Solidity Tutorial Chapter 8: Hey there, Solidity explorer! Welcome to Chapter 8, where we’re about to make your Solidity experience a lot more intuitive with Structs. If you’re looking to group data without creating a mess of variables and mappings, structs are the magic ingredient that will keep your code clean, organized, and easy to read. Structs help you handle complex data structures with a simple, powerful way to organize related information under one roof.
Imagine you’re working on a decentralized application (dApp) that needs to store user info like name, age, and wallet address. Without structs, you’d probably end up juggling several mappings or arrays, which can get confusing quickly. Structs allow you to group that data into one tidy package, making your smart contracts way more manageable.
Why Use Structs in Solidity?
Think of structs as customizable containers for data. They’re ideal for organizing related information and make your code clearer and less error-prone. When you’ve got data like user profiles or product details, structs let you group this data together as one unit. So instead of separate mappings for names, ages, and addresses, you can bundle all that into a single struct, making your life (and code) much simpler.
Declaring a Struct in Solidity
Declaring a struct is simple. Let’s say you want to store details for a User
. Here’s how you’d define it:
pragma solidity ^0.8.0;
contract StructExample {
// Define the "User" struct with three properties
struct User {
string name;
uint age;
address walletAddress;
}
}
In this example:
- We’ve created a struct named
User
with three fields:name
,age
, andwalletAddress
. - Each field has a specific data type, just like individual variables.
Creating and Storing Structs in Solidity
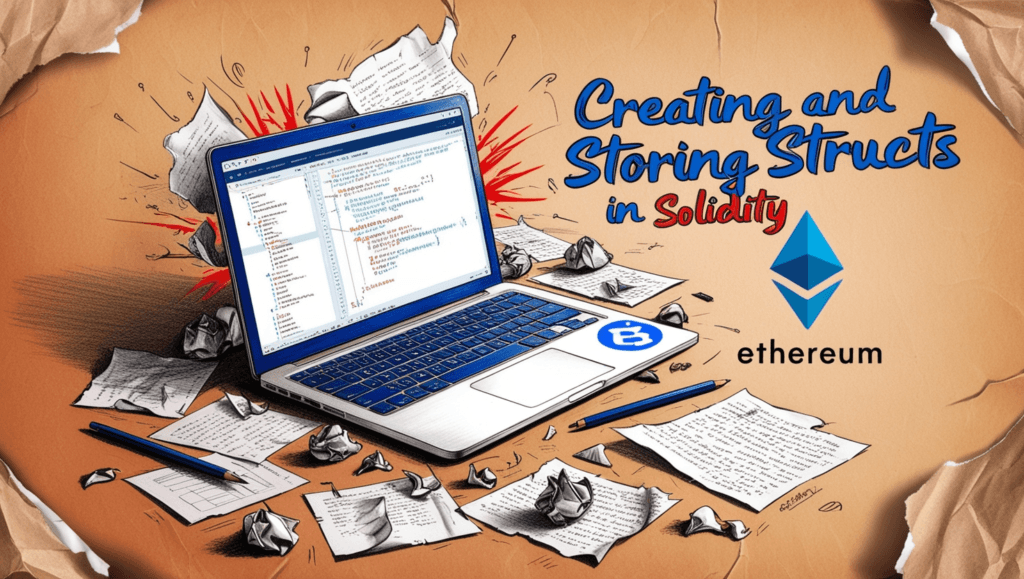
Now that you know how to declare a struct, it’s time to start using it. To store multiple users, we can use an array or a mapping. Here’s an example that shows how to create and store structs in an array:
pragma solidity ^0.8.0;
contract StructExample {
struct User {
string name;
uint age;
address walletAddress;
}
User[] public users;
// Function to add a new user
function addUser(string memory _name, uint _age, address _walletAddress) public {
users.push(User(_name, _age, _walletAddress));
}
}
In this setup:
- We use an array called
users
to store all ourUser
structs. - The
addUser
function takes in the name, age, and wallet address of a user and adds a newUser
struct to theusers
array.
Accessing and Updating Struct Data
Once you’ve stored data in a struct, accessing and updating it is straightforward. Solidity lets you get to any struct field directly, which is a huge plus for readability.
For instance, if you want to retrieve a user’s name or update their age, here’s how you can do it:
pragma solidity ^0.8.0;
contract StructExample {
struct User {
string name;
uint age;
address walletAddress;
}
User[] public users;
function addUser(string memory _name, uint _age, address _walletAddress) public {
users.push(User(_name, _age, _walletAddress));
}
// Get the name of a user by index
function getUserName(uint index) public view returns (string memory) {
require(index < users.length, "Invalid index");
return users[index].name;
}
// Update the age of a user
function updateUserAge(uint index, uint newAge) public {
require(index < users.length, "Invalid index");
users[index].age = newAge;
}
}
Here’s what’s happening:
getUserName
lets you access the name of any user by their index in theusers
array.updateUserAge
enables updating a user’s age by index.
Mappings with Structs
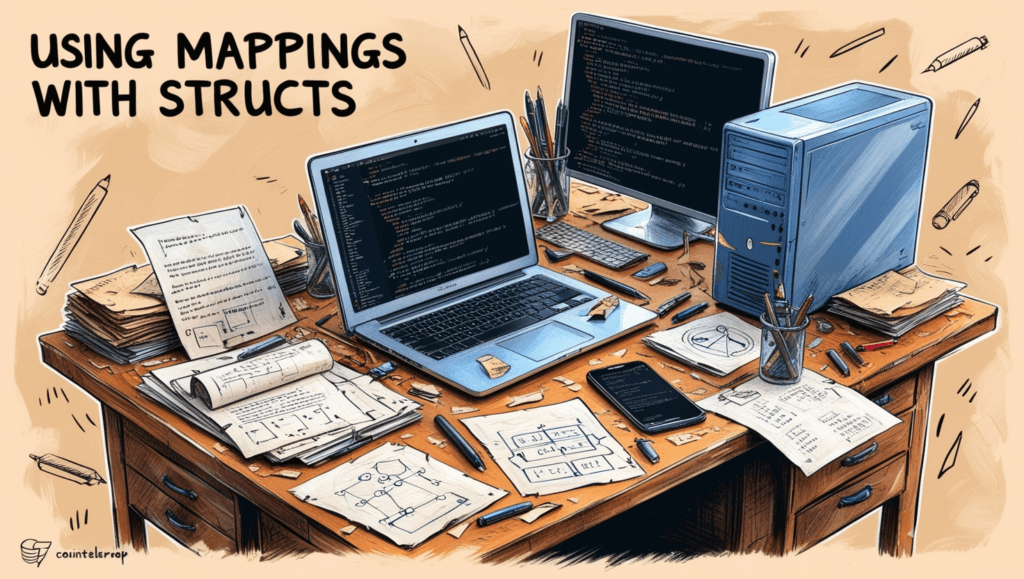
Structs also work great with mappings. If you want to access data based on a unique key (like a wallet address), mappings can make retrieval super quick and easy. Here’s an example:
pragma solidity ^0.8.0;
contract MappingWithStructs {
struct User {
string name;
uint age;
address walletAddress;
}
mapping(address => User) public users;
// Add a new user to the mapping
function addUser(string memory _name, uint _age) public {
users[msg.sender] = User(_name, _age, msg.sender);
}
// Retrieve user information by address
function getUser(address _walletAddress) public view returns (string memory, uint) {
User storage user = users[_walletAddress];
return (user.name, user.age);
}
}
In this example:
- We store each
User
struct in amapping
with the wallet address as the key. - The
addUser
function takes the name and age, and assigns it to the caller’s address. - The
getUser
function allows you to retrieve a user’s name and age using their address.
Why Combine Structs and Mappings?
Using structs with mappings can streamline data storage and make retrieval faster. This setup is ideal for projects where each entity has a unique identifier, like a user’s wallet address. It keeps data organized and makes it easy to retrieve specific entries.
Advanced Structs: Nested Structs
You can even nest structs within each other. Nested structs let you group related entities, which can come in handy when you need complex data structures. Here’s how you can create nested structs:
pragma solidity ^0.8.0;
contract NestedStructs {
struct ContactInfo {
string email;
string phone;
}
struct User {
string name;
uint age;
address walletAddress;
ContactInfo contact;
}
mapping(address => User) public users;
// Add a user with contact info
function addUser(string memory _name, uint _age, string memory _email, string memory _phone) public {
users[msg.sender] = User(_name, _age, msg.sender, ContactInfo(_email, _phone));
}
// Retrieve a user's contact details
function getUserContact(address _walletAddress) public view returns (string memory, string memory) {
ContactInfo storage contact = users[_walletAddress].contact;
return (contact.email, contact.phone);
}
}
In this setup:
- We’ve created a
ContactInfo
struct that’s nested within theUser
struct. - The
addUser
function allows you to input both user and contact information. - The
getUserContact
function retrieves the contact details for a user based on their wallet address.
Best Practices for Structs in Solidity
Here are some tips for getting the most out of structs in Solidity:
- Keep Structs Simple: Solidity’s stack can get overloaded with complex structs. Stick to basic data types whenever possible.
- Minimize Struct Size: Since structs live in
storage
, reducing their size can help cut down on gas costs. - Avoid Arrays Inside Structs: Arrays within structs can lead to increased complexity and higher costs for data updates.
Wrapping Up: Structs as Solidity’s Super Tool for Data Management
Well done! You’ve just added another powerful tool to your Solidity toolkit. Structs make organizing and managing data a breeze, so your smart contracts stay clean, simple, and efficient. Let’s recap what we covered in this chapter:
- Struct Basics: Organize related data fields into a single container.
- Creating and Storing Structs: How to declare, store, and retrieve data in structs.
- Using Mappings with Structs: Combine structs with mappings to quickly access data by unique keys.
- Nested Structs: Grouping multiple structs together for advanced data relationships.
In the next chapter, we’ll be looking at events and logging in Solidity, which will take your smart contracts up a notch by letting them “speak” to the blockchain and share real-time info. Get ready to build even more engaging dApps!