Solidity Tutorial Chapter 12: Expert Guide to Inheritance in Solidity for Efficient Smart Contracts Welcome to Chapter 12 of our Solidity Tutorial series! Today, we’re diving into a crucial concept that makes smart contracts more manageable, maintainable, and reusable – Inheritance in Solidity. This is a core feature that enables you to build complex systems efficiently by reusing code across different smart contracts.
So, why is inheritance such a game-changer for Solidity developers? Let’s jump into it and explore how Solidity inheritance works, some best practices, and how it can boost your smart contract development journey!
Table of Contents
What is Inheritance in Solidity?
Inheritance in Solidity allows you to create new contracts based on existing ones. Think of it like creating a “template” contract with common functions and properties, which other contracts can then use, modify, or expand. It’s one of the most efficient ways to write modular and scalable smart contracts, avoiding redundant code and promoting easier maintenance.
Inheritance essentially lets you define a base contract and then build upon it. When you inherit from a contract, all its variables, functions, and behaviors come along with it.
Real-Life Analogy: Inheritance in a Family Business
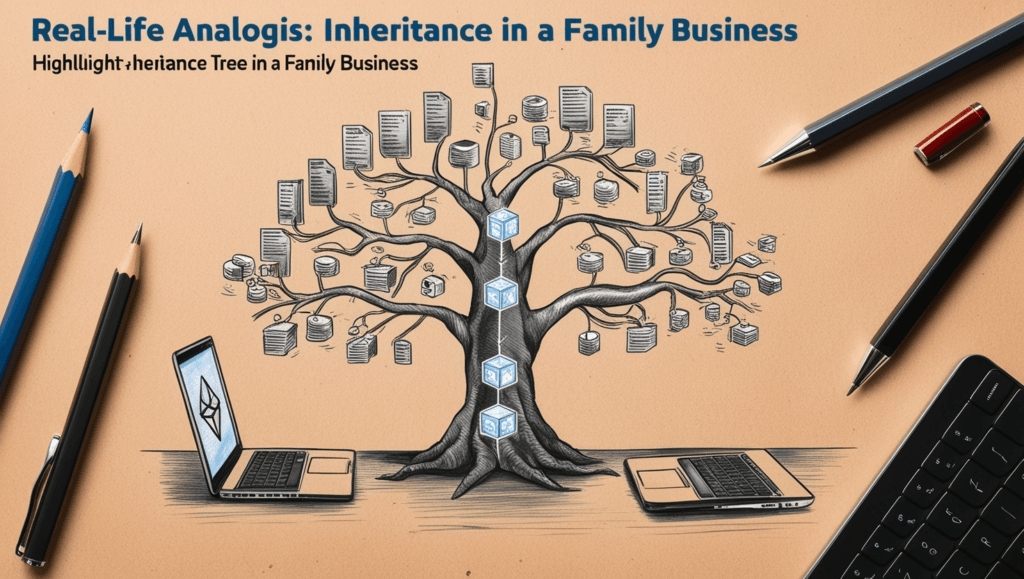
Imagine a family business that’s been handed down for generations. Let’s call it “The Smith Bakery.” The Smith family has been baking delicious pastries for years, and each generation inherits the recipe book and shop rules created by the founders. These “inherited” recipes and rules become the foundation for every new generation.
Similarly, in Solidity, when you create a new contract, it can inherit all the functions, variables, and properties from a base contract—just like inheriting recipes and rules in a family business. If you’re building a series of related contracts, inheritance helps you start with the basics and add new customizations, rather than building from scratch each time.
Why is Inheritance Important in Solidity?
Inheritance is more than just a “nice-to-have” feature. It allows you to:
- Reuse Code: Write functions once and use them across multiple contracts.
- Maintain Consistency: Use a single “base” contract that has the logic needed across all related contracts.
- Modularize Your Code: Break complex logic into smaller, manageable pieces, promoting code readability and maintainability.
Inheritance is the backbone of many decentralized applications (dApps) because it supports a structure that can grow and adapt over time.
Basic Syntax of Solidity Inheritance
To declare inheritance in Solidity, you use the is
keyword. Here’s a basic example to illustrate:
// Base contract
contract Animal {
function speak() public pure returns (string memory) {
return "Animal Sound";
}
}
// Derived contract
contract Dog is Animal {
function bark() public pure returns (string memory) {
return "Woof!";
}
}
In this example, the Dog
contract inherits from the Animal
contract. The Dog
contract now has access to the speak
function from the Animal
contract, alongside its own bark
function.
Types of Inheritance in Solidity
Solidity supports several types of inheritance, each useful in different scenarios. Let’s look at them in detail.
1. Single Inheritance
In single inheritance, a derived contract inherits from one and only one base contract. This is the simplest form of inheritance and is quite common in Solidity.
Example:
contract Parent {
function parentFunction() public pure returns (string memory) {
return "Parent function called";
}
}
contract Child is Parent {
function childFunction() public pure returns (string memory) {
return "Child function called";
}
}
2. Multiple Inheritance
In Solidity, you can inherit from multiple contracts using comma-separated base contracts. This enables you to access features from multiple contracts in one derived contract.
contract A {
function foo() public pure returns (string memory) {
return "Function from Contract A";
}
}
contract B {
function bar() public pure returns (string memory) {
return "Function from Contract B";
}
}
contract C is A, B {
// C can use functions from both A and B
}
With multiple inheritance, the C
contract inherits from both A
and B
, gaining access to foo
and bar
.
3. Multilevel Inheritance
In multilevel inheritance, contracts inherit in layers. A contract inherits from another derived contract rather than directly from the base contract.
contract Grandparent {
function grandparentFunction() public pure returns (string memory) {
return "Grandparent function";
}
}
contract Parent is Grandparent {
function parentFunction() public pure returns (string memory) {
return "Parent function";
}
}
contract Child is Parent {
// Child inherits from both Parent and Grandparent
}
Here, the Child
contract has access to both parentFunction
and grandparentFunction
by inheriting from Parent
, which in turn inherits from Grandparent
.
4. Hierarchical Inheritance
In hierarchical inheritance, multiple derived contracts inherit from a single base contract. This pattern is useful when you want to create several contracts with similar foundational behaviors.
contract Vehicle {
function move() public pure returns (string memory) {
return "Moving";
}
}
contract Car is Vehicle {
function drive() public pure returns (string memory) {
return "Driving a car";
}
}
contract Bike is Vehicle {
function ride() public pure returns (string memory) {
return "Riding a bike";
}
}
In this example, both Car
and Bike
inherit from Vehicle
, allowing both to use the move
function.
Overriding Functions in Solidity Inheritance
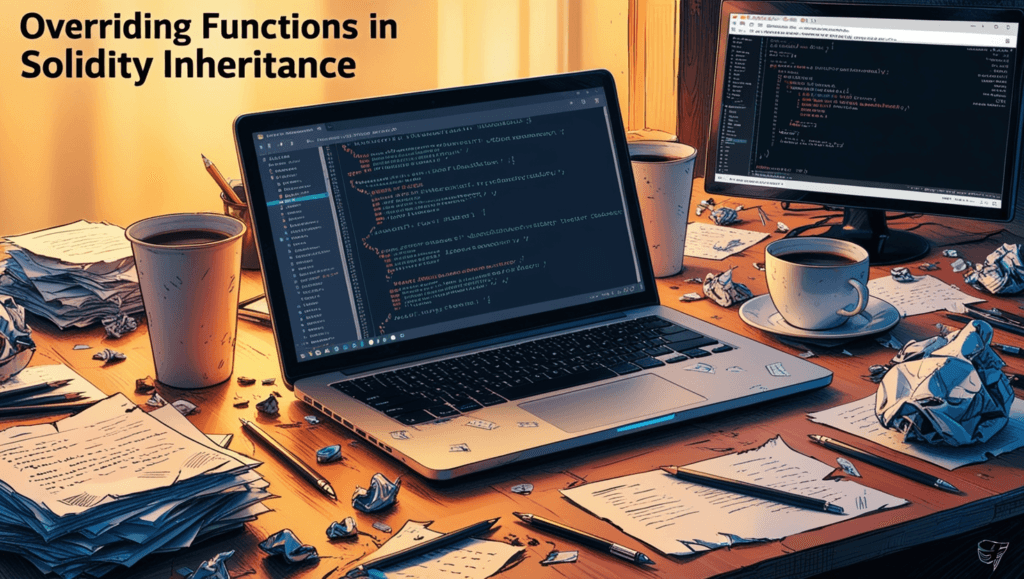
One powerful feature of Solidity inheritance is the ability to override functions. Suppose a derived contract needs to redefine a function inherited from a base contract. You can achieve this using the override
keyword.
Example:
contract Animal {
function sound() public virtual pure returns (string memory) {
return "Some sound";
}
}
contract Dog is Animal {
function sound() public override pure returns (string memory) {
return "Woof!";
}
}
Here, the Dog
contract overrides the sound
function in the Animal
contract. The override
keyword ensures that this version of sound
replaces the original version in any inherited context.
Important Note on Function Overriding
When overriding functions, remember:
- The base function must be marked as
virtual
. - The overriding function must use the
override
keyword.
These keywords are essential for clarity, helping Solidity understand that you’re purposefully changing the behavior of an inherited function.
Practical Tips for Using Inheritance in Solidity
- Avoid Deep Inheritance Chains: Long chains of inheritance can make contracts difficult to read, debug, and audit.
- Prefer Composition Over Inheritance: In many cases, combining smaller contracts (composition) is better than inheriting them, especially if you’re only using a small part of a base contract.
- Use
super
Wisely: When calling functions from a base contract, usesuper
to access the overridden function while preserving the derived function’s implementation. - Stay Organized: Use inheritance thoughtfully and avoid redundant or conflicting functions.
Example Use Case: Access Control with Inheritance
A popular application of inheritance is implementing access control. Suppose you have a BaseAccessControl
contract that defines roles, like admin and user. You can create specialized contracts that inherit these roles, adding extra functionality as needed.
contract BaseAccessControl {
address public admin;
constructor() {
admin = msg.sender;
}
modifier onlyAdmin() {
require(msg.sender == admin, "Not an admin");
_;
}
}
contract User is BaseAccessControl {
function sensitiveAction() public onlyAdmin {
// only admin can call this function
}
}
In this case, User
inherits the onlyAdmin
modifier from BaseAccessControl
, enforcing access control without redundant code.
Wrapping Up
Congratulations on mastering Solidity inheritance! We’ve covered the essentials, from basic syntax to advanced features like function overriding and modifier-based access control. Inheritance is a powerful tool that can streamline your smart contracts and make them more adaptable and maintainable. Whether you’re working on single or multi-level inheritance, this knowledge will make your code modular, reusable, and efficient.
In the next chapter, we’ll explore Abstract Contracts and Interfaces, which build upon inheritance to create even more flexible and reusable Solidity code.