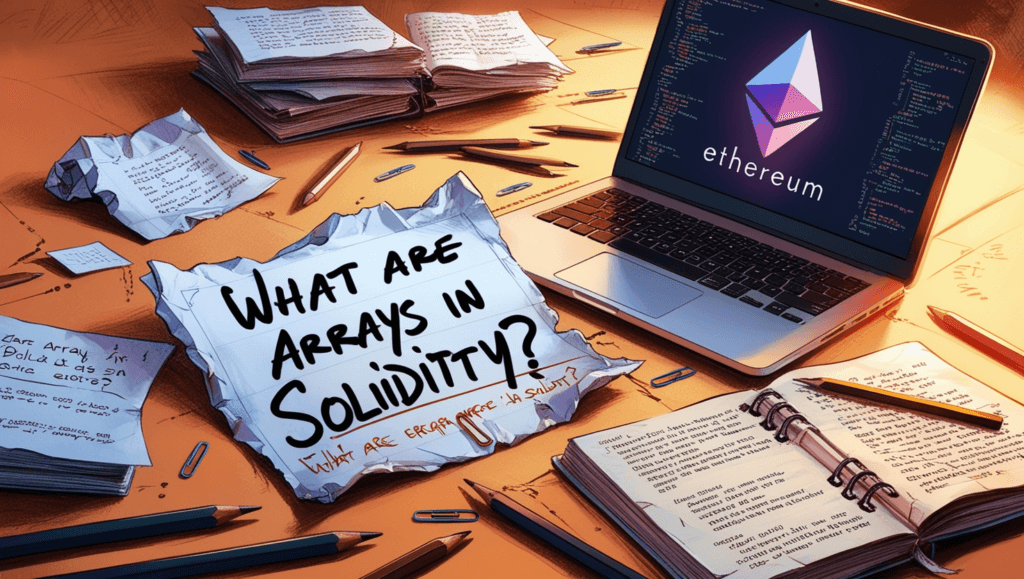
In Solidity, there are two main types of arrays:
- Fixed-size arrays: These arrays have a set number of slots, and you can’t add or remove any more items.
- Dynamic arrays: These arrays can grow or shrink as needed, giving you more flexibility.
Declaring Arrays in Solidity
Let’s start with a simple example. Here’s how you can declare both types of arrays:
pragma solidity ^0.8.0;
contract ArrayExample {
// Fixed-size array of 5 integers
uint[5] fixedArray;
// Dynamic array of integers
uint[] dynamicArray;
}
In this example:
fixedArray
is an array that can hold exactly 5 unsigned integers.dynamicArray
is a flexible array that can grow or shrink, depending on what you need.
Adding and Accessing Data in Arrays
Now, let’s see how to work with arrays. You’ll need to know how to add and access values in an array. Here’s a simple contract that shows you how:
pragma solidity ^0.8.0;
contract ArrayManipulation {
uint[] public dynamicArray;
// Add a value to the array
function addValue(uint value) public {
dynamicArray.push(value);
}
// Get a value from the array based on its index
function getValue(uint index) public view returns (uint) {
require(index < dynamicArray.length, "Index out of bounds");
return dynamicArray[index];
}
}
In this example:
- We use
dynamicArray.push(value)
to add a new value to the end of the array. - The
getValue
function allows us to retrieve the value at a specific index (just like picking a cupcake from the tray!).
Removing Data from Arrays
Solidity doesn’t have a built-in method for removing an element from an array. However, there’s a workaround. You can “remove” an element by setting it to zero or shifting the elements, depending on your needs.
Here’s an example where we “remove” an element by shifting all the values to the left:
pragma solidity ^0.8.0;
contract ArrayRemoval {
uint[] public dynamicArray;
// Add values to the array
function addValue(uint value) public {
dynamicArray.push(value);
}
// Remove an element by shifting values
function removeValue(uint index) public {
require(index < dynamicArray.length, "Index out of bounds");
// Shift elements to the left
for (uint i = index; i < dynamicArray.length - 1; i++) {
dynamicArray[i] = dynamicArray[i + 1];
}
// Remove the last element
dynamicArray.pop();
}
}
This method “removes” an element by shifting all the values after it to the left and then using pop()
to remove the last element.
What Are Mappings in Solidity?
Now that we’ve tackled arrays, let’s talk about mappings. If arrays are like trays of cupcakes, then mappings are like directories where you can look up specific values based on a unique key.
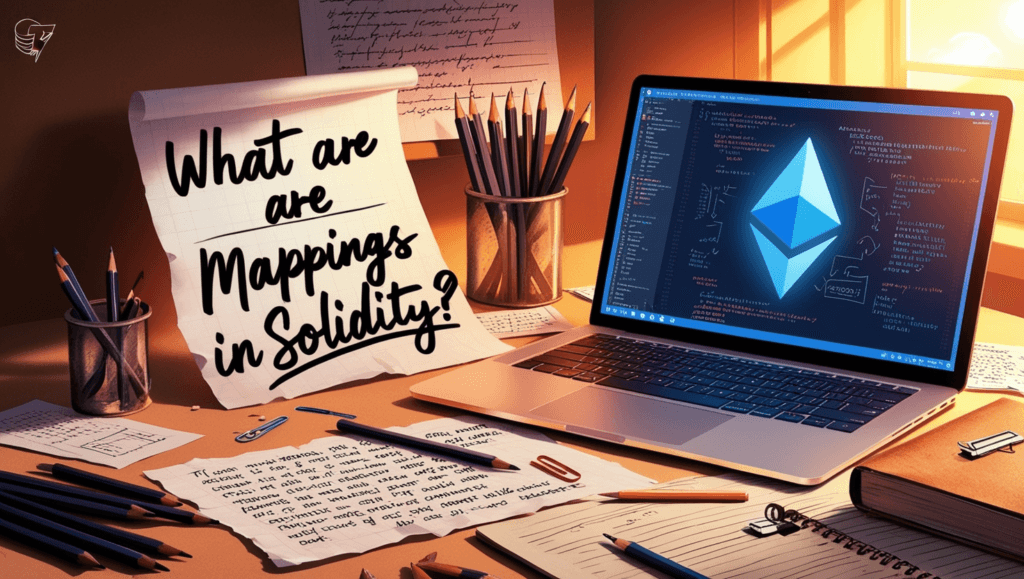
In other words, a mapping is a collection of key-value pairs. Think of it like a phonebook: you look up a name (key), and the phonebook gives you the corresponding phone number (value).
Here’s how you declare a mapping in Solidity:
pragma solidity ^0.8.0;
contract MappingExample {
// Mapping of an address to an unsigned integer
mapping(address => uint) public balances;
}
In this example:
- We’re mapping Ethereum addresses (the keys) to unsigned integers (the values), which could represent something like an account balance.
Adding and Accessing Data in Mappings
Adding data to a mapping is super easy. You just assign a value to a key, and Solidity takes care of the rest.
Here’s a simple contract that demonstrates how to use mappings:
pragma solidity ^0.8.0;
contract MappingManipulation {
mapping(address => uint) public balances;
// Add or update the balance for an address
function updateBalance(uint newBalance) public {
balances[msg.sender] = newBalance;
}
// Get the balance for a specific address
function getBalance(address user) public view returns (uint) {
return balances[user];
}
}
In this example:
- The
updateBalance
function allows you to set or update the balance for the caller’s address (msg.sender
). - The
getBalance
function lets you look up the balance for any Ethereum address by passing it as the key.
Why Use Mappings?
Mappings are incredibly useful in Solidity smart contracts because they allow for constant time lookups. This means you can retrieve a value from a mapping in a single step, regardless of how large the data set is. In contrast, arrays may require looping through every element to find the one you’re looking for.
Mappings are perfect for things like storing user balances, permissions, or any other data that you need to access quickly.
Limitations of Mappings
Before you get too excited, it’s important to know that mappings come with a few limitations. For one, you can’t iterate over a mapping or get a list of all the keys. This is because Solidity doesn’t keep track of the keys you’ve added. So if you need to loop through data, you might want to stick with arrays.
Combining Arrays and Mappings
In many cases, you’ll want to use arrays and mappings together to take advantage of their strengths. For example, you could store data in an array and then use a mapping to keep track of each value’s position in the array.
Here’s an example where we track users and their scores:
pragma solidity ^0.8.0;
contract ArrayMappingCombination {
address[] public users;
mapping(address => uint) public scores;
// Add a new user and their score
function addUser(address user, uint score) public {
users.push(user);
scores[user] = score;
}
// Get a user's score by their address
function getScore(address user) public view returns (uint) {
return scores[user];
}
}
In this contract:
- We’re using an array to store a list of user addresses.
- We’re also using a mapping to store each user’s score.
By combining these two data structures, we can easily manage users and their scores without sacrificing performance.
When to Use Arrays vs. Mappings
Now that you know how to use both arrays and mappings, the big question is: when should you use one over the other?
- Use arrays when you need to store a list of values and might need to iterate through them.
- Use mappings when you need to store data in key-value pairs and want to access them quickly.
If you need both, don’t be afraid to combine them like we did in the last example!
Recap: Arrays and Mappings Are Your Best Friends
Congrats! You’ve just unlocked another major part of your Solidity toolkit. Arrays and mappings are the backbone of smart contracts, allowing you to organize and access data efficiently. Whether you’re building a token, a voting system, or a decentralized app (dApp), these data structures will be invaluable.
Here’s what we covered today:
- Arrays: Great for storing lists of values, with both fixed and dynamic options.
- Mappings: Perfect for storing key-value pairs with fast lookups.
- Combining arrays and mappings: Use them together to manage complex data structures.
In the next chapter, we’ll dive into structs, another powerful tool for organizing data in Solidity. Stay tuned, and happy coding!