Yul Programming Tutorial Basics : If you’re diving into the world of Ethereum and smart contracts, you’ve probably come across the term Yul programming. At first appearance, it may appear scary, but don’t worry!, we’ll break down the fundamentals of Yul programming and explain its role in Solidity development. Whether you’re new to the space or looking to improve your smart contract skills, this beginner’s guide will help you get a solid understanding of Yul programming basics. So, let’s dive right in!
Table of Contents
What is Yul Programming? And Why Should You Use It?
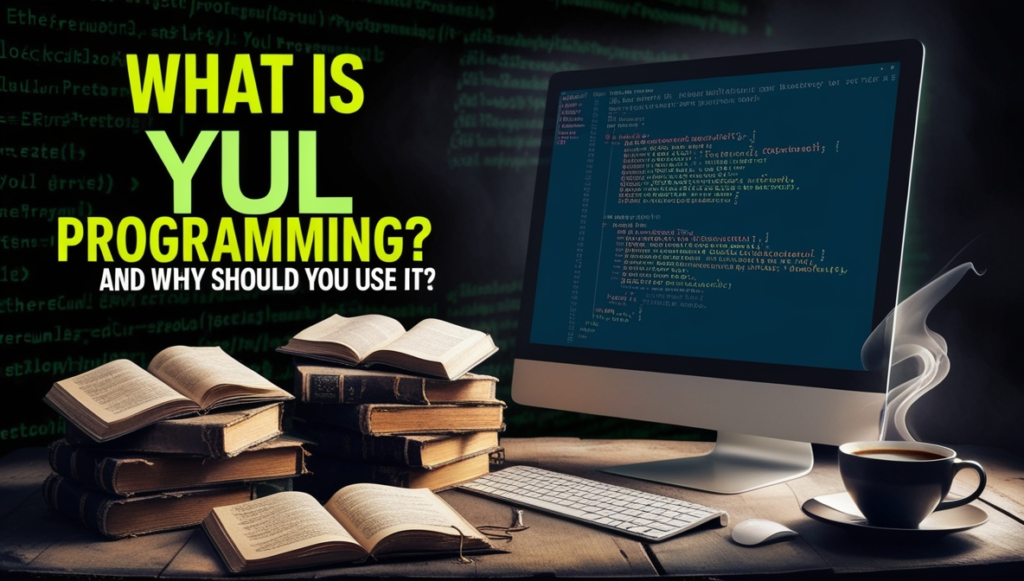
In the world of Ethereum development, Yul is a unique, low-level programming language that serves as an intermediate step between high-level programming languages like Solidity and the Ethereum Virtual Machine (EVM).
While Solidity is great for most smart contract development, it’s not always the most efficient for specific tasks. That’s where Yul comes in. It allows developers to write code that’s closer to the underlying machine level, giving you more control over how your smart contracts execute on the Ethereum network. This can lead to faster execution times and lower gas costs.
Even if you’re a beginner, learning Yul programming can enhance your understanding of Ethereum’s inner workings and help you optimize your smart contracts for better performance. It’s a powerful tool that can take your Solidity projects to the next level!
Understanding the Ethereum Virtual Machine (EVM)
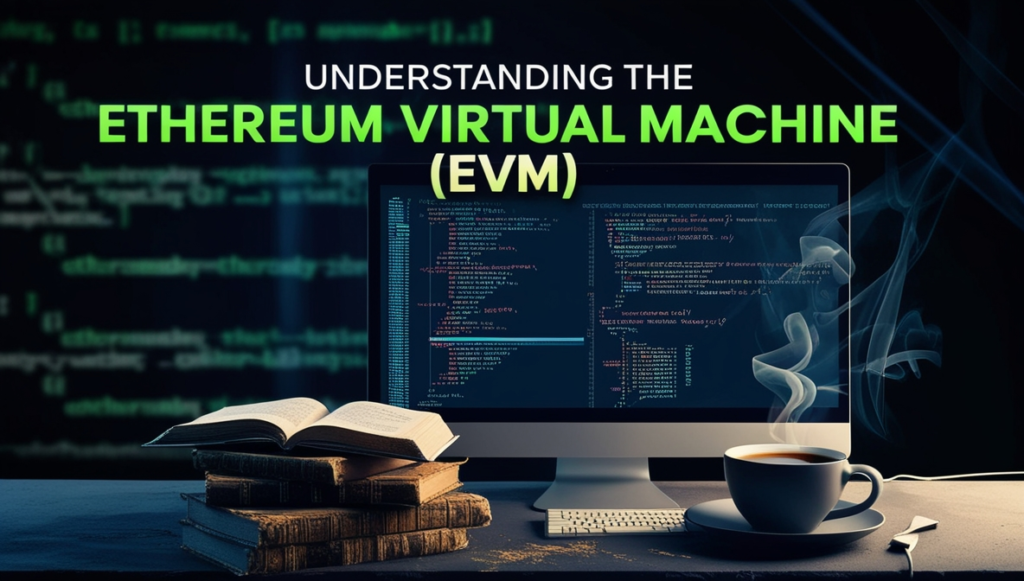
Before we get into Yul programming, it’s important to understand the Ethereum Virtual Machine (EVM). The EVM is essentially the decentralized computer that runs the Ethereum network. It processes the bytecode of smart contracts and ensures they execute properly across the Ethereum blockchain.
When you write a Solidity smart contract, it’s eventually compiled down to bytecode — the language the EVM understands. However, sometimes Solidity’s bytecode might be large and inefficient
With Yul programming, developers can write more efficient, low-level code that interacts directly with the EVM. This reduces inefficiencies, helping you create more optimized contracts that consume less gas and execute faster.
The Role of Low-Level Programming in Solidity
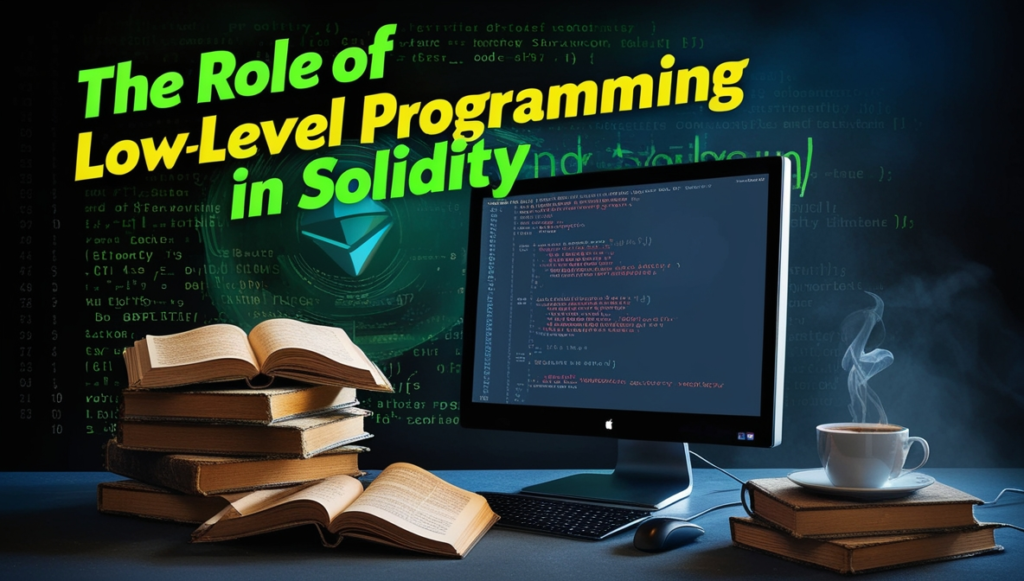
In Solidity, you work with higher-level abstractions like functions, variables, and complex data structures. While this makes writing code easier, these abstractions aren’t always efficient. Low-level programming allows you to manipulate memory and storage directly, offering more control over how data is handled during contract execution.
This is where Yul programming becomes crucial. By writing Yul assembly code, you gain the ability to control how your smart contract interacts with the EVM’s stack and memory, resulting in optimized and cost-effective code.
Here are a few basic concepts in low-level programming:
- Memory: Temporary storage used during contract execution.
- Storage: Permanent storage on the Ethereum blockchain.
- Stack: Temporary storage used for computations during execution.
By using Yul alongside Solidity, you can reduce the gas fees of your smart contract and make your application much more efficient.
Advancing Your Solidity Skills with Yul
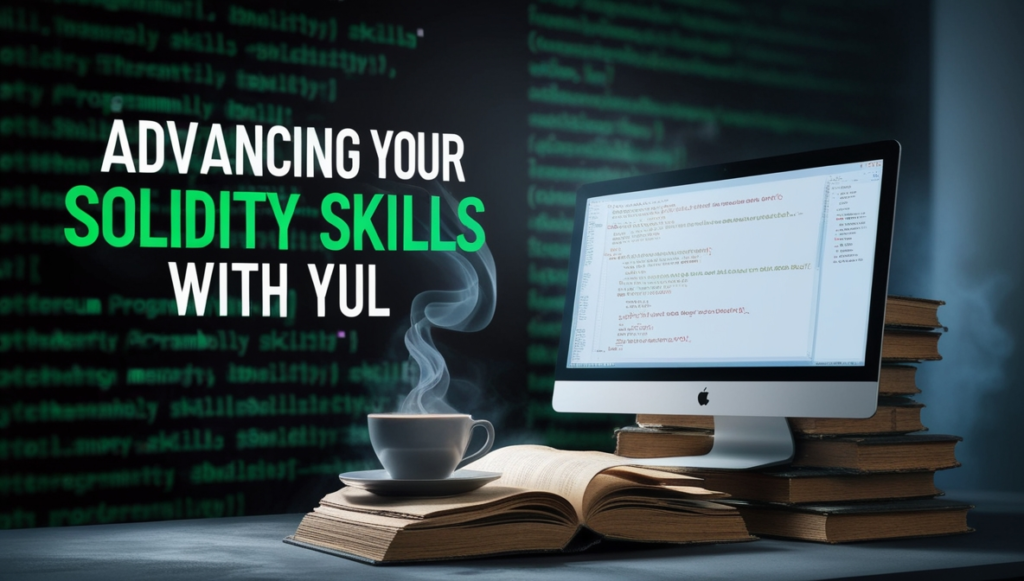
As you become more comfortable with Solidity, you’ll start exploring advanced Solidity topics. This is where Yul programming really shines. By leveraging Yul assembly, you can write highly optimized smart contracts that outperform others in terms of gas efficiency and execution speed.
Here’s a basic example of how Yul programming can optimize gas usage:
contract Optimized {
function optimizedFunction() public pure returns (uint) {
assembly {
let result := add(5, 3)
return(result, 32)
}
}
}
In this example, we’ve used Solidity assembly programming within a Solidity contract to perform a simple addition operation. This method reduces complexity and gas usage, making it more efficient than using standard Solidity syntax.
If you’re looking to dive deeper, check out Yul programming tutorials to learn more about memory management, gas optimization, and advanced EVM operations.
What Makes Solidity Yul Assembly Programming So Powerful?
Let’s take a closer look at Solidity Yul Assembly, and why it’s such a game-changer for Ethereum developers.
Yul assembly is a low-level programming language that directly interacts with the EVM’s stack and memory. It allows developers to write more efficient and optimized code, which leads to lower gas costs and faster execution.
Some benefits of using Yul include:
- Gas Optimization: Writing code that directly manipulates memory and storage can significantly reduce the gas used in smart contract execution.
- Flexibility: You can write functions and logic that would be too complex or inefficient to achieve with higher-level Solidity code.
- Direct Memory Access: By using Yul, you can directly manipulate data, which gives you better control over the EVM and how it runs your code.
Mastering Yul programming gives you the tools to write faster, more efficient smart contracts for Ethereum.
Your First Yul Programming Tutorial
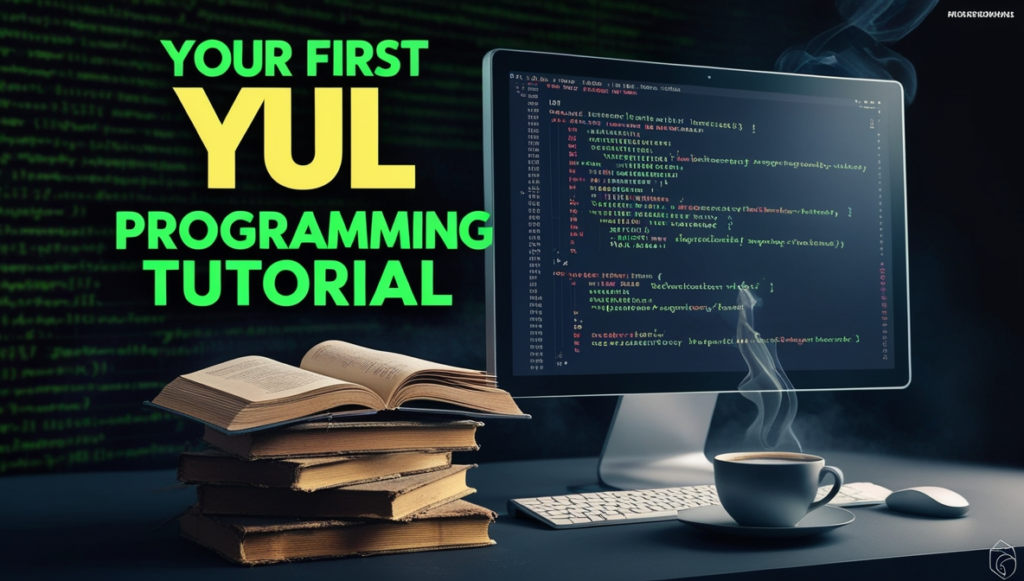
If you’re just getting started with Yul programming in Solidity, here’s a beginner-friendly tutorial to help you learn the basics. We’ll walk through how to set up your environment and write a simple Yul function.
- Set Up Your Development Environment First, you’ll need a few tools:
- Solidity compiler (Solc)
- Foundry or Hardhat for contract development and testing
- Write Your First Yul Function Let’s write a basic Yul function within a Solidity contract:
pragma solidity ^0.8.0;
contract SimpleYulExample {
function addNumbers(uint a, uint b) public pure returns (uint) {
assembly {
let result := add(a, b)
return(result, 32)
}
}
}
This simple function adds two numbers using Solidity Yul assembly. Notice how we use the assembly block to directly interact with the EVM stack, performing the addition operation. This is a more efficient way of executing simple logic than using higher-level Solidity syntax.
- Test Your Contract After writing your contract, you can test it using Truffle or Hardhat. These frameworks make it easy to deploy and test Solidity smart contracts that use Yul assembly.
Why Should You Learn Yul Programming?
Learning Yul programming is an invaluable skill for any Ethereum developer. Whether you want to optimize your Solidity smart contracts or explore the inner workings of the EVM, mastering Yul will allow you to create more efficient and cost-effective decentralized applications.
By following this Yul programming tutorial, you’re on your way to becoming an expert in Solidity Yul assembly. As you gain hands-on experience, you’ll be able to craft gas-efficient smart contracts that outperform others on the Ethereum blockchain.
So, keep practicing, dive deeper into Yul programming resources, and explore advanced topics like memory management, gas optimization, and EVM operations. With the right tools and knowledge, you’ll unlock the true potential of Ethereum smart contract development!
Resources to Keep Learning
To continue your journey into Yul programming, check out the following resources:
- Yul documentation
- Yul programming tutorials
- Yul programming software
- Yul programming PDFs
These materials will help deepen your understanding of Solidity Yul assembly and help you write smart contracts that are fast, efficient, and optimized for Ethereum.