Control Structures in Yul: When diving into low-level programming within the Ethereum Virtual Machine (EVM), one of the most powerful tools at your disposal is Yul. Known for its simplicity and efficiency, Yul is an intermediate language designed to maximize performance while offering flexibility for developers. One of Yul’s most critical aspects is its control structures, which are fundamental for managing the flow of execution. In this article, we’ll delve deep into conditional statements (if), loops (for, while), and error handling (revert), providing an insightful and easy-to-understand guide for both beginners and advanced users.
Table of Contents
What is Yul?
Yul is a low-level, intermediate language often used with Solidity for writing inline assembly or creating optimized smart contracts. Unlike high-level languages, Yul offers direct access to EVM opcodes, making it ideal for scenarios where performance is crucial. If you’ve worked with Solidity and are curious about the Yul language, this article will bridge the gap for you.
Why Learn Yul?
Yul allows developers to:
- Optimize gas costs: By offering finer control over operations, Yul enables efficient contract execution.
- Simplify debugging: Yul’s concise syntax makes it easier to pinpoint issues.
- Leverage low-level control: Unlike Solidity, Yul grants direct access to EVM operations.
If you’re diving into advanced Solidity assembly or working on gas-sensitive contracts, understanding control structures in Yul is indispensable.
Conditional Statements in Yul
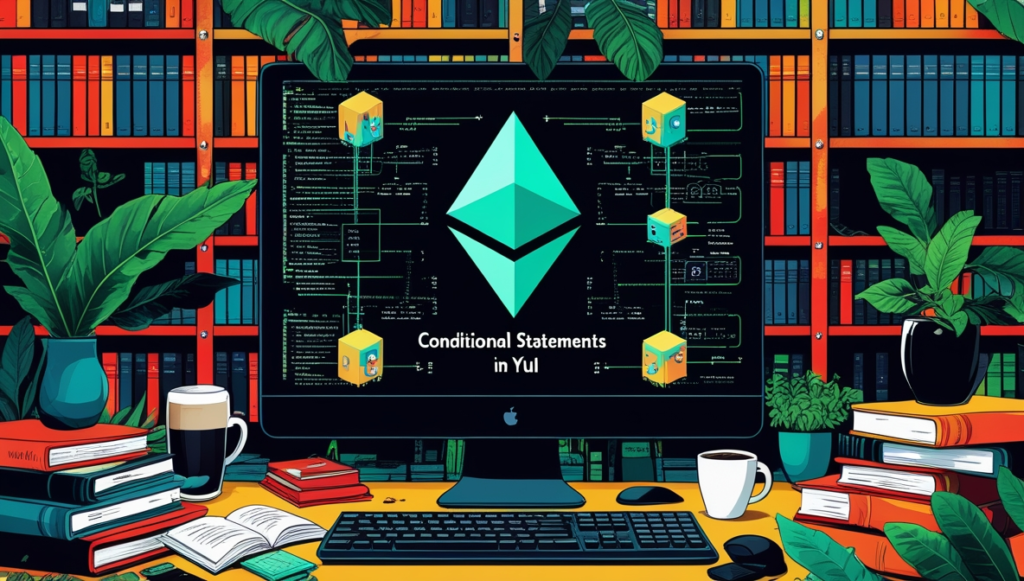
Conditional statements in Yul are straightforward yet powerful. They enable you to make decisions during runtime, offering precise control over program execution.
Syntax of If Statements in Yul
In Yul, the if
statement follows this format:
if condition {
// code to execute if condition is true
}
Example of an If Statement
Let’s consider a scenario where you check if a number is positive:
{
let number := 10
if gt(number, 0) {
// This block executes because 10 is greater than 0
sstore(0x0, number)
}
}
Key Notes:
- Yul doesn’t support
else
. You can mimic it using nested or multipleif
conditions. - Conditions use EVM opcodes like
lt
(less than),gt
(greater than), oreq
(equal).
Advanced Conditional Statements in Yul
For complex conditions, you can combine multiple expressions:
if and(gt(x, 0), lt(x, 100)) {
// Code executes if x is between 0 and 100
}
Loops in Yul Assembly
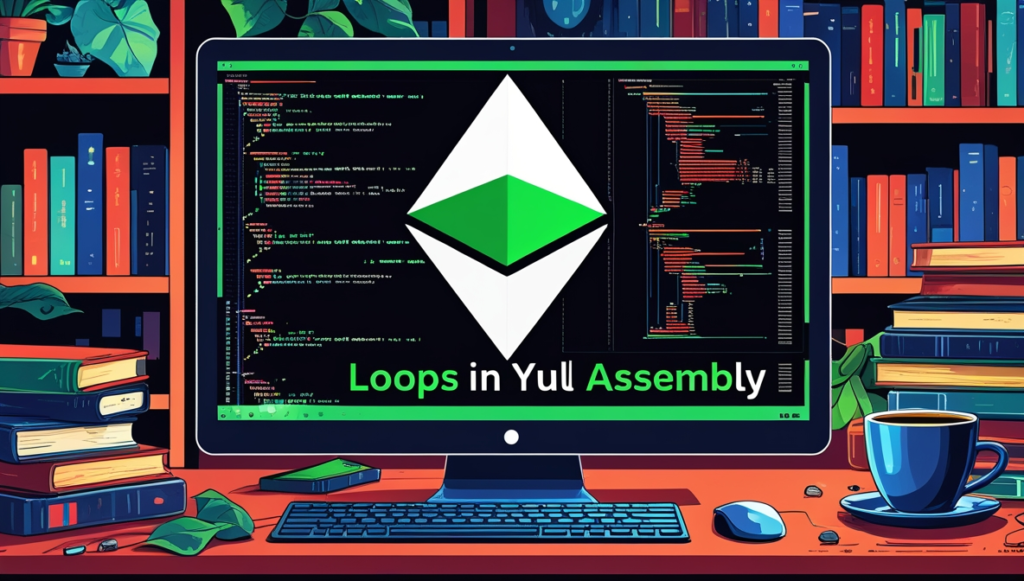
Loops are essential in programming for repeating tasks. Yul supports two types of loops: for and while.
For Loops in Yul
A for
loop is ideal when you know the exact number of iterations.
Syntax:
for { initialization } condition { post-loop operation } {
// code to execute
}
Example :
{
for { let i := 0 } lt(i, 10) { i := add(i, 1) } {
sstore(i, mul(i, 2))
}
}
Here, the loop initializes i
to 0
, checks if i < 10
, increments i
by 1, and stores the double of i
in storage.
While Loops in Yul
The while
loop is used when the number of iterations isn’t predetermined.
Syntax:
while condition {
// code to execute
}
Example:
{
let i := 0
while lt(i, 5) {
sstore(i, i)
i := add(i, 1)
}
}
This loop continues until i
is no longer less than 5.
Error Handling in Yul Assembly
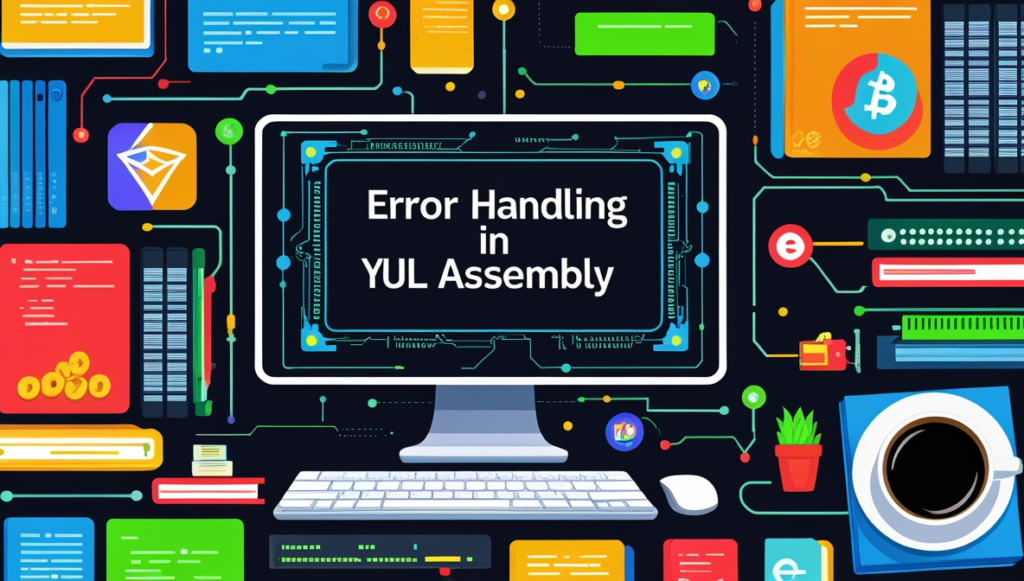
Errors are inevitable in programming, and handling them effectively is critical. Yul uses the revert
statement for error handling, which halts execution and reverts any state changes made during the transaction.
Revert Syntax in Yul
revert(memoryPointer, memorySize)
Example of Revert
{
let x := -1
if lt(x, 0) {
revert(0, 0) // Reverts if x is negative
}
}
Throwing Error Strings in Yul
You can use memory to store and return error strings.
{
let error := "Invalid input"
mstore(0x0, error)
revert(0x0, 32) // Returns the error message
}
This approach is especially useful when debugging or providing meaningful error messages.
Exploring Control Structures in Solidity Yul
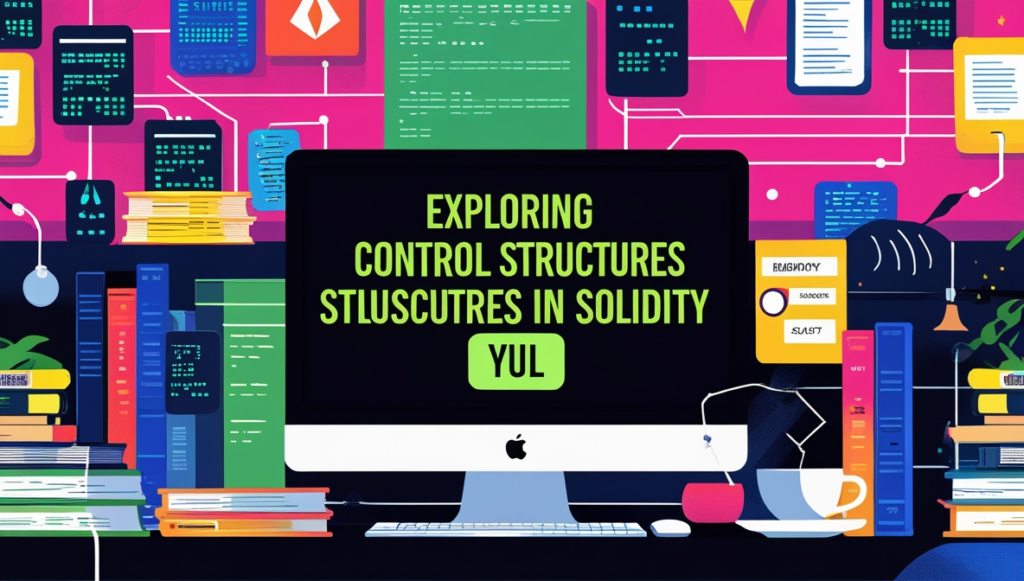
When combining Yul control structures with Solidity, inline assembly can provide powerful optimizations. Here’s an example that combines if
, for
, and revert
:
{
let x := 0
for { let i := 0 } lt(i, 10) { i := add(i, 1) } {
if eq(i, 5) {
revert(0, 0) // Stops execution when i equals 5
}
x := add(x, i)
}
sstore(0x0, x)
}
This snippet demonstrates the integration of conditional checks, looping, and error handling.
Best Practices for Yul Programming
- Write Clean Code: Use meaningful variable names and avoid overly nested structures.
- Optimize Gas Usage: Reuse variables and avoid unnecessary memory allocations.
- Test Extensively: Use Solidity’s test frameworks to validate your Yul code.
- Refer to Documentation: The Yul Solidity documentation is a great resource for exploring advanced features.
Frequently Asked Questions (FAQs)
Q1: What is Yul used for in Solidity?
Yul is used as an intermediate language for writing optimized EVM bytecode, often through inline assembly in Solidity.
Q2: How do I handle errors in Yul?
Errors in Yul are handled using the revert
statement, which halts execution and rolls back any state changes.
Q3: What are the benefits of using Yul?
Yul provides finer control over contract execution, reduces gas costs, and simplifies debugging compared to high-level languages.
Q4: Can I use if-else
in Yul?
Yul doesn’t natively support else
. You can achieve similar functionality using additional if
statements.
Q5: Where can I learn more about Yul?
Check the Yul Solidity documentation or online resources dedicated to low-level Solidity assembly.
Conclusion
Understanding control structures in Yul is vital for developers seeking to optimize their Ethereum smart contracts. By mastering conditional statements (if), loops (for, while), and error handling (revert), you can unlock the full potential of the Yul language. Whether you’re debugging complex logic or squeezing out the last bit of performance, Yul offers unparalleled flexibility and power.
For those new to Solidity assembly, this guide should provide a strong foundation. Keep experimenting, stay updated with Yul documentation, and remember: practice makes perfect!