Solidity Tutorial Chapter 13: Welcome to Chapter 13! Here, we’re going to dive into one of the most fascinating aspects of Solidity: events and logging. This is essential for making our smart contracts not only transparent but also more user-friendly. If you’ve ever wondered how to keep track of actions in your smart contract or why events are even needed, you’re in the right place. We’ll walk through how Solidity events work, why they matter, and how to use them to make sure every action is logged and easy to follow. Let’s get started!
Table of Contents
What Are Solidity Events?
In Solidity, events are a way to log specific actions or data. Think of events as a smart contract’s way of saying, “Hey, something just happened, and here are the details!” When a transaction triggers an event, it records specific information on the blockchain. This can be incredibly useful for tracking data changes, debugging, or creating more interactive applications, as external applications can “listen” to these events and respond.
Why Are Events Important?
Events aren’t just for tracking purposes; they’re also a key part of how decentralized applications (DApps) interact with smart contracts. Here’s why they’re valuable:
- Transparency: Every event is logged on the blockchain, meaning anyone can view it. This helps make your DApp or contract more trustworthy.
- Efficiency: Events are cheaper to store than regular data. Since they’re recorded on a separate area of the blockchain, they don’t consume as much gas.
- Interactivity: With events, you can enable real-time interactions with DApps, notifying users or triggering external applications based on smart contract actions.
Real-Life Example: Tracking Purchases in a Marketplace
Imagine you’re running a blockchain-based marketplace where users buy and sell products. You want to track when a purchase is made. An event would let you log each transaction, including buyer and seller info, product details, and price. With an event, you’d get a snapshot of the transaction data in real time, which could then be used by the UI to notify both the buyer and seller.
Setting Up Your First Solidity Event
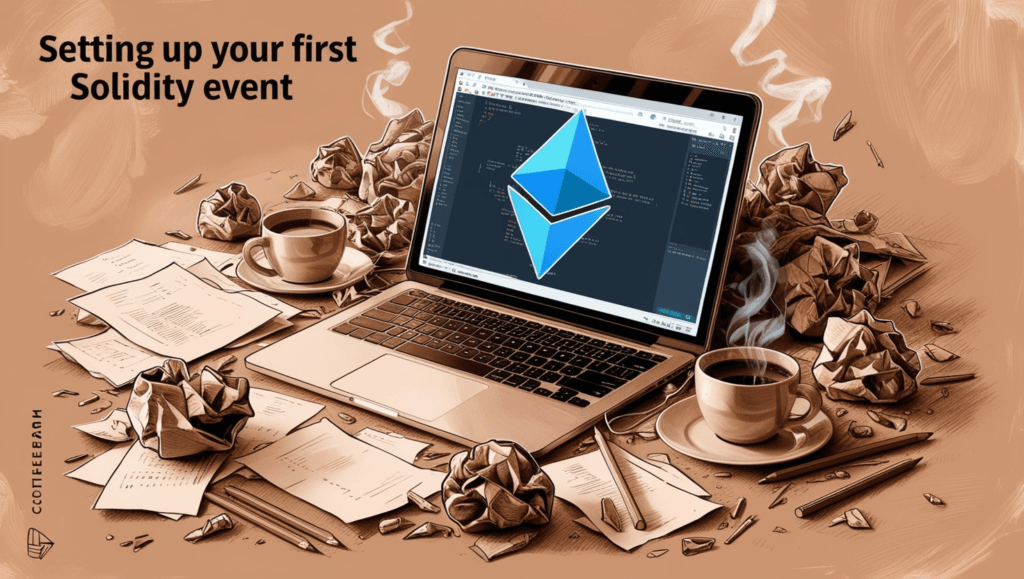
Let’s break down the steps for creating a basic event in Solidity. We’ll keep it simple for now.
- Define the Event: First, we declare an event in our contract.
- Emit the Event: When a specific action occurs (like a function being called), we emit the event to log the details.
Here’s how it would look:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract Marketplace {
// Define the event
event PurchaseMade(address buyer, uint productId, uint price);
// Function that triggers the event
function buyProduct(uint _productId, uint _price) public {
// Emit the event
emit PurchaseMade(msg.sender, _productId, _price);
}
}
In this example, whenever a product is purchased, the PurchaseMade event is emitted. This event includes the buyer’s address, the product ID, and the price, giving us a quick summary of each purchase.
Breaking Down the Code
Defining the Event
- Events are declared outside functions, just like variables. In our example, the event is named
PurchaseMade
. - Parameters inside the event (such as
address buyer
,uint productId
, anduint price
) tell us what information will be stored when the event is emitted.
Emitting the Event
When the buyProduct
function is called, it emits the PurchaseMade
event, which records the data passed to it. By emitting the event, we’re essentially sending a message to the blockchain that something important has happened.
Keyword Optimization: How Events Work in Solidity
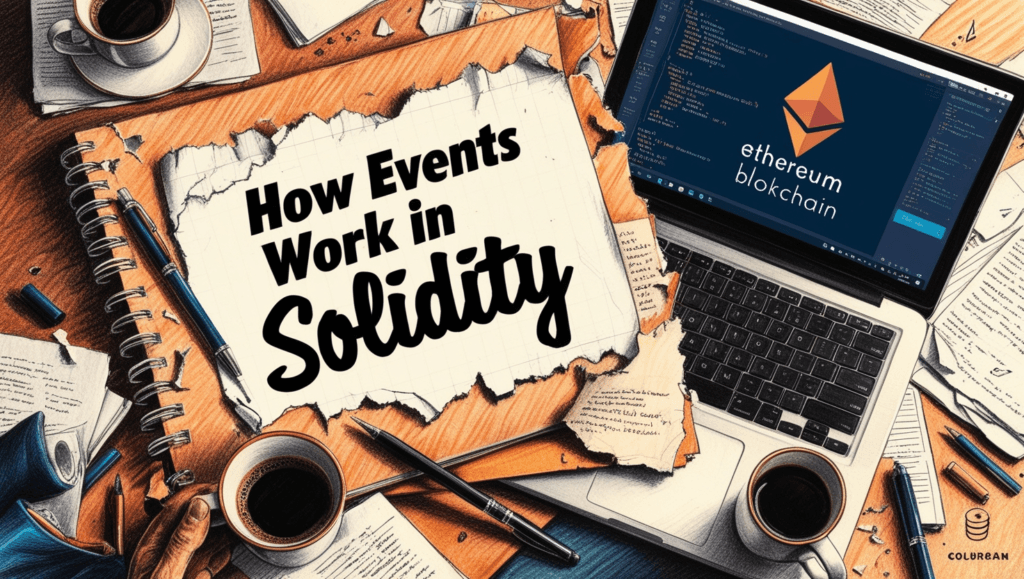
Using events in Solidity effectively is key for writing optimized, transparent smart contracts. Events play a crucial role in real-world blockchain applications, helping developers create responsive and secure smart contracts. When we use events to keep users informed of blockchain transactions, we’re making Solidity contracts more interactive and user-friendly.
Making Events Work for You: Indexed Parameters
In Solidity, you can use up to three indexed parameters in an event. Indexed parameters allow you to filter events by specific values, making it easier to search for particular actions.
Here’s how to make some parameters indexed in our example:
event PurchaseMade(address indexed buyer, uint productId, uint price);
With address indexed buyer
, you can later filter events to find all purchases made by a particular buyer. This is especially useful for DApps with a lot of users or transactions, as it allows you to sift through the data more efficiently.
Practical Use Case: Real-Time Notifications
Let’s go back to our marketplace example. Suppose you want to notify the seller whenever a product is sold. By listening to the PurchaseMade event from an external application, you can send the seller an alert in real time. This creates a better user experience, as the seller doesn’t need to check the blockchain manually to see if a sale was made.
Using Events in External Applications
Here’s how external applications interact with Solidity events:
- Listen to the Event: Applications like a DApp frontend can listen for specific events using Web3.js or Ethers.js.
- Respond in Real Time: When the event is detected, the application can trigger an action, like updating a user’s dashboard or sending a notification.
// JavaScript example using Web3.js
const contractInstance = new web3.eth.Contract(contractABI, contractAddress);
contractInstance.events.PurchaseMade({
filter: { buyer: userAddress }, // Optional filtering
fromBlock: 0
}, (error, event) => {
if (!error) {
console.log("Purchase Event Detected:", event);
}
});
With this code, the frontend is listening for PurchaseMade
events. When it detects one, it can then react to it, such as by updating the UI or alerting the user.
Common Mistakes with Solidity Events
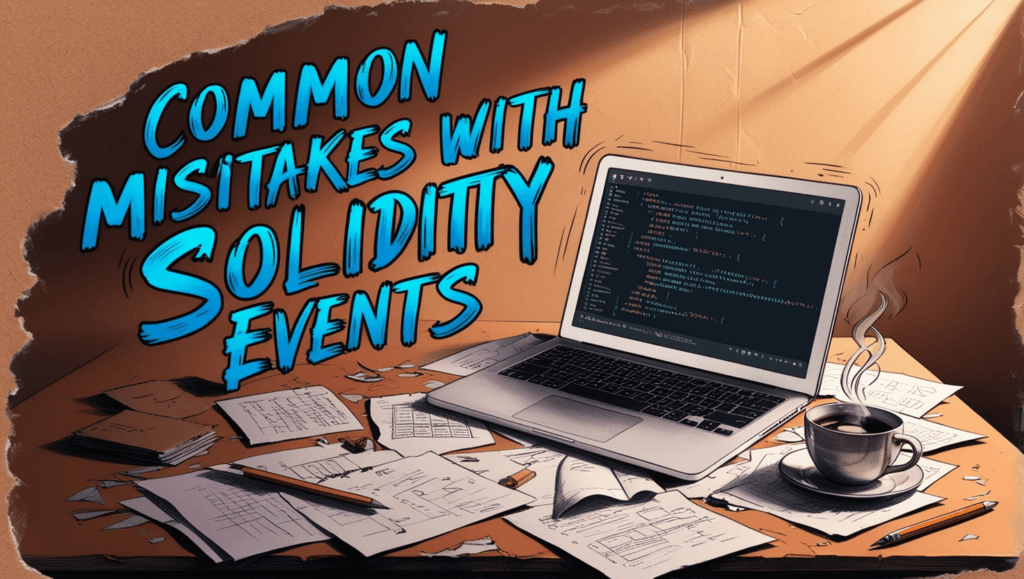
- Overusing Events: Since events cost gas, try to emit them only for significant actions.
- Forgetting Indexed Parameters: If you need to filter events, make sure to index the necessary parameters.
- Misplacing the Event: Be sure to emit the event only after the transaction is confirmed (for example, after payment is received in a marketplace).
Summary: Why Events Make Solidity Better
In this chapter, we’ve covered how Solidity events work, why they’re essential, and how you can use them in real-world scenarios. Events allow you to track and log important actions in a smart contract—from marketplace sales to fund transfers—adding transparency and enhancing user experience. They also provide a unique way to make your contracts more interactive and efficient, a win for both developers and users.
Key Takeaways
- Events are your tool for logging actions within a Solidity contract.
- Emitting events records information, making your contract more transparent.
- Indexed parameters allow you to filter events for more efficient searches.
- External applications can listen to events and respond in real time, enhancing interactivity.
With a solid grasp of Solidity events and how to apply them, you’re ready to take your smart contract skills to the next level. Stay tuned for the next chapter, where we’ll cover more essential topics to boost your Solidity knowledge!
Wrapping Up Chapter 13
In this chapter, we’ve broken down how to use events in Solidity to create transparent, efficient logs of contract activity. Events serve as a bridge between your smart contract and the outside world, providing a way for users and DApps to stay updated with what’s happening in your contract.
Next up, we’ll dive into Error Handling and Require Statements to help you build more resilient, reliable smart contracts. Keep up the great work! You’re moving closer to mastering Solidity every step of the way.
NOTE : FOR MORE DEEPER INFORMATION WITH PRACTICAL USE CASES IN UPCOMING TUTORIAL ABOUT EVENT AND LOGGING