Master Event Logging in Solidity: When you’re building smart contracts in Solidity, event logging is a powerful tool that can take your contracts to the next level. Events are like messages broadcasted from the blockchain that off-chain applications can listen to. They’re vital for debugging, tracking transactions, and creating a bridge between your decentralized application (DApp) and the real world.
In this chapter, we’ll take a deep dive into event logging and emitting events in Solidity, explaining what they are, why they matter, and how to use them effectively. Whether you’re a beginner or brushing up your skills, this guide will provide step-by-step clarity with practical examples and insights.
Table of Contents
What Are Events in Solidity?
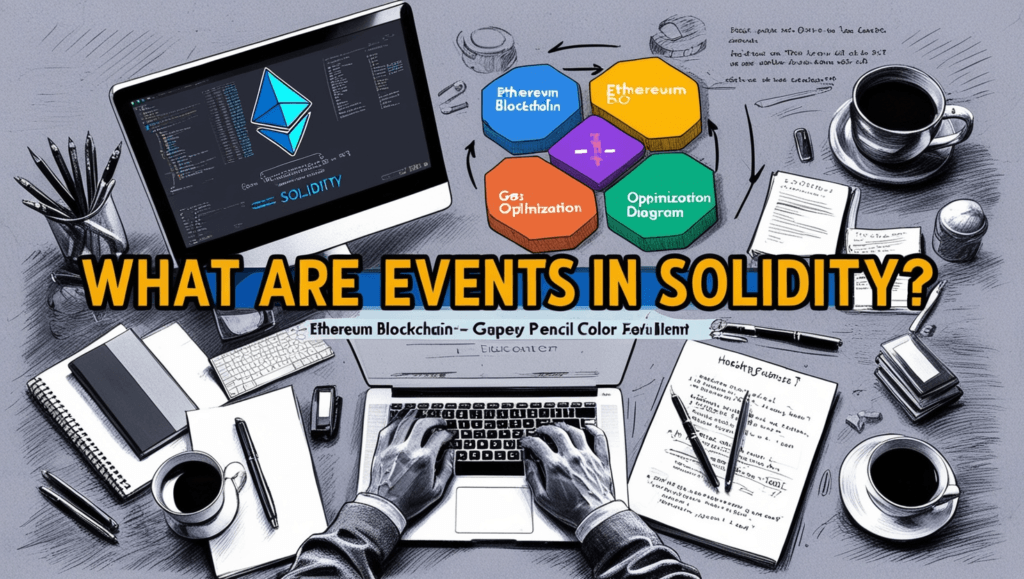
In Solidity, events are interfaces that enable smart contracts to communicate with the outside world. When an event is triggered in your contract, the corresponding log is stored on the blockchain, and off-chain tools (like a Web3.js application) can listen and respond to these logs.
Think of events as a notification system for the blockchain:
- The contract emits an event when something important happens (e.g., a transfer of tokens).
- This event can then be picked up by applications like Ethers.js, The Graph, or even custom front-end interfaces.
Why Are Events Important?
- Gas Efficiency: Events are stored in the blockchain’s log section, which is cheaper in terms of gas compared to state variables.
- Debugging: Events can help developers trace the flow of execution and identify bugs.
- Transparency: Users can verify actions taken by the contract, enhancing trust.
- DApp Integration: Events are the glue between your smart contract and your DApp’s user interface.
Declaring Events in Solidity
Defining an event in Solidity is simple. Here’s the syntax:
event EventName(DataType indexed variable1, DataType variable2, ...);
event
: The keyword to define an event.indexed
: Allows filtering of specific event parameters, making it easier to find specific logs.- Parameters: These are the data fields you want to include in the event.
Example: Basic Event Declaration
Here’s an example of declaring and emitting an event in a Solidity contract:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.24;
contract EventExample {
// Declare an event
event LogDeposit(address indexed sender, uint256 amount, string message);
// A function to emit the event
function deposit(string memory message) public payable {
require(msg.value > 0, "You must send some ether!");
// Emit the event
emit LogDeposit(msg.sender, msg.value, message);
}
}
Breaking Down the Example
- Event Declaration:
LogDeposit
: The name of the event.address indexed sender
: The sender’s address, indexed for easier filtering.uint256 amount
: The value of ether sent.string message
: An optional message.
- Emit the Event:
- Inside the
deposit
function, we use theemit
keyword to trigger the event. - This event gets logged on the blockchain, and any off-chain listener can respond to it.
- Inside the
How to Listen to Events Off-Chain
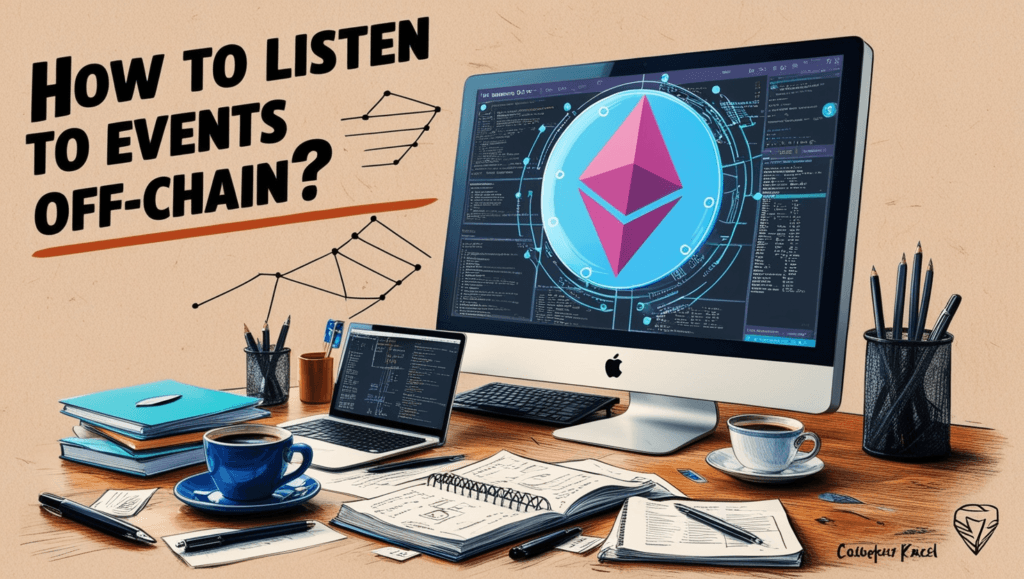
After emitting an event in Solidity, we need to capture it off-chain using tools like Ethers.js or Web3.js.
Example: Listening to Events with Ethers.js
const contract = new ethers.Contract(contractAddress, abi, provider);
contract.on("LogDeposit", (sender, amount, message) => {
console.log(`Sender: ${sender}`);
console.log(`Amount: ${ethers.utils.formatEther(amount)} ETH`);
console.log(`Message: ${message}`);
});
In this example:
- We subscribe to the
LogDeposit
event using Ethers.js. - Whenever the event is emitted, the callback function captures the details.
Indexed Parameters: Filtering Events
Solidity allows up to three indexed parameters per event. These indexed parameters make it possible to filter logs by specific values.
Example: Using Indexed Parameters
event LogTransfer(address indexed from, address indexed to, uint256 amount);
function transfer(address to, uint256 amount) public {
emit LogTransfer(msg.sender, to, amount);
}
Off-Chain Filtering with Web3.js
const logs = await contract.getPastEvents("LogTransfer", {
filter: { from: userAddress },
fromBlock: 0,
toBlock: "latest",
});
console.log(logs);
Best Practices for Logging Events
- Limit Indexed Parameters: Use indexed parameters wisely, as they incur additional gas costs.
- Avoid Overlogging: Only log essential data to minimize storage and costs.
- Use Events for External Communication: Don’t rely on events for internal contract logic.
- Include Meaningful Data: Make sure your events are informative and descriptive.
Debugging Solidity with Events
Events can act as bread crumbs during the debugging process. By emitting events at strategic points in your contract, you can trace the flow of execution.
Example: Debugging with Events
event Debug(string message, uint256 value);
function debugExample(uint256 input) public {
emit Debug("Function entered", input);
uint256 result = input * 2;
emit Debug("After calculation", result);
}
Output Example
When you call debugExample(5)
, you’ll get logs like:
"Function entered": 5
"After calculation": 10
This approach helps pinpoint where issues occur in your contract.
Advanced Use: Emitting Events in Proxies
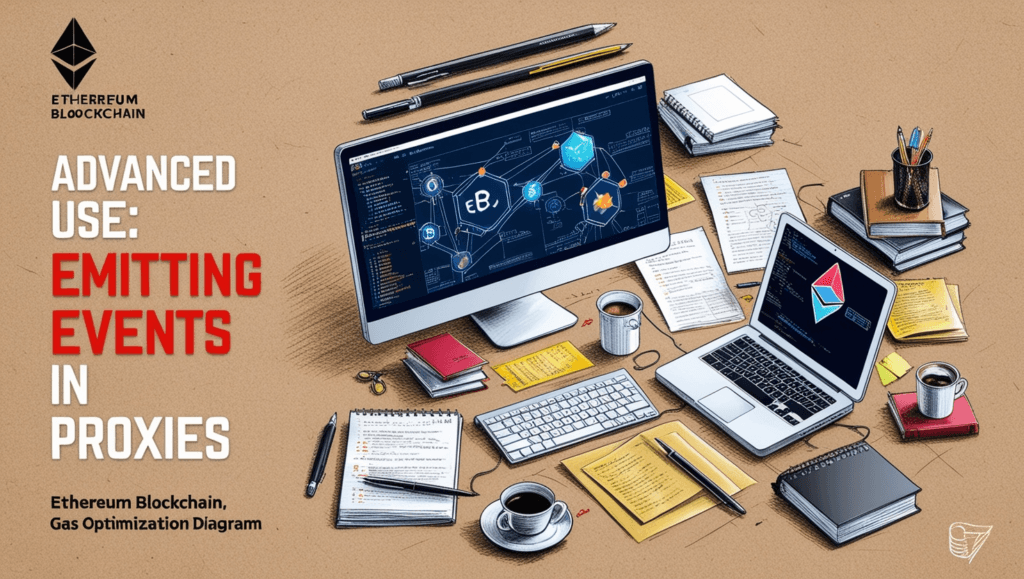
When using proxy contracts (e.g., for upgradable smart contracts), events play a critical role in monitoring the interaction between the proxy and implementation contracts.
Example: Transparent Proxy with Events
event Upgraded(address indexed newImplementation);
function upgradeTo(address newImplementation) public {
emit Upgraded(newImplementation);
}
Benefits of Emitting Events in Proxies
- Tracks upgrades for accountability.
- Helps users verify which implementation contract is active.
Gas Optimization for Events
Logging events is cheaper than using state variables, but it’s not free. To optimize gas usage:
- Limit Data Size: Use shorter data types (e.g.,
uint8
instead ofuint256
where applicable). - Avoid Unnecessary Logs: Log only what’s needed for off-chain use.
- Batch Emissions: Combine related data into a single event if possible.
Complete Example: Practical Use Case
Let’s combine everything into a more practical example:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.24;
contract TokenSale {
event TokensPurchased(address indexed buyer, uint256 amount, uint256 cost);
event SaleEnded(address indexed owner, uint256 totalSales);
address public owner;
uint256 public pricePerToken;
uint256 public totalSales;
modifier onlyOwner() {
require(msg.sender == owner, "Not the owner!");
_;
}
constructor(uint256 _pricePerToken) {
owner = msg.sender;
pricePerToken = _pricePerToken;
}
function buyTokens(uint256 tokenAmount) public payable {
uint256 cost = tokenAmount * pricePerToken;
require(msg.value >= cost, "Insufficient Ether sent!");
totalSales += tokenAmount;
emit TokensPurchased(msg.sender, tokenAmount, cost);
}
function endSale() public onlyOwner {
emit SaleEnded(owner, totalSales);
}
}
Conclusion
Event logging in Solidity is essential for building robust and user-friendly smart contracts. From debugging to enhancing the user experience, mastering events gives you a powerful tool to interact with the blockchain efficiently.
Key takeaways:
- Use events for off-chain communication and debugging.
- Optimize event usage to save on gas costs.
- Always test event emissions with tools like Hardhat or Ethers.js to ensure reliability.
By following best practices and experimenting with examples, you’ll soon be an expert in handling event logging in Solidity. Keep learning, stay curious, and make your smart contracts truly exceptional!