Solidity Tutorial Chapter 9 : Welcome back, Solidity explorer! Now that we’ve covered variables, mappings, and structs, it’s time to introduce another crucial data type in the world of Solidity programming arrays. Arrays are basically lists that let you organize data in a neat, structured way. If you’re building a smart contract and want to manage a list of items, whether they’re addresses, numbers, or strings, arrays are your go-to tool.
Arrays are simple but powerful; learning to use them effectively can give your Solidity skills a huge boost. In this chapter, we’ll look at the ins and outs of arrays, from the different types to best practices that can make your code leaner and more efficient. So, let’s dive in and unlock the power of arrays in Solidity!
Table of Contents
What Exactly Are Arrays in Solidity?
In simple terms, an array is a collection of items of the same type. Think of it as a row of cubbies or slots where each one holds a specific piece of data, like numbers, strings, or even your custom structs.
In Solidity, arrays come in two main types:
- Fixed-size arrays: These arrays have a set length that cannot change.
- Dynamic arrays: These arrays can grow or shrink, adapting to the data you add or remove.
Arrays make organizing and accessing data simple. They’re a perfect fit for managing ordered lists and grouping data that you want to manipulate in a structured way.
Two Types of Arrays: Fixed-Size and Dynamic
To understand how arrays work in Solidity, let’s look at these two types in detail.
Fixed-Size Arrays
Fixed-size arrays are straightforward and, as the name suggests, they have a fixed number of slots. You set their length when you create them, and that’s it; the size won’t change. Here’s how to define a fixed-size array:
uint[5] numbers = [1, 2, 3, 4, 5];
In this example, numbers
is a fixed-size array with five elements. Each slot holds a number, and the array’s length is set in stone.
Dynamic Arrays
Dynamic arrays, on the other hand, are flexible and can grow or shrink in size. Want to add an item? Go for it. Need to remove something? Not a problem. Dynamic arrays are incredibly handy when you don’t know in advance how much data you’ll be handling.
uint[] public dynamicNumbers;
function addNumber(uint _number) public {
dynamicNumbers.push(_number);
}
In this example, dynamicNumbers
is a dynamic array. We can keep adding numbers as needed using the .push()
function, which will automatically increase the array’s size.
Real-Life Use Case: Arrays in a Voting Smart Contract
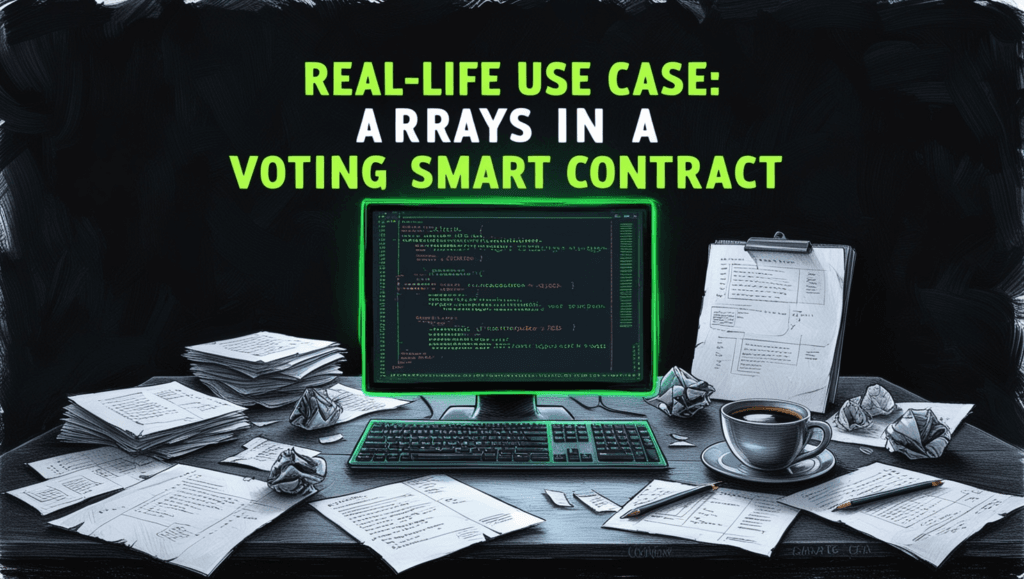
Let’s put arrays to practical use in a Solidity smart contract. Imagine you’re building a simple voting system where you want to keep track of candidates and voters. Arrays can make this easy and efficient.
pragma solidity ^0.8.0;
contract Voting {
string[] public candidates;
address[] public voters;
function addCandidate(string memory _name) public {
candidates.push(_name);
}
function vote(address _voter) public {
voters.push(_voter);
}
}
In this example:
- The
candidates
array stores the names of all the candidates. - The
voters
array keeps track of voter addresses.
Here, the .push()
method is used to add new candidates and voters to their respective arrays. Dynamic arrays make it easy to keep adding items as the contract progresses, without having to specify a maximum size.
Key Array Operations in Solidity
Arrays let you store and retrieve data easily. Here’s a quick look at how you can interact with arrays in Solidity programming:
- Accessing Elements: You can access any element by its index. For example,
candidates[0]
would give you the first candidate in thecandidates
array. - Adding Elements: For dynamic arrays,
.push()
is your go-to method for adding new items. - Updating Elements: Modify an element by referencing its index, like
candidates[0] = "Updated Candidate"
. - Removing Elements: You can remove the last element in a dynamic array with
.pop()
.
Using these operations, you can handle data in a flexible and organized way, allowing you to build more interactive smart contracts.
Best Practices for Using Arrays in Solidity
Arrays are handy, but it’s essential to use them efficiently in Solidity programming. Here are some best practices to keep in mind:
- Opt for Fixed-Size Arrays When Possible: Fixed-size arrays consume less gas and can be more efficient, so use them if the array’s length won’t change.
- Limit Array Length: Dynamic arrays can grow quickly, which can become costly in terms of gas. Limit the length of your array if you know it will be large.
- Be Cautious with
.pop()
:.pop()
is handy, but it only removes the last element in the array. If you need to remove an element at a specific index, you’ll need a workaround. - Avoid Nested Arrays: Arrays within arrays (nested arrays) are difficult to manage in Solidity and can lead to higher gas costs. Try to flatten your data structure if possible.
These practices can help you make the most out of arrays in your smart contracts by improving efficiency and keeping gas costs under control.
How to Manipulate Arrays in Solidity: Practical Tips
Let’s dive deeper into some practical examples for manipulating arrays. Solidity doesn’t have built-in support for every array operation, so sometimes you’ll need to get creative.
Removing Elements by Shifting
Solidity doesn’t support removing a specific element directly, but you can shift elements to “remove” an item.
function removeElement(uint index) public {
require(index < dynamicArray.length, "Index out of bounds");
for (uint i = index; i < dynamicArray.length - 1; i++) {
dynamicArray[i] = dynamicArray[i + 1];
}
dynamicArray.pop();
}
This function removes an element by shifting each item one position to the left, filling the gap. Finally, .pop()
removes the last item to prevent a duplicate.
Arrays and Structs: A Perfect Pair
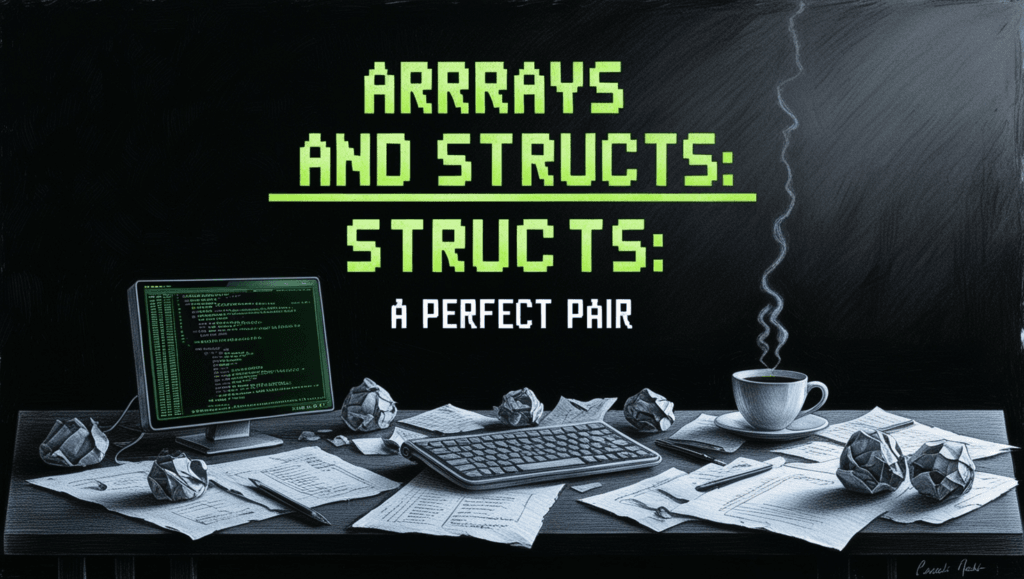
You can use arrays and structs together to handle complex data more effectively. Here’s an example:
struct Task {
string description;
bool completed;
}
Task[] public tasks;
function createTask(string memory _description) public {
tasks.push(Task(_description, false));
}
function completeTask(uint index) public {
tasks[index].completed = true;
}
In this example, Task
is a struct that’s used in an array called tasks
. You can add tasks to the array and mark them as completed. This approach lets you manage lists of tasks (or any other data) with more structure.
Arrays vs. Mappings: When to Use Which
Wondering when to use arrays and when to use mappings? Here’s a quick rundown:
- Use Arrays: When you need an ordered list of items and want to access them by their position.
- Use Mappings: When you want to store data based on unique keys, like addresses.
Both data types have their strengths, so choosing one over the other depends on what you need to achieve. Mappings are more efficient for lookups, while arrays are great for managing sequential data.
Optimizing Arrays for Lower Gas Costs
Arrays can consume a lot of gas, especially if they’re long or require frequent resizing. Here are some tips for keeping your gas costs down:
- Short Arrays Are Best: Large arrays mean higher gas costs, so try to limit the length of dynamic arrays.
- Prefer Mappings for Large Data Sets: If you’re dealing with lots of data and don’t need order, mappings can be a better choice.
- Fixed-Size Arrays for Predictable Data: Fixed-size arrays use less gas, so choose them if you know the data length won’t change.
Remember, gas costs are part of what makes Solidity unique, so it’s wise to keep optimization in mind when designing your contract.
Final Thoughts: Arrays as Essential Tools in Solidity
Arrays are versatile, flexible, and make handling data in Solidity programming easier. From organizing information to building more complex functionalities, arrays provide the structure you need. By understanding array types, operations, and best practices, you’ll be well-equipped to use arrays in your smart contracts.
That’s it for arrays! In Chapter 10, we’ll take a closer look at Solidity modifiers and function visibility—important concepts for securing and organizing your smart contracts.
Happy coding, and enjoy exploring the power of arrays in Solidity!